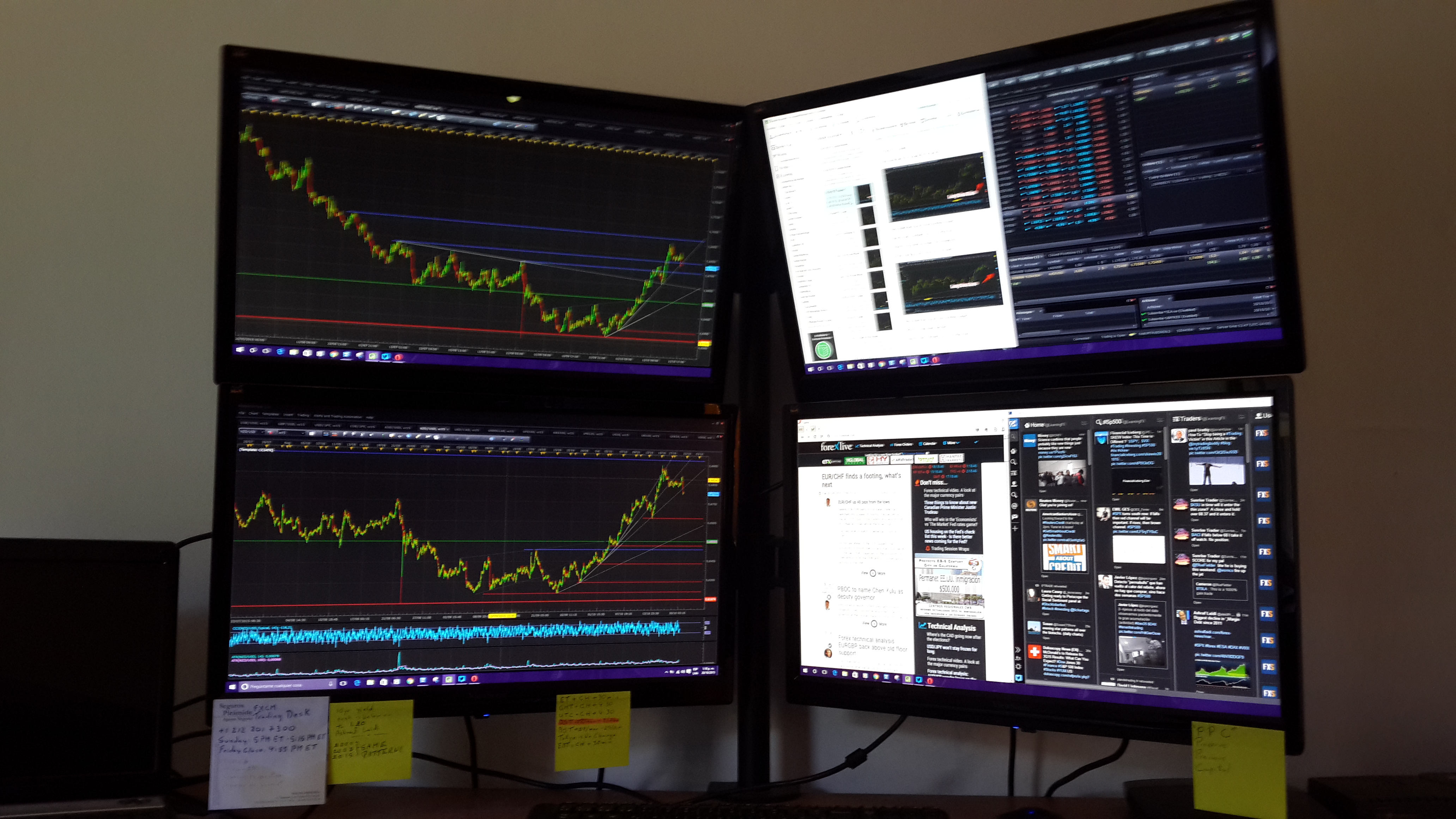
Issues with loading tick data on a renko chart
24 Feb 2025, 19:23
Hello cTrader,
I'm encountering some issues with tick data on a renko chart, it's a bit erratic, sometimes it loads, and sometimes it doesn't
24/02/2025 19:16:15.348 | Error | Indicator instance [TicksPerRenkoBar, EURUSD, h1] process stopped responding and was terminated.
Also, when I try to load historical data (the commented code), it doesn't work at all. Would you please check this issue?
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Collections;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo;
[Indicator(AccessRights = AccessRights.None, IsOverlay = false)]
public class TicksPerRenkoBar : Indicator
{
[Output("Ticks Per Bar", LineColor = "Lime")]
public IndicatorDataSeries OutputTicksPerBar { get; set; }
private Ticks _historicalTicks;
protected override void Initialize()
{
_historicalTicks = MarketData.GetTicks();
//
// while (_historicalTicks.First().Time > Times.First())
// {
// var loaded = _historicalTicks.LoadMoreHistory();
// Print($"Loaded {loaded} historical ticks");
//
// if (loaded == 0)
// Print($"No more bars to load");
// }
}
public override void Calculate(int index)
{
OutputTicksPerBar[index - 1] = _historicalTicks.Count(t => t.Time >= Times[index - 1] && t.Time <= Times[index]);
}
protected override void OnException(Exception exception)
{
Print($"Exception Occurred: {exception.Message}");
Print($"Stack Trace: {exception.StackTrace}");
}
public DataSeries Open => Bars.OpenPrices;
public DataSeries High => Bars.HighPrices;
public DataSeries Low => Bars.LowPrices;
public DataSeries Close => Bars.ClosePrices;
public TimeSeries Times => Bars.OpenTimes;
public int Index => Bars.Count - 1;
}
Thanks once again for your support.
Regards,
firemyst
25 Feb 2025, 00:04
You're falling into a trap a lot of people do when trying to load back tick data for Renko charts.
The trap is you're doing everything in the initialize method, instead of setting up your code to load asynchronously. The issue is, depending on how far back you load the data from, there could be an absolutely boat-load of data to download. For instance, if you do more than a week on the indices such as the DOW, DAX, or NAS, there's going to be hundreds of thousands of ticks.
And to compound the issue, if the server connection isn't the fastest, or you have slow internet, or something else, it will take a loooooooooooong time to download, hence the process being automatically terminated by cTrader for not responding.
So you need to start loading the back-tick data asynchronously and keep that going until all the tick data you want is loaded asychronously. Set a flag to indicate when it's done.
In the meantime, in the calculate method (on each incoming tick), check that the back data is fully loaded (eg, your flag) before you do anything else.
In regards to your commented code, I tried to make heads/tails out of it. In a nutshell, doing it asynchronously, you want to do something like this:
yourStructureForStoringTickdata.OpenTimes.Reverse().LastOrDefault() > _fromDateTime
{
yourStrutureForStoringTickData.LoadMoreHistoryAsync( … )
}
@firemyst