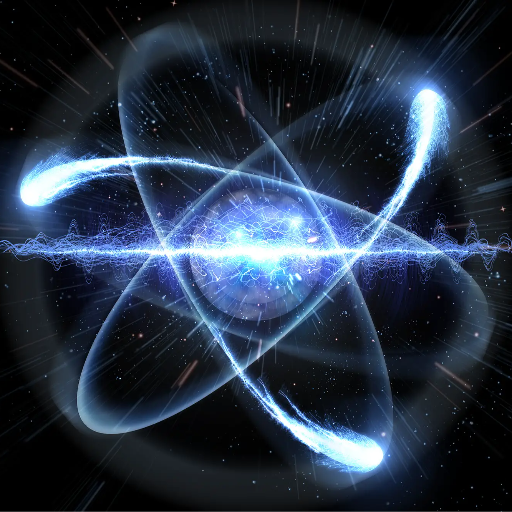
Consecutive Up and down bars
22 May 2019, 18:20
using System; using cAlgo.API; using cAlgo.API.Internals; using cAlgo.API.Indicators; using cAlgo.Indicators; namespace cAlgo { [Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class ConsecutiveDownAndUpBars : Indicator { [Parameter()] public DataSeries Source { get; set; } [Output("Up Bars", LineColor = "Green")] public IndicatorDataSeries UpBarsResult { get; set; } [Output("Down Bars", LineColor = "Red")] public IndicatorDataSeries DownBarsResult { get; set; } public int UpBars; public int DownBars; public override void Calculate(int index) { if (MarketSeries.Close.LastValue > MarketSeries.Open.LastValue) { UpBarsResult[index] = UpBars++; DownBarsResult[index] = 0; DownBars = 0; } else { DownBarsResult[index] = DownBars++; UpBarsResult[index] = 0; UpBars = 0; } } } }
Making my first indicator here, trying to have total number of down bars ina row and number of up bars in a row, but it just keeps adding +1 every tick.
Thanks for help.
Replies
jani
02 Nov 2019, 19:34
Add this to Calculate:
public override void Calculate(int index) { if (IsLastBar) { if (IsLastBar) { if (index != lastindex) lastindex = index; else return; }
...And to Parameters:
int lastindex = 0;
That should do it
If I only need values on closed bars. I use this to save CPU resources.
@jani
PanagiotisCharalampous
23 May 2019, 09:58
Hi wisegprs,
Calculate() gets triggered on each tick, that's why. You need to run your code only when the bar count changes.
Best Regards,
Panagiotis
@PanagiotisCharalampous