c# indicator for calgo PivotSuperTrend-TXMA combi for ctrader community
Status
Open
Budget
0.00 USD
Payment Method
Direct Payment
Job Description
Good morning,
cansomeone do my c# indicator script to algo? And post it to algorithms→indicator for everyone?
Regards
Andreas
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = true, AccessRights = AccessRights.None)]
public class CombinedIndicator : Indicator
{
private PivotSuperTrend pivotSuperTrend;
private TXMA txma;
[Parameter("Fractal Length", DefaultValue = 2)]
public int FractalLength { get; set; }
[Parameter("Center Line Smoothing", DefaultValue = 5)]
public int CenterSmoothing { get; set; }
[Parameter("ATR Period", DefaultValue = 10)]
public int AtrPeriod { get; set; }
[Parameter("Atr Multiplier", DefaultValue = 3)]
public double AtrMulti { get; set; }
[Parameter("TXMA Period", DefaultValue = 14)]
public int TxmaPeriod { get; set; }
[Parameter("TXMA Order", DefaultValue = 6, MinValue = 3)]
public int TxmaOrder { get; set; }
[Parameter("TXMA Volume Factor", DefaultValue = 0.7)]
public double TxmaVolumeFactor { get; set; }
[Parameter("Show Direction", DefaultValue = true)]
public bool ShowDirection { get; set; }
[Parameter("Min Threshold", DefaultValue = 0.0, Step = 0.001, MinValue = 0)]
public double MinThreshold { get; set; }
[Parameter("Start Bar", DefaultValue = 1, MinValue = 0)]
public int StartBar { get; set; }
[Parameter("Stop Bar", DefaultValue = 2, MinValue = 0)]
public int StopBar { get; set; }
[Output("Trend Line", LineColor = "Transparent", PlotType = PlotType.Line, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries TrendLine { get; set; }
[Output("TXMA Steady", LineColor = "Gray", PlotType = PlotType.DiscontinuousLine, Thickness = 3)]
public IndicatorDataSeries TxmaSteady { get; set; }
[Output("TXMA Rise", LineColor = "Green", PlotType = PlotType.DiscontinuousLine, Thickness = 3)]
public IndicatorDataSeries TxmaRise { get; set; }
[Output("TXMA Fall", LineColor = "Red", PlotType = PlotType.DiscontinuousLine, Thickness = 3)]
public IndicatorDataSeries TxmaFall { get; set; }
protected override void Initialize()
{
pivotSuperTrend = Indicators.GetIndicator<PivotSuperTrend>(FractalLength, CenterSmoothing, AtrPeriod, AtrMulti);
txma = Indicators.GetIndicator<TXMA>(Source, TxmaPeriod, TxmaOrder, TxmaVolumeFactor, ShowDirection, MinThreshold, StartBar, StopBar);
}
public override void Calculate(int index)
{
pivotSuperTrend.Calculate(index);
txma.Calculate(index);
TrendLine[index] = pivotSuperTrend.TrendLine[index];
TxmaSteady[index] = txma.steady[index];
TxmaRise[index] = txma.rise[index];
TxmaFall[index] = txma.fall[index];
}
}
}
Comments
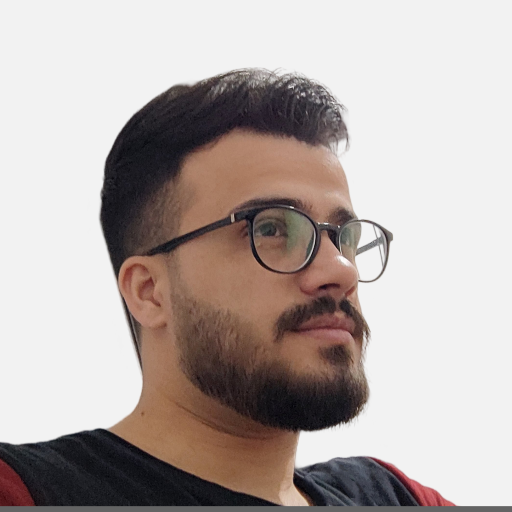