FIX SocketSSL
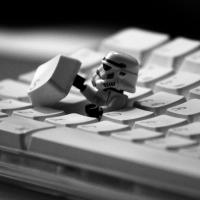
FIX SocketSSL
01 Sep 2016, 17:57
Hi how connect and get data from server ???
It is my code:
using System; using System.Collections.Generic; using System.Linq; using System.Net; using System.Net.Security; using System.Net.Sockets; using System.Security.Cryptography.X509Certificates; using System.Text; using System.Threading; using System.Threading.Tasks; namespace FIX { class Program { public static string host = "h51.p.ctrader.com"; static void Main(string[] args) { while (true) { StartClient(host); Thread.Sleep(5000); } } public static void StartClient(string host) { // Data buffer for incoming data. byte[] bytes = new byte[1024]; // Connect to a remote device. try { IPHostEntry ipHostInfo = Dns.GetHostEntry(host); IPAddress ipAddress = ipHostInfo.AddressList[0]; Console.WriteLine(ipAddress); TcpClient client = new TcpClient(host, 5201); SslStream sslStream = new SslStream(client.GetStream(), false, new RemoteCertificateValidationCallback(ValidateServerCertificate), null); try { sslStream.AuthenticateAsClient(host); //sslStream.AuthenticateAsClientAsync(host); } catch (Exception e) { Console.WriteLine(e); client.Close(); return; } // Connect the socket to the remote endpoint. Catch any errors. try { byte[] messsage = Encoding.UTF8.GetBytes("8=FIX.4.4|9=102|35=A|34=1|49=fxpro.9910892|50=Quote|52=20131129-15:40:10.276|56=cServer|98=0|108=30|141=Y|553=1047|554=PASSWORD|10=140|"); // Send hello message to the server. sslStream.Write(messsage); sslStream.Flush(); // Read message from the server. string serverMessage = ReadMessage(sslStream); Console.WriteLine("Server says: {0}", serverMessage); //Console.WriteLine("SERVER SEND: {0}", Encoding.ASCII.GetString(bytes, 0, bytesRec)); } catch (ArgumentNullException ane) { Console.WriteLine("ArgumentNullException : {0}", ane.ToString()); } catch (SocketException se) { Console.WriteLine("SocketException : {0}", se.ToString()); } catch (Exception e) { Console.WriteLine("Unexpected exception : {0}", e.ToString()); } } catch (Exception e) { Console.WriteLine(e.ToString()); } } static string ReadMessage(SslStream sslStream) { byte[] buffer = new byte[2048]; StringBuilder messageData = new StringBuilder(); int bytes = -1; do { bytes = sslStream.Read(buffer, 0, buffer.Length); Decoder decoder = Encoding.UTF8.GetDecoder(); char[] chars = new char[decoder.GetCharCount(buffer, 0, bytes)]; decoder.GetChars(buffer, 0, bytes, chars, 0); messageData.Append(chars); Console.WriteLine(chars); // Check for EOF. if (messageData.ToString().IndexOf(" ") != -1) { break; } } while (bytes != 0); return messageData.ToString(); } // The following method is invoked by the RemoteCertificateValidationDelegate. public static bool ValidateServerCertificate(object sender, X509Certificate certificate, X509Chain chain, SslPolicyErrors sslPolicyErrors) { if (sslPolicyErrors == SslPolicyErrors.None) return true; Console.WriteLine("Certificate error: {0}", sslPolicyErrors); // Do not allow this client to communicate with unauthenticated servers. //return false; //Force ssl certyfikates as correct return true; } } }
Thanks for the help
Replies
mindbreaker
01 Sep 2016, 20:48
Fix SSL
Thanks
I need example with C# and SSL (Financial app whitout ssl its stupid).
I don't like Java and Android but:
You can show example.
Nice day
@mindbreaker
ianj
01 Sep 2016, 21:00
RE: Fix SSL
You got a good point there about SSL - the only time i have ever seen a non SSL connection is within a data centre where the endpoints are physically known and the routing is dedicated or at least private
Am sure you can find a FIX engine in C#
Try looking here https://en.wikipedia.org/wiki/QuickFIX
mindbreaker said:
Thanks
I need example with C# and SSL (Financial app whitout ssl its stupid).
I don't like Java and Android but:
You can show example.
Nice day
@ianj
tetra
10 Sep 2016, 00:23
RE: RE: Fix SSL
No SSL certificate provided, without that it is not possible to use secure SSL connection.
Without SSL the price can be intercepted and twisted. This gives the possibility for the broker to use a virtual dealer plugin type software.
ianj said:
You got a good point there about SSL - the only time i have ever seen a non SSL connection is within a data centre where the endpoints are physically known and the routing is dedicated or at least private
Am sure you can find a FIX engine in C#
Try looking here https://en.wikipedia.org/wiki/QuickFIX
mindbreaker said:
Thanks
I need example with C# and SSL (Financial app whitout ssl its stupid).
I don't like Java and Android but:
You can show example.
Nice day
@tetra
tetra
10 Sep 2016, 00:27
RE:
This might be a little help but the fix separator is 0x01.
Use Replace('|', (char)1) on the strings that you want to send.
With the example below:
"8=FIX.4.4|9=102|35=A|34=1|49=fxpro.9910892|50=Quote|52=20131129-15:40:10.276|56=cServer|98=0|108=30|141=Y|553=1047|554=PASSWORD|10=140|".Replace('|', (char)1)
mindbreaker said:
Hi how connect and get data from server ???
It is my code:
using System; using System.Collections.Generic; using System.Linq; using System.Net; using System.Net.Security; using System.Net.Sockets; using System.Security.Cryptography.X509Certificates; using System.Text; using System.Threading; using System.Threading.Tasks; namespace FIX { class Program { public static string host = "h51.p.ctrader.com"; static void Main(string[] args) { while (true) { StartClient(host); Thread.Sleep(5000); } } public static void StartClient(string host) { // Data buffer for incoming data. byte[] bytes = new byte[1024]; // Connect to a remote device. try { IPHostEntry ipHostInfo = Dns.GetHostEntry(host); IPAddress ipAddress = ipHostInfo.AddressList[0]; Console.WriteLine(ipAddress); TcpClient client = new TcpClient(host, 5201); SslStream sslStream = new SslStream(client.GetStream(), false, new RemoteCertificateValidationCallback(ValidateServerCertificate), null); try { sslStream.AuthenticateAsClient(host); //sslStream.AuthenticateAsClientAsync(host); } catch (Exception e) { Console.WriteLine(e); client.Close(); return; } // Connect the socket to the remote endpoint. Catch any errors. try { byte[] messsage = Encoding.UTF8.GetBytes("8=FIX.4.4|9=102|35=A|34=1|49=fxpro.9910892|50=Quote|52=20131129-15:40:10.276|56=cServer|98=0|108=30|141=Y|553=1047|554=PASSWORD|10=140|"); // Send hello message to the server. sslStream.Write(messsage); sslStream.Flush(); // Read message from the server. string serverMessage = ReadMessage(sslStream); Console.WriteLine("Server says: {0}", serverMessage); //Console.WriteLine("SERVER SEND: {0}", Encoding.ASCII.GetString(bytes, 0, bytesRec)); } catch (ArgumentNullException ane) { Console.WriteLine("ArgumentNullException : {0}", ane.ToString()); } catch (SocketException se) { Console.WriteLine("SocketException : {0}", se.ToString()); } catch (Exception e) { Console.WriteLine("Unexpected exception : {0}", e.ToString()); } } catch (Exception e) { Console.WriteLine(e.ToString()); } } static string ReadMessage(SslStream sslStream) { byte[] buffer = new byte[2048]; StringBuilder messageData = new StringBuilder(); int bytes = -1; do { bytes = sslStream.Read(buffer, 0, buffer.Length); Decoder decoder = Encoding.UTF8.GetDecoder(); char[] chars = new char[decoder.GetCharCount(buffer, 0, bytes)]; decoder.GetChars(buffer, 0, bytes, chars, 0); messageData.Append(chars); Console.WriteLine(chars); // Check for EOF. if (messageData.ToString().IndexOf(" ") != -1) { break; } } while (bytes != 0); return messageData.ToString(); } // The following method is invoked by the RemoteCertificateValidationDelegate. public static bool ValidateServerCertificate(object sender, X509Certificate certificate, X509Chain chain, SslPolicyErrors sslPolicyErrors) { if (sslPolicyErrors == SslPolicyErrors.None) return true; Console.WriteLine("Certificate error: {0}", sslPolicyErrors); // Do not allow this client to communicate with unauthenticated servers. //return false; //Force ssl certyfikates as correct return true; } } }Thanks for the help
@tetra
ianj
10 Sep 2016, 00:59
RE: RE: RE: Fix SSL
The broker can just play with the messages in the engine if they want to - intercepting FIX is the last thing they would look at. I think in this case the servers are owned by spotware and leased to brokers but am not really sure.
The main problem is that the credentials can be captured/intercepted by intermediaries on the public internet - they can then login and do what they like
tetra said:
No SSL certificate provided, without that it is not possible to use secure SSL connection.
Without SSL the price can be intercepted and twisted. This gives the possibility for the broker to use a virtual dealer plugin type software.
@ianj
tetra
10 Sep 2016, 13:45
RE:
Hi mindbreaker,
Few notes about your code:
-The fix message body length is wrong in the example
-The fix message checksum number is also wrong
-Use proper time: /52=/ DateTime.Now.ToUniversalTime().ToString("yyyyMMdd-hh:mm:ss.fff")
-Since there is no ssl, use client.GetStream() directly
-Do not forget to change the separator / .Replace('|', '\u0001') /
if you fix these, your code will work.
mindbreaker said:
Hi how connect and get data from server ???
It is my code:
using System; using System.Collections.Generic; using System.Linq; using System.Net; using System.Net.Security; using System.Net.Sockets; using System.Security.Cryptography.X509Certificates; using System.Text; using System.Threading; using System.Threading.Tasks; namespace FIX { class Program { public static string host = "h51.p.ctrader.com"; static void Main(string[] args) { while (true) { StartClient(host); Thread.Sleep(5000); } } public static void StartClient(string host) { // Data buffer for incoming data. byte[] bytes = new byte[1024]; // Connect to a remote device. try { IPHostEntry ipHostInfo = Dns.GetHostEntry(host); IPAddress ipAddress = ipHostInfo.AddressList[0]; Console.WriteLine(ipAddress); TcpClient client = new TcpClient(host, 5201); SslStream sslStream = new SslStream(client.GetStream(), false, new RemoteCertificateValidationCallback(ValidateServerCertificate), null); try { sslStream.AuthenticateAsClient(host); //sslStream.AuthenticateAsClientAsync(host); } catch (Exception e) { Console.WriteLine(e); client.Close(); return; } // Connect the socket to the remote endpoint. Catch any errors. try { byte[] messsage = Encoding.UTF8.GetBytes("8=FIX.4.4|9=102|35=A|34=1|49=fxpro.9910892|50=Quote|52=20131129-15:40:10.276|56=cServer|98=0|108=30|141=Y|553=1047|554=PASSWORD|10=140|"); // Send hello message to the server. sslStream.Write(messsage); sslStream.Flush(); // Read message from the server. string serverMessage = ReadMessage(sslStream); Console.WriteLine("Server says: {0}", serverMessage); //Console.WriteLine("SERVER SEND: {0}", Encoding.ASCII.GetString(bytes, 0, bytesRec)); } catch (ArgumentNullException ane) { Console.WriteLine("ArgumentNullException : {0}", ane.ToString()); } catch (SocketException se) { Console.WriteLine("SocketException : {0}", se.ToString()); } catch (Exception e) { Console.WriteLine("Unexpected exception : {0}", e.ToString()); } } catch (Exception e) { Console.WriteLine(e.ToString()); } } static string ReadMessage(SslStream sslStream) { byte[] buffer = new byte[2048]; StringBuilder messageData = new StringBuilder(); int bytes = -1; do { bytes = sslStream.Read(buffer, 0, buffer.Length); Decoder decoder = Encoding.UTF8.GetDecoder(); char[] chars = new char[decoder.GetCharCount(buffer, 0, bytes)]; decoder.GetChars(buffer, 0, bytes, chars, 0); messageData.Append(chars); Console.WriteLine(chars); // Check for EOF. if (messageData.ToString().IndexOf(" ") != -1) { break; } } while (bytes != 0); return messageData.ToString(); } // The following method is invoked by the RemoteCertificateValidationDelegate. public static bool ValidateServerCertificate(object sender, X509Certificate certificate, X509Chain chain, SslPolicyErrors sslPolicyErrors) { if (sslPolicyErrors == SslPolicyErrors.None) return true; Console.WriteLine("Certificate error: {0}", sslPolicyErrors); // Do not allow this client to communicate with unauthenticated servers. //return false; //Force ssl certyfikates as correct return true; } } }Thanks for the help
@tetra
mindbreaker
10 Sep 2016, 19:18
RE: RE:
Hi tetra I know but:
1) I dont know how check message body length (Which part)
-The fix message body length is wrong in the example
2) Checksum this example:
https://github.com/fxstar/Chash/blob/master/FIX4.4/Checksum.cs
string message = "8=FIX.4.1|9=61|35=A|34=1|49=EXEC|52=20121105-23:24:06|" + "56=BANZAI|98=0|108=30|"; message = message.Replace('|', '\u0001'); byte[] messageBytes = Encoding.ASCII.GetBytes(message); for (int i = 0; i < message.Length; i++) total += messageBytes[i]; int checksum = total % 256; string checksumStr = checksum.ToString().PadLeft(3, '0');
3) if I check ssl there was on server(multicard ssl) why i can't use SSL?
Thanks for the help
tetra said:
Hi mindbreaker,
Few notes about your code:
-The fix message body length is wrong in the example
-The fix message checksum number is also wrong
-Use proper time: /52=/ DateTime.Now.ToUniversalTime().ToString("yyyyMMdd-hh:mm:ss.fff")
-Since there is no ssl, use client.GetStream() directly
-Do not forget to change the separator / .Replace('|', '\u0001') /
if you fix these, your code will work.
mindbreaker said:
Hi how connect and get data from server ???
It is my code:
using System; using System.Collections.Generic; using System.Linq; using System.Net; using System.Net.Security; using System.Net.Sockets; using System.Security.Cryptography.X509Certificates; using System.Text; using System.Threading; using System.Threading.Tasks; namespace FIX { class Program { public static string host = "h51.p.ctrader.com"; static void Main(string[] args) { while (true) { StartClient(host); Thread.Sleep(5000); } } public static void StartClient(string host) { // Data buffer for incoming data. byte[] bytes = new byte[1024]; // Connect to a remote device. try { IPHostEntry ipHostInfo = Dns.GetHostEntry(host); IPAddress ipAddress = ipHostInfo.AddressList[0]; Console.WriteLine(ipAddress); TcpClient client = new TcpClient(host, 5201); SslStream sslStream = new SslStream(client.GetStream(), false, new RemoteCertificateValidationCallback(ValidateServerCertificate), null); try { sslStream.AuthenticateAsClient(host); //sslStream.AuthenticateAsClientAsync(host); } catch (Exception e) { Console.WriteLine(e); client.Close(); return; } // Connect the socket to the remote endpoint. Catch any errors. try { byte[] messsage = Encoding.UTF8.GetBytes("8=FIX.4.4|9=102|35=A|34=1|49=fxpro.9910892|50=Quote|52=20131129-15:40:10.276|56=cServer|98=0|108=30|141=Y|553=1047|554=PASSWORD|10=140|"); // Send hello message to the server. sslStream.Write(messsage); sslStream.Flush(); // Read message from the server. string serverMessage = ReadMessage(sslStream); Console.WriteLine("Server says: {0}", serverMessage); //Console.WriteLine("SERVER SEND: {0}", Encoding.ASCII.GetString(bytes, 0, bytesRec)); } catch (ArgumentNullException ane) { Console.WriteLine("ArgumentNullException : {0}", ane.ToString()); } catch (SocketException se) { Console.WriteLine("SocketException : {0}", se.ToString()); } catch (Exception e) { Console.WriteLine("Unexpected exception : {0}", e.ToString()); } } catch (Exception e) { Console.WriteLine(e.ToString()); } } static string ReadMessage(SslStream sslStream) { byte[] buffer = new byte[2048]; StringBuilder messageData = new StringBuilder(); int bytes = -1; do { bytes = sslStream.Read(buffer, 0, buffer.Length); Decoder decoder = Encoding.UTF8.GetDecoder(); char[] chars = new char[decoder.GetCharCount(buffer, 0, bytes)]; decoder.GetChars(buffer, 0, bytes, chars, 0); messageData.Append(chars); Console.WriteLine(chars); // Check for EOF. if (messageData.ToString().IndexOf(" ") != -1) { break; } } while (bytes != 0); return messageData.ToString(); } // The following method is invoked by the RemoteCertificateValidationDelegate. public static bool ValidateServerCertificate(object sender, X509Certificate certificate, X509Chain chain, SslPolicyErrors sslPolicyErrors) { if (sslPolicyErrors == SslPolicyErrors.None) return true; Console.WriteLine("Certificate error: {0}", sslPolicyErrors); // Do not allow this client to communicate with unauthenticated servers. //return false; //Force ssl certyfikates as correct return true; } } }Thanks for the help
@mindbreaker
tetra
10 Sep 2016, 20:13
RE: RE: RE:
Body is after the 9 section, without checksum part:
8=FIX.4.4|9=102| 35=A|34=1|49=fxpro.9910892|50=Quote|52=20131129-15:40:10.276|56=cServer|98=0|108=30|141=Y|553=1047|554=PASSWORD|
ssl: there is no ssl on port 5201 -> https://www.htbridge.com/ssl/?id=a65c629cbd93adf53071f4be8d1fe92e31fbeedc5bfc9cc93796139487b117c1
mindbreaker said:
Hi tetra I know but:
1) I dont know how check message body length (Which part)
-The fix message body length is wrong in the example
2) Checksum this example:
https://github.com/fxstar/Chash/blob/master/FIX4.4/Checksum.cs
string message = "8=FIX.4.1|9=61|35=A|34=1|49=EXEC|52=20121105-23:24:06|" + "56=BANZAI|98=0|108=30|"; message = message.Replace('|', '\u0001'); byte[] messageBytes = Encoding.ASCII.GetBytes(message); for (int i = 0; i < message.Length; i++) total += messageBytes[i]; int checksum = total % 256; string checksumStr = checksum.ToString().PadLeft(3, '0');
3) if I check ssl there was on server(multicard ssl) why i can't use SSL?
Thanks for the help
tetra said:
Hi mindbreaker,
Few notes about your code:
-The fix message body length is wrong in the example
-The fix message checksum number is also wrong
-Use proper time: /52=/ DateTime.Now.ToUniversalTime().ToString("yyyyMMdd-hh:mm:ss.fff")
-Since there is no ssl, use client.GetStream() directly
-Do not forget to change the separator / .Replace('|', '\u0001') /
if you fix these, your code will work.
mindbreaker said:
Hi how connect and get data from server ???
It is my code:
using System; using System.Collections.Generic; using System.Linq; using System.Net; using System.Net.Security; using System.Net.Sockets; using System.Security.Cryptography.X509Certificates; using System.Text; using System.Threading; using System.Threading.Tasks; namespace FIX { class Program { public static string host = "h51.p.ctrader.com"; static void Main(string[] args) { while (true) { StartClient(host); Thread.Sleep(5000); } } public static void StartClient(string host) { // Data buffer for incoming data. byte[] bytes = new byte[1024]; // Connect to a remote device. try { IPHostEntry ipHostInfo = Dns.GetHostEntry(host); IPAddress ipAddress = ipHostInfo.AddressList[0]; Console.WriteLine(ipAddress); TcpClient client = new TcpClient(host, 5201); SslStream sslStream = new SslStream(client.GetStream(), false, new RemoteCertificateValidationCallback(ValidateServerCertificate), null); try { sslStream.AuthenticateAsClient(host); //sslStream.AuthenticateAsClientAsync(host); } catch (Exception e) { Console.WriteLine(e); client.Close(); return; } // Connect the socket to the remote endpoint. Catch any errors. try { byte[] messsage = Encoding.UTF8.GetBytes("8=FIX.4.4|9=102|35=A|34=1|49=fxpro.9910892|50=Quote|52=20131129-15:40:10.276|56=cServer|98=0|108=30|141=Y|553=1047|554=PASSWORD|10=140|"); // Send hello message to the server. sslStream.Write(messsage); sslStream.Flush(); // Read message from the server. string serverMessage = ReadMessage(sslStream); Console.WriteLine("Server says: {0}", serverMessage); //Console.WriteLine("SERVER SEND: {0}", Encoding.ASCII.GetString(bytes, 0, bytesRec)); } catch (ArgumentNullException ane) { Console.WriteLine("ArgumentNullException : {0}", ane.ToString()); } catch (SocketException se) { Console.WriteLine("SocketException : {0}", se.ToString()); } catch (Exception e) { Console.WriteLine("Unexpected exception : {0}", e.ToString()); } } catch (Exception e) { Console.WriteLine(e.ToString()); } } static string ReadMessage(SslStream sslStream) { byte[] buffer = new byte[2048]; StringBuilder messageData = new StringBuilder(); int bytes = -1; do { bytes = sslStream.Read(buffer, 0, buffer.Length); Decoder decoder = Encoding.UTF8.GetDecoder(); char[] chars = new char[decoder.GetCharCount(buffer, 0, bytes)]; decoder.GetChars(buffer, 0, bytes, chars, 0); messageData.Append(chars); Console.WriteLine(chars); // Check for EOF. if (messageData.ToString().IndexOf(" ") != -1) { break; } } while (bytes != 0); return messageData.ToString(); } // The following method is invoked by the RemoteCertificateValidationDelegate. public static bool ValidateServerCertificate(object sender, X509Certificate certificate, X509Chain chain, SslPolicyErrors sslPolicyErrors) { if (sslPolicyErrors == SslPolicyErrors.None) return true; Console.WriteLine("Certificate error: {0}", sslPolicyErrors); // Do not allow this client to communicate with unauthenticated servers. //return false; //Force ssl certyfikates as correct return true; } } }Thanks for the help
@tetra
mindbreaker
11 Sep 2016, 10:10
RE: RE: RE: RE:
Hi
Thank you for your explanation. If however this fix works without SSL it does not interest me such a flawed system.
Thanks for the help
Ps.
I think it display certs info (but maybe on 443 port I see in monday.)
static void DisplayCertificateInformation(SslStream stream) { Console.WriteLine("Certificate revocation list checked: {0}", stream.CheckCertRevocationStatus); X509Certificate localCertificate = stream.LocalCertificate; if (stream.LocalCertificate != null) { Console.WriteLine("Local cert was issued to {0} and is valid from {1} until {2}.", localCertificate.Subject, localCertificate.GetEffectiveDateString(), localCertificate.GetExpirationDateString()); } else { Console.WriteLine("Local certificate is null."); } // Display the properties of the client's certificate. X509Certificate remoteCertificate = stream.RemoteCertificate; if (stream.RemoteCertificate != null) { Console.WriteLine("Remote cert was issued to {0} and is valid from {1} until {2}.", remoteCertificate.Subject, remoteCertificate.GetEffectiveDateString(), remoteCertificate.GetExpirationDateString()); } else { Console.WriteLine("Remote certificate is null."); } }
Nice day
@mindbreaker
tetra
11 Sep 2016, 11:14
RE: RE: RE: RE: RE:
I agree, FIX without SSL is a joke.
Try to reach someone competent if you can:
https://www.linkedin.com/in/andrey-pavlov-38ba746
https://www.linkedin.com/in/vladimir-galaiko-6689087b
https://www.linkedin.com/in/james-glyde-2b507164
their regular support give only canned answers, and might not even understand what the problem is.
mindbreaker said:
Hi
Thank you for your explanation. If however this fix works without SSL it does not interest me such a flawed system.
Thanks for the help
Ps.
I think it display certs info (but maybe on 443 port I see in monday.)
static void DisplayCertificateInformation(SslStream stream) { Console.WriteLine("Certificate revocation list checked: {0}", stream.CheckCertRevocationStatus); X509Certificate localCertificate = stream.LocalCertificate; if (stream.LocalCertificate != null) { Console.WriteLine("Local cert was issued to {0} and is valid from {1} until {2}.", localCertificate.Subject, localCertificate.GetEffectiveDateString(), localCertificate.GetExpirationDateString()); } else { Console.WriteLine("Local certificate is null."); } // Display the properties of the client's certificate. X509Certificate remoteCertificate = stream.RemoteCertificate; if (stream.RemoteCertificate != null) { Console.WriteLine("Remote cert was issued to {0} and is valid from {1} until {2}.", remoteCertificate.Subject, remoteCertificate.GetEffectiveDateString(), remoteCertificate.GetExpirationDateString()); } else { Console.WriteLine("Remote certificate is null."); } }Nice day
@tetra
ianj
11 Sep 2016, 14:17
RE: RE: RE: RE:
I know its repetition but another view always helps:
http://stackoverflow.com/questions/32708068/how-to-calculate-checksum-in-fix-manually
and
Body length
Body length is the character count starting at tag 35 (included) all the way to tag 10 (excluded). SOH delimiters do count in body length.
For Example: (SOH have been replaced by'|')
8=FIX.4.2|9=65|35=A|49=SERVER|56=CLIENT|34=177|52=20090107-18:15:16|98=0|108=30|10=062| 0 + 0 + 5 + 10 + 10 + 7 + 21 + 5 + 7 + 0 = 65
Has a Body length of 65.
The SOH delimiter at the end of a Tag=Value belongs to the Tag.
Checksum
The checksum algorithm of FIX consists of summing up the decimal value of the ASCII representation all the bytes up to but not including the checksum field (which is last) and return the value modulo 256.
@ianj
mindbreaker
13 Sep 2016, 11:33
FIX message
Hi, is it correct FIX message?
string time = DateTime.UtcNow.ToString("yyyyMMdd-hh:mm:ss.fff"); string body = "35=A|34=1|49=fxpro.9910892|554=PASSWORD|50=Quote|52=" + time + "|56=cServer|98=0|108=30|141=Y|553=1047|"; // body length int bodylen = body.Length; string fix = "8=FIX.4.4|9=" + bodylen + "|"; // message string message = fix + body; message = message.Replace('|', '\u0001'); byte[] messageBytes = Encoding.ASCII.GetBytes(message); for (int i = 0; i < message.Length; i++) total += messageBytes[i]; int checksum = total % 256; string checksumStr = checksum.ToString().PadLeft(3, '0'); string sum = "10=" + checksumStr + "|"; string m = fix + body + sum; Console.WriteLine(m);
Thanks
@mindbreaker
mindbreaker
13 Sep 2016, 11:40
Hi Spotware,
where is a simple example, how to send data to the server via FIX and how connect to the server over SSL socket?
What is wrong with my example ?
using System; using System.Collections.Generic; using System.Linq; using System.Net; using System.Net.Security; using System.Net.Sockets; using System.Security.Cryptography.X509Certificates; using System.Text; using System.Threading; using System.Threading.Tasks; namespace FIX { class Program { public static string host = "h51.p.ctrader.com"; public static int total; static void Main(string[] args) { while (true) { StartClient(host); Thread.Sleep(5000); } } public static void StartClient(string host) { string time = DateTime.UtcNow.ToString("yyyyMMdd-hh:mm:ss.fff"); string body = "35=A|34=1|49=fxpro.9910892|554=PASSWORD|50=Quote|52=" + time + "|56=cServer|98=0|108=30|141=Y|553=1047|"; // body length int bodylen = body.Length; string fix = "8=FIX.4.4|9=" + bodylen + "|"; // message string message = fix + body; message = message.Replace('|', '\u0001'); byte[] messageBytes = Encoding.ASCII.GetBytes(message); for (int i = 0; i < message.Length; i++) total += messageBytes[i]; int checksum = total % 256; string checksumStr = checksum.ToString().PadLeft(3, '0'); string sum = "10=" + checksumStr + "|"; string m = fix + body + sum; m = m.Replace('|', '\u0001'); Console.WriteLine(m); Console.ReadKey(); // Data buffer for incoming data. byte[] bytes = new byte[1024]; // Connect to a remote device. try { IPHostEntry ipHostInfo = Dns.GetHostEntry(host); IPAddress ipAddress = ipHostInfo.AddressList[0]; Console.WriteLine(ipAddress); TcpClient client = new TcpClient(host, 5201); SslStream sslStream = new SslStream(client.GetStream(), false, new RemoteCertificateValidationCallback(ValidateServerCertificate), null); try { sslStream.AuthenticateAsClient(host); //sslStream.AuthenticateAsClientAsync(host); } catch (Exception e) { Console.WriteLine(e); client.Close(); return; } // Connect the socket to the remote endpoint. Catch any errors. try { //byte[] messsage = Encoding.UTF8.GetBytes("8=FIX.4.4|9=102|35=A|34=1|49=fxpro.9910892|50=Quote|52=20131129-15:40:10.276|56=cServer|98=0|108=30|141=Y|553=1047|554=PASSWORD|10=140|"); byte[] messsage = Encoding.UTF8.GetBytes(m); // Send hello message to the server. sslStream.Write(messsage); sslStream.Flush(); // Read message from the server. string serverMessage = ReadMessage(sslStream); Console.WriteLine("Server says: {0}", serverMessage); //Console.WriteLine("SERVER SEND: {0}", Encoding.ASCII.GetString(bytes, 0, bytesRec)); } catch (ArgumentNullException ane) { Console.WriteLine("ArgumentNullException : {0}", ane.ToString()); } catch (SocketException se) { Console.WriteLine("SocketException : {0}", se.ToString()); } catch (Exception e) { Console.WriteLine("Unexpected exception : {0}", e.ToString()); } } catch (Exception e) { Console.WriteLine(e.ToString()); } } static string ReadMessage(SslStream sslStream) { byte[] buffer = new byte[2048]; StringBuilder messageData = new StringBuilder(); int bytes = -1; do { bytes = sslStream.Read(buffer, 0, buffer.Length); Decoder decoder = Encoding.UTF8.GetDecoder(); char[] chars = new char[decoder.GetCharCount(buffer, 0, bytes)]; decoder.GetChars(buffer, 0, bytes, chars, 0); messageData.Append(chars); Console.WriteLine(chars); // Check for EOF. if (messageData.ToString().IndexOf(" ") != -1) { break; } } while (bytes != 0); return messageData.ToString(); } // The following method is invoked by the RemoteCertificateValidationDelegate. public static bool ValidateServerCertificate(object sender, X509Certificate certificate, X509Chain chain, SslPolicyErrors sslPolicyErrors) { if (sslPolicyErrors == SslPolicyErrors.None) return true; Console.WriteLine("Certificate error: {0}", sslPolicyErrors); // Do not allow this client to communicate with unauthenticated servers. //return false; //Force ssl certyfikates as correct return true; } } }
@mindbreaker
channel11
13 Sep 2016, 18:35
RE: RE: RE: RE:
Small corrections:
-Put the 554 section after the 553 block
-Little change in the time format: ToString("yyyyMMdd-HH:mm:ss.fff") (the hours need to be in 1-24 format)
-Since SSL is not enabled , you need to use client.GetStream() directly. (not sure if they read this forum too much, maybe they answer to your ssl question faster in email)
@channel11
mindbreaker
22 Sep 2016, 10:41
RE: RE: RE: RE: RE:
Hi FIX working login example (NO SSL)
using System; using System.Collections.Generic; using System.Linq; using System.Net; using System.Net.Sockets; using System.Text; using System.Threading; using System.Threading.Tasks; namespace FixNoSSL { public class FixSocketClient { // cserver ip fix public static string host = "80.86.83.5"; // fix server id public static string FixID = "fxpro.123456"; // ctrader account public static string Account = "9910892"; // ctrader password public static string Password = "PASSWORD"; public static int total; public static string m = ""; public static void StartClient(string host) { string time = DateTime.UtcNow.ToString("yyyyMMdd-HH:mm:ss.fff"); string body = "35=A|34=1|49="+FixID+"|50=QUOTE|52=" + time + "|56=CSERVER|98=0|108=30|553="+Account+"|554="+Password+"|"; // body length int bodylen = body.Length; string fix = "8=FIX.4.4|9=" + bodylen + "|"; // message string message = fix + body; message = message.Replace('|', '\u0001'); byte[] messageBytes = Encoding.ASCII.GetBytes(message); for (int i = 0; i < message.Length; i++) total += messageBytes[i]; int checksum = total % 256; string checksumStr = checksum.ToString().PadLeft(3, '0'); string sum = "10=" + checksumStr + "|"; string m = fix + body + sum; m = m.Replace('|', '\u0001'); Console.WriteLine("Send to server (hit any key) {0}", m); Console.ReadKey(); // Data buffer for incoming data. byte[] bytes = new byte[1024]; // Connect to a remote device. try { // Establish the remote endpoint for the socket. // This example uses port 11000 on the local computer. IPHostEntry ipHostInfo = Dns.GetHostEntry(host); IPAddress ipAddress = ipHostInfo.AddressList[0]; IPEndPoint remoteEP = new IPEndPoint(ipAddress, 5201); // Create a TCP/IP socket. Socket sender = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp); // Connect the socket to the remote endpoint. Catch any errors. try { sender.Connect(remoteEP); Console.WriteLine("SOCKET CONECTED {0}", sender.RemoteEndPoint.ToString()); // Encode the data string into a byte array. byte[] msg = Encoding.ASCII.GetBytes(m); // Send the data through the socket. int bytesSent = sender.Send(msg); // Receive the response from the remote device. int bytesRec = sender.Receive(bytes); Console.WriteLine("SERVER SEND >> {0}", Encoding.ASCII.GetString(bytes, 0, bytesRec)); // Release the socket. sender.Shutdown(SocketShutdown.Both); sender.Close(); } catch (ArgumentNullException ane) { Console.WriteLine("ArgumentNullException : {0}", ane.ToString()); } catch (SocketException se) { Console.WriteLine("SocketException : {0}", se.ToString()); } catch (Exception e) { Console.WriteLine("Unexpected exception : {0}", e.ToString()); } } catch (Exception e) { Console.WriteLine(e.ToString()); } } public static int Main(String[] args) { while (true) { StartClient(host); Thread.Sleep(5000); } } } }
But whats after login how send message (some example)?
@mindbreaker
tetra
22 Sep 2016, 13:56
RE: RE: RE: RE: RE: RE:
Here is a full book request example:
8=FIX.4.4|9={bodylength}|35=V|34=2|49={accountid}|52={time}|50=QUOTE|56=CSERVER|262={messageGuid}|263=1|264=0|267=1|269=0|146=1|55={symbolid}|10={checksum}|
mindbreaker said:
But whats after login how send message (some example)?
@tetra
mindbreaker
28 Sep 2016, 11:03
How open new order
Hi
How open trade is it correct?
public static string SetTrade(int buy = 1, long lot = 1000, long symbol = 1) { string id = md5(DateTime.UtcNow.ToString()); string time = DateTime.UtcNow.ToString("yyyyMMdd-HH:mm:ss.fff"); string body = "35=D|49="+FixID+"|56=cServer|34=2|52="+time+"|11="+id+"|55="+symbol+"|54="+buy+"|60="+time+"|38="+lot+"|40=1|44=1.12473|59=3|"; // body length int bodylen = body.Length; string fix = "8=FIX.4.4|9=" + bodylen + "|"; // message string message = fix + body; message = message.Replace('|', '\u0001'); byte[] messageBytes = Encoding.ASCII.GetBytes(message); for (int i = 0; i < message.Length; i++) total += messageBytes[i]; int checksum = total % 256; string checksumStr = checksum.ToString().PadLeft(3, '0'); string sum = "10=" + checksumStr + "|"; string m = fix + body + sum; m = m.Replace('|', '\u0001'); return m; }
@mindbreaker
mindbreaker
28 Sep 2016, 11:07
where is error n this example
using System; using System.Collections.Generic; using System.Linq; using System.Net; using System.Net.Sockets; using System.Text; using System.Threading; using System.Threading.Tasks; using System.IO; using System.Security.Cryptography; namespace FixNoSSL { public class FixSocketClient { // cserver ip fix public static string host = "46.105.103.224"; // fix server id public static string FixID = "fxpro.9910892"; // ctrader account public static string Account = "9910892"; // ctrader password public static string Password = "ajdsakdhkash"; public static int total; public static string mm = ""; public static string md5(string input) { // step 1, calculate MD5 hash from input MD5 md5 = System.Security.Cryptography.MD5.Create(); byte[] inputBytes = System.Text.Encoding.ASCII.GetBytes(input); byte[] hash = md5.ComputeHash(inputBytes); // step 2, convert byte array to hex string StringBuilder sb = new StringBuilder(); for (int i = 0; i < hash.Length; i++) { sb.Append(hash[i].ToString("X2")); } return sb.ToString(); } public static string SetTrade(int buy = 1, long lot = 1000, long symbol = 1) { string id = md5(DateTime.UtcNow.ToString()); string time = DateTime.UtcNow.ToString("yyyyMMdd-HH:mm:ss.fff"); string body = "35=D|49="+FixID+"|56=cServer|34=2|52="+time+"|11="+id+"|55="+symbol+"|54="+buy+"|60="+time+"|38="+lot+"|40=1|44=1.12473|59=3|"; // body length int bodylen = body.Length; string fix = "8=FIX.4.4|9=" + bodylen + "|"; // message string message = fix + body; message = message.Replace('|', '\u0001'); byte[] messageBytes = Encoding.ASCII.GetBytes(message); for (int i = 0; i < message.Length; i++) total += messageBytes[i]; int checksum = total % 256; string checksumStr = checksum.ToString().PadLeft(3, '0'); string sum = "10=" + checksumStr + "|"; string m = fix + body + sum; m = m.Replace('|', '\u0001'); return m; } public static string GetStatus(int buy = 1, long lot = 1000, long symbol = 1) { string id = md5(DateTime.UtcNow.ToString()); string time = DateTime.UtcNow.ToString("yyyyMMdd-HH:mm:ss.fff"); string body = "35=H|49=" + FixID + "|56=cServer|34=2|11=" + id + "|55=" + symbol + "|54=" + buy + "|"; // body length int bodylen = body.Length; string fix = "8=FIX.4.4|9=" + bodylen + "|"; // message string message = fix + body; message = message.Replace('|', '\u0001'); byte[] messageBytes = Encoding.ASCII.GetBytes(message); for (int i = 0; i < message.Length; i++) total += messageBytes[i]; int checksum = total % 256; string checksumStr = checksum.ToString().PadLeft(3, '0'); string sum = "10=" + checksumStr + "|"; string m = fix + body + sum; m = m.Replace('|', '\u0001'); return m; } public static void StartClient(string host) { string time = DateTime.UtcNow.ToString("yyyyMMdd-HH:mm:ss.fff"); string body = "35=A|34=1|49="+FixID+"|50=QUOTE|52=" + time + "|56=CSERVER|98=0|108=30|553="+Account+"|554="+Password+"|"; // body length int bodylen = body.Length; string fix = "8=FIX.4.4|9=" + bodylen + "|"; // message string message = fix + body; message = message.Replace('|', '\u0001'); byte[] messageBytes = Encoding.ASCII.GetBytes(message); for (int i = 0; i < message.Length; i++) total += messageBytes[i]; int checksum = total % 256; string checksumStr = checksum.ToString().PadLeft(3, '0'); string sum = "10=" + checksumStr + "|"; string m = fix + body + sum; m = m.Replace('|', '\u0001'); Console.WriteLine("Send to server (hit any key) {0}", m); Console.ReadKey(); mm = m; // Data buffer for incoming data. byte[] bytes = new byte[1024]; // Connect to a remote device. try { // Establish the remote endpoint for the socket. // This example uses port 11000 on the local computer. IPHostEntry ipHostInfo = Dns.GetHostEntry(host); IPAddress ipAddress = ipHostInfo.AddressList[0]; IPEndPoint remoteEP = new IPEndPoint(ipAddress, 5202); // Create a TCP/IP socket. Socket sender = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp); // Connect the socket to the remote endpoint. Catch any errors. try { sender.Connect(remoteEP); Console.WriteLine("SOCKET CONECTED {0}", sender.RemoteEndPoint.ToString()); // Encode the data string into a byte array. byte[] msg = Encoding.ASCII.GetBytes(mm); // Send the data through the socket. int bytesSent = sender.Send(msg); // Receive the response from the remote device. int bytesRec = sender.Receive(bytes); Console.WriteLine("SERVER SEND >> {0}", Encoding.ASCII.GetString(bytes, 0, bytesRec)); //string ms = SetTrade(1,1000,1); string ms = GetStatus(1, 1000, 1); string desktop = Environment.GetFolderPath(Environment.SpecialFolder.Desktop); desktop = desktop + @"\cmd.txt"; File.WriteAllText(desktop,ms); Console.WriteLine("TRADE ADD "+ms); Console.ReadKey(); // Encode the data string into a byte array. byte[] msg1 = Encoding.ASCII.GetBytes(ms); // Send the data through the socket. int bytesSent1 = sender.Send(msg1); byte[] bytes1 = new byte[1024]; // Receive the response from the remote device. int bytesRec1 = sender.Receive(bytes1); Console.WriteLine("SERVER SEND (SET LOT) >> {0}", Encoding.ASCII.GetString(bytes1, 0, bytesRec1)); // Release the socket. sender.Shutdown(SocketShutdown.Both); sender.Close(); } catch (ArgumentNullException ane) { Console.WriteLine("ArgumentNullException : {0}", ane.ToString()); } catch (SocketException se) { Console.WriteLine("SocketException : {0}", se.ToString()); } catch (Exception e) { Console.WriteLine("Unexpected exception : {0}", e.ToString()); } } catch (Exception e) { Console.WriteLine(e.ToString()); } } public static int Main(String[] args) { while (true) { StartClient(host); Thread.Sleep(15000); } } } }
@mindbreaker
mindbreaker
04 Oct 2016, 20:59
RE:
darhk said:
Hi Mindbreaker,
In your working logon code (post #17), were you able to get a logon message as a response after you send your logon message? I have similar code done in Python, but when I try to send my message, the FIX server disconnects me.
Thanks
try send this line it is correct msg:
8=FIX.4.4|9=108|35=A|34=1|49=fxpro.9910892|50=QUOTE|52=20161004-17:53:27.083|56=CSERVER|98=0|108=30|553=9910892|554=uthj1gv|10=069
and my demo test account
// cserver ip fix public static string host = "46.105.103.224"; // fix server id public static string FixID = "fxpro.9910892"; // ctrader account public static string Account = "9910892";
mayby will work (i dont want install python on my vps)
@mindbreaker
mindbreaker
08 Feb 2017, 17:11
FIX DLL
// Decompiled with JetBrains decompiler // Type: FIX_API_Library.MessageConstructor // Assembly: FIX API Library, Version=1.0.0.0, Culture=neutral, PublicKeyToken=null // MVID: 34DF1E64-4374-4F03-8043-79A2A1904730 // Assembly location: C:\Users\ByczyPalowrson\Desktop\FIX-API-Sample-master\FIX API Library.dll using System; using System.Text; namespace FIX_API_Library { public class MessageConstructor { private string _host; private string _username; private string _password; private string _senderCompID; private string _senderSubID; private string _targetCompID; public MessageConstructor(string host, string username, string password, string senderCompID, string senderSubID, string targetCompID) { this._host = host; this._username = username; this._password = password; this._senderCompID = senderCompID; this._senderSubID = senderSubID; this._targetCompID = targetCompID; } public string LogonMessage(MessageConstructor.SessionQualifier qualifier, int messageSequenceNumber, int heartBeatSeconds, bool resetSeqNum) { StringBuilder stringBuilder = new StringBuilder(); stringBuilder.Append("98=0|"); stringBuilder.Append("108=" + (object) heartBeatSeconds + "|"); if (resetSeqNum) stringBuilder.Append("141=Y|"); stringBuilder.Append("553=" + this._username + "|"); stringBuilder.Append("554=" + this._password + "|"); string str1 = this.ConstructHeader(qualifier, this.SessionMessageCode(MessageConstructor.SessionMessageType.Logon), messageSequenceNumber, stringBuilder.ToString()); string str2 = this.ConstructTrailer(str1 + (object) stringBuilder); return (str1 + (object) stringBuilder + str2).Replace("|", "\x0001"); } public string HeartbeatMessage(MessageConstructor.SessionQualifier qualifier, int messageSequenceNumber) { string message = this.ConstructHeader(qualifier, this.SessionMessageCode(MessageConstructor.SessionMessageType.Heartbeat), messageSequenceNumber, string.Empty); string str = this.ConstructTrailer(message); return (message + str).Replace("|", "\x0001"); } public string TestRequestMessage(MessageConstructor.SessionQualifier qualifier, int messageSequenceNumber, int testRequestID) { StringBuilder stringBuilder = new StringBuilder(); stringBuilder.Append("112=" + (object) testRequestID + "|"); string str1 = this.ConstructHeader(qualifier, this.SessionMessageCode(MessageConstructor.SessionMessageType.TestRequest), messageSequenceNumber, stringBuilder.ToString()); string str2 = this.ConstructTrailer(str1 + (object) stringBuilder); return (str1 + (object) stringBuilder + str2).Replace("|", "\x0001"); } public string LogoutMessage(MessageConstructor.SessionQualifier qualifier, int messageSequenceNumber) { string message = this.ConstructHeader(qualifier, this.SessionMessageCode(MessageConstructor.SessionMessageType.Logout), messageSequenceNumber, string.Empty); string str = this.ConstructTrailer(message); return (message + str).Replace("|", "\x0001"); } public string ResendMessage(MessageConstructor.SessionQualifier qualifier, int messageSequenceNumber, int endSequenceNo) { StringBuilder stringBuilder = new StringBuilder(); stringBuilder.Append("16=" + (object) endSequenceNo + "|"); string str1 = this.ConstructHeader(qualifier, this.SessionMessageCode(MessageConstructor.SessionMessageType.Resend), messageSequenceNumber, stringBuilder.ToString()); string str2 = this.ConstructTrailer(str1 + (object) stringBuilder); return (str1 + (object) stringBuilder + str2).Replace("|", "\x0001"); } public string RejectMessage(MessageConstructor.SessionQualifier qualifier, int messageSequenceNumber, int rejectSequenceNumber) { StringBuilder stringBuilder = new StringBuilder(); stringBuilder.Append("45=" + (object) rejectSequenceNumber + "|"); string str1 = this.ConstructHeader(qualifier, this.SessionMessageCode(MessageConstructor.SessionMessageType.Reject), messageSequenceNumber, string.Empty); string str2 = this.ConstructTrailer(str1 + (object) stringBuilder); return (str1 + (object) stringBuilder + str2).Replace("|", "\x0001"); } public string SequenceResetMessage(MessageConstructor.SessionQualifier qualifier, int messageSequenceNumber, int rejectSequenceNumber) { StringBuilder stringBuilder = new StringBuilder(); stringBuilder.Append("36=" + (object) rejectSequenceNumber + "|"); string str1 = this.ConstructHeader(qualifier, this.SessionMessageCode(MessageConstructor.SessionMessageType.SequenceReset), messageSequenceNumber, string.Empty); string str2 = this.ConstructTrailer(str1 + (object) stringBuilder); return (str1 + (object) stringBuilder + str2).Replace("|", "\x0001"); } public string MarketDataRequestMessage(MessageConstructor.SessionQualifier qualifier, int messageSequenceNumber, string marketDataRequestID, int subscriptionRequestType, int marketDepth, int marketDataEntryType, int noRelatedSymbol, long symbol = 0) { StringBuilder stringBuilder = new StringBuilder(); stringBuilder.Append("262=" + marketDataRequestID + "|"); stringBuilder.Append("263=" + (object) subscriptionRequestType + "|"); stringBuilder.Append("264=" + (object) marketDepth + "|"); stringBuilder.Append("265=1|"); stringBuilder.Append("267=2|"); stringBuilder.Append("269=0|269=1|"); stringBuilder.Append("146=" + (object) noRelatedSymbol + "|"); stringBuilder.Append("55=" + (object) symbol + "|"); string str1 = this.ConstructHeader(qualifier, this.ApplicationMessageCode(MessageConstructor.ApplicationMessageType.MarketDataRequest), messageSequenceNumber, stringBuilder.ToString()); string str2 = this.ConstructTrailer(str1 + (object) stringBuilder); return (str1 + (object) stringBuilder + str2).Replace("|", "\x0001"); } public string MarketDataSnapshotMessage(MessageConstructor.SessionQualifier qualifier, int messageSequenceNumber, long symbol, string noMarketDataEntries, int entryType, Decimal entryPrice, string marketDataRequestID = "") { StringBuilder stringBuilder = new StringBuilder(); stringBuilder.Append("262=" + marketDataRequestID + "|"); stringBuilder.Append("55=" + (object) symbol + "|"); stringBuilder.Append("268=" + noMarketDataEntries + "|"); stringBuilder.Append("269=" + (object) entryType + "|"); stringBuilder.Append("270=" + (object) entryPrice + "|"); string str1 = this.ConstructHeader(qualifier, this.ApplicationMessageCode(MessageConstructor.ApplicationMessageType.MarketDataRequest), messageSequenceNumber, stringBuilder.ToString()); string str2 = this.ConstructTrailer(str1 + (object) stringBuilder); return (str1 + (object) stringBuilder + str2).Replace("|", "\x0001"); } public string MarketDataIncrementalRefreshMessage(MessageConstructor.SessionQualifier qualifier, int messageSequenceNumber, string marketDataRequestID, int noMarketDataEntries, int marketDataUpdateAction, int marketDataEntryType, string marketDataEntryID, int noRelatedSymbol, Decimal marketDataEntryPrice = 0M, double marketDataEntrySize = 0.0, long symbol = 0) { StringBuilder stringBuilder = new StringBuilder(); stringBuilder.Append("262=" + marketDataRequestID + "|"); stringBuilder.Append("268=" + (object) noMarketDataEntries + "|"); stringBuilder.Append("279=" + (object) marketDataUpdateAction + "|"); stringBuilder.Append("269=" + (object) marketDataEntryType + "|"); stringBuilder.Append("278=" + marketDataEntryID + "|"); stringBuilder.Append("55=" + (object) symbol + "|"); if (marketDataEntryPrice > Decimal.Zero) stringBuilder.Append("270=" + (object) marketDataEntryPrice + "|"); if (marketDataEntrySize > 0.0) stringBuilder.Append("271=" + (object) marketDataEntrySize + "|"); string str1 = this.ConstructHeader(qualifier, this.ApplicationMessageCode(MessageConstructor.ApplicationMessageType.MarketDataIncrementalRefresh), messageSequenceNumber, string.Empty); string str2 = this.ConstructTrailer(str1 + (object) stringBuilder); return (str1 + (object) stringBuilder + str2).Replace("|", "\x0001"); } public string NewOrderSingleMessage(MessageConstructor.SessionQualifier qualifier, int messageSequenceNumber, string orderID, long symbol, int side, string transactTime, int orderQuantity, int orderType, string timeInForce, Decimal price = 0M, Decimal stopPrice = 0M, string expireTime = "", string positionID = "") { StringBuilder stringBuilder = new StringBuilder(); stringBuilder.Append("11=" + orderID + "|"); stringBuilder.Append("55=" + (object) symbol + "|"); stringBuilder.Append("54=" + (object) side + "|"); stringBuilder.Append("60=" + transactTime + "|"); stringBuilder.Append("38=" + (object) orderQuantity + "|"); stringBuilder.Append("40=" + (object) orderType + "|"); if (price != Decimal.Zero) stringBuilder.Append("44=" + (object) price + "|"); if (stopPrice != Decimal.Zero) stringBuilder.Append("99=" + (object) stopPrice + "|"); stringBuilder.Append("59=" + timeInForce + "|"); if (expireTime != string.Empty) stringBuilder.Append("126=" + expireTime + "|"); if (positionID != string.Empty) stringBuilder.Append("721=" + positionID + "|"); string str1 = this.ConstructHeader(qualifier, this.ApplicationMessageCode(MessageConstructor.ApplicationMessageType.NewOrderSingle), messageSequenceNumber, stringBuilder.ToString()); string str2 = this.ConstructTrailer(str1 + (object) stringBuilder); return (str1 + (object) stringBuilder + str2).Replace("|", "\x0001"); } public string OrderStatusRequest(MessageConstructor.SessionQualifier qualifier, int messageSequenceNumber, string orderID) { StringBuilder stringBuilder = new StringBuilder(); stringBuilder.Append("11=" + orderID + "|"); stringBuilder.Append("54=1|"); string str1 = this.ConstructHeader(qualifier, this.ApplicationMessageCode(MessageConstructor.ApplicationMessageType.OrderStatusRequest), messageSequenceNumber, stringBuilder.ToString()); string str2 = this.ConstructTrailer(str1 + (object) stringBuilder); return (str1 + (object) stringBuilder + str2).Replace("|", "\x0001"); } public string ExecutionReport(MessageConstructor.SessionQualifier qualifier, int messageSequenceNumber, string cTraderOrderID, string orderStatus, string transactTime, long symbol = 0, int side = 1, int averagePrice = 0, int orderQuantity = 0, int leavesQuantity = 0, int cumQuantity = 0, string orderID = "", string orderType = "", int price = 0, int stopPrice = 0, string timeInForce = "", string expireTime = "", string text = "", int orderRejectionReason = -1, string positionID = "") { StringBuilder stringBuilder = new StringBuilder(); stringBuilder.Append("37=" + cTraderOrderID + "|"); stringBuilder.Append("11=" + orderID + "|"); stringBuilder.Append("150=F|"); stringBuilder.Append("39=" + orderStatus + "|"); stringBuilder.Append("55=" + (object) symbol + "|"); stringBuilder.Append("54=" + (object) side + "|"); stringBuilder.Append("60=" + transactTime + "|"); if (averagePrice > 0) stringBuilder.Append("6=" + (object) averagePrice + "|"); if (orderQuantity > 0) stringBuilder.Append("38=" + (object) orderQuantity + "|"); if (leavesQuantity > 0) stringBuilder.Append("151=" + (object) leavesQuantity + "|"); if (cumQuantity > 0) stringBuilder.Append("14=" + (object) cumQuantity + "|"); if (orderType != string.Empty) stringBuilder.Append("40=" + orderType + "|"); if (price > 0) stringBuilder.Append("44=" + (object) price + "|"); if (stopPrice > 0) stringBuilder.Append("99=" + (object) stopPrice + "|"); if (timeInForce != string.Empty) stringBuilder.Append("59=" + timeInForce + "|"); if (expireTime != string.Empty) stringBuilder.Append("126=" + expireTime + "|"); if (text != string.Empty) stringBuilder.Append("58=" + text + "|"); if (orderRejectionReason != -1) stringBuilder.Append("103=" + (object) orderRejectionReason + "|"); if (positionID != string.Empty) stringBuilder.Append("721=" + positionID + "|"); string str1 = this.ConstructHeader(qualifier, this.ApplicationMessageCode(MessageConstructor.ApplicationMessageType.OrderStatusRequest), messageSequenceNumber, stringBuilder.ToString()); string str2 = this.ConstructTrailer(str1 + (object) stringBuilder); return (str1 + (object) stringBuilder + str2).Replace("|", "\x0001"); } public string BusinessMessageReject(MessageConstructor.SessionQualifier qualifier, int messageSequenceNumber, int referenceSequenceNum = 0, string referenceMessageType = "", string businessRejectRefID = "", int businessRejectReason = -1, string text = "") { StringBuilder stringBuilder = new StringBuilder(); if ((uint) referenceSequenceNum > 0U) stringBuilder.Append("45=" + (object) referenceSequenceNum + "|"); if (referenceMessageType != string.Empty) stringBuilder.Append("372=" + referenceMessageType + "|"); if (businessRejectRefID != string.Empty) stringBuilder.Append("379=" + businessRejectRefID + "|"); if (businessRejectReason != -1) stringBuilder.Append("380=" + (object) businessRejectReason + "|"); if (text != string.Empty) stringBuilder.Append("58=" + text + "|"); string str1 = this.ConstructHeader(qualifier, this.ApplicationMessageCode(MessageConstructor.ApplicationMessageType.BusinessMessageReject), messageSequenceNumber, stringBuilder.ToString()); string str2 = this.ConstructTrailer(str1 + (object) stringBuilder); return (str1 + (object) stringBuilder + str2).Replace("|", "\x0001"); } public string RequestForPositions(MessageConstructor.SessionQualifier qualifier, int messageSequenceNumber, string requestID, string positionRequestID = "") { StringBuilder stringBuilder = new StringBuilder(); stringBuilder.Append("710=" + requestID + "|"); if (positionRequestID != string.Empty) stringBuilder.Append("721=" + positionRequestID + "|"); string str1 = this.ConstructHeader(qualifier, this.ApplicationMessageCode(MessageConstructor.ApplicationMessageType.RequestForPosition), messageSequenceNumber, stringBuilder.ToString()); string str2 = this.ConstructTrailer(str1 + (object) stringBuilder); return (str1 + (object) stringBuilder + str2).Replace("|", "\x0001"); } public string PositionReport(MessageConstructor.SessionQualifier qualifier, int messageSequenceNumber, string requestID, string totalNumberOfPositionReports, string positionRequestResult, string positionID = "", string symbol = "", string noOfPositions = "", string longQuantity = "", string shortQuantity = "", string settlementPrice = "") { StringBuilder stringBuilder = new StringBuilder(); stringBuilder.Append("710=" + requestID + "|"); if (positionID != string.Empty) stringBuilder.Append("721=" + positionID + "|"); stringBuilder.Append("727=" + totalNumberOfPositionReports + "|"); stringBuilder.Append("728=" + positionRequestResult + "|"); if (symbol != string.Empty) stringBuilder.Append("55=" + symbol + "|"); if (noOfPositions != string.Empty) stringBuilder.Append("702=" + noOfPositions + "|"); if (longQuantity != string.Empty) stringBuilder.Append("704=" + requestID + "|"); if (shortQuantity != string.Empty) stringBuilder.Append("705=" + shortQuantity + "|"); if (settlementPrice != string.Empty) stringBuilder.Append("730=" + settlementPrice + "|"); string str1 = this.ConstructHeader(qualifier, this.ApplicationMessageCode(MessageConstructor.ApplicationMessageType.PositionReport), messageSequenceNumber, string.Empty); string str2 = this.ConstructTrailer(str1 + (object) stringBuilder); return (str1 + (object) stringBuilder + str2).Replace("|", "\x0001"); } private string ConstructHeader(MessageConstructor.SessionQualifier qualifier, string type, int messageSequenceNumber, string bodyMessage) { StringBuilder stringBuilder1 = new StringBuilder(); stringBuilder1.Append("8=FIX.4.4|"); StringBuilder stringBuilder2 = new StringBuilder(); stringBuilder2.Append("35=" + type + "|"); stringBuilder2.Append("49=" + this._senderCompID + "|"); stringBuilder2.Append("56=" + this._targetCompID + "|"); stringBuilder2.Append("57=" + qualifier.ToString() + "|"); stringBuilder2.Append("50=" + this._senderSubID + "|"); stringBuilder2.Append("34=" + (object) messageSequenceNumber + "|"); stringBuilder2.Append("52=" + DateTime.UtcNow.ToString("yyyyMMdd-HH:mm:ss") + "|"); int num = stringBuilder2.Length + bodyMessage.Length; stringBuilder1.Append("9=" + (object) num + "|"); stringBuilder1.Append((object) stringBuilder2); return stringBuilder1.Replace("|", "\x0001").ToString(); } private string ConstructTrailer(string message) { return ("10=" + (object) this.CalculateChecksum(message.Replace("|", "\x0001").ToString()) + "|").Replace("|", "\x0001"); } private int CalculateChecksum(string dataToCalculate) { byte[] bytes = Encoding.ASCII.GetBytes(dataToCalculate); int num1 = 0; foreach (byte num2 in bytes) num1 += (int) num2; return num1 % 256; } private string SessionMessageCode(MessageConstructor.SessionMessageType type) { switch (type) { case MessageConstructor.SessionMessageType.Logon: return "A"; case MessageConstructor.SessionMessageType.Logout: return "5"; case MessageConstructor.SessionMessageType.Heartbeat: return "0"; case MessageConstructor.SessionMessageType.TestRequest: return "1"; case MessageConstructor.SessionMessageType.Resend: return "2"; case MessageConstructor.SessionMessageType.Reject: return "3"; case MessageConstructor.SessionMessageType.SequenceReset: return "4"; default: return "0"; } } private string ApplicationMessageCode(MessageConstructor.ApplicationMessageType type) { switch (type) { case MessageConstructor.ApplicationMessageType.MarketDataRequest: return "V"; case MessageConstructor.ApplicationMessageType.MarketDataIncrementalRefresh: return "X"; case MessageConstructor.ApplicationMessageType.NewOrderSingle: return "D"; case MessageConstructor.ApplicationMessageType.OrderStatusRequest: return "H"; case MessageConstructor.ApplicationMessageType.ExecutionReport: return "8"; case MessageConstructor.ApplicationMessageType.BusinessMessageReject: return "j"; case MessageConstructor.ApplicationMessageType.RequestForPosition: return "AN"; case MessageConstructor.ApplicationMessageType.PositionReport: return "AP"; default: return "0"; } } public enum SessionMessageType { Logon, Logout, Heartbeat, TestRequest, Resend, Reject, SequenceReset, } public enum ApplicationMessageType { MarketDataRequest, MarketDataIncrementalRefresh, NewOrderSingle, OrderStatusRequest, ExecutionReport, BusinessMessageReject, RequestForPosition, PositionReport, } public enum SessionQualifier { QUOTE, TRADE, } } }
@mindbreaker
mindbreaker
08 Feb 2017, 17:13
Fix api socket
using FIX_API_Library; using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Net.Sockets; using System.Text; using System.Threading; using System.Threading.Tasks; using System.Windows.Forms; namespace FIX_API_Sample { public partial class frmFIXAPISample : Form { private int _pricePort = 5201; private int _tradePort = 5202; private string _host = "h20.p.ctrader.com"; private string _username = "1234567"; private string _password = "0000000"; private string _senderCompID = "fxpro.1234567"; private string _senderSubID = "TRADE"; private string _targetCompID = "cSERVER"; private int _messageSequenceNumber = 1; private int _testRequestID = 1; TcpClient _priceClient; NetworkStream _priceStream; TcpClient _tradeClient; NetworkStream _tradeStream; MessageConstructor _messageConstructor; public frmFIXAPISample() { InitializeComponent(); _priceClient = new TcpClient(_host, _pricePort); _priceStream = _priceClient.GetStream(); _tradeClient = new TcpClient(_host, _tradePort); _tradeStream = _priceClient.GetStream(); _messageConstructor = new MessageConstructor(_host, _username, _password, _senderCompID, _senderSubID, _targetCompID); } private void ClearText() { txtMessageSend.Text = ""; txtMessageReceived.Text = ""; } private string SendPriceMessage(string message) { return SendMessage(message, _priceStream); } private string SendTradeMessage(string message) { return SendMessage(message, _tradeStream); } private string SendMessage(string message, NetworkStream stream) { var byteArray = Encoding.ASCII.GetBytes(message); stream.Write(byteArray, 0, byteArray.Length); var buffer = new byte[1024]; int i = 0; while (!stream.DataAvailable && i < 10) { Thread.Sleep(100); i++; } if(stream.DataAvailable) stream.Read(buffer, 0, 1024); _messageSequenceNumber++; var returnMessage = Encoding.ASCII.GetString(buffer); return returnMessage; } private void btnLogon_Click(object sender, EventArgs e) { ClearText(); var message = _messageConstructor.LogonMessage(MessageConstructor.SessionQualifier.QUOTE, _messageSequenceNumber, 30, false); txtMessageSend.Text = message; txtMessageReceived.Text = SendPriceMessage(message); } private void btnTestRequest_Click(object sender, EventArgs e) { ClearText(); var message = _messageConstructor.TestRequestMessage(MessageConstructor.SessionQualifier.QUOTE, _messageSequenceNumber,_testRequestID); _testRequestID++; txtMessageSend.Text = message; txtMessageReceived.Text = SendPriceMessage(message); } private void btnLogout_Click(object sender, EventArgs e) { ClearText(); var message = _messageConstructor.LogoutMessage(MessageConstructor.SessionQualifier.QUOTE, _messageSequenceNumber); txtMessageSend.Text = message; txtMessageReceived.Text = SendPriceMessage(message); _messageSequenceNumber = 1; } private void btnMarketDataRequest_Click(object sender, EventArgs e) { ClearText(); var message = _messageConstructor.MarketDataRequestMessage(MessageConstructor.SessionQualifier.QUOTE, _messageSequenceNumber, "EURUSD:WDqsoT", 1,0,0,1,1); txtMessageSend.Text = message; txtMessageReceived.Text = SendPriceMessage(message); } private void btnHeartbeat_Click(object sender, EventArgs e) { ClearText(); var message = _messageConstructor.HeartbeatMessage(MessageConstructor.SessionQualifier.QUOTE, _messageSequenceNumber); txtMessageSend.Text = message; txtMessageReceived.Text = SendPriceMessage(message); } private void btnResendRequest_Click(object sender, EventArgs e) { ClearText(); var message = _messageConstructor.ResendMessage(MessageConstructor.SessionQualifier.QUOTE, _messageSequenceNumber,_messageSequenceNumber - 1); _testRequestID++; txtMessageSend.Text = message; txtMessageReceived.Text = SendPriceMessage(message); } private void btnReject_Click(object sender, EventArgs e) { ClearText(); var message = _messageConstructor.RejectMessage(MessageConstructor.SessionQualifier.QUOTE, _messageSequenceNumber,0); _testRequestID++; txtMessageSend.Text = message; txtMessageReceived.Text = SendPriceMessage(message); } private void btnSequenceReset_Click(object sender, EventArgs e) { ClearText(); var message = _messageConstructor.SequenceResetMessage(MessageConstructor.SessionQualifier.QUOTE, _messageSequenceNumber,0); _testRequestID++; txtMessageSend.Text = message; txtMessageReceived.Text = SendPriceMessage(message); } private void btnNewOrderSingle_Click(object sender, EventArgs e) { ClearText(); var message = _messageConstructor.NewOrderSingleMessage(MessageConstructor.SessionQualifier.TRADE, _messageSequenceNumber,"1408471",1,1, DateTime.UtcNow.ToString("yyyyMMdd-HH:mm:ss"), 1000,1, "3"); _testRequestID++; txtMessageSend.Text = message; txtMessageReceived.Text = SendTradeMessage(message); } private void btnOrderStatusRequest_Click(object sender, EventArgs e) { ClearText(); var message = _messageConstructor.OrderStatusRequest(MessageConstructor.SessionQualifier.TRADE, _messageSequenceNumber, "1408471"); _testRequestID++; txtMessageSend.Text = message; txtMessageReceived.Text = SendTradeMessage(message); } private string Timestamp() { return (DateTime.UtcNow.Subtract(new DateTime(1970, 1, 1))).TotalSeconds.ToString(); } private void btnRequestForPositions_Click(object sender, EventArgs e) { ClearText(); var message = _messageConstructor.RequestForPositions(MessageConstructor.SessionQualifier.TRADE, _messageSequenceNumber, "876316401"); _testRequestID++; txtMessageSend.Text = message; txtMessageReceived.Text = SendTradeMessage(message); } private void btnLogonT_Click(object sender, EventArgs e) { ClearText(); var message = _messageConstructor.LogonMessage(MessageConstructor.SessionQualifier.TRADE, _messageSequenceNumber, 30, false); txtMessageSend.Text = message; txtMessageReceived.Text = SendTradeMessage(message); } private void btnHeartbeatT_Click(object sender, EventArgs e) { ClearText(); var message = _messageConstructor.HeartbeatMessage(MessageConstructor.SessionQualifier.TRADE, _messageSequenceNumber); txtMessageSend.Text = message; txtMessageReceived.Text = SendTradeMessage(message); } private void btnTestRequestT_Click(object sender, EventArgs e) { ClearText(); var message = _messageConstructor.TestRequestMessage(MessageConstructor.SessionQualifier.TRADE, _messageSequenceNumber, _testRequestID); _testRequestID++; txtMessageSend.Text = message; txtMessageReceived.Text = SendTradeMessage(message); } private void btnLogoutT_Click(object sender, EventArgs e) { ClearText(); var message = _messageConstructor.LogoutMessage(MessageConstructor.SessionQualifier.TRADE, _messageSequenceNumber); txtMessageSend.Text = message; txtMessageReceived.Text = SendTradeMessage(message); _messageSequenceNumber = 1; } private void btnResendRequestT_Click(object sender, EventArgs e) { ClearText(); var message = _messageConstructor.ResendMessage(MessageConstructor.SessionQualifier.TRADE, _messageSequenceNumber, _messageSequenceNumber - 1); _testRequestID++; txtMessageSend.Text = message; txtMessageReceived.Text = SendTradeMessage(message); } private void btnSpotMarketData_Click(object sender, EventArgs e) { ClearText(); var message = _messageConstructor.MarketDataRequestMessage(MessageConstructor.SessionQualifier.QUOTE, _messageSequenceNumber, "EURUSD:WDqsoT", 1, 1, 0, 1, 1); txtMessageSend.Text = message; txtMessageReceived.Text = SendPriceMessage(message); } private void btnStopOrder_Click(object sender, EventArgs e) { ClearText(); var message = _messageConstructor.NewOrderSingleMessage(MessageConstructor.SessionQualifier.TRADE, _messageSequenceNumber, "10", 1, 1, DateTime.UtcNow.ToString("yyyyMMdd-HH:mm:ss"), 1000, 3, "3",0, (decimal)1.08); _testRequestID++; txtMessageSend.Text = message; txtMessageReceived.Text = SendTradeMessage(message); } private void btnLimitOrder_Click(object sender, EventArgs e) { ClearText(); var message = _messageConstructor.NewOrderSingleMessage(MessageConstructor.SessionQualifier.TRADE, _messageSequenceNumber, "10", 1, 1, DateTime.UtcNow.ToString("yyyyMMdd-HH:mm:ss"), 1000,2, "3", (decimal)1.08); _testRequestID++; txtMessageSend.Text = message; txtMessageReceived.Text = SendTradeMessage(message); } } }
@mindbreaker
taskman9
26 Mar 2017, 19:30
RE: RE: RE: where is error n this example
mindbreaker said:
Thanks
Hi, can any one help?
I tried codes from post #17 & got this exception:
System.Net.Sockets.SocketException (0x80004005): No such host is known at System.Net.Dns.InternalGetHostByAddress(IPAddress address, Boolean include IPv6) at System.Net.Dns.GetHostEntry(String hostNameOrAddress) at FixNoSSL.FixSocketClient.StartClient(String host) in c:\users\workstation\ documents\visual studio 2015\Projects\test01\test01\Program.cs:line 65
the code:
using System; using System.Collections.Generic; using System.Linq; using System.Net; using System.Net.Sockets; using System.Text; using System.Threading; using System.Threading.Tasks; namespace FixNoSSL { public class FixSocketClient { // cserver ip fix public static string host = "78.129.190.32"; // fix server id public static string FixID = "pepperstone.3190557"; // ctrader account public static string Account = "3190557"; // ctrader password public static string Password = "2276"; public static int total; public static string m = ""; public static void StartClient(string host) { string time = DateTime.UtcNow.ToString("yyyyMMdd-HH:mm:ss.fff"); string body = "35=A|34=1|49=" + FixID + "|50=QUOTE|52=" + time + "|56=CSERVER|98=0|108=30|553=" + Account + "|554=" + Password + "|"; // body length int bodylen = body.Length; string fix = "8=FIX.4.4|9=" + bodylen + "|"; // message string message = fix + body; message = message.Replace('|', '\u0001'); byte[] messageBytes = Encoding.ASCII.GetBytes(message);//converted to 0's &1s for (int i = 0; i < message.Length; i++) total += messageBytes[i]; int checksum = total % 256; string checksumStr = checksum.ToString().PadLeft(3, '0'); string sum = "10=" + checksumStr + "|"; string m = fix + body + sum; m = m.Replace('|', '\u0001'); Console.WriteLine("Send to server (hit any key) {0}", m); Console.ReadKey(); // Data buffer for incoming data. byte[] bytes = new byte[1024]; // Connect to a remote device. try { // Establish the remote endpoint for the socket. // This example uses port 11000 on the local computer. IPHostEntry ipHostInfo = Dns.GetHostEntry(host); IPAddress ipAddress = ipHostInfo.AddressList[0]; IPEndPoint remoteEP = new IPEndPoint(ipAddress, 5201); // Create a TCP/IP socket. Socket sender = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp); // Connect the socket to the remote endpoint. Catch any errors. try { sender.Connect(remoteEP); Console.WriteLine("SOCKET CONECTED {0}", sender.RemoteEndPoint.ToString()); // Encode the data string into a byte array. byte[] msg = Encoding.ASCII.GetBytes(m); // Send the data through the socket. int bytesSent = sender.Send(msg); // Receive the response from the remote device. int bytesRec = sender.Receive(bytes); Console.WriteLine("SERVER SEND >> {0}", Encoding.ASCII.GetString(bytes, 0, bytesRec)); // Release the socket. sender.Shutdown(SocketShutdown.Both); sender.Close(); } catch (ArgumentNullException ane) { Console.WriteLine("ArgumentNullException : {0}", ane.ToString()); } catch (SocketException se) { Console.WriteLine("SocketException : {0}", se.ToString()); } catch (Exception e) { Console.WriteLine("Unexpected exception : {0}", e.ToString()); } } catch (Exception e) { Console.WriteLine(e.ToString()); } } public static int Main(String[] args) { while (true) { StartClient(host); Thread.Sleep(5000); } } } }
@taskman9
ianj
01 Sep 2016, 19:40
Try without SSL - i am using quickfixJ without SSL and it "works" fine (with caveats)
@ianj