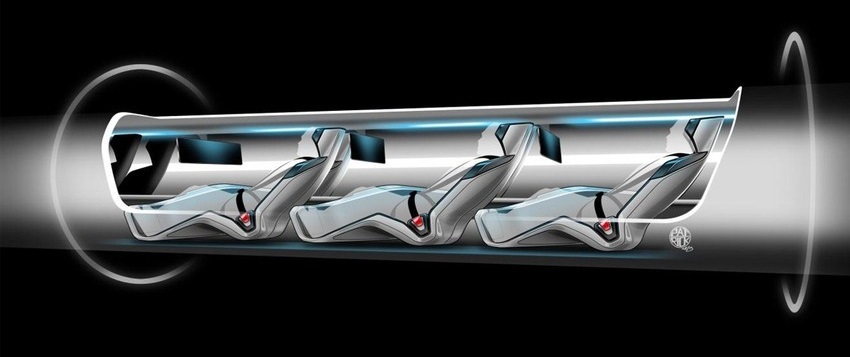
Multi-Timeframe results don't line up
25 Nov 2013, 22:55
I wrote an indicator that finds the net change between the current price and the open of "Lookback" periods ago. The code is below:
// ------------------------------------------------------------------------------- // // NetChange.cs // // ------------------------------------------------------------------------------- using System; using cAlgo.API; using cAlgo.API.Internals; using cAlgo.API.Indicators; namespace cAlgo.Indicators { [Indicator(IsOverlay = false, TimeZone = TimeZones.UTC)] public class NetChange : Indicator { [Parameter(DefaultValue = 30)] public int Lookback { get; set; } [Output("Main")] public IndicatorDataSeries Result { get; set; } public override void Calculate(int index) { double NetChange = MarketSeries.Close[index] - MarketSeries.Open[MarketSeries.Close.Count - Lookback]; Result[index] = NetChange * 10000; } } }
The code works as needed. But I am trying to make a multi-timeframe version of this but the results greatly differ from the original. The code for the multi-timeframe version is below:
// ------------------------------------------------------------------------------- // // NetChange MTF.cs // // ------------------------------------------------------------------------------- using System; using cAlgo.API; using cAlgo.API.Internals; using cAlgo.API.Indicators; namespace cAlgo.Indicators { [Indicator(IsOverlay = false, TimeZone = TimeZones.UTC)] public class NetChangeMTF : Indicator { [Parameter("Main Timeframe", DefaultValue = "Hour4")] public TimeFrame MainTimeframe { get; set; } [Parameter(DefaultValue = 30)] public int Lookback { get; set; } [Output("Main")] public IndicatorDataSeries Result { get; set; } private MarketSeries MainSeries; protected override void Initialize() { MainSeries = MarketData.GetSeries(MainTimeframe); } public override void Calculate(int index) { int MainIndex = GetIndexByDate(MarketSeries.OpenTime[index]); double NetChange = MainSeries.Close[MainIndex] - MainSeries.Open[MainSeries.Close.Count - Lookback]; Result[index] = NetChange * 10000; } private int GetIndexByDate(DateTime argDateTime) { for (int i = MainSeries.Close.Count - 1; i > 0; i--) { if (argDateTime == MainSeries.OpenTime[i] || (argDateTime > MainSeries.OpenTime[i] && argDateTime < MainSeries.OpenTime[i + 1])) return i; } return -1; } } }
When the NetChange MTF is placed on the same timeframe, it works just as well as the original NetChange.
Any help would be appreciated, thanks!
Replies
Hyperloop
27 Nov 2013, 21:47
I ended up fixing it by sacrificing the value for the current bar on MainSeries. It doesn't lose much as the current bar is still open and the value changes anyways and I trade mainly OnBar.
Revised code:
public override void Calculate(int index) { int MainIndex = GetIndexByDate(MarketSeries.OpenTime[index]); double NetChange = MainSeries.Close[MainIndex - 1] - MainSeries.Open[MainIndex - Lookback]; Result[index] = NetChange * 10000; }
@Hyperloop
Spotware
27 Nov 2013, 17:00
RE:
Hyperloop said:
The result on the chart will be different if a different Timeframe is used. The code looks fine.
@Spotware