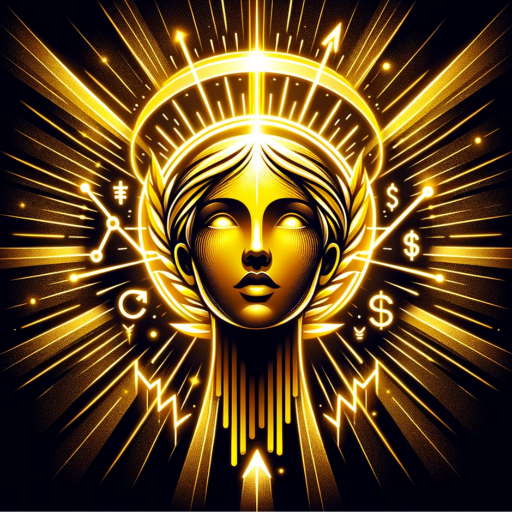
if (PositionBuy == null) doesn't work
13 Aug 2021, 22:11
I have a problem of
var b1 = Positions.Find("b1");
var s1 = Positions.Find("s1");
not finding anything and being permanently null. Whereas there are open positions, here's my simple bot below:
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class NewcBot : Robot
{
[Parameter("Source")]
public DataSeries SourceSeries { get; set; }
private RelativeStrengthIndex _rsi;
protected override void OnStart()
{
_rsi = Indicators.RelativeStrengthIndex(SourceSeries, 14);
}
protected override void OnTick()
{
var b1 = Positions.Find("b1");
var s1 = Positions.Find("s1");
double rsi = _rsi.Result.Last(0);
if (b1 == null && s1 == null && rsi < 30)
{
ExecuteMarketOrder(TradeType.Buy, Symbol, 10000, "b1", 25, 35);
}
if (b1 == null && s1 == null && rsi < 30)
{
ExecuteMarketOrder(TradeType.Sell, Symbol, 10000, "s1", 25, 35);
}
}
protected override void OnStop()
{
// Put your deinitialization logic here
}
}
}
So here the SELL position is not supposed to be open while BUY is opened, and vice versa. But since 'b1' and 's1' are always 'null' the bot doesn't behave as expected...
PanagiotisCharalampous
16 Aug 2021, 08:47
Hi mywebsidekicks,
This happens because you get the positions before you open a buy position. See below a better way to do this.
Best Regards,
Panagiotis
Join us on Telegram and Facebook
@PanagiotisCharalampous