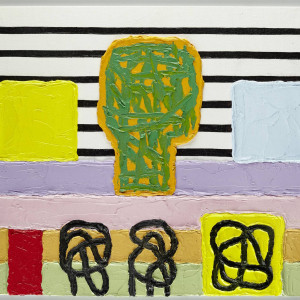
Optimization Error (Again)
28 Aug 2021, 01:35
Hi all.
This post is related to cTDN Forum - Historical data corrupted? (ctrader.com) Unfortunately I still have the same problem. The skeleton of my bot is shown bellow and still has a strange behaviour: (for EURJPY H1, from 1/1/2018 to current date) with the first set of parameters the optimization process works fine, and with the second set of parameters the process does not show any result and the time runs with no end, like that:
Parameters OK:
Parameters NO OK:
The skeleton of my bot is shown below. I've deleted a lot of lines trying to find the bug but it still works wrong:
//------------------------------------------------------------------------------------------------------------------------------------------------------------------
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
using System.IO;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)]
public class TAGLAXerr : Robot
{
[Parameter("Bot Name", Group = "Configuration", DefaultValue = "TAGLAX")]
public String BotName { get; set; }
[Parameter("Label Suffix", Group = "Configuration", DefaultValue = DEFAULT_LABEL)]
public String Label { get; set; }
[Parameter("Config File", Group = "Configuration", DefaultValue = DEFAULT_CONFIGFILENAME)]
public String ConfigFile { get; set; }
[Parameter("Stop Loss VUD", Group = "Finance", DefaultValue = 2.8)]
public double StopLossFactor { get; set; }
[Parameter("Profit/Risk Factor", Group = "Finance", DefaultValue = 1.0)]
public double ProfitRiskFactor { get; set; }
[Parameter("Max Spread", Group = "Finance", DefaultValue = 1.0)]
public double MaxSpread { get; set; }
//------------------Params. Indicadores-----------------------
[Parameter("Ax", Group = "Ind", DefaultValue = 0.6, MinValue = 0)]
public double Ax { get; set; }
[Parameter("Bx", Group = "Ind", DefaultValue = 0.2, MinValue = 0)]
public double Bx { get; set; }
[Parameter("Cx", Group = "Ind", DefaultValue = 1.0, MinValue = 0)]
public double Cx { get; set; }
[Parameter("Dx", Group = "Ind21", DefaultValue = 10, MinValue = 0)]
public double Dx { get; set; }
[Parameter("Ex", Group = "Ind21", DefaultValue = 0.1, MinValue = 0)]
public double Ex { get; set; }
//--------------------Time Indicadores------------------------
[Parameter("Start Hour", Group = "Time", DefaultValue = 0, MinValue = 0, MaxValue = 24)]
public int StartHour { get; set; }
[Parameter("End Hour", Group = "Time", DefaultValue = 24, MinValue = 0, MaxValue = 24)]
public int EndHour { get; set; }
private const string PATH = "c:\\Trading\\";
private const string DEFAULT_LABEL = "Default";
private const string DEFAULT_CONFIGFILENAME = "config";
private const int VUD_PERIOD = 50;
private string label;
private string configFile = "";
private int initialIndex = -1;
private AverageTrueRange atr;
private SimpleMovingAverage smaATR;
protected override void OnStart()
{
try
{
if (StopLossFactor == 0)
closeBot();
atr = Indicators.AverageTrueRange(14, MovingAverageType.Exponential);
smaATR = Indicators.SimpleMovingAverage(atr.Result, VUD_PERIOD);
initialize();
} catch (Exception e)
{
log(e);
}
}
//se ejecuta cada inicio de vela
protected override void OnBar()
{
}
protected override void OnStop()
{
// Put your deinitialization logic here
}
//==========================================================================
// UTILIDADES
//==========================================================================
private void initialize()
{
try
{
if ((Label == null) || (string.Compare(Label, "Default") == 0) || (Label.Length == 0))
{
label = BotName + Symbol.Name + TimeFrame.ToString();
}
else
label = Label;
configFile = PATH + ConfigFile + ".txt";
initialIndex = 2;
} catch (Exception e)
{
throw new TradeException(TradeException.GENERIC_ERROR, "Error al inicializar variables", e);
}
}
private void log(TradeException ex)
{
string message = "";
if (ex.InnerException != null)
message = ex.Message + ". " + ex.InnerException.Message;
else
message = ex.Message;
using (StreamWriter w = File.AppendText(PATH + "log.txt"))
{
w.Write("\r\n" + BotName + ";" + Label + ";" + Symbol.Name + ";" + TimeFrame.ToString() + ";" + DateTime.Now.ToLongDateString() + ";" + DateTime.Now.ToLongTimeString() + ";" + ex.id + ";" + message);
}
}
private void log(Exception ex)
{
string message = "";
if (ex.InnerException != null)
message = ex.Message + ". " + ex.InnerException.Message;
else
message = ex.Message;
using (StreamWriter w = File.AppendText(PATH + "log.txt"))
{
w.Write("\r\n" + BotName + ";" + Label + ";" + Symbol.Name + ";" + TimeFrame.ToString() + ";" + DateTime.Now.ToLongDateString() + ";" + DateTime.Now.ToLongTimeString() + ";" + "" + ";" + message);
}
}
private void handleError(int id)
{
switch (id)
{
case TradeException.CLOSE_POSITION_ERROR:
case TradeException.CONVERSION_ERROR:
case TradeException.DATETIME_BAR_ERROR:
case TradeException.READ_CONFIGFILE_ERROR:
case TradeException.POSITION_ACCESS_ERROR:
case TradeException.GENERIC_ERROR:
case TradeException.INDICATOR_ERROR:
//nada
break;
case TradeException.EXECUTE_ORDER_ERROR:
break;
case TradeException.POSITION_NOSTOPLOSS_ERROR:
break;
}
}
private void closeBot()
{
Stop();
}
private bool isOperationAllowed()
{
bool ret = false;
try
{
int h = Bars.OpenTimes.LastValue.Hour;
if (isNumber(h))
{
if ((h >= StartHour) && (h <= EndHour))
ret = true;
}
} catch (Exception e)
{
throw new TradeException(TradeException.DATETIME_BAR_ERROR, "Error en la lectura de fecha y hora de las barras", e);
}
return ret;
}
private double getIncrementDESC(DataSeries Source, int index)
{
double ret = double.NaN;
if (index >= initialIndex)
{
ret = Source[index - 1] - Source[index];
}
return ret;
}
private double getIncrementASC(DataSeries Source, int index)
{
double ret = double.NaN;
if (index >= initialIndex)
{
ret = Source[index] - Source[index - 1];
}
return ret;
}
private double DIVZ(double dividend, double divisor)
{
if ((divisor == 0) || (double.IsNaN(divisor)))
return double.NaN;
else
return dividend / divisor;
}
private double getPIPs(double v0, double v1)
{
return DIVZ(v0 - v1, Symbol.PipSize);
}
private double getVUD(int index)
{
return getPIPs(smaATR.Result[index], 0);
}
private double NZ(double val)
{
return double.IsNaN(val) ? 0 : val;
}
private double NZ(double val, double ret)
{
return double.IsNaN(val) ? ret : val;
}
private bool isNumber(double val)
{
return !double.IsNaN(val);
}
private bool isNaN(double val)
{
return double.IsNaN(val);
}
//==========================================================================
// INDICADORES
//==========================================================================
private bool openBuyOperation(int index)
{
bool ret = false;
try
{
} catch (Exception e)
{
throw new TradeException(TradeException.INDICATOR_ERROR, "Error consulta indicador para compra", e);
}
return ret;
}
private bool openSellOperation(int index)
{
bool ret = false;
try
{
} catch (Exception e)
{
throw new TradeException(TradeException.INDICATOR_ERROR, "Error consulta indicador para venta", e);
}
return ret;
}
private bool isClosePositionGranted(Position position, TradeType type, int index)
{
bool ret = false;
try
{
if (type == TradeType.Buy)
{
ret = false;
}
else if (type == TradeType.Sell)
{
ret = false;
}
} catch (Exception e)
{
throw new TradeException(TradeException.INDICATOR_ERROR, "Error consulta indicador para cierre de posicion", e);
}
return ret;
}
}
}
public class TradeException : Exception
{
public const int CLOSE_POSITION_ERROR = 0;
public const int EXECUTE_ORDER_ERROR = 1;
public const int READ_CONFIGFILE_ERROR = 2;
public const int POSITION_NOSTOPLOSS_ERROR = 3;
public const int SPREAD_ERROR = 4;
public const int GET_VUD_ERROR = 5;
public const int TAKEPROFIT_ERROR = 6;
public const int STOPLOSS_ERROR = 7;
public const int INDICATOR_ERROR = 8;
public const int BOT_INITIALIZATION_ERROR = 9;
public const int GENERIC_ERROR = 10;
public const int DATETIME_BAR_ERROR = 11;
public const int CONVERSION_ERROR = 12;
public const int POSITION_ACCESS_ERROR = 14;
public const int RISK_ERROR = 15;
public int id;
public TradeException()
{
}
public TradeException(string message) : base(message)
{
}
/*public TradeException(int id)
{
this.id = id;
}*/
public TradeException(int id, string message) : base(message)
{
this.id = id;
}
public TradeException(string message, Exception inner) : base(message, inner)
{
}
public TradeException(int id, string message, Exception inner) : base(message, inner)
{
this.id = id;
}
}
//------------------------------------------------------------------------------------------------------------------------------------------------------------------
Thanks in advance.
Replies
Abstract
29 Aug 2021, 03:03
RE: RE:
The last version is still with the same error. If Dx Step is 5 works fine. It DX Step is 10 works wrong:
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)]
public class TAGLAXerrr : Robot
{
[Parameter("Bot Name", Group = "Configuration", DefaultValue = "TAGLAX")]
public String BotName { get; set; }
[Parameter("Label Suffix", Group = "Configuration", DefaultValue = DEFAULT_LABEL)]
public String Label { get; set; }
[Parameter("Config File", Group = "Configuration", DefaultValue = DEFAULT_CONFIGFILENAME)]
public String ConfigFile { get; set; }
[Parameter("Stop Loss VUD", Group = "Finance", DefaultValue = 2.8)]
public double StopLossFactor { get; set; }
[Parameter("Profit/Risk Factor", Group = "Finance", DefaultValue = 1.0)]
public double ProfitRiskFactor { get; set; }
[Parameter("Max Spread", Group = "Finance", DefaultValue = 1.0)]
public double MaxSpread { get; set; }
//------------------Params. Indicadores-----------------------
[Parameter("Ax", Group = "Ind", DefaultValue = 0.6, MinValue = 0)]
public double Ax { get; set; }
[Parameter("Bx", Group = "Ind", DefaultValue = 0.2, MinValue = 0)]
public double Bx { get; set; }
[Parameter("Cx", Group = "Ind", DefaultValue = 1.0, MinValue = 0)]
public double Cx { get; set; }
[Parameter("Dx", Group = "Ind21", DefaultValue = 10, MinValue = 0)]
public double Dx { get; set; }
[Parameter("Ex", Group = "Ind21", DefaultValue = 0.1, MinValue = 0)]
public double Ex { get; set; }
//--------------------Time Indicadores------------------------
[Parameter("Start Hour", Group = "Time", DefaultValue = 0, MinValue = 0, MaxValue = 24)]
public int StartHour { get; set; }
[Parameter("End Hour", Group = "Time", DefaultValue = 24, MinValue = 0, MaxValue = 24)]
public int EndHour { get; set; }
private const string DEFAULT_LABEL = "Default";
private const string DEFAULT_CONFIGFILENAME = "config";
private const int VUD_PERIOD = 50;
private AverageTrueRange atr;
private SimpleMovingAverage smaATR;
protected override void OnStart()
{
atr = Indicators.AverageTrueRange(14, MovingAverageType.Exponential);
smaATR = Indicators.SimpleMovingAverage(atr.Result, VUD_PERIOD);
}
//se ejecuta cada inicio de vela
protected override void OnBar()
{
}
}
}
@Abstract
Abstract
31 Aug 2021, 00:34
( Updated at: 21 Dec 2023, 09:22 )
RE: RE: RE:
Hello,
I've had the following error message trying to find the bug:
The last version of my robot is "naked" -shown below- but still with the same problem: an error is thrown for high steps in parameters. Maybe my Ctrader is corrupted... I would appreciate an answer to resolve this problem. Thank you.
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)]
public class TAGLAXerrr : Robot
{
[Parameter("Dx", Group = "Ind21", DefaultValue = 10, MinValue = 0)]
public double Dx { get; set; }
protected override void OnStart()
{
}
//se ejecuta cada inicio de vela
protected override void OnBar()
{
}
}
}
@Abstract
PanagiotisCharalampous
31 Aug 2021, 09:10
Hi Abstract,
We managed to reproduce the problem and our team looking at it.
Best Regards,
Panagiotis
Join us on Telegram and Facebook
@PanagiotisCharalampous
Abstract
28 Aug 2021, 15:41 ( Updated at: 29 Aug 2021, 03:06 )
RE:
I've deleted many lines in order to find the bug, even I've replaced my class TradeException -using only the class Exception- but nothing changes. I've found the solution but it's so strange: when the value of the step in the parameter Dx is 5 then the bot works fine. I cannot understand this behaviour, maybe my Ctrader is corrupted and I need to install it again...
I show the last version of my bot -with the same problem-
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
using System.IO;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)]
public class TAGLAXerrr : Robot
{
[Parameter("Bot Name", Group = "Configuration", DefaultValue = "TAGLAX")]
public String BotName { get; set; }
[Parameter("Label Suffix", Group = "Configuration", DefaultValue = DEFAULT_LABEL)]
public String Label { get; set; }
[Parameter("Config File", Group = "Configuration", DefaultValue = DEFAULT_CONFIGFILENAME)]
public String ConfigFile { get; set; }
[Parameter("Stop Loss VUD", Group = "Finance", DefaultValue = 2.8)]
public double StopLossFactor { get; set; }
[Parameter("Profit/Risk Factor", Group = "Finance", DefaultValue = 1.0)]
public double ProfitRiskFactor { get; set; }
[Parameter("Max Spread", Group = "Finance", DefaultValue = 1.0)]
public double MaxSpread { get; set; }
//------------------Params. Indicadores-----------------------
[Parameter("Ax", Group = "Ind", DefaultValue = 0.6, MinValue = 0)]
public double Ax { get; set; }
[Parameter("Bx", Group = "Ind", DefaultValue = 0.2, MinValue = 0)]
public double Bx { get; set; }
[Parameter("Cx", Group = "Ind", DefaultValue = 1.0, MinValue = 0)]
public double Cx { get; set; }
[Parameter("Dx", Group = "Ind21", DefaultValue = 10, MinValue = 0)]
public double Dx { get; set; }
[Parameter("Ex", Group = "Ind21", DefaultValue = 0.1, MinValue = 0)]
public double Ex { get; set; }
//--------------------Time Indicadores------------------------
[Parameter("Start Hour", Group = "Time", DefaultValue = 0, MinValue = 0, MaxValue = 24)]
public int StartHour { get; set; }
[Parameter("End Hour", Group = "Time", DefaultValue = 24, MinValue = 0, MaxValue = 24)]
public int EndHour { get; set; }
private const string PATH = "c:\\Trading\\";
private const string DEFAULT_LABEL = "Default";
private const string DEFAULT_CONFIGFILENAME = "config";
private const int VUD_PERIOD = 50;
private string label;
private string configFile = "";
private AverageTrueRange atr;
private SimpleMovingAverage smaATR;
protected override void OnStart()
{
try
{
if (StopLossFactor == 0)
closeBot();
atr = Indicators.AverageTrueRange(14, MovingAverageType.Exponential);
smaATR = Indicators.SimpleMovingAverage(atr.Result, VUD_PERIOD);
initialize();
} catch (Exception e)
{
log(e);
}
}
//se ejecuta cada inicio de vela
protected override void OnBar()
{
}
protected override void OnStop()
{
// Put your deinitialization logic here
}
//==========================================================================
// UTILIDADES
//==========================================================================
private void initialize()
{
try
{
if ((Label == null) || (string.Compare(Label, "Default") == 0) || (Label.Length == 0))
{
label = BotName + Symbol.Name + TimeFrame.ToString();
}
else
label = Label;
configFile = PATH + ConfigFile + ".txt";
} catch (Exception e)
{
throw new Exception("Error al inicializar variables", e);
}
}
private void log(Exception ex)
{
string message = "";
if (ex.InnerException != null)
message = ex.Message + ". " + ex.InnerException.Message;
else
message = ex.Message;
using (StreamWriter w = File.AppendText(PATH + "log.txt"))
{
w.Write("\r\n" + BotName + ";" + Label + ";" + Symbol.Name + ";" + TimeFrame.ToString() + ";" + DateTime.Now.ToLongDateString() + ";" + DateTime.Now.ToLongTimeString() + ";" + "" + ";" + message);
}
}
private void closeBot()
{
Stop();
}
private bool isOperationAllowed()
{
bool ret = false;
try
{
int h = Bars.OpenTimes.LastValue.Hour;
if (isNumber(h))
{
if ((h >= StartHour) && (h <= EndHour))
ret = true;
}
} catch (Exception e)
{
throw new Exception("Error en la lectura de fecha y hora de las barras", e);
}
return ret;
}
private bool isNumber(double val)
{
return !double.IsNaN(val);
}
}
}
Any advice would be appreciated. Thank you.
@Abstract