cBot works locally but not on the cloud
20 Jan 2025, 21:24
i have made a custom indicator and used it in a cBot. the bot works perfectly fine when used locally on my PC but does not work when used on the cloud (does not open or close positions) , what could be the reason and how can i solve this ?
This is the custom indicator code :
using System;
using cAlgo.API;
using cAlgo.API.Collections;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Indicator(AccessRights = AccessRights.None)]
public class bullbearpower : Indicator
{
[Parameter(DefaultValue = 9)]
public int Periods { get; set; }
[Output("BBPhisto", LineColor = "Gray", PlotType = PlotType.Histogram)]
public IndicatorDataSeries BBPhisto { get; set; }
[Output("BBP Green histo", LineColor = "Green", PlotType = PlotType.Histogram)]
public IndicatorDataSeries BBPGreenhisto { get; set; }
[Output("BBP Red histo", LineColor = "Red", PlotType = PlotType.Histogram)]
public IndicatorDataSeries BBPRedhisto { get; set; }
private MovingAverage Hullopen;
private MovingAverage HullHigh;
private MovingAverage HullLow;
protected override void Initialize()
{
Hullopen = Indicators.MovingAverage(Bars.OpenPrices, Periods, MovingAverageType.Hull);
HullHigh = Indicators.MovingAverage(Bars.HighPrices, Periods, MovingAverageType.Hull);
HullLow = Indicators.MovingAverage(Bars.LowPrices, Periods, MovingAverageType.Hull);
}
public override void Calculate(int index)
{
double hullo = Hullopen.Result[index];
double hullh = HullHigh.Result[index];
double hulll = HullLow.Result[index];
double bullp = hullh - hullo;
double bearp = hulll - hullo;
double BBP = bullp + bearp;
BBPhisto[index] = BBP;
if (BBP > 0)
{
BBPGreenhisto[index] = BBP;
BBPRedhisto[index] = 0;
}
else
{
BBPGreenhisto[index] = 0;
BBPRedhisto[index] = BBP;
}
}
}
}
And this is the cBot code :
using System;
using cAlgo.API;
using cAlgo.API.Collections;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo.Robots
{
[Robot(AccessRights = AccessRights.None, AddIndicators = true)]
public class Bbpbot : Robot
{
[Parameter(DefaultValue = 1)]
public double Volume { get; set; }
bullbearpower _BBP;
protected override void OnStart()
{
_BBP = Indicators.GetIndicator<bullbearpower>();
}
protected override void OnTick()
{
double currentBBP = _BBP.BBPhisto.LastValue;
double previousBBP = _BBP.BBPhisto.Last(1);
if (currentBBP > 0 && previousBBP <= 0)
{
Open(TradeType.Buy);
Close(TradeType.Sell);
}
else if (currentBBP < 0 && previousBBP >= 0)
{
Close(TradeType.Buy);
Open(TradeType.Sell);
}
}
private void Open(TradeType tradeType)
{
if (Positions.Count == 0)
ExecuteMarketOrder(tradeType, SymbolName, Volume);
}
private void Close(TradeType tradeType)
{
foreach (var position in Positions)
{
if (position.TradeType == tradeType) // Manually filter positions by TradeType
{
position.Close();
}
}
}
protected override void OnStop()
{
// Handle cBot stop here
}
}
}
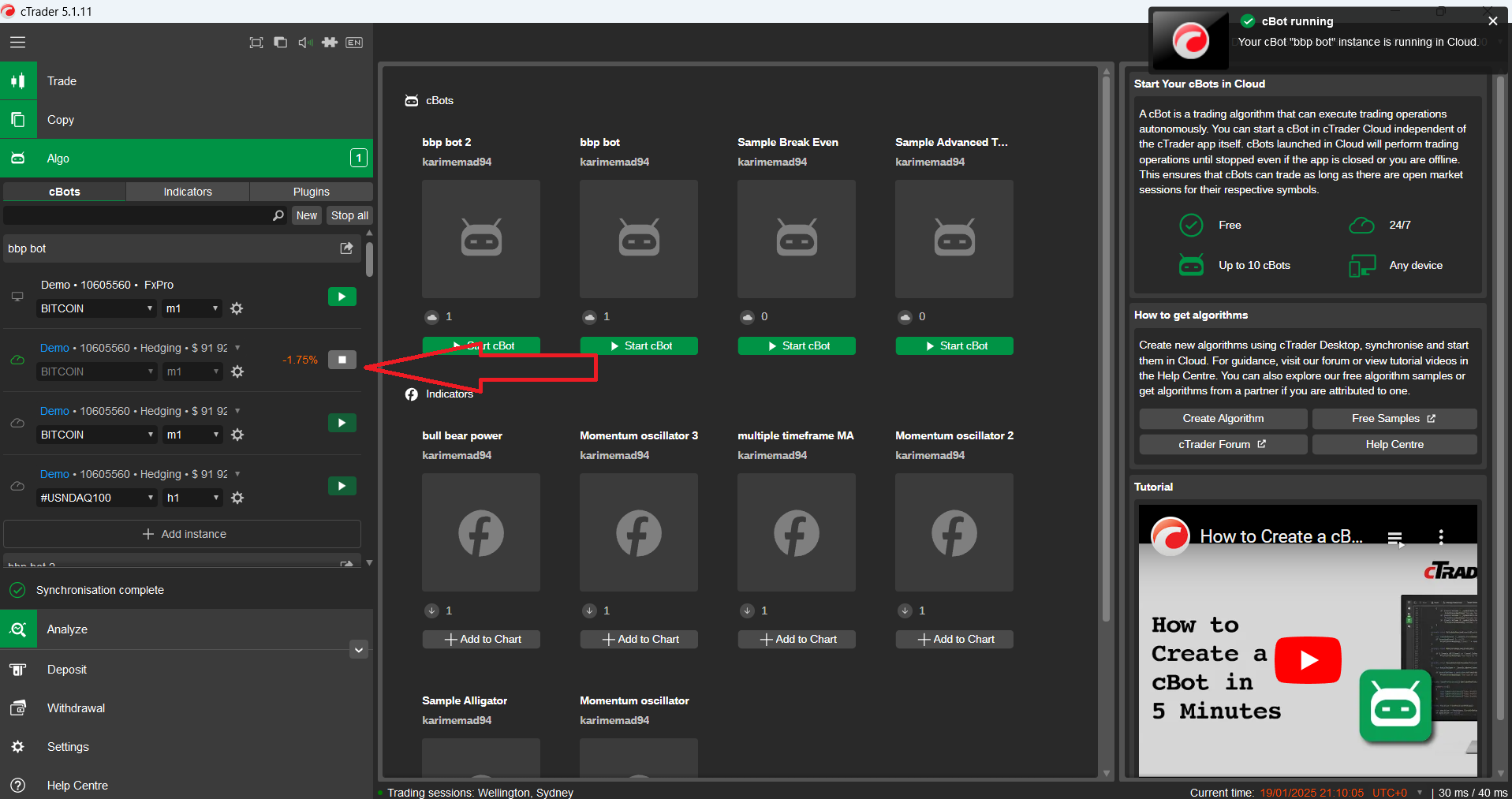
firemyst
07 Feb 2025, 02:52 ( Updated at: 14 Feb 2025, 18:18 )
Limitations of cbots in the cloud:
https://www.spotware.com/terms-of-services
@firemyst