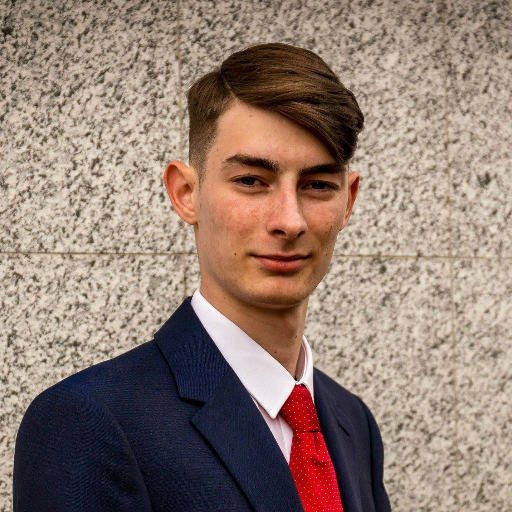
cAlgo - Moving average crossover problem
23 Jan 2023, 19:39
Hi guys,
I am currently using samcple trend cBot, however it makes one error quite often. Sometimes the market goes sideways and therefore both slow MA and fast MA are at same price for multiple minutes or candles which causes that cBot does not close current position and does not open reverse position so the current open position is still open and it goes against the trend for really long time till a next crossover happens. Can you help me fix it please? I am noob when it comes to programming. Code listed bellow. And example of a "bad trade" as well. (it was closed manually by me).
Screenshots of bad trade - You can see that aroun 15:55 both EMAs were at same price and it did not switch trade sides as it was supposed to do.
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class SampleTrendcBot : Robot
{
[Parameter("Quantity (Lots)", Group = "Volume", DefaultValue = 1, MinValue = 0.01, Step = 0.01)]
public double Quantity { get; set; }
[Parameter("MA Type", Group = "Moving Average")]
public MovingAverageType MAType { get; set; }
[Parameter("Source", Group = "Moving Average")]
public DataSeries SourceSeries { get; set; }
[Parameter("Slow Periods", Group = "Moving Average", DefaultValue = 10)]
public int SlowPeriods { get; set; }
[Parameter("Fast Periods", Group = "Moving Average", DefaultValue = 5)]
public int FastPeriods { get; set; }
private MovingAverage slowMa;
private MovingAverage fastMa;
private const string label = "Sample Trend cBot";
protected override void OnStart()
{
fastMa = Indicators.MovingAverage(SourceSeries, FastPeriods, MAType);
slowMa = Indicators.MovingAverage(SourceSeries, SlowPeriods, MAType);
}
protected override void OnTick()
{
var longPosition = Positions.Find(label, SymbolName, TradeType.Buy);
var shortPosition = Positions.Find(label, SymbolName, TradeType.Sell);
var currentSlowMa = slowMa.Result.Last(0);
var currentFastMa = fastMa.Result.Last(0);
var previousSlowMa = slowMa.Result.Last(1);
var previousFastMa = fastMa.Result.Last(1);
if (previousSlowMa > previousFastMa && currentSlowMa <= currentFastMa && longPosition == null)
{
if (shortPosition != null)
ClosePosition(shortPosition);
ExecuteMarketOrder(TradeType.Buy, SymbolName, VolumeInUnits, label);
}
else if (previousSlowMa < previousFastMa && currentSlowMa >= currentFastMa && shortPosition == null)
{
if (longPosition != null)
ClosePosition(longPosition);
ExecuteMarketOrder(TradeType.Sell, SymbolName, VolumeInUnits, label);
}
}
private double VolumeInUnits
{
get { return Symbol.QuantityToVolumeInUnits(Quantity); }
}
}
}
Replies
martin-stejsky
25 Jan 2023, 11:45
RE:
Sure.
It runs on 5 minutes chart, MA type - exponential, Source - close, Slow period - 30, Fast period - 10.
Thank you :)
@martin-stejsky
PanagiotisChar
26 Jan 2023, 09:42
( Updated at: 21 Dec 2023, 09:23 )
Hi there,
Seems to work fine on backtesting
Need help? Join us on Telegram
Need premium support? Trade with us
@PanagiotisChar
martin-stejsky
26 Jan 2023, 10:40
RE:
That might be true, however it happens quite often I would say. I am currently testing it on demo account to find out which pairs work in forward test and which dont so I am currently testing it on two platform IC Markets and FTMO and it happens on both platforms.
I test 18 different pairs and it opens like 80 trades a day and this bug happens like 3 times in the day.
The weird thing is that sometimes is opens a oposite possition even when I can see that the two MAs indicators havent crossed. So either there is a problem in code which needs and actual "crossover" function or in cTrader platform as other platforms I use dont have this problem.
@martin-stejsky
PanagiotisChar
26 Jan 2023, 10:49
Hi there,
I believe you, it's just hard to help you if I cannot reproduce the problem.
Need help? Join us on Telegram
Need premium support? Trade with us
@PanagiotisChar
PanagiotisChar
24 Jan 2023, 14:43
Hi there,
Can you provide cBot parameters to reproduce this on backtesting?
Aieden Technologies
Need help? Join us on Telegram
Need premium support? Trade with us
@PanagiotisChar