Status
Open
Budget
15.00 USD
Payment Method
Direct Payment
Job Description
Hi,
In this Cbot when the RSI last value pass 70 or drop below 30 it open or close the the trade, I need to change it to when the candle stick is closed and then if the RSI value is over 70 or under 30 it open or close the trade.
Also the TP and SL is not working ,
Thanks
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class SampleRSIRobot : Robot
{
[Parameter("Source")]
public DataSeries Source { get; set; }
[Parameter("Periods", DefaultValue = 5)]
public int Periods { get; set; }
[Parameter("Stop Loss (pips)", DefaultValue = 100, MinValue = 1)]
public int StopLoss { get; set; }
[Parameter("Take Profit (pips)", DefaultValue = 100, MinValue = 1)]
public int TakeProfit { get; set; }
[Parameter("Volume", DefaultValue = 10000, MinValue = 1000)]
public int Volume { get; set; }
private RelativeStrengthIndex rsi;
protected override void OnStart()
{
rsi = Indicators.RelativeStrengthIndex(Source, Periods);
}
protected override void OnTick()
{
if (rsi.Result.LastValue < 30)
{
Close(TradeType.Sell);
Open(TradeType.Buy);
}
else if (rsi.Result.LastValue > 70)
{
Close(TradeType.Buy);
Open(TradeType.Sell);
}
}
private void Close(TradeType tradeType)
{
foreach (var position in Positions.FindAll("SampleRSI", Symbol, tradeType))
ClosePosition(position);
}
private void Open(TradeType tradeType)
{
var position = Positions.Find("SampleRSI", Symbol, tradeType);
if (position == null)
ExecuteMarketOrder(tradeType, Symbol, Volume, "SampleRSI");
}
}
}
Comments
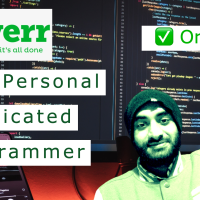
Hi,
hope you doing well. I'll develop RSI based EA/indicator for you at your affordability. would you like to have a connection with me. Feel free to contact me. we can have a discussion.
Regards
Nabeel
Email: privateforexdna@gmail.com
Fiverr:
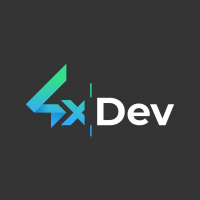
Hello!
My name is Mike from 4xdev. I am a member of the 4xdev development company, specialized in creating trading tools:
custom indicators, EAs, scripts, bots, alerts, cAlgo, cBots, etc.
We have a great experience in a сTrader platforms.
Our clients are satisfied with the quality of our work and repeatedly contact us after completing an order to optimize their own cAlgos.
You can see reviews of us at forexpeacearmy.com and trustpilot.com, and our website 4xdev.com.
We can help you with your project. Please let me know if you are interested (support@4xdev.com).
WhatsApp: +380508146716
Skype: live:.cid.285d85434983f29e
Telegram: https://t.me/Forexdev
We offer the rate in the amount of $40 per hour, including the work of our programmers and QA-engineer.
The cost starts from $150 on average.
Looking forward to hearing from you.
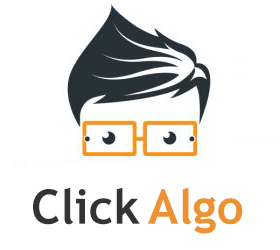
Hi there,
We can help you with your project. You can contact us at contact@clickalgo.com for more information.
Best Regards,
Donald
// -------------------------------------------------------------------------------------------------------------//
// //
// This cBot is intended to be used as a TEST and does not guarantee any particular outcome or //
// profit of any kind. Use it at your own risk. //
// //
// ALL buy and sell orders are executed at the beginning of a new bar and if the RSI conditions are met. //
// //
// The "OBiAL_RSI_cBot" will create a buy order when: //
// "Relative Strength Index indicator < LVDOUP" and "Relative Strength Index indicator > LVDODO" . //
// //
// The "OBiAL_RSI_cBot" will create a Sell order when: //
// "Relative Strength Index indicator > LVUPDO" and "Relative Strength Index indicator < LVUPUP" . //
// //
// The SL and TP parameters are set for every order of different than zero. //
// //
// The cBot can generate only one Buy or Sell order at any given time. //
// //
// IF YOU LIKE THE CODE AND WOULD LIKE TO DONATE: //
// //
// XRP (XRP) can be sent to this wallet address //
// rMdG3ju8pgyVh29ELPWaDuA74CpWW6Fxns //
// //
// Basic Attention Token (BAT) can be sent to this wallet address //
// 0x0bFc0EB112E76C96BA8374AEe8005aFfdca7D4c2 //
// //
// Litecoin (LTC) can be sent to this wallet address //
// LLnDKTB4VEyGLDi33itqZxhvN3AX694zNT //
// //
// -------------------------------------------------------------------------------------------------------------//
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class OBiAL_RSI_cBot : Robot
{
[Parameter("Source")]
public DataSeries Source { get; set; }
[Parameter("RSI Periods", DefaultValue = 14)]
public int Periods { get; set; }
[Parameter("Quantity (Lots)", DefaultValue = 1, MinValue = 0.01, Step = 0.01)]
public double Quantity { get; set; }
[Parameter("Def SL", DefaultValue = 20, MinValue = 0)]
public int DefSL { get; set; }
[Parameter("Def TP", DefaultValue = 20, MinValue = 0)]
public int DefTP { get; set; }
[Parameter("LVUPUP", DefaultValue = 80, MinValue = 0)]
public int LVUPUP { get; set; }
[Parameter("LVUPDO", DefaultValue = 70, MinValue = 0)]
public int LVUPDO { get; set; }
[Parameter("LVDOUP", DefaultValue = 30, MinValue = 0)]
public int LVDOUP { get; set; }
[Parameter("LVDODO", DefaultValue = 20, MinValue = 0)]
public int LVDODO { get; set; }
private RelativeStrengthIndex rsi;
protected override void OnStart()
{
rsi = Indicators.RelativeStrengthIndex(Source, Periods);
}
protected override void OnBar()
{
if (rsi.Result.LastValue < LVDOUP && rsi.Result.LastValue > LVDODO)
{
Open(TradeType.Buy);
}
else if (rsi.Result.LastValue > LVUPDO && rsi.Result.LastValue < LVUPUP)
{
Open(TradeType.Sell);
}
}
private void Close(TradeType tradeType)
{
foreach (var position in Positions.FindAll("TEST_RSI", SymbolName, tradeType))
ClosePosition(position);
}
private void Open(TradeType tradeType)
{
var position = Positions.Find("TEST_RSI", SymbolName, tradeType);
var volumeInUnits = Symbol.QuantityToVolume(Quantity);
if (position == null)
ExecuteMarketOrder(tradeType, SymbolName, volumeInUnits, "TEST_RSI", DefSL, DefTP);
}
}
}