Status
Open
Budget
20.00 USD
Payment Method
Direct Payment
Job Description
I am trying to create a simple RSI bot that opens orders based on RSI values that closes orders using a trailing stoploss.
This is the RSI code I used to base it on.
// ------------------------------------------------------------------------------------------------- // // This code is a cAlgo API sample. // // This robot is intended to be used as a sample and does not guarantee any particular outcome or // profit of any kind. Use it at your own risk. // // All changes to this file will be lost on next application start. // If you are going to modify this file please make a copy using the "Duplicate" command. // // The "Sample RSI Range Robot" will create a buy order when the Relative Strength Index indicator crosses the level 30, // and a Sell order when the RSI indicator crosses the level 70. The order is closed be either a Stop Loss, defined in // the "Stop Loss" parameter, or by the opposite RSI crossing signal (buy orders close when RSI crosses the 70 level // and sell orders are closed when RSI crosses the 30 level). // // The robot can generate only one Buy or Sell order at any given time. // // ------------------------------------------------------------------------------------------------- using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class SampleRSIRobot : Robot { [Parameter("Source")] public DataSeries Source { get; set; } [Parameter("Periods", DefaultValue = 14)] public int Periods { get; set; } [Parameter("Stop Loss (pips)", DefaultValue = 10, MinValue = 1)] public int StopLoss { get; set; } [Parameter("Volume", DefaultValue = 10000, MinValue = 1000)] public int Volume { get; set; } private RelativeStrengthIndex rsi; protected override void OnStart() { rsi = Indicators.RelativeStrengthIndex(Source, Periods); } protected override void OnTick() { if (rsi.Result.LastValue < 30) { Close(TradeType.Sell); Open(TradeType.Buy); } else if (rsi.Result.LastValue > 70) { Close(TradeType.Buy); Open(TradeType.Sell); } } private void Close(TradeType tradeType) { foreach (var position in Positions.FindAll("SampleRSI", Symbol, tradeType)) ClosePosition(position); } private void Open(TradeType tradeType) { var position = Positions.Find("SampleRSI", Symbol, tradeType); if (position == null) ExecuteMarketOrder(tradeType, Symbol, Volume, "SampleRSI"); } } }
Note: Unlike this version, my bot only opens orders, and it buys at high rsi values and sells at low rsi values.
And this is the trailing stoploss with trigger sample I was using:
// ------------------------------------------------------------------------------------------------- // // This robot is intended to be used as a sample and does not guarantee any particular outcome or // profit of any kind. Use it at your own risk // // The "Trailing Stop Loss Sample" Robot places a Buy or Sell Market order according to user input. // When the order is filled it implements trailing stop loss. // // ------------------------------------------------------------------------------------------------- using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class TrailingStopLossSample : Robot { [Parameter("Volume", DefaultValue = 1000)] public int Volume { get; set; } [Parameter("Buy")] public bool Buy { get; set; } [Parameter("Stop Loss", DefaultValue = 5)] public double StopLoss { get; set; } [Parameter("Trigger When Gaining", DefaultValue = 1)] public double TriggerWhenGaining { get; set; } [Parameter("Trailing Stop Loss Distance", DefaultValue = 1)] public double TrailingStopLossDistance { get; set; } private double _highestGain; private bool _isTrailing; protected override void OnStart() { //Execute a market order based on the direction parameter ExecuteMarketOrder(Buy ? TradeType.Buy : TradeType.Sell, Symbol, Volume, "SampleTrailing", StopLoss, null); //Set the position's highest gain in pips _highestGain = Positions[0].Pips; } protected override void OnTick() { var position = Positions.Find("SampleTrailing"); if (position == null) { Stop(); return; } //If the trigger is reached, the robot starts trailing if (!_isTrailing && position.Pips >= TriggerWhenGaining) { _isTrailing = true; } //If the cBot is trailing and the profit in pips is at the highest level, we need to readjust the stop loss if (_isTrailing && _highestGain < position.Pips) { //Based on the position's direction, we calculate the new stop loss price and we modify the position if (position.TradeType == TradeType.Buy) { var newSLprice = Symbol.Ask - (Symbol.PipValue * TrailingStopLossDistance); if (newSLprice > position.StopLoss) { ModifyPosition(position, newSLprice, null); } } else { var newSLprice = Symbol.Bid + (Symbol.PipValue * TrailingStopLossDistance); if (newSLprice < position.StopLoss) { ModifyPosition(position, newSLprice, null); } } //We reset the highest gain _highestGain = position.Pips; } } protected override void OnStop() { // Put your deinitialization logic here } } }
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class TheLittlePrince2 : Robot { [Parameter("Source")] public DataSeries Source { get; set; } [Parameter("Periods", DefaultValue = 14)] public int Periods { get; set; } [Parameter("Stop Loss (pips)", DefaultValue = 10, MinValue = 1)] public int StopLoss { get; set; } [Parameter("Volume", DefaultValue = 10000, MinValue = 1000)] public int Volume { get; set; } [Parameter("Buy")] public bool Buy { get; set; } [Parameter("Trigger When Gaining", DefaultValue = 1)] public double TriggerWhenGaining { get; set; } [Parameter("Trailing Stop Loss Distance", DefaultValue = 1)] public double TrailingStopLossDistance { get; set; } private RelativeStrengthIndex rsi; protected override void OnStart() { rsi = Indicators.RelativeStrengthIndex(Source, Periods); } protected override void OnTick() { if (rsi.Result.LastValue < 30) { Close(TradeType.Sell); } else if (rsi.Result.LastValue > 70) { Close(TradeType.Buy); } var position = Positions.Find("SampleTrailing"); if (position == null) { Stop(); return; } //If the trigger is reached, the robot starts trailing if (!_isTrailing && position.Pips >= TriggerWhenGaining) { _isTrailing = true; } //If the cBot is trailing and the profit in pips is at the highest level, we need to readjust the stop loss if (_isTrailing && _highestGain < position.Pips) { //Based on the position's direction, we calculate the new stop loss price and we modify the position if (position.TradeType == TradeType.Buy) { var newSLprice = Symbol.Ask - (Symbol.PipValue * TrailingStopLossDistance); if (newSLprice > position.StopLoss) { ModifyPosition(position, newSLprice, null); } } else { var newSLprice = Symbol.Bid + (Symbol.PipValue * TrailingStopLossDistance); if (newSLprice < position.StopLoss) { ModifyPosition(position, newSLprice, null); } } //We reset the highest gain _highestGain = position.Pips; } } private void Close(TradeType tradeType) { foreach (var position in Positions.FindAll("TheLittlePrince2", Symbol, tradeType)) ClosePosition(position); } private void Open(TradeType tradeType) { var position = Positions.Find("TheLittlePrince2", Symbol, tradeType); if (position == null) ExecuteMarketOrder(tradeType, Symbol, Volume, "TheLittlePrince2"); } } }
This is what I have put together, I call it TheLittlePrince2 because it is designed to catch spikes. I plan to use it on the tick charts.
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class TheLittlePrince2 : Robot { [Parameter("Source")] public DataSeries Source { get; set; } [Parameter("Periods", DefaultValue = 14)] public int Periods { get; set; } [Parameter("Stop Loss (pips)", DefaultValue = 10, MinValue = 1)] public int StopLoss { get; set; } [Parameter("Volume", DefaultValue = 10000, MinValue = 1000)] public int Volume { get; set; } [Parameter("Buy")] public bool Buy { get; set; } [Parameter("Trigger When Gaining", DefaultValue = 1)] public double TriggerWhenGaining { get; set; } [Parameter("Trailing Stop Loss Distance", DefaultValue = 1)] public double TrailingStopLossDistance { get; set; } private RelativeStrengthIndex rsi; protected override void OnStart() { rsi = Indicators.RelativeStrengthIndex(Source, Periods); } protected override void OnTick() { if (rsi.Result.LastValue < 30) { Close(TradeType.Sell); } else if (rsi.Result.LastValue > 70) { Close(TradeType.Buy); } var position = Positions.Find("SampleTrailing"); if (position == null) { Stop(); return; } //If the trigger is reached, the robot starts trailing if (!_isTrailing && position.Pips >= TriggerWhenGaining) { _isTrailing = true; } //If the cBot is trailing and the profit in pips is at the highest level, we need to readjust the stop loss if (_isTrailing && _highestGain < position.Pips) { //Based on the position's direction, we calculate the new stop loss price and we modify the position if (position.TradeType == TradeType.Buy) { var newSLprice = Symbol.Ask - (Symbol.PipValue * TrailingStopLossDistance); if (newSLprice > position.StopLoss) { ModifyPosition(position, newSLprice, null); } } else { var newSLprice = Symbol.Bid + (Symbol.PipValue * TrailingStopLossDistance); if (newSLprice < position.StopLoss) { ModifyPosition(position, newSLprice, null); } } //We reset the highest gain _highestGain = position.Pips; } } private void Close(TradeType tradeType) { foreach (var position in Positions.FindAll("TheLittlePrince2", Symbol, tradeType)) ClosePosition(position); } private void Open(TradeType tradeType) { var position = Positions.Find("TheLittlePrince2", Symbol, tradeType); if (position == null) ExecuteMarketOrder(tradeType, Symbol, Volume, "TheLittlePrince2"); } } }
But I lack the ability to finish it on my own. It keeps coming up with problems that I don't understand.
Comments
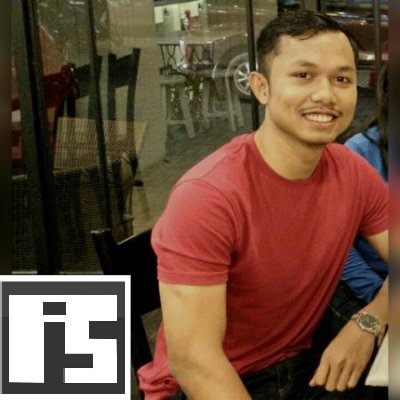
Greetings...
i have read your job description and i can grasp the idea that you want to build.
if i can conclude that you want to made RSI bot, with strategies as mentioned below :
Entry Strategy :
Market sell order on RSI => 70
Market buy order on RSI =< 30
Exit Strategy :
By trailing stops
I can make it for you. In fact, i already did some progress :)
PS :
as long as the goals reached, do you mind if tinker your code by a lot ?
You can reach me at rony.sitepu@gmail.com for further discussion
Regards
RKS
I The execute order code should look like this (in place of above)
protected override void OnTick()
{
if (rsi.Result.LastValue < 30)
{
Open(TradeType.Sell);
}
else if (rsi.Result.LastValue > 70)
{
Open(TradeType.Buy);
Hi it will be a pleasure for me to work with you, I am a very experienced developer in MT5/MT4, cTrade, Trading View. let's discuss about your project.
Email: dolandjosama34@gmail.com
Phone/Telegram: +50938512703