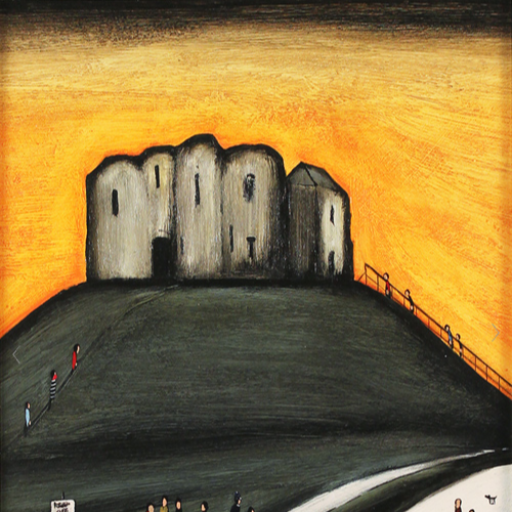
Indicator signal didn't appear where it suppose to
03 Jan 2022, 02:41
Hello,
I have the below indicator which is supposed to give a signal when the price crossing 50h SMA. The signal should be displayed when a new bar is just established.
However, in the test, I didn't see the signal displayed correctly. The signal only appears after I refresh the chart, i.e. switch timeframe to others and back to H1 timeframe again.
Please advise, if anyone could. Thank you!
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class X_SMA : Indicator
{
[Output("SMA Move", LineColor = "75FFFFFF", Thickness = 2, PlotType = PlotType.Line)]
public IndicatorDataSeries SMA_Move{ get; set; } //White SMA line
[Output("Cross SMA Above", LineColor = "BB77FF77", IsHistogram = false, LineStyle = LineStyle.Dots, PlotType = PlotType.Points, Thickness = 5)]
public IndicatorDataSeries CrossAbove { get; set; } //Green Dot Signal
[Output("Cross SMA Below", LineColor = "BBFF7777", IsHistogram = false, LineStyle = LineStyle.Dots, PlotType = PlotType.Points, Thickness = 5)]
public IndicatorDataSeries CrossBelow { get; set; } //Red Dot Signal
private SimpleMovingAverage SMA;
protected override void Initialize()
{
SMA = Indicators.SimpleMovingAverage(Bars.ClosePrices, 50);
}
public override void Calculate(int index)
{
SMA_Move[index] = SMA.Result.LastValue;
if (!IsLastBar && TimeFrame.Name == "Hour")
{
if ( Bars.ClosePrices.LastValue>SMA.Result.Last(0) && Bars.ClosePrices.Last(1)<SMA.Result.Last(1) )
{ CrossAbove[index] = Bars.LowPrices[index] - 10*Symbol.PipSize ;
Notifications.SendEmail("*****@icloud.com", "*****@icloud.com", "X 50 SMA - " + Symbol.Name, "X ABOVE");
}
if ( Bars.ClosePrices.LastValue<SMA.Result.Last(0) && Bars.ClosePrices.Last(1)>SMA.Result.Last(1))
{ CrossBelow[index] = Bars.HighPrices[index] + 10*Symbol.PipSize ;
Notifications.SendEmail("*****@icloud.com", "*****@icloud.com", "X 50 SMA - " + Symbol.Name, "X BELOW");
}
}
}
}
}
Replies
Capt.Z-Fort.Builder
03 Jan 2022, 20:27
( Updated at: 03 Jan 2022, 20:37 )
RE:
Thank you amusleh,
I've tested your code, it has perfectly solved the problem of signalling on the chart. Thanks.
But it will continue sending emails when the single triggered in the last bar running. However, I've managed to add 2 bools to control the email sending, which make it what it originally planned to do.
Thank you very much.
amusleh said:
Hi,
It doesn't show the dots because your limiting it to only draw the dot if it's a LastBar, try this:
using cAlgo.API; using cAlgo.API.Internals; using cAlgo.API.Indicators; namespace cAlgo { ... private SimpleMovingAverage SMA; private bool _XaboveSilent; private bool _XbelowSilent; ... public override void Calculate(int index) { ... if (IsLastBar && !_XaboveSilent) { Notifications.SendEmail("*****@icloud.com", "*****@icloud.com", "X 50 SMA - " + Symbol.Name, "X ABOVE"); _XaboveSilent = true; _XbelowSilent = false; } ... if (IsLastBar && !_XbelowSilent) { Notifications.SendEmail("*****@icloud.com", "*****@icloud.com", "X 50 SMA - " + Symbol.Name, "X BELOW"); _XbelowSilent = true; _XaboveSilent = false; } ... }
@Capt.Z-Fort.Builder
amusleh
03 Jan 2022, 09:06
Hi,
It doesn't show the dots because your limiting it to only draw the dot if it's a LastBar, try this:
There is still one issue with above code, you are drawing a dot for bars that are not closed/completed yet.
And that will cause repaint issue as the close price can change, instead you should use Last closed bar data:
@amusleh