Check visually where the bot is placing orders
07 Feb 2024, 16:39
Hi,
I am very new to trading and ctrader. I am trying to do small scripts based on my little knowledge.
The bot is placing orders and many of them end in loss. I want to check visually what the bot is doing, so I can correct the behaviour. Some kind of marker to show where the order is being placed.
My script is pretty simple. I am using 2 exponential moving averages (8 and 21) and, after crossing, I wait for them to separate by a value greater than a chosen value in pips, which is the difference between bot MAs. This way, I can decide if I should place an order or not, and not base my decision on crossings only. With this, I can avoid situations in which the ema curves will cross over and over again with very little difference, leading to loss.
Is there a way of doing this?
Please find below my script.
Kind regards,
Pedro
using System; using cAlgo.API; using cAlgo.API.Indicators;
namespace EMACrossWithConfirmation { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)] public class EMACrossBot : Robot { //[Parameter("Volume", DefaultValue = 10000, MinValue = 1)] //public int Volume { get; set; }
private ExponentialMovingAverage _ema1; private ExponentialMovingAverage _ema2; private Position _lastPosition; private double _lastDifference; private int Volume=1000;
// Variable for the minimum difference in pips [Parameter("EMAs delta", Group = "Moving Averages",DefaultValue = 0.5)] public double _minimumDifferenceInPips { get; set; }
[Parameter("Stop Loss", Group = "Protection", DefaultValue = 0)] public int StopLoss { get; set; }
[Parameter("Take Profit", Group = "Protection",DefaultValue =0)] public int TakeProfit { get; set; }
[Parameter("Fast EMA", Group = "Moving Averages",DefaultValue = 8)] public int period1 { get; set; }
[Parameter("Slow EMA", Group = "Moving Averages",DefaultValue = 21)] public int period2 { get; set; } protected override void OnStart() {
protected override void OnBar() { // Calculate the difference between two EMAs in pips double differenceInPips = CalculateDifferenceInPips();
// Check for EMA crossover after closing the position to avoid reopening immediately if (IsEMACrossOver()) { // Close the previous order (if any) if (_lastPosition != null) { ClosePosition(_lastPosition); _lastPosition = null; // Reset the last position }
// Place a new buy or sell order based on the direction of the difference if (Math.Abs(differenceInPips) > _minimumDifferenceInPips && differenceInPips>0) { PlaceOrder(TradeType.Buy); Print("Buy"); Print(differenceInPips); } else if (Math.Abs(differenceInPips) > _minimumDifferenceInPips && differenceInPips < 0) { PlaceOrder(TradeType.Sell); Print("Sell!"); Print(differenceInPips); } } }
private double CalculateDifferenceInPips() { // Get the pip size double pipSize = Symbol.PipSize;
// Calculate the difference between two EMAs double ema1Value = _ema1.Result.LastValue; double ema2Value = _ema2.Result.LastValue;
double difference = ema1Value - ema2Value;
// Calculate the difference in pips double differenceInPips = difference / pipSize;
private void PlaceOrder(TradeType tradeType) { // Place a new order ExecuteMarketOrder(tradeType, Symbol, Volume, "EMACross Confirmation", StopLoss, TakeProfit, null, "EMA");
// Store the position _lastPosition = Positions.Find("EMA", Symbol, tradeType); } } }
If your Deal map is enabled, then when you click on a deal in history, you will see it drawn on the chart
Hi,
Thanks for your reply. In backtesting, it is not saving history do I can't see anything. Is there a way to enable history? I only have data in the “positions” column.
If your Deal map is enabled, then when you click on a deal in history, you will see it drawn on the chart
Hi,
Thanks for your reply. In backtesting, it is not saving history do I can't see anything. Is there a way to enable history? I only have data in the “positions” column.
Kind regards,
Pedro
Hi there,
History is saved in backtesting. If you do not see anything, it means no positions have been closed.
PanagiotisCharalampous
08 Feb 2024, 07:00
Hi there,
You can use Visual Backtesting and visualize your trades using Deal Map
https://help.ctrader.com/ctrader-automate/backtesting-and-optimizing-cbots/?h=visual+backtesting#real-time-visual-mode-backtesting
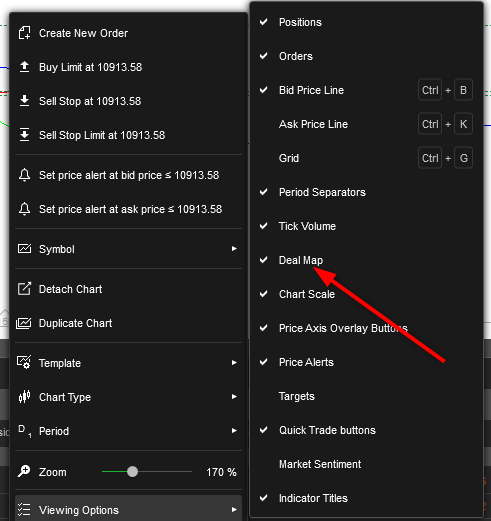
If your Deal map is enabled, then when you click on a deal in history, you will see it drawn on the chart
@PanagiotisCharalampous