combobox.SelectedItemChanged
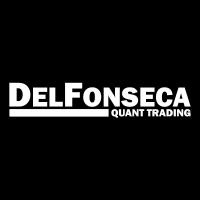
combobox.SelectedItemChanged
14 Feb 2023, 23:15
Hi all,
I am asking for your support to help me solve a problem where I create a combobox and when selecting a certain item from that combobox I want to execute a function. In the example below just a simple print.
I get no overload for matches delegate error.
Thanks in advance!
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using cAlgo.API;
using cAlgo.API.Collections;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Indicator(AccessRights = AccessRights.None)]
public class ComboBoxTest : Indicator
{
private ComboBox myDropList;
protected override void Initialize()
{
myDropList = new ComboBox
{
Width = 100,
Height = 20,
HorizontalContentAlignment = HorizontalAlignment.Center,
VerticalContentAlignment = VerticalAlignment.Center
};
myDropList.AddItem("Item 1");
myDropList.AddItem("Item 2");
myDropList.AddItem("Item 3");
Chart.AddControl(myDropList);
myDropList.SelectedItemChanged += myDropList_SelectedIndexChanged;
}
private void myDropList_SelectedIndexChanged(object sender, ComboBoxSelectedItemChangedEventArgs e)
{
if (myDropList.SelectedIndex != -1)
{
string selectedValue = myDropList.SelectedItem.ToString();
switch (selectedValue)
{
case "Item 1":
Print("Item 1 selected");
break;
case "Item 2":
Print("Item 2 selected");
break;
case "Item 3":
Print("Item 3 selected");
break;
default:
MessageBox.Show("The selected item was not recognized. Please contact the developer for assistance. Telegram: @DelFonseca");
break;
}
}
else
{
MessageBox.Show("Please select an item from the dropdown list.");
}
}
public override void Calculate(int index)
{
//...
}
}
}
Replies
ctid1830215
21 Feb 2023, 16:43
RE:
DelFonseca said:
up
try this
private void myDropList_SelectedIndexChanged(object sender, ComboBoxSelectedItemChangedEventArgs e)
{
string selectedValue = e.SelectedItem;
switch (selectedValue)
{
...
}
}
@ctid1830215
amusleh
21 Feb 2023, 18:04
( Updated at: 21 Feb 2023, 18:06 )
Hi,
This works fine for me on 4.6.2 and .NET 6:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using cAlgo.API;
using cAlgo.API.Collections;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Indicator(AccessRights = AccessRights.None)]
public class ComboBoxTest : Indicator
{
private ComboBox myDropList;
protected override void Initialize()
{
myDropList = new ComboBox
{
Width = 100,
Height = 20,
HorizontalContentAlignment = HorizontalAlignment.Center,
VerticalContentAlignment = VerticalAlignment.Center
};
myDropList.AddItem("Item 1");
myDropList.AddItem("Item 2");
myDropList.AddItem("Item 3");
Chart.AddControl(myDropList);
myDropList.SelectedItemChanged += myDropList_SelectedIndexChanged;
}
private void myDropList_SelectedIndexChanged(ComboBoxSelectedItemChangedEventArgs e)
{
if (myDropList.SelectedIndex != -1)
{
string selectedValue = myDropList.SelectedItem.ToString();
switch (selectedValue)
{
case "Item 1":
Print("Item 1 selected");
break;
case "Item 2":
Print("Item 2 selected");
break;
case "Item 3":
Print("Item 3 selected");
break;
default:
MessageBox.Show("The selected item was not recognized. Please contact the developer for assistance. Telegram: @DelFonseca");
break;
}
}
else
{
MessageBox.Show("Please select an item from the dropdown list.");
}
}
public override void Calculate(int index)
{
//...
}
}
}
Check event signature before using it: SelectedItemChanged Event - cTrader Automate API
@amusleh
DelFonseca
21 Feb 2023, 18:19
( Updated at: 21 Feb 2023, 23:41 )
RE:
amusleh said:
Hi,
This works fine for me on 4.6.2 and .NET 6:
//...
Check event signature before using it: SelectedItemChanged Event - cTrader Automate API
Thank you so much my friend!! Thank you
For those who are interested, the solution:
Change the line:
private void myDropList_SelectedIndexChanged(object sender, ComboBoxSelectedItemChangedEventArgs e)
to:
private void indicatorsDropList_SelectedIndexChanged(ComboBoxSelectedItemChangedEventArgs obj)
@DelFonseca
DelFonseca
06 May 2023, 17:40
RE:
amusleh said:
Hi,
This works fine for me on 4.6.2 and .NET 6:
(...)
Check event signature before using it: SelectedItemChanged Event - cTrader Automate API
Hi amusleh,
I was testing the indicator I am developing and came across a ctrader bug.
For example, with the code you provided, select "Item 2", change chart tab and go back to the tab where you have the indicator. It should keep "Item 2" selected. I have tried to solve it via code, but the logs return "Item 2" selected, I believe it is a visual bug. Can you test it there please?
Thanks in advance
@DelFonseca
TraderExperto
31 Jul 2023, 19:46
( Updated at: 21 Dec 2023, 09:23 )
RE: combobox.SelectedItemChanged
amusleh said:
Hi,
This works fine for me on 4.6.2 and .NET 6:
using System;using System.Collections.Generic;using System.Linq;using System.Text;using cAlgo.API;using cAlgo.API.Collections;using cAlgo.API.Indicators;using cAlgo.API.Internals;namespace cAlgo{ [Indicator(AccessRights = AccessRights.None)] public class ComboBoxTest : Indicator { private ComboBox myDropList; protected override void Initialize() { myDropList = new ComboBox { Width = 100, Height = 20, HorizontalContentAlignment = HorizontalAlignment.Center, VerticalContentAlignment = VerticalAlignment.Center }; myDropList.AddItem("Item 1"); myDropList.AddItem("Item 2"); myDropList.AddItem("Item 3"); Chart.AddControl(myDropList); myDropList.SelectedItemChanged += myDropList_SelectedIndexChanged; } private void myDropList_SelectedIndexChanged(ComboBoxSelectedItemChangedEventArgs e) { if (myDropList.SelectedIndex != -1) { string selectedValue = myDropList.SelectedItem.ToString(); switch (selectedValue) { case "Item 1": Print("Item 1 selected"); break; case "Item 2": Print("Item 2 selected"); break; case "Item 3": Print("Item 3 selected"); break; default: MessageBox.Show("The selected item was not recognized. Please contact the developer for assistance. Telegram: @DelFonseca"); break; } } else { MessageBox.Show("Please select an item from the dropdown list."); } } public override void Calculate(int index) { //... } }}
Check event signature before using it: SelectedItemChanged Event - cTrader Automate API
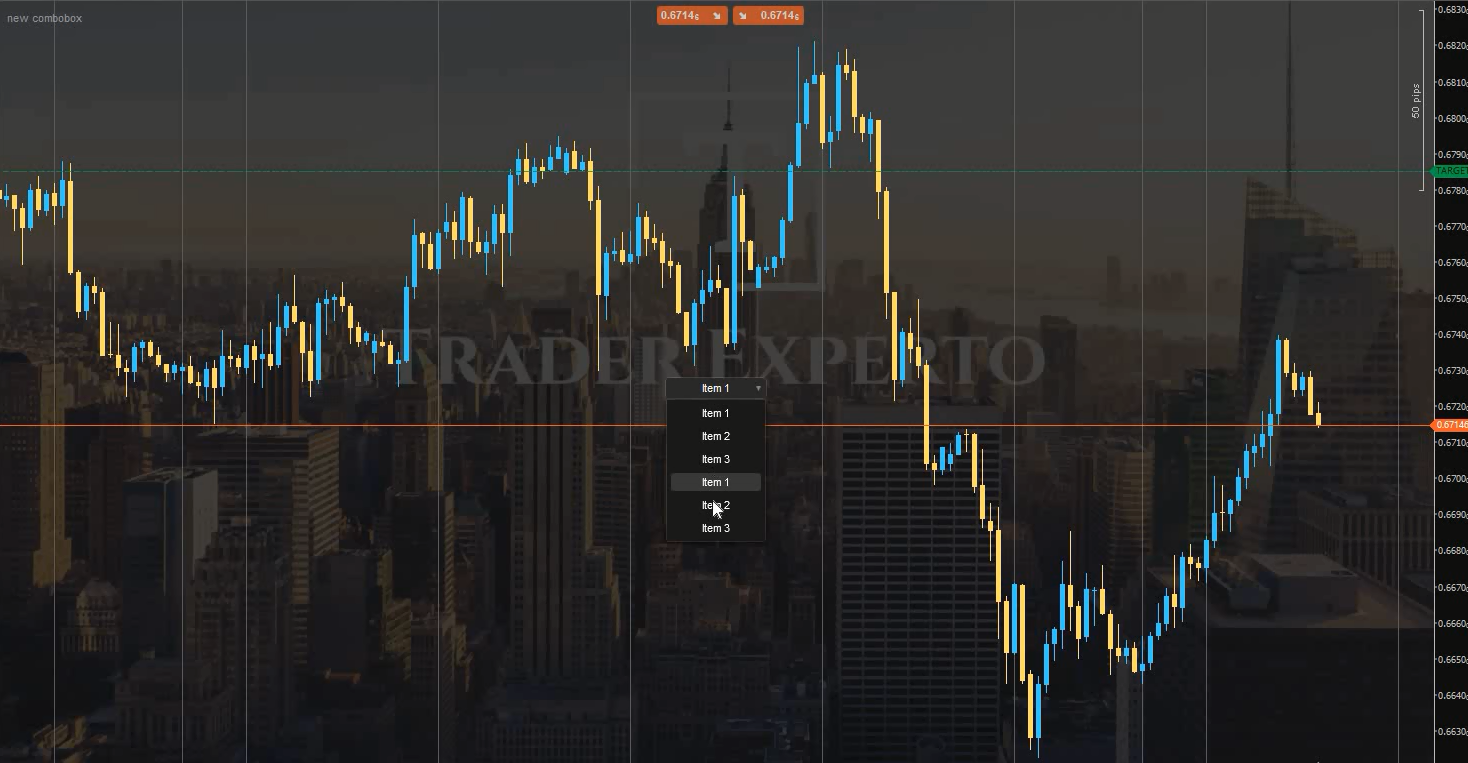
I'm getting this on Ctrader 3.8.17 the code has only Item 1, Item 2, Item 3 and i'm getting it twice for every item. And when i click on the additional items it returns the message as it doesn't exist! Is anything wrong with this example or is this a bug?
@TraderExperto
TraderExperto
31 Jul 2023, 20:05
( Updated at: 21 Dec 2023, 09:23 )
RE: RE: combobox.SelectedItemChanged
TraderExperto said:
amusleh said:
Hi,
This works fine for me on 4.6.2 and .NET 6:
using System;using System.Collections.Generic;using System.Linq;using System.Text;using cAlgo.API;using cAlgo.API.Collections;using cAlgo.API.Indicators;using cAlgo.API.Internals;namespace cAlgo{ [Indicator(AccessRights = AccessRights.None)] public class ComboBoxTest : Indicator { private ComboBox myDropList; protected override void Initialize() { myDropList = new ComboBox { Width = 100, Height = 20, HorizontalContentAlignment = HorizontalAlignment.Center, VerticalContentAlignment = VerticalAlignment.Center }; myDropList.AddItem("Item 1"); myDropList.AddItem("Item 2"); myDropList.AddItem("Item 3"); Chart.AddControl(myDropList); myDropList.SelectedItemChanged += myDropList_SelectedIndexChanged; } private void myDropList_SelectedIndexChanged(ComboBoxSelectedItemChangedEventArgs e) { if (myDropList.SelectedIndex != -1) { string selectedValue = myDropList.SelectedItem.ToString(); switch (selectedValue) { case "Item 1": Print("Item 1 selected"); break; case "Item 2": Print("Item 2 selected"); break; case "Item 3": Print("Item 3 selected"); break; default: MessageBox.Show("The selected item was not recognized. Please contact the developer for assistance. Telegram: @DelFonseca"); break; } } else { MessageBox.Show("Please select an item from the dropdown list."); } } public override void Calculate(int index) { //... } }}
Check event signature before using it: SelectedItemChanged Event - cTrader Automate API
I'm getting this on Ctrader 3.8.17 the code has only Item 1, Item 2, Item 3 and i'm getting it twice for every item. And when i click on the additional items it returns the message as it doesn't exist! Is anything wrong with this example or is this a bug?
Ok it looks like if you put the Combobox as a child of a Border Control the problem is solved. Here is the code!
protected override void Initialize()
{
myDropList = new ComboBox
{
Width = 100,
Height = 20,
HorizontalContentAlignment = HorizontalAlignment.Center,
VerticalContentAlignment = VerticalAlignment.Center
};
myDropList.AddItem("Item 1");
myDropList.AddItem("Item 2");
myDropList.AddItem("Item 3");
myDropList.SelectedIndex = 0;
Border myDropListBorder = new Border()
{
Child = myDropList,
};
Chart.AddControl(myDropListBorder);
myDropList.SelectedItemChanged += myDropList_SelectedIndexChanged;
}

@TraderExperto
newbee
20 Oct 2023, 02:12
( Updated at: 20 Oct 2023, 05:18 )
RE: combobox.SelectedItemChanged
amusleh said:
Hi,
This works fine for me on 4.6.2 and .NET 6:
using System;using System.Collections.Generic;using System.Linq;using System.Text;using cAlgo.API;using cAlgo.API.Collections;using cAlgo.API.Indicators;using cAlgo.API.Internals;namespace cAlgo{ [Indicator(AccessRights = AccessRights.None)] public class ComboBoxTest : Indicator { private ComboBox myDropList; protected override void Initialize() { myDropList = new ComboBox { Width = 100, Height = 20, HorizontalContentAlignment = HorizontalAlignment.Center, VerticalContentAlignment = VerticalAlignment.Center }; myDropList.AddItem("Item 1"); myDropList.AddItem("Item 2"); myDropList.AddItem("Item 3"); Chart.AddControl(myDropList); myDropList.SelectedItemChanged += myDropList_SelectedIndexChanged; } private void myDropList_SelectedIndexChanged(ComboBoxSelectedItemChangedEventArgs e) { if (myDropList.SelectedIndex != -1) { string selectedValue = myDropList.SelectedItem.ToString(); switch (selectedValue) { case "Item 1": Print("Item 1 selected"); break; case "Item 2": Print("Item 2 selected"); break; case "Item 3": Print("Item 3 selected"); break; default: MessageBox.Show("The selected item was not recognized. Please contact the developer for assistance. Telegram: @DelFonseca"); break; } } else { MessageBox.Show("Please select an item from the dropdown list."); } } public override void Calculate(int index) { //... } }}
Check event signature before using it: SelectedItemChanged Event - cTrader Automate API
Hello amusleh,
You helped resolve a query with ComboBox's not so long ago, (found here https://ctrader.com/forum/calgo-support/40141) and I was hoping you may be able to help me too. I've been trying to add a this ComboBox to the SampleTradePanel for some time now but unfortunately have not been able to work it out.
Are you able to help me with a sample code showing how to add this combobox to the SampleTradingPanel. If we just replaced the “close all button” with this ComboBox please.
Below is the code from the SampleTradePanel
Any assistance would be greatly appreciated. Thank you
Following is the Sample trade Panel:
using System;
using System.Collections.Generic;
using cAlgo.API;
using cAlgo.API.Internals;
namespace cAlgo
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class SampleTradingPanel : Robot
{
[Parameter("Vertical Position", Group = "Panel alignment", DefaultValue = VerticalAlignment.Top)]
public VerticalAlignment PanelVerticalAlignment { get; set; }
[Parameter("Horizontal Position", Group = "Panel alignment", DefaultValue = HorizontalAlignment.Left)]
public HorizontalAlignment PanelHorizontalAlignment { get; set; }
[Parameter("Default Lots", Group = "Default trade parameters", DefaultValue = 0.01)]
public double DefaultLots { get; set; }
[Parameter("Default Take Profit (pips)", Group = "Default trade parameters", DefaultValue = 20)]
public double DefaultTakeProfitPips { get; set; }
[Parameter("Default Stop Loss (pips)", Group = "Default trade parameters", DefaultValue = 20)]
public double DefaultStopLossPips { get; set; }
protected override void OnStart()
{
var tradingPanel = new TradingPanel(this, Symbol, DefaultLots, DefaultStopLossPips, DefaultTakeProfitPips);
var border = new Border
{
VerticalAlignment = PanelVerticalAlignment,
HorizontalAlignment = PanelHorizontalAlignment,
Style = Styles.CreatePanelBackgroundStyle(),
Margin = "20 40 20 20",
Width = 225,
Child = tradingPanel
};
Chart.AddControl(border);
}
}
public class TradingPanel : CustomControl
{
private const string LotsInputKey = "LotsKey";
private const string TakeProfitInputKey = "TPKey";
private const string StopLossInputKey = "SLKey";
private readonly IDictionary<string, TextBox> _inputMap = new Dictionary<string, TextBox>();
private readonly Robot _robot;
private readonly Symbol _symbol;
public TradingPanel(Robot robot, Symbol symbol, double defaultLots, double defaultStopLossPips, double defaultTakeProfitPips)
{
_robot = robot;
_symbol = symbol;
AddChild(CreateTradingPanel(defaultLots, defaultStopLossPips, defaultTakeProfitPips));
}
private ControlBase CreateTradingPanel(double defaultLots, double defaultStopLossPips, double defaultTakeProfitPips)
{
var mainPanel = new StackPanel();
var header = CreateHeader();
mainPanel.AddChild(header);
var contentPanel = CreateContentPanel(defaultLots, defaultStopLossPips, defaultTakeProfitPips);
mainPanel.AddChild(contentPanel);
return mainPanel;
}
private ControlBase CreateHeader()
{
var headerBorder = new Border
{
BorderThickness = "0 0 0 1",
Style = Styles.CreateCommonBorderStyle()
};
var header = new TextBlock
{
Text = "Quick Trading Panel",
Margin = "10 7",
Style = Styles.CreateHeaderStyle()
};
headerBorder.Child = header;
return headerBorder;
}
private StackPanel CreateContentPanel(double defaultLots, double defaultStopLossPips, double defaultTakeProfitPips)
{
var contentPanel = new StackPanel
{
Margin = 10
};
var grid = new Grid(4, 3);
grid.Columns[1].SetWidthInPixels(5);
var sellButton = CreateTradeButton("SELL", Styles.CreateSellButtonStyle(), TradeType.Sell);
grid.AddChild(sellButton, 0, 0);
var buyButton = CreateTradeButton("BUY", Styles.CreateBuyButtonStyle(), TradeType.Buy);
grid.AddChild(buyButton, 0, 2);
var lotsInput = CreateInputWithLabel("Quantity (Lots)", defaultLots.ToString("F2"), LotsInputKey);
grid.AddChild(lotsInput, 1, 0, 1, 3);
var stopLossInput = CreateInputWithLabel("Stop Loss (Pips)", defaultStopLossPips.ToString("F1"), StopLossInputKey);
grid.AddChild(stopLossInput, 2, 0);
var takeProfitInput = CreateInputWithLabel("Take Profit (Pips)", defaultTakeProfitPips.ToString("F1"), TakeProfitInputKey);
grid.AddChild(takeProfitInput, 2, 2);
var closeAllButton = CreateCloseAllButton();
grid.AddChild(closeAllButton, 3, 0, 1, 3);
contentPanel.AddChild(grid);
return contentPanel;
}
private Button CreateTradeButton(string text, Style style, TradeType tradeType)
{
var tradeButton = new Button
{
Text = text,
Style = style,
Height = 25
};
tradeButton.Click += args => ExecuteMarketOrderAsync(tradeType);
return tradeButton;
}
private ControlBase CreateCloseAllButton()
{
var closeAllBorder = new Border
{
Margin = "0 10 0 0",
BorderThickness = "0 1 0 0",
Style = Styles.CreateCommonBorderStyle()
};
var closeButton = new Button
{
Style = Styles.CreateCloseButtonStyle(),
Text = "Close All",
Margin = "0 10 0 0"
};
closeButton.Click += args => CloseAll();
closeAllBorder.Child = closeButton;
return closeAllBorder;
}
private Panel CreateInputWithLabel(string label, string defaultValue, string inputKey)
{
var stackPanel = new StackPanel
{
Orientation = Orientation.Vertical,
Margin = "0 10 0 0"
};
var textBlock = new TextBlock
{
Text = label
};
var input = new TextBox
{
Margin = "0 5 0 0",
Text = defaultValue,
Style = Styles.CreateInputStyle()
};
_inputMap.Add(inputKey, input);
stackPanel.AddChild(textBlock);
stackPanel.AddChild(input);
return stackPanel;
}
private void ExecuteMarketOrderAsync(TradeType tradeType)
{
var lots = GetValueFromInput(LotsInputKey, 0);
if (lots <= 0)
{
_robot.Print(string.Format("{0} failed, invalid Lots", tradeType));
return;
}
var stopLossPips = GetValueFromInput(StopLossInputKey, 0);
var takeProfitPips = GetValueFromInput(TakeProfitInputKey, 0);
_robot.Print(string.Format("Open position with: LotsParameter: {0}, StopLossPipsParameter: {1}, TakeProfitPipsParameter: {2}", lots, stopLossPips, takeProfitPips));
var volume = _symbol.QuantityToVolumeInUnits(lots);
_robot.ExecuteMarketOrderAsync(tradeType, _symbol.Name, volume, "Trade Panel Sample", stopLossPips, takeProfitPips);
}
private double GetValueFromInput(string inputKey, double defaultValue)
{
double value;
return double.TryParse(_inputMap[inputKey].Text, out value) ? value : defaultValue;
}
private void CloseAll()
{
foreach (var position in _robot.Positions)
_robot.ClosePositionAsync(position);
}
}
public static class Styles
{
public static Style CreatePanelBackgroundStyle()
{
var style = new Style();
style.Set(ControlProperty.CornerRadius, 3);
style.Set(ControlProperty.BackgroundColor, GetColorWithOpacity(Color.FromHex("#292929"), 0.85m), ControlState.DarkTheme);
style.Set(ControlProperty.BackgroundColor, GetColorWithOpacity(Color.FromHex("#FFFFFF"), 0.85m), ControlState.LightTheme);
style.Set(ControlProperty.BorderColor, Color.FromHex("#3C3C3C"), ControlState.DarkTheme);
style.Set(ControlProperty.BorderColor, Color.FromHex("#C3C3C3"), ControlState.LightTheme);
style.Set(ControlProperty.BorderThickness, new Thickness(1));
return style;
}
public static Style CreateCommonBorderStyle()
{
var style = new Style();
style.Set(ControlProperty.BorderColor, GetColorWithOpacity(Color.FromHex("#FFFFFF"), 0.12m), ControlState.DarkTheme);
style.Set(ControlProperty.BorderColor, GetColorWithOpacity(Color.FromHex("#000000"), 0.12m), ControlState.LightTheme);
return style;
}
public static Style CreateHeaderStyle()
{
var style = new Style();
style.Set(ControlProperty.ForegroundColor, GetColorWithOpacity("#FFFFFF", 0.70m), ControlState.DarkTheme);
style.Set(ControlProperty.ForegroundColor, GetColorWithOpacity("#000000", 0.65m), ControlState.LightTheme);
return style;
}
public static Style CreateInputStyle()
{
var style = new Style(DefaultStyles.TextBoxStyle);
style.Set(ControlProperty.BackgroundColor, Color.FromHex("#1A1A1A"), ControlState.DarkTheme);
style.Set(ControlProperty.BackgroundColor, Color.FromHex("#111111"), ControlState.DarkTheme | ControlState.Hover);
style.Set(ControlProperty.BackgroundColor, Color.FromHex("#E7EBED"), ControlState.LightTheme);
style.Set(ControlProperty.BackgroundColor, Color.FromHex("#D6DADC"), ControlState.LightTheme | ControlState.Hover);
style.Set(ControlProperty.CornerRadius, 3);
return style;
}
public static Style CreateBuyButtonStyle()
{
return CreateButtonStyle(Color.FromHex("#009345"), Color.FromHex("#10A651"));
}
public static Style CreateSellButtonStyle()
{
return CreateButtonStyle(Color.FromHex("#F05824"), Color.FromHex("#FF6C36"));
}
public static Style CreateCloseButtonStyle()
{
return CreateButtonStyle(Color.FromHex("#F05824"), Color.FromHex("#FF6C36"));
}
private static Style CreateButtonStyle(Color color, Color hoverColor)
{
var style = new Style(DefaultStyles.ButtonStyle);
style.Set(ControlProperty.BackgroundColor, color, ControlState.DarkTheme);
style.Set(ControlProperty.BackgroundColor, color, ControlState.LightTheme);
style.Set(ControlProperty.BackgroundColor, hoverColor, ControlState.DarkTheme | ControlState.Hover);
style.Set(ControlProperty.BackgroundColor, hoverColor, ControlState.LightTheme | ControlState.Hover);
style.Set(ControlProperty.ForegroundColor, Color.FromHex("#FFFFFF"), ControlState.DarkTheme);
style.Set(ControlProperty.ForegroundColor, Color.FromHex("#FFFFFF"), ControlState.LightTheme);
return style;
}
private static Color GetColorWithOpacity(Color baseColor, decimal opacity)
{
var alpha = (int)Math.Round(byte.MaxValue * opacity, MidpointRounding.AwayFromZero);
return Color.FromArgb(alpha, baseColor);
}
}
}
@newbee
newbee
20 Oct 2023, 09:54
( Updated at: 21 Oct 2023, 06:57 )
RE: combobox.SelectedItemChanged
amusleh said:
Hi,
This works fine for me on 4.6.2 and .NET 6:
using System;using System.Collections.Generic;using System.Linq;using System.Text;using cAlgo.API;using cAlgo.API.Collections;using cAlgo.API.Indicators;using cAlgo.API.Internals;namespace cAlgo{ [Indicator(AccessRights = AccessRights.None)] public class ComboBoxTest : Indicator { private ComboBox myDropList; protected override void Initialize() { myDropList = new ComboBox { Width = 100, Height = 20, HorizontalContentAlignment = HorizontalAlignment.Center, VerticalContentAlignment = VerticalAlignment.Center }; myDropList.AddItem("Item 1"); myDropList.AddItem("Item 2"); myDropList.AddItem("Item 3"); Chart.AddControl(myDropList); myDropList.SelectedItemChanged += myDropList_SelectedIndexChanged; } private void myDropList_SelectedIndexChanged(ComboBoxSelectedItemChangedEventArgs e) { if (myDropList.SelectedIndex != -1) { string selectedValue = myDropList.SelectedItem.ToString(); switch (selectedValue) { case "Item 1": Print("Item 1 selected"); break; case "Item 2": Print("Item 2 selected"); break; case "Item 3": Print("Item 3 selected"); break; default: MessageBox.Show("The selected item was not recognized. Please contact the developer for assistance. Telegram: @DelFonseca"); break; } } else { MessageBox.Show("Please select an item from the dropdown list."); } } public override void Calculate(int index) { //... } }}
Check event signature before using it: SelectedItemChanged Event - cTrader Automate API
Hello again amusleh,
Further to my query earlier today please ignore it. I've actually got closer to working in out for myself. thank you anyway.
May be one general question please. with the trading panel can we make it Interactive instead of static. (like IsInteractive = true?)
thank you
@newbee
DelFonseca
21 Feb 2023, 15:46
up
@DelFonseca