Is there any way to retrieve the bar's bar count at which the position was entered?
Is there any way to retrieve the bar's bar count at which the position was entered?
12 Oct 2023, 03:07
Hi, anyone please help if there is any way to retrieve the bar count at which the position was entered? my strategy is using OnBarClosed() method, please guide me how should I exactly retrieve that bar count or retrieve that bar's OHLC value, thanks in advanced !
Replies
JXTA
18 Oct 2023, 09:38
RE: Is there any way to retrieve the bar's bar count at which the position was entered?
firemyst said:
To get the index of the bar where the latest position was opened:
Position p; //get your position information
//then get the index:
int barIndex = Bars.OpenTimes.GetIndexByTime(p.EntryTime);
Hi Firemyst just saw your comment! Thanks for your help! It helps me a lot!
I use the below code instead of Position p; you gave me
public Position[] LongPositions
{
get
{
return Positions.FindAll(LongLabel);
}
}
public Position[] ShortPositions
{
get
{
return Positions.FindAll(ShortLabel);
}
}
and it says :
‘Position[]’ does not contain a definition for ‘EntryTime’ and no accessible extension method ‘EntryTime’ accepting a fist argument of type ‘Position[]’ could be found
Is there any way to define current position type by Buy and Sell separately ? Because my strategy is allowing buy and sell logic execute at the same time
@JXTA
firemyst
18 Oct 2023, 11:52
RE: RE: Is there any way to retrieve the bar's bar count at which the position was entered?
JINGXUAN said:
and it says :
‘Position[]’ does not contain a definition for ‘EntryTime’ and no accessible extension method ‘EntryTime’ accepting a fist argument of type ‘Position[]’ could be found
Of course it doesn't contain a definition. Your getting Long/Short positions returns arrays of Position objects; my example is with a single Position object.
The property “EntryTime” is for a single Position object, not an array of Position objects.
@firemyst
JXTA
18 Oct 2023, 13:06
( Updated at: 21 Dec 2023, 09:23 )
RE: RE: RE: Is there any way to retrieve the bar's bar count at which the position was entered?
firemyst said:
JINGXUAN said:
and it says :
‘Position[]’ does not contain a definition for ‘EntryTime’ and no accessible extension method ‘EntryTime’ accepting a fist argument of type ‘Position[]’ could be found
Of course it doesn't contain a definition. Your getting Long/Short positions returns arrays of Position objects; my example is with a single Position object.
The property “EntryTime” is for a single Position object, not an array of Position objects.
I see, pardon my mistake on that what I've asked, I am not a programmer,
Anyway, from what you have showed me previously
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using cAlgo.API;
using cAlgo.API.Collections;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo.Robots
{
[Robot(AccessRights = AccessRights.None)]
public class XXX: Robot
{
Position p;
protected override void OnStart()
{
int barIndex = Bars.OpenTimes.GetIndexByTime(p.EntryTime);
}
protected override void OnBar()
{
... ...
}
}
}
is that possible to extend it into something to define the current position trade type and then get that specific bar index ? or is there anyway to do it?
I will explain my idea here with code and photo
I have created a timer to let it count once a position is opened, but I doesnt know how to command it count specifically after a Buy order is opened or a Sell order is opened, because I am allowing my strategy to execute another trade type before one is closed, so I am needing this so much
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using cAlgo.API;
using cAlgo.API.Collections;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo.Robots
{
[Robot(AccessRights = AccessRights.None)]
public class TimerExample : Robot
{
//******************************************Generals******************************************
[Parameter("Volume (Lots)", DefaultValue = 0.1, MaxValue = 5, MinValue = 0.1, Step = 0.1)]
public double VolumeInLots { get; set; }
//******************************************Timer******************************************
private int _barCountToPosition;
private bool _startTimer;
//******************************************SMAs******************************************
[Parameter("SMA Source")]
public DataSeries SMASource { get; set; }
[Parameter("SMA_A Period", DefaultValue = 30)]
public int Sma_30_Period { get; set; }
[Parameter("SMA_B Period", DefaultValue = 60)]
public int Sma_60_Period { get; set; }
private SampleSMA _sma30;
private SampleSMA _sma60;
protected override void OnStart()
{
//Positions Timer
Positions.Opened += PositionOnOpened;
//SMA
_sma30 = Indicators.GetIndicator<SampleSMA>(SMASource, Sma_30_Period);
_sma60 = Indicators.GetIndicator<SampleSMA>(SMASource, Sma_60_Period);
}
void PositionOnOpened(PositionOpenedEventArgs obj)
{
_startTimer = true;
}
protected override void OnBar()
{
//LongLogic
if
( Positions.Find("LONG", SymbolName, TradeType.Buy) == null
&&
_sma30.Result.LastValue > _sma60.Result.LastValue || Bars.ClosePrices.LastValue > _sma30.Result.LastValue
)
{
ExecuteMarketOrder(TradeType.Buy, SymbolName, VolumeInLots, "LONG");
}
//ShortLogic
if
( Positions.Find("SHORT", SymbolName, TradeType.Sell) == null
&&
_sma30.Result.LastValue < _sma60.Result.LastValue || Bars.ClosePrices.LastValue < _sma30.Result.LastValue)
{
ExecuteMarketOrder(TradeType.Sell, SymbolName, VolumeInLots, "SHORT");
}
//LongTP
else if
(
Bars.ClosePrices.LastValue < _sma60.Result.LastValue && Positions[0].NetProfit > 0
)
{
ClosePosition(Positions.Find("LONG", SymbolName, TradeType.Buy));
}
//ShortTP
else if
(
Bars.ClosePrices.LastValue > _sma60.Result.LastValue && Positions[0].NetProfit > 0
)
{
ClosePosition(Positions.Find("SHORT", SymbolName, TradeType.Sell));
}
//LongStopLoss
/* (I want to write a Long stop loss based on the LowPrices of the Bar where the latest position was entered) */
else if
(
Bars.ClosePrices.Last(1) < Bars.LowPrices.Last( /*LongPositionEnteredBarIndex*/ ) && Positions[0].NetProfit < 0
)
{
ClosePosition(Positions.Find("LONG", SymbolName, TradeType.Buy));
}
//ShortStopLoss
/* (I want to write a Short stop loss based on the HighPrices of the Bar where the latest position was entered) */
else if
(
Bars.ClosePrices.LastValue > Bars.HighPrices.Last( /*ShortPositionEnteredBarIndex*/ ) && Positions[0].NetProfit < 0
)
{
ClosePosition(Positions.Find("SHORT", SymbolName, TradeType.Sell));
}
}
}
}
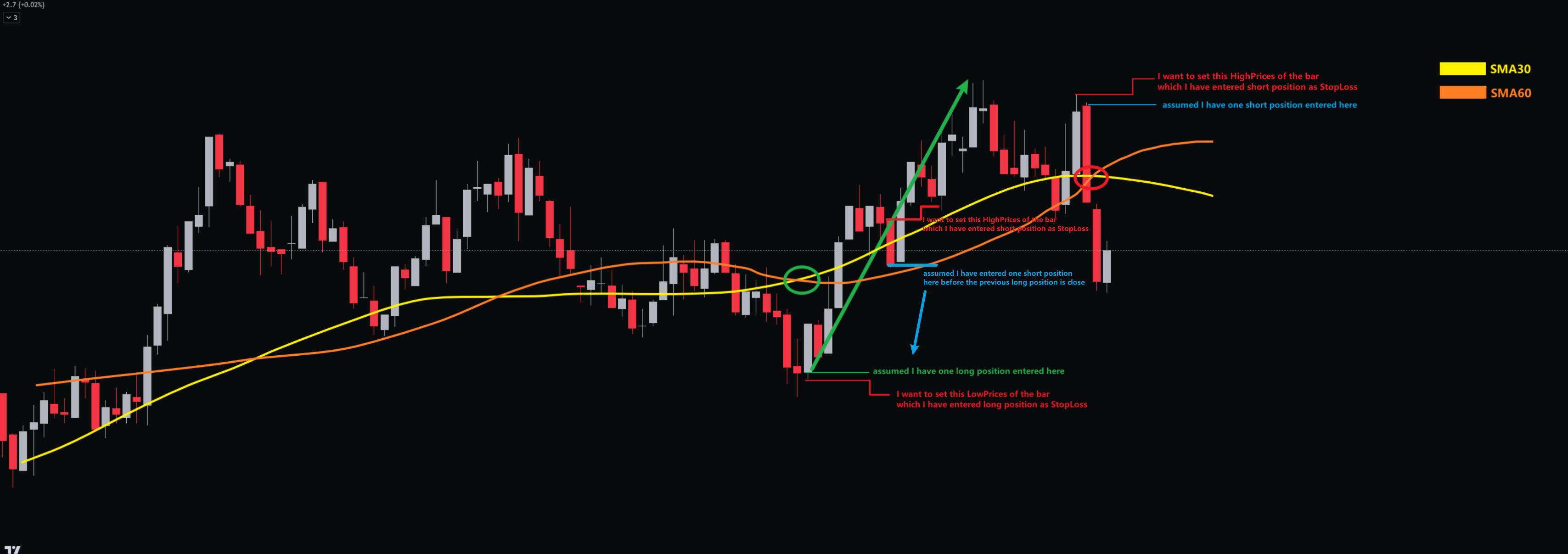
Could you help me with it ? How should I get that bar index
or Anyone could help me with this, thanks in advanced !
@JXTA
firemyst
14 Oct 2023, 15:34
To get the index of the bar where the latest position was opened:
Position p; //get your position information
//then get the index:
int barIndex = Bars.OpenTimes.GetIndexByTime(p.EntryTime);
@firemyst