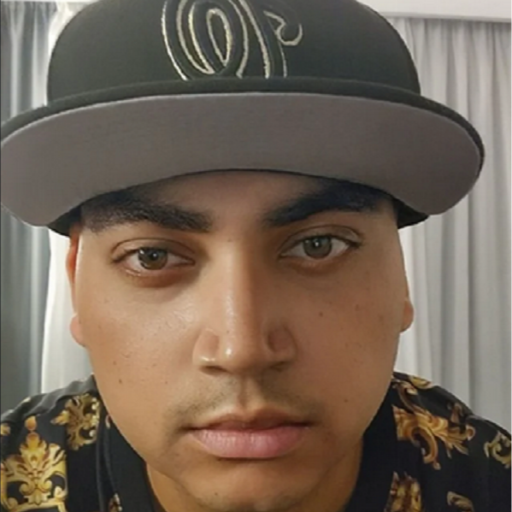
Status
Open
Budget
450.00 USD
Payment Method
Direct Payment
Job Description
Hello I would like to have an HFT cAlgo cBot with simulor performance to Luxor HFT XAU Bot in the attached image file.
If you do not know of a method to replicated such profits in a cAlgo cBot. Then use the following as example to code some type of profitalbe HFT bot with the following Paramaters:
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class OptimizedHFTradingBot : Robot
{
[Parameter("Percent Risk Per Trade", DefaultValue = 1.0)]
public double PercentRiskPerTrade { get; set; }
[Parameter("Fixed Lot Size", DefaultValue = 10000)]
public int FixedLotSize { get; set; }
[Parameter("Dynamic Stop Loss Multiplier (ATR)", DefaultValue = 1.5)]
public double StopLossMultiplier { get; set; }
[Parameter("Dynamic Take Profit Multiplier (ATR)", DefaultValue = 2.0)]
public double TakeProfitMultiplier { get; set; }
private RelativeStrengthIndex rsi;
private ExponentialMovingAverage ema;
private BollingerBands bb;
private MacdHistogram macd;
private OnBalanceVolume obv;
private AverageTrueRange atr;
protected override void OnStart()
{
rsi = Indicators.RelativeStrengthIndex(Bars.ClosePrices, 14);
ema = Indicators.ExponentialMovingAverage(Bars.ClosePrices, 21);
bb = Indicators.BollingerBands(Bars.ClosePrices, 20, 2, MovingAverageType.Simple);
macd = Indicators.MacdHistogram(Bars.ClosePrices, 12, 26, 9);
obv = Indicators.OnBalanceVolume(Bars.ClosePrices);
atr = Indicators.AverageTrueRange(Bars, 14, MovingAverageType.Simple);
}
protected override void OnBar()
{
double lastClose = Bars.ClosePrices.LastValue;
double currentEMA = ema.Result.LastValue;
double currentOBV = obv.Result.LastValue;
double lastOBV = obv.Result.Last(1);
double stopLossPips = atr.Result.LastValue * StopLossMultiplier;
double takeProfitPips = atr.Result.LastValue * TakeProfitMultiplier;
if (ShouldBuy(lastClose, currentEMA, currentOBV, lastOBV))
{
ExecuteTrade(TradeType.Buy, stopLossPips, takeProfitPips);
}
else if (ShouldSell(lastClose, currentEMA, currentOBV, lastOBV))
{
ExecuteTrade(TradeType.Sell, stopLossPips, takeProfitPips);
}
}
private bool ShouldBuy(double lastClose, double currentEMA, double currentOBV, double lastOBV)
{
return lastClose > currentEMA && currentOBV > lastOBV && macd.Histogram.LastValue > 0;
}
private bool ShouldSell(double lastClose, double currentEMA, double currentOBV, double lastOBV)
{
return lastClose < currentEMA && currentOBV < lastOBV && macd.Histogram.LastValue < 0;
}
private void ExecuteTrade(TradeType tradeType, double stopLossPips, double takeProfitPips)
{
long volume = CalculateVolume();
var result = ExecuteMarketOrder(tradeType, SymbolName, volume, label: tradeType.ToString() + "Order", -stopLossPips, takeProfitPips);
if (result.IsSuccessful)
{
Print($"{tradeType} order executed successfully at {result.Position.EntryPrice}");
}
else
{
Print($"Error executing {tradeType} order: {result.Error}");
}
}
private long CalculateVolume()
{
double riskAmount = Account.Balance * PercentRiskPerTrade / 100;
return riskAmount >= FixedLotSize ? Convert.ToInt64(riskAmount) : FixedLotSize;
}
}
}
Please try to make this following code profitable tweak or invent any code that can achieve such trading results as below image.
Thank you.
Comments
Hi this is MWAVES TRADING LIMITED We are confident in our base ability to develop an HFT cAlgo cBot that meets or exceeds the performance of the Luxor HFT XAU Bot. I’ll carefully review the image file you provided to understand its specifications and performance metrics. If replication proves challenging, We fully capable of creating a profitable HFT bot based on the parameters you’ve outlined. we will use the provided code example and incorporate additional optimizations to ensure the bot’s effectiveness in achieving your desired outcomes. We eager to get started on this project and look forward to delivering exceptional results. So that Mwaves can solve people’s compromises problems and make everything a lot easier while getting what you want Reach out t us on @mwavestradinglimited@gmail.com
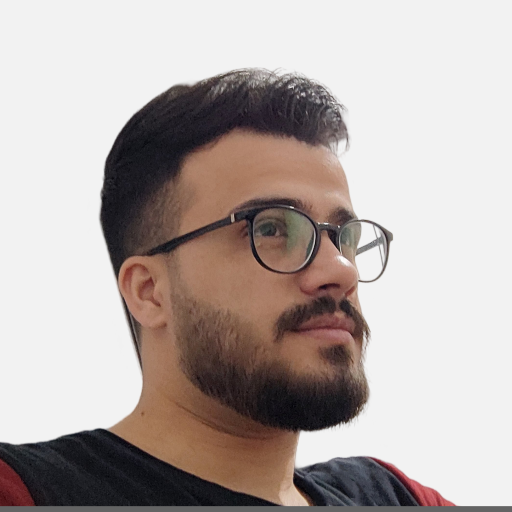
Hi, I can help you with this project. With several years of experience in programming, forex analysis and trading. Email: joseph.tradingbot@gmail.com Telegram: @iamjosepe
Hi, we can help you with this project. We are a team of Developer and we have a lot of experience in cTrader Platform. Please contact us on info@reavolution.com