Status
In progress
Budget
150.00 USD
Payment Method
Direct Payment
Job Description
Dear Freeflancer,
I have an existing cBot that is working well but I want to change it to read a CSV file for the input parameters. If you have done a job like this in the past and would like to do this for me please apply. Please find below the cBot source code. I will provide you with the CSV input file once we have agreed to the work.
I have a budget of $150 if this is not enough then give me an indication whatyou would charge and the timeframe for delivering the work. In order for me to sign off the work and provide payment I will have to test the end result with the CSV file I provide.
If you have any other queries or concerns please let me know.
Regards,
Hennie Smith
using System;
using System.Threading;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.API.Requests;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC)]
public class MRS17 : Robot
{
[Parameter("Slowing", DefaultValue = 3)]
public int _Slowing { get; set; }
[Parameter("Period", DefaultValue = 11)]
public int _Period { get; set; }
[Parameter("Grid_Points", DefaultValue = 200)]
public int _Grid_Points { get; set; }
[Parameter("Buy_TSL_in_Points", DefaultValue = 300)]
public int _Buy_SL_in_Points { get; set; }
[Parameter("RSI_Buy_Limit", DefaultValue = 10)]
public int _RSI_Buy_Limit { get; set; }
// [Parameter("Buy_TP_in_Points", DefaultValue = 100)]
// public int _Buy_TP_in_Points { get; set; }
[Parameter("Max_Days", DefaultValue = 9)]
public int _Max_Days { get; set; }
[Parameter("Stoc_Buy_Limit", DefaultValue = 20)]
public int _Stoc_Buy_Limit { get; set; }
[Parameter("Mom_Buy_Limit", DefaultValue = 100)]
public double _Mom_Buy_Limit { get; set; }
[Parameter("D_Period", DefaultValue = 3)]
public int _D_Period { get; set; }
[Parameter("Sell_TSL_in_Points", DefaultValue = 300)]
public int _Sell_SL_in_Points { get; set; }
[Parameter("Stoc_Sell_Limit", DefaultValue = 80)]
public int _Stoc_Sell_Limit { get; set; }
[Parameter("RSI_Sell_Limit", DefaultValue = 91)]
public int _RSI_Sell_Limit { get; set; }
[Parameter("Mom_Sell_Limit", DefaultValue = 100)]
public double _Mom_Sell_Limit { get; set; }
[Parameter("Max_Open_Trades", DefaultValue = 5)]
public int _Max_Open_Trades { get; set; }
[Parameter("Lot_Percentage", DefaultValue = 0.0005)]
public double _Lot_Percentage { get; set; }
[Parameter("Label", DefaultValue = "MRS17_")]
public string _Lable { get; set; }
[Parameter("Trailing SL", DefaultValue = true)]
public bool _isTrailing { get; set; }
//Global declaration
private MomentumOscillator i_Momentum;
private StochasticOscillator i_Stochastic_3;
private RelativeStrengthIndex i_RSI;
double _Momentum;
double _Stochastic_3;
double _RSI;
bool _Compare_7;
bool _Compare_10;
bool _Compare_1;
bool _Compare_9;
bool _Compare_11;
double _Arithmetic;
bool _Compare_12;
bool _Compare_2;
DateTime LastTradeExecution = new DateTime(0);
protected override void OnStart()
{
i_Momentum = Indicators.MomentumOscillator(MarketSeries.Close, (int)_Period);
i_Stochastic_3 = Indicators.StochasticOscillator((int)_Period, (int)_Slowing, (int)_D_Period, MovingAverageType.Simple);
i_RSI = Indicators.RelativeStrengthIndex(MarketSeries.Close, (int)_Period);
}
protected override void OnTick()
{
if (Trade.IsExecuting)
return;
//Local declaration
TriState _Close_Position_1 = new TriState();
TriState _Sell = new TriState();
TriState _Buy = new TriState();
TriState _Buy_2 = new TriState();
TriState _Sell_2 = new TriState();
//Step 1
_Momentum = i_Momentum.Result.Last(0);
_Stochastic_3 = i_Stochastic_3.PercentK.Last(0);
_RSI = i_RSI.Result.Last(0);
//Step 2
_Compare_7 = (_RSI > _RSI_Sell_Limit);
_Compare_10 = (_Momentum > _Mom_Sell_Limit);
_Compare_1 = (_RSI < _RSI_Buy_Limit);
_Compare_9 = (_Momentum < _Mom_Buy_Limit);
_Compare_11 = (Number_of_Open_Trades(1) < _Max_Open_Trades);
_Arithmetic = ((_Grid_Points * (-1)) * (Number_of_Open_Trades(1)));
_Compare_12 = (_Stochastic_3 > _Stoc_Sell_Limit);
_Compare_2 = (_Stochastic_3 < _Stoc_Buy_Limit);
//Step 3
//Step 4
if (((new Func<string, double, DateTime>((symbolCode, magicIndex) =>
{
Symbol symbol = (symbolCode == "" || symbolCode == Symbol.Code) ? Symbol : MarketData.GetSymbol(symbolCode);
var pos = Positions.Find(_Lable + magicIndex.ToString("F0"), symbol);
return pos == null ? new DateTime(0) : pos.EntryTime;
})("", 1)).AddDays(_Max_Days) < Server.Time))
_Close_Position_1 = _ClosePosition(1, Symbol.Code, 0);
//Step 5
//Step 6
if (((_Compare_7 && (_Compare_12 && NoOrders(Symbol.Code, new double[]
{
}))) && _Compare_10))
_Sell = Sell(1, ((_Lot_Percentage * (Account.FreeMargin)) / (100000)), 0, _Sell_SL_in_Points, 0, 0, 5, _Max_Open_Trades, 0, "");
if ((((_Compare_2 && NoOrders(Symbol.Code, new double[]
{
})) && _Compare_1) && _Compare_9))
_Buy = Buy(1, ((_Lot_Percentage * (Account.FreeMargin)) / (100000)), 0, _Buy_SL_in_Points, 0, 0, 5, _Max_Open_Trades, 0, "");
//Step 8
//Step 9
//Step 10
if ((((((!NoOrders(Symbol.Code, new double[]
{
}) && ((_Arithmetic < 0) && ((new Func<string, double, double>((symbolCode, magicIndex) =>
{
Symbol symbol = (symbolCode == "" || symbolCode == Symbol.Code) ? Symbol : MarketData.GetSymbol(symbolCode);
var pos = Positions.Find(_Lable + magicIndex.ToString("F0"), symbol);
if (pos != null)
return pos.Pips * ((Symbol.Digits == 5 || Symbol.Digits == 3) ? 10 : 1);
var po = PendingOrders.__Find(_Lable + magicIndex.ToString("F0"), symbol);
return po == null ? 0 : 0;
})("", 1)) < _Arithmetic))) && _Compare_11) && _Compare_2) && _Compare_1) && _Compare_9))
_Buy_2 = Buy(1, ((_Lot_Percentage * (Account.FreeMargin)) / (100000)), 0, _Buy_SL_in_Points, 0, 0, 5, _Max_Open_Trades, 0, "");
if ((((((!NoOrders(Symbol.Code, new double[]
{
}) && ((_Arithmetic < 0) && ((new Func<string, double, double>((symbolCode, magicIndex) =>
{
Symbol symbol = (symbolCode == "" || symbolCode == Symbol.Code) ? Symbol : MarketData.GetSymbol(symbolCode);
var pos = Positions.Find(_Lable + magicIndex.ToString("F0"), symbol);
if (pos != null)
return pos.Pips * ((Symbol.Digits == 5 || Symbol.Digits == 3) ? 10 : 1);
var po = PendingOrders.__Find(_Lable + magicIndex.ToString("F0"), symbol);
return po == null ? 0 : 0;
})("", 1)) < _Arithmetic))) && _Compare_11) && _Compare_12) && _Compare_7) && _Compare_10))
_Sell_2 = Sell(1, ((_Lot_Percentage * (Account.FreeMargin)) / (100000)), 0, _Sell_SL_in_Points, 0, 0, 5, _Max_Open_Trades, 0, "");
}
bool NoOrders(string symbolCode, double[] magicIndecies)
{
if (symbolCode == "")
symbolCode = Symbol.Code;
string[] labels = new string[magicIndecies.Length];
for (int i = 0; i < magicIndecies.Length; i++)
{
labels[i] = _Lable + magicIndecies[i].ToString("F0");
}
foreach (Position pos in Positions)
{
if (pos.SymbolCode != symbolCode)
continue;
if (labels.Length == 0)
return false;
foreach (var label in labels)
{
if (pos.Label == label)
return false;
}
}
foreach (PendingOrder po in PendingOrders)
{
if (po.SymbolCode != symbolCode)
continue;
if (labels.Length == 0)
return false;
foreach (var label in labels)
{
if (po.Label == label)
return false;
}
}
return true;
}
TriState _OpenPosition(double magicIndex, bool noOrders, string symbolCode, TradeType tradeType, double lots, double slippage, double? stopLoss, double? takeProfit, string comment)
{
Symbol symbol = (Symbol.Code == symbolCode) ? Symbol : MarketData.GetSymbol(symbolCode);
if (noOrders && Positions.Find(_Lable + magicIndex.ToString("F0"), symbol) != null)
return new TriState();
if (stopLoss < 1)
stopLoss = null;
if (takeProfit < 1)
takeProfit = null;
if (symbol.Digits == 5 || symbol.Digits == 3)
{
if (stopLoss != null)
stopLoss /= 10;
if (takeProfit != null)
takeProfit /= 10;
slippage /= 10;
}
int volume = Convert.ToInt32(lots * 100000);
if (!ExecuteMarketOrder(tradeType, symbol, volume, _Lable + magicIndex.ToString("F0"), stopLoss, takeProfit, slippage, comment, _isTrailing).IsSuccessful)
{
Thread.Sleep(400);
return false;
}
return true;
}
TriState _SendPending(double magicIndex, bool noOrders, string symbolCode, PendingOrderType poType, TradeType tradeType, double lots, int priceAction, double priceValue, double? stopLoss, double? takeProfit,
DateTime? expiration, string comment)
{
Symbol symbol = (Symbol.Code == symbolCode) ? Symbol : MarketData.GetSymbol(symbolCode);
if (noOrders && PendingOrders.__Find(_Lable + magicIndex.ToString("F0"), symbol) != null)
return new TriState();
if (stopLoss < 1)
stopLoss = null;
if (takeProfit < 1)
takeProfit = null;
if (symbol.Digits == 5 || symbol.Digits == 3)
{
if (stopLoss != null)
stopLoss /= 10;
if (takeProfit != null)
takeProfit /= 10;
}
int volume = Convert.ToInt32(lots * 100000);
double targetPrice;
switch (priceAction)
{
case 0:
targetPrice = priceValue;
break;
case 1:
targetPrice = symbol.Bid - priceValue * symbol.TickSize;
break;
case 2:
targetPrice = symbol.Bid + priceValue * symbol.TickSize;
break;
case 3:
targetPrice = symbol.Ask - priceValue * symbol.TickSize;
break;
case 4:
targetPrice = symbol.Ask + priceValue * symbol.TickSize;
break;
default:
targetPrice = priceValue;
break;
}
if (expiration.HasValue && (expiration.Value.Ticks == 0 || expiration.Value == DateTime.Parse("1970.01.01 00:00:00")))
expiration = null;
if (poType == PendingOrderType.Limit)
{
if (!PlaceLimitOrder(tradeType, symbol, volume, targetPrice, _Lable + magicIndex.ToString("F0"), stopLoss, takeProfit, expiration, comment).IsSuccessful)
{
Thread.Sleep(400);
return false;
}
return true;
}
else if (poType == PendingOrderType.Stop)
{
if (!PlaceStopOrder(tradeType, symbol, volume, targetPrice, _Lable + magicIndex.ToString("F0"), stopLoss, takeProfit, expiration, comment).IsSuccessful)
{
Thread.Sleep(400);
return false;
}
return true;
}
return new TriState();
}
TriState _ModifyPosition(double magicIndex, string symbolCode, int slAction, double slValue, int tpAction, double tpValue)
{
Symbol symbol = (Symbol.Code == symbolCode) ? Symbol : MarketData.GetSymbol(symbolCode);
var pos = Positions.Find(_Lable + magicIndex.ToString("F0"), symbol);
if (pos == null)
return new TriState();
double? sl, tp;
if (slValue == 0)
sl = null;
else
{
switch (slAction)
{
case 0:
sl = pos.StopLoss;
break;
case 1:
if (pos.TradeType == TradeType.Buy)
sl = pos.EntryPrice - slValue * symbol.TickSize;
else
sl = pos.EntryPrice + slValue * symbol.TickSize;
break;
case 2:
sl = slValue;
break;
default:
sl = pos.StopLoss;
break;
}
}
if (tpValue == 0)
tp = null;
else
{
switch (tpAction)
{
case 0:
tp = pos.TakeProfit;
break;
case 1:
if (pos.TradeType == TradeType.Buy)
tp = pos.EntryPrice + tpValue * symbol.TickSize;
else
tp = pos.EntryPrice - tpValue * symbol.TickSize;
break;
case 2:
tp = tpValue;
break;
default:
tp = pos.TakeProfit;
break;
}
}
if (!ModifyPosition(pos, sl, tp).IsSuccessful)
{
Thread.Sleep(400);
return false;
}
return true;
}
TriState _ModifyPending(double magicIndex, string symbolCode, int slAction, double slValue, int tpAction, double tpValue, int priceAction, double priceValue, int expirationAction, DateTime? expiration)
{
Symbol symbol = (Symbol.Code == symbolCode) ? Symbol : MarketData.GetSymbol(symbolCode);
var po = PendingOrders.__Find(_Lable + magicIndex.ToString("F0"), symbol);
if (po == null)
return new TriState();
double targetPrice;
double? sl, tp;
if (slValue == 0)
sl = null;
else
{
switch (slAction)
{
case 0:
sl = po.StopLoss;
break;
case 1:
if (po.TradeType == TradeType.Buy)
sl = po.TargetPrice - slValue * symbol.TickSize;
else
sl = po.TargetPrice + slValue * symbol.TickSize;
break;
case 2:
sl = slValue;
break;
default:
sl = po.StopLoss;
break;
}
}
if (tpValue == 0)
tp = null;
else
{
switch (tpAction)
{
case 0:
tp = po.TakeProfit;
break;
case 1:
if (po.TradeType == TradeType.Buy)
tp = po.TargetPrice + tpValue * symbol.TickSize;
else
tp = po.TargetPrice - tpValue * symbol.TickSize;
break;
case 2:
tp = tpValue;
break;
default:
tp = po.TakeProfit;
break;
}
}
switch (priceAction)
{
case 0:
targetPrice = po.TargetPrice;
break;
case 1:
targetPrice = priceValue;
break;
case 2:
targetPrice = po.TargetPrice + priceValue * symbol.TickSize;
break;
case 3:
targetPrice = po.TargetPrice - priceValue * symbol.TickSize;
break;
case 4:
targetPrice = symbol.Bid - priceValue * symbol.TickSize;
break;
case 5:
targetPrice = symbol.Bid + priceValue * symbol.TickSize;
break;
case 6:
targetPrice = symbol.Ask - priceValue * symbol.TickSize;
break;
case 7:
targetPrice = symbol.Ask + priceValue * symbol.TickSize;
break;
default:
targetPrice = po.TargetPrice;
break;
}
if (expiration.HasValue && (expiration.Value.Ticks == 0 || expiration.Value == DateTime.Parse("1970.01.01 00:00:00")))
expiration = null;
if (expirationAction == 0)
expiration = po.ExpirationTime;
if (!ModifyPendingOrder(po, targetPrice, sl, tp, expiration).IsSuccessful)
{
Thread.Sleep(400);
return false;
}
return true;
}
TriState _ClosePosition(double magicIndex, string symbolCode, double lots)
{
Symbol symbol = (Symbol.Code == symbolCode) ? Symbol : MarketData.GetSymbol(symbolCode);
var pos = Positions.Find(_Lable + magicIndex.ToString("F0"), symbol);
if (pos == null)
return new TriState();
TradeResult result;
if (lots == 0)
{
result = ClosePosition(pos);
}
else
{
int volume = Convert.ToInt32(lots * 100000);
result = ClosePosition(pos, volume);
}
if (!result.IsSuccessful)
{
Thread.Sleep(400);
return false;
}
return true;
}
TriState _DeletePending(double magicIndex, string symbolCode)
{
Symbol symbol = (Symbol.Code == symbolCode) ? Symbol : MarketData.GetSymbol(symbolCode);
var po = PendingOrders.__Find(_Lable + magicIndex.ToString("F0"), symbol);
if (po == null)
return new TriState();
if (!CancelPendingOrder(po).IsSuccessful)
{
Thread.Sleep(400);
return false;
}
return true;
}
bool _OrderStatus(double magicIndex, string symbolCode, int test)
{
Symbol symbol = (Symbol.Code == symbolCode) ? Symbol : MarketData.GetSymbol(symbolCode);
var pos = Positions.Find(_Lable + magicIndex.ToString("F0"), symbol);
if (pos != null)
{
if (test == 0)
return true;
if (test == 1)
return true;
if (test == 3)
return pos.TradeType == TradeType.Buy;
if (test == 4)
return pos.TradeType == TradeType.Sell;
}
var po = PendingOrders.__Find(_Lable + magicIndex.ToString("F0"), symbol);
if (po != null)
{
if (test == 0)
return true;
if (test == 2)
return true;
if (test == 3)
return po.TradeType == TradeType.Buy;
if (test == 4)
return po.TradeType == TradeType.Sell;
if (test == 5)
return po.OrderType == PendingOrderType.Limit;
if (test == 6)
return po.OrderType == PendingOrderType.Stop;
}
return false;
}
int TimeframeToInt(TimeFrame tf)
{
if (tf == TimeFrame.Minute)
return 1;
else if (tf == TimeFrame.Minute2)
return 2;
else if (tf == TimeFrame.Minute3)
return 3;
else if (tf == TimeFrame.Minute4)
return 4;
else if (tf == TimeFrame.Minute5)
return 5;
else if (tf == TimeFrame.Minute10)
return 10;
else if (tf == TimeFrame.Minute15)
return 15;
else if (tf == TimeFrame.Minute30)
return 30;
else if (tf == TimeFrame.Hour)
return 60;
else if (tf == TimeFrame.Hour4)
return 240;
else if (tf == TimeFrame.Daily)
return 1440;
else if (tf == TimeFrame.Weekly)
return 10080;
else if (tf == TimeFrame.Monthly)
return 43200;
return 1;
}
DateTime __currentBarTime = DateTime.MinValue;
bool __isNewBar(bool triggerAtStart)
{
DateTime newTime = MarketSeries.OpenTime.LastValue;
if (__currentBarTime != newTime)
{
if (!triggerAtStart && __currentBarTime == DateTime.MinValue)
{
__currentBarTime = newTime;
return false;
}
__currentBarTime = newTime;
return true;
}
return false;
}
int Number_of_Open_Trades(double magicIndex)
{
int res = 0;
foreach (Position pos in Positions.FindAll(_Lable + magicIndex.ToString("F0"), Symbol))
{
res++;
}
return res;
}
TriState Sell(double magicIndex, double Lots, int StopLossMethod, double stopLossValue, int TakeProfitMethod, double takeProfitValue, double Slippage, double MaxOpenTrades, double MaxFrequencyMins, string TradeComment)
{
double? stopLossPips, takeProfitPips;
int numberOfOpenTrades = 0;
var res = new TriState();
foreach (Position pos in Positions.FindAll(_Lable + magicIndex.ToString("F0"), Symbol))
{
numberOfOpenTrades++;
}
if (MaxOpenTrades > 0 && numberOfOpenTrades >= MaxOpenTrades)
return res;
if (MaxFrequencyMins > 0)
{
if (((TimeSpan)(Server.Time - LastTradeExecution)).TotalMinutes < MaxFrequencyMins)
return res;
foreach (Position pos in Positions.FindAll(_Lable + magicIndex.ToString("F0"), Symbol))
{
if (((TimeSpan)(Server.Time - pos.EntryTime)).TotalMinutes < MaxFrequencyMins)
return res;
}
}
int pipAdjustment = Convert.ToInt32(Symbol.PipSize / Symbol.TickSize);
if (stopLossValue > 0)
{
if (StopLossMethod == 0)
stopLossPips = stopLossValue / pipAdjustment;
else if (StopLossMethod == 1)
stopLossPips = stopLossValue;
else
stopLossPips = (stopLossValue - Symbol.Bid) / Symbol.PipSize;
}
else
stopLossPips = null;
if (takeProfitValue > 0)
{
if (TakeProfitMethod == 0)
takeProfitPips = takeProfitValue / pipAdjustment;
else if (TakeProfitMethod == 1)
takeProfitPips = takeProfitValue;
else
takeProfitPips = (Symbol.Bid - takeProfitValue) / Symbol.PipSize;
}
else
takeProfitPips = null;
Slippage /= pipAdjustment;
long volume = Symbol.NormalizeVolume(Lots * 100000, RoundingMode.ToNearest);
if (!ExecuteMarketOrder(TradeType.Sell, Symbol, volume, _Lable + magicIndex.ToString("F0"), stopLossPips, 0, Slippage, TradeComment, _isTrailing).IsSuccessful)
{
Thread.Sleep(400);
return false;
}
LastTradeExecution = Server.Time;
return true;
}
TriState Buy(double magicIndex, double Lots, int StopLossMethod, double stopLossValue, int TakeProfitMethod, double takeProfitValue, double Slippage, double MaxOpenTrades, double MaxFrequencyMins, string TradeComment)
{
double? stopLossPips, takeProfitPips;
int numberOfOpenTrades = 0;
var res = new TriState();
foreach (Position pos in Positions.FindAll(_Lable + magicIndex.ToString("F0"), Symbol))
{
numberOfOpenTrades++;
}
if (MaxOpenTrades > 0 && numberOfOpenTrades >= MaxOpenTrades)
return res;
if (MaxFrequencyMins > 0)
{
if (((TimeSpan)(Server.Time - LastTradeExecution)).TotalMinutes < MaxFrequencyMins)
return res;
foreach (Position pos in Positions.FindAll(_Lable + magicIndex.ToString("F0"), Symbol))
{
if (((TimeSpan)(Server.Time - pos.EntryTime)).TotalMinutes < MaxFrequencyMins)
return res;
}
}
int pipAdjustment = Convert.ToInt32(Symbol.PipSize / Symbol.TickSize);
if (stopLossValue > 0)
{
if (StopLossMethod == 0)
stopLossPips = stopLossValue / pipAdjustment;
else if (StopLossMethod == 1)
stopLossPips = stopLossValue;
else
stopLossPips = (Symbol.Ask - stopLossValue) / Symbol.PipSize;
}
else
stopLossPips = null;
if (takeProfitValue > 0)
{
if (TakeProfitMethod == 0)
takeProfitPips = takeProfitValue / pipAdjustment;
else if (TakeProfitMethod == 1)
takeProfitPips = takeProfitValue;
else
takeProfitPips = (takeProfitValue - Symbol.Ask) / Symbol.PipSize;
}
else
takeProfitPips = null;
Slippage /= pipAdjustment;
long volume = Symbol.NormalizeVolume(Lots * 100000, RoundingMode.ToNearest);
if (!ExecuteMarketOrder(TradeType.Buy, Symbol, volume, _Lable + magicIndex.ToString("F0"), stopLossPips, 0, Slippage, TradeComment, _isTrailing).IsSuccessful)
{
Thread.Sleep(400);
return false;
}
LastTradeExecution = Server.Time;
return true;
}
}
}
public struct TriState
{
public static readonly TriState NonExecution = new TriState(0);
public static readonly TriState False = new TriState(-1);
public static readonly TriState True = new TriState(1);
sbyte value;
TriState(int value)
{
this.value = (sbyte)value;
}
public bool IsNonExecution
{
get { return value == 0; }
}
public static implicit operator TriState(bool x)
{
return x ? True : False;
}
public static TriState operator ==(TriState x, TriState y)
{
if (x.value == 0 || y.value == 0)
return NonExecution;
return x.value == y.value ? True : False;
}
public static TriState operator !=(TriState x, TriState y)
{
if (x.value == 0 || y.value == 0)
return NonExecution;
return x.value != y.value ? True : False;
}
public static TriState operator !(TriState x)
{
return new TriState(-x.value);
}
public static TriState operator &(TriState x, TriState y)
{
return new TriState(x.value < y.value ? x.value : y.value);
}
public static TriState operator |(TriState x, TriState y)
{
return new TriState(x.value > y.value ? x.value : y.value);
}
public static bool operator true(TriState x)
{
return x.value > 0;
}
public static bool operator false(TriState x)
{
return x.value < 0;
}
public static implicit operator bool(TriState x)
{
return x.value > 0;
}
public override bool Equals(object obj)
{
if (!(obj is TriState))
return false;
return value == ((TriState)obj).value;
}
public override int GetHashCode()
{
return value;
}
public override string ToString()
{
if (value > 0)
return "True";
if (value < 0)
return "False";
return "NonExecution";
}
}
public static class PendingEx
{
public static PendingOrder __Find(this cAlgo.API.PendingOrders pendingOrders, string label, Symbol symbol)
{
foreach (PendingOrder po in pendingOrders)
{
if (po.SymbolCode == symbol.Code && po.Label == label)
return po;
}
return null;
}
}
Comments
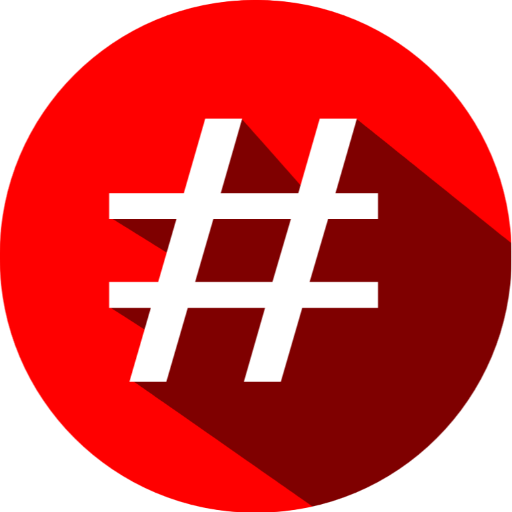
Hi there, (we offer you 20% discount if you reply within 5 days from the date of this message)
We can help you with your project. You can contact us at :
- Email, info@ctrader.guru
- Telegram, https://t.me/ctraderguru
- Messenger, https://m.me/ctrader.guru
Best Regards,
Hi, I can do it for you.
with several years of experience in programming and forex analysis and trading.
Telegram: @iamjosepe
Email: joseph.tradingbot@gmail.com