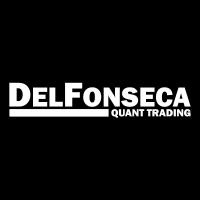
Problems with .AddChild and .RemoveChild after update
14 Jul 2023, 23:26
Hi,
After the last update (version 4.8.15.18913) the .AddChild and .RemoveChild stopped working.
I have a combobox with an extensive list of options, by choosing, it creates grids with texbox, textblock, checkbox, etc ... and removes all grids created previously.
Interesting, my combobox has LocalStorage, if I restart the indicator, it starts with the controls properly created, it loads everything correctly.
Here is a code example, it should work, but it ignores the creation and removal childs. But in the Log it does the Print().
Thanks in advance!
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using cAlgo.API;
using cAlgo.API.Collections;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Indicator(AccessRights = AccessRights.None)]
public class Test : Indicator
{
private ComboBox myDropList;
private Grid myDropListGrid, gridItemA, gridItemB, gridItemC;
private string selectedValue;
private TextBlock lblTextA, lblTextB, lblTextC;
private bool lockGridExecuteA, lockGridExecuteB, lockGridExecuteC;
protected override void Initialize()
{
selectedValue = null;
lockGridExecuteA = false;
lockGridExecuteB = false;
lockGridExecuteC = false;
myDropListGrid = new Grid(2, 1)
{
Width = 100,
Height = 100,
HorizontalAlignment = HorizontalAlignment.Center,
VerticalAlignment = VerticalAlignment.Center,
ShowGridLines = true
};
myDropList = new ComboBox
{
Width = 100,
Height = 20,
HorizontalContentAlignment = HorizontalAlignment.Center,
VerticalContentAlignment = VerticalAlignment.Center
};
myDropList.AddItem("A");
myDropList.AddItem("B");
myDropList.AddItem("C");
myDropList.SelectedItemChanged += myDropList_SelectedIndexChanged;
Border myDropListBorder = new Border()
{
Child = myDropList,
};
myDropListGrid.AddChild(myDropListBorder, 0, 0);
Chart.AddControl(myDropListGrid);
}
private void myDropList_SelectedIndexChanged(ComboBoxSelectedItemChangedEventArgs obj)
{
if (myDropList.SelectedIndex >= 0)
{
selectedValue = myDropList.SelectedItem.ToString();
switch (selectedValue)
{
case "A":
RemoveIndicatorGrids();
ExecuteA();
break;
case "B":
RemoveIndicatorGrids();
ExecuteB();
break;
case "C":
RemoveIndicatorGrids();
ExecuteC();
break;
}
}
}
private void ExecuteA()
{
if(!lockGridExecuteA)
{
gridItemA = new Grid(1, 1);
myDropListGrid.AddChild(gridItemA, 1, 0);
lblTextA = CreateLabel("Item A");
Print("Item A created");
gridItemA.AddChild(lblTextA, 0, 0);
lockGridExecuteA = true;
}
}
private void ExecuteB()
{
if(!lockGridExecuteB)
{
gridItemB = new Grid(1, 1);
myDropListGrid.AddChild(gridItemB, 1, 0);
lblTextB = CreateLabel("Item B");
Print("Item B created");
gridItemB.AddChild(lblTextB, 0, 0);
lockGridExecuteB = true;
}
}
private void ExecuteC()
{
if(!lockGridExecuteC)
{
gridItemC = new Grid(1, 1);
myDropListGrid.AddChild(gridItemC, 1, 0);
lblTextC = CreateLabel("Item C");
Print("Item C created");
gridItemC.AddChild(lblTextC, 0, 0);
lockGridExecuteC = true;
}
}
private void RemoveIndicatorGrids()
{
if (selectedValue != null)
{
if (selectedValue != "A" && lockGridExecuteA)
{
myDropListGrid.RemoveChild(gridItemA);
Print("Remove gridItemA");
lockGridExecuteA = false;
}
if (selectedValue != "B" && lockGridExecuteB)
{
myDropListGrid.RemoveChild(gridItemB);
Print("Remove gridItemB");
lockGridExecuteB = false;
}
if (selectedValue != "C" && lockGridExecuteC)
{
myDropListGrid.RemoveChild(gridItemC);
Print("Remove gridItemC");
lockGridExecuteC = false;
}
}
}
private TextBlock CreateLabel(string text)
{
return new TextBlock
{
Text = text,
TextAlignment = TextAlignment.Center,
HorizontalAlignment = HorizontalAlignment.Center,
VerticalAlignment = VerticalAlignment.Center,
ForegroundColor = Color.Red,
FontSize = 12
};
}
public override void Calculate(int index)
{
//...
}
}
}
Replies
AlexFrei
18 Jul 2023, 19:09
( Updated at: 18 Jul 2023, 19:12 )
RE:
Spotware said:
Hi DelFonseca,
Thank you for reporting this issue. We managed to reproduce this and we will fix it in an upcoming update.
Best regards,
cTrader Team
Hello, I have very similar problem ! I have Grid control in Chart and Button as child of this Grid. On Button Click event I create next control (child of the same Grid) close to first Button. It worked on 4.7. version. But It doesnt work on 4.8.15 anymore. Button doesnt appear on click
Thank you for solving this issue.
Alex
@AlexFrei
DelFonseca
17 Jul 2023, 22:35
cTrader Team,
Check out this example, where a button is created at startup. Clicking on it should bring up two new buttons.
@DelFonseca