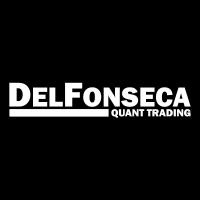
Telegram notifications with AccessRights.None
02 Jun 2023, 00:41
Hi all,
I have already developed several tests to use telegram notifications using AccessRights.None.
This is the simplest code using HttpClient that I developed. It works if it is AccessRights.FullAccess, if it is AccessRights.None it gives the error described below.
Can anyone assist me in this regard or is it a cAlgo bug? Thanks in advance!!
TEST: EXCEPTION: One or more errors occurred. (The type initializer for 'System.Net.CookieContainer' threw an exception.)
in System.Threading.Tasks.Task.ThrowIfExceptional(Boolean includeTaskCanceledExceptions)
in System.Threading.Tasks.Task`1.GetResultCore(Boolean waitCompletionNotification)
in System.Threading.Tasks.Task`1.get_Result()
in cAlgo.AllInOneDataByDelFonseca.SendTelegram(String msg)
using System;
using cAlgo.API;
using System.Net.Http;
namespace cAlgo
{
[Indicator(IsOverlay = true, AccessRights = AccessRights.None)]
public class TelegramSample : Indicator
{
[Parameter("Token", DefaultValue = "")]
public string TOKEN { get; set; }
[Parameter("ChatId", DefaultValue = "")]
public string CHATID { get; set; }
private HttpClient httpClient;
protected override void Initialize()
{
httpClient = new HttpClient();
}
public void SendTelegram(string msg)
{
try
{
var url = $"https://api.telegram.org/bot{TOKEN}/sendMessage?chat_id={CHATID}&text={msg}";
var response = httpClient.GetAsync(url).Result;
if (!response.IsSuccessStatusCode)
{
msg += $": Error Sending Telegram Message: {response.StatusCode}, {response.Content.ReadAsStringAsync().Result}";
}
else
{
msg += ": Success";
}
}
catch (Exception e)
{
msg += ": EXCEPTION : " + e.Message + "\n\n" + e.StackTrace;
}
Print(msg);
}
public override void Calculate(int index)
{
SendTelegram("Hello World.");
}
}
}
Replies
DelFonseca
02 Jun 2023, 22:56
RE:
firemyst said:
(...)
The example in the video does not work as it requires AccessRights.FullAccess.
According to the documentation, the example I shared should work with AccessRights.None, but it gives the error that is in my thread.
I don't know if I'm missing something because I've tried several methods without success to make it work with AccessRights.None
@DelFonseca
DelFonseca
03 Jun 2023, 19:45
[SOLVED]
Problem solved. My cTrader was not up to date, follow the code below with HttpClient replaced by cAlgo.API.Http.
Thanks to "The T"
using System;
using cAlgo.API;
namespace cAlgo
{
[Indicator(IsOverlay = true, AccessRights = AccessRights.None)]
public class TelegramSample : Indicator
{
[Parameter("Token", DefaultValue = "")]
public string TOKEN { get; set; }
[Parameter("ChatId", DefaultValue = "")]
public string CHATID { get; set; }
protected override void Initialize()
{
}
public void SendTelegram(string msg)
{
try
{
var url = $"https://api.telegram.org/bot{TOKEN}/sendMessage?chat_id={CHATID}&text={msg}";
var response = Http.Get(url);//httpClient.GetAsync(url).Result;
if (!response.IsSuccessful)
{
msg += $": Error Sending Telegram Message: {response.StatusCode}, {response.Body}";
}
else
{
msg += ": Success";
}
}
catch (Exception e)
{
msg += ": EXCEPTION : " + e.Message + "\n\n" + e.StackTrace;
}
Print(msg);
}
public override void Calculate(int index)
{
SendTelegram("Hello World.");
}
}
}
@DelFonseca
firemyst
02 Jun 2023, 03:52
Examples you can look at to see irf they help?
And also watching this video:
@firemyst