MTF VWAP
MTF VWAP
23 Feb 2020, 12:34
Hi,
I would like to be able to select different time frames to run this VWAP indicator on the one chart. It appears to look okay when choosing the same time frame as the chart, but when selecting other time frames, it does not look right. What am I doing wrong in the code? Thanks.
Sascha
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class BVwap : Indicator
{
[Parameter("Period Type", Group = "VWAP", DefaultValue = PeriodType.Daily)]
public PeriodType Type { get; set; }
[Parameter("Time Frame", DefaultValue = "Hour")]
public TimeFrame timeframe { get; set; }
[Parameter("Offset Pips", Group = "VWAP", DefaultValue = 1, Step = 0.1)]
public double OffsetPips { get; set; }
[Output("Main", Color = Colors.Yellow, Thickness = 1)]
public IndicatorDataSeries VWAP { get; set; }
[Output("Upper Band", Color = Colors.Yellow, Thickness = 1)]
public IndicatorDataSeries UpperBand { get; set; }
[Output("Lower Band", Color = Colors.Yellow, Thickness = 1)]
public IndicatorDataSeries LowerBand { get; set; }
private DataSeries high, low, close, volume;
private double culmultivePV;
private double culmutiveVolume;
private MarketSeries anothertimeframe;
private double offset;
public enum PeriodType
{
Daily,
Weekly,
Monthly
}
protected override void Initialize()
{
anothertimeframe = MarketData.GetSeries(SymbolName, timeframe);
high = anothertimeframe.High;
low = anothertimeframe.Low;
close = anothertimeframe.Close;
volume = anothertimeframe.TickVolume;
culmutiveVolume = 0;
culmultivePV = 0;
offset = OffsetPips * Symbol.PipSize;
}
public override void Calculate(int index)
{
var index2 = GetIndexByDate(anothertimeframe, MarketSeries.OpenTime[index]);
if (IsFirstBarOfSession(index))
{
culmultivePV = (((close[index2] + high[index2] + low[index2]) / 3) * volume[index2]);
culmutiveVolume = volume[index2];
}
else
{
culmultivePV = (((close[index2 - 1] + high[index2 - 1] + low[index2 - 1]) / 3) * volume[index2 - 1]) + culmultivePV;
culmutiveVolume = volume[index2 - 1] + culmutiveVolume;
}
VWAP[index] = culmultivePV / culmutiveVolume;
UpperBand[index] = VWAP[index] + offset;
LowerBand[index] = VWAP[index] - offset;
}
private int GetIndexByDate(MarketSeries series, DateTime time)
{
for (int i = series.Close.Count - 1; i > 0; i--)
{
if (time == series.OpenTime[i])
{
return i;
}
else if (series.OpenTime[i] < time)
{
return i;
}
}
return -1;
}
private bool IsFirstBarOfSession(int index)
{
var time = MarketSeries.OpenTime;
//var time = anothertimeframe.OpenTime;
switch (Type)
{
case PeriodType.Daily:
return time[index].Date > time[index - 1].Date;
case PeriodType.Weekly:
return time[index].DayOfWeek == DayOfWeek.Monday && time[index - 1].DayOfWeek != DayOfWeek.Monday;
case PeriodType.Monthly:
return time[index].Month != time[index - 1].Month;
default:
throw new ArgumentOutOfRangeException();
}
}
}
}
Replies
sascha.dawe
24 Feb 2020, 13:20
( Updated at: 21 Dec 2023, 09:21 )
RE:
PanagiotisCharalampous said:
Hi sascha.dawe,
Can you explain to us what do you think is wrong?
Best Regards,
Panagiotis
Hi Panagiotis,
See two attached screenshots. Both show daily time frame VWAP on 1 hr chart. The reference indicator (VWAP) shows the band correctly. The other one (BVWAP) is warped. BVWAP includes two offset bands, but these can be ignored.
I would use the original VWAP indicator, but the time frame user input is not included in the source code at all, even though the source code is accessible?
What am I doing wrong here?
Thanks,
Sascha
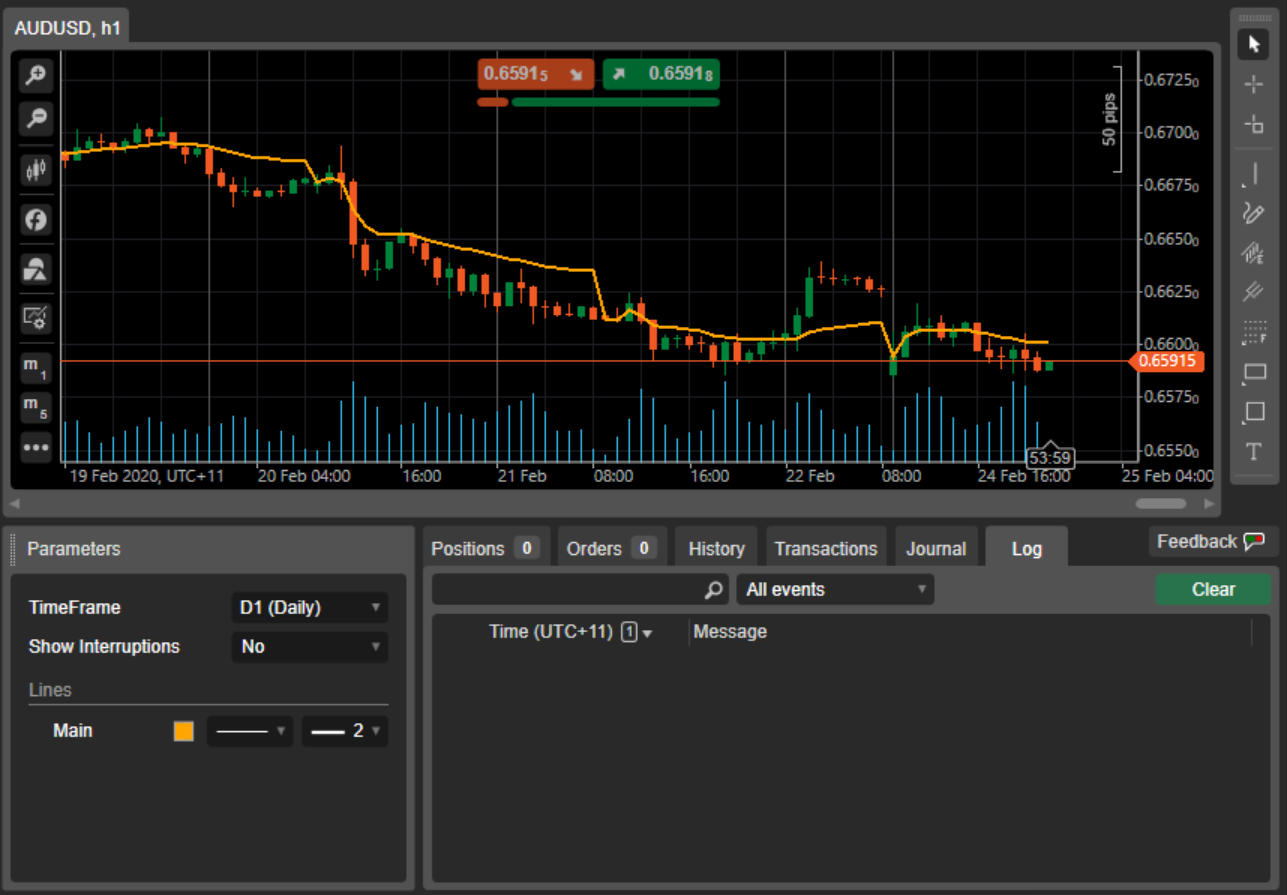
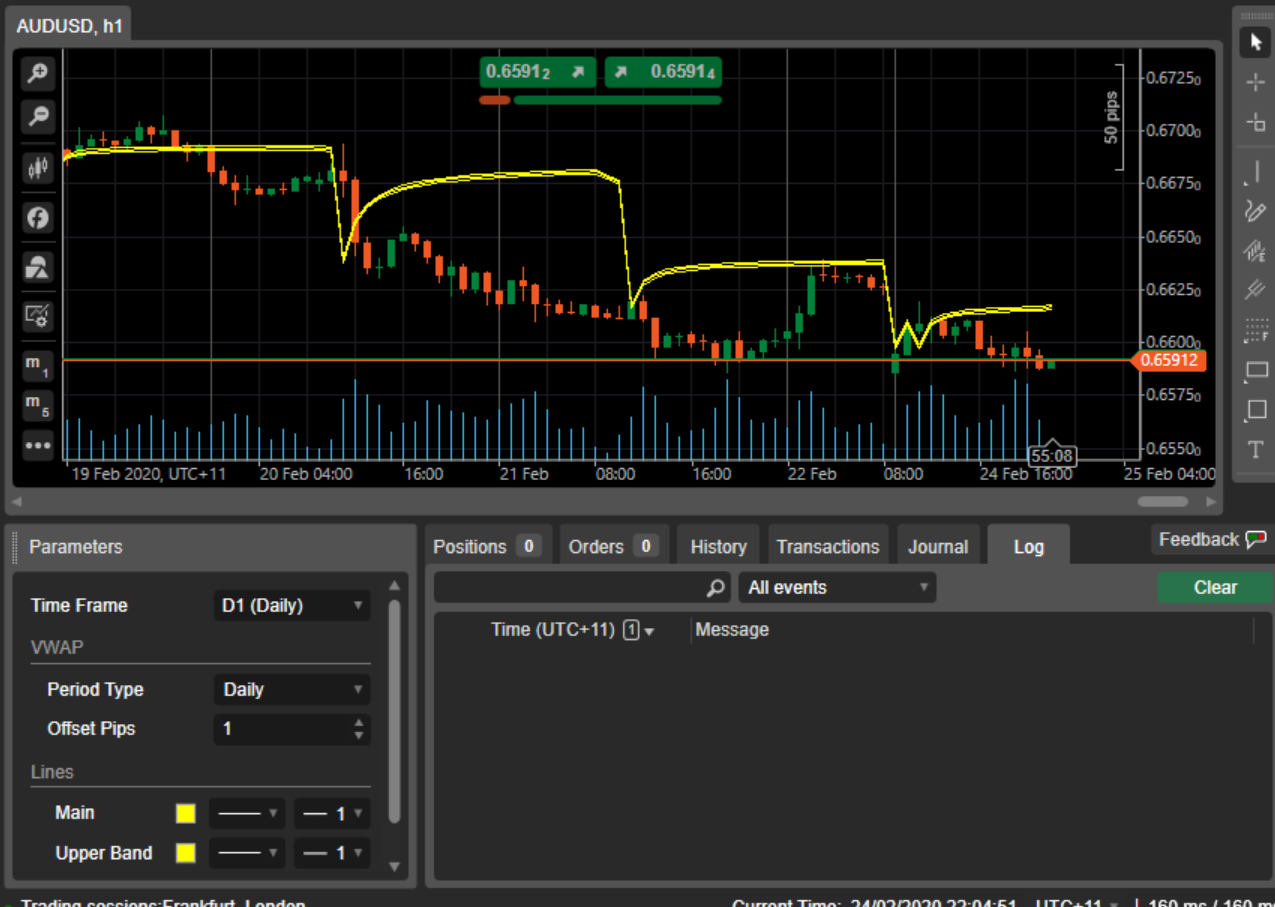
@sascha.dawe
PanagiotisCharalampous
24 Feb 2020, 10:02
Hi sascha.dawe,
Can you explain to us what do you think is wrong?
Best Regards,
Panagiotis
Join us on Telegram
@PanagiotisCharalampous