How to debug specific parts of the code when it doesn't show any error?
Created at 18 Jul 2021, 02:11
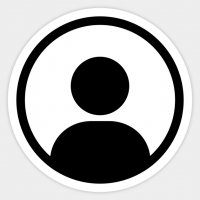
How to debug specific parts of the code when it doesn't show any error?
18 Jul 2021, 02:11
The following code for example doesn't display correctly in the charts. There isn't any errors in the "build result" or in the "log" section.
How do we realize where is the error?
Thanks
using cAlgo.API; namespace cAlgo { // This sample shows how to use Symbols collection to get symbols data [Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class SymbolsSample : Indicator { protected override void Initialize() { var scrollViewer = new ScrollViewer { HorizontalAlignment = HorizontalAlignment.Center, VerticalAlignment = VerticalAlignment.Center, BackgroundColor = Color.Gold, Opacity = 0.7, HorizontalScrollBarVisibility = ScrollBarVisibility.Auto, VerticalScrollBarVisibility = ScrollBarVisibility.Visible, Height = 300 }; var grid = new Grid(Symbols.Count + 1, 2) { BackgroundColor = Color.Gold, HorizontalAlignment = HorizontalAlignment.Center, VerticalAlignment = VerticalAlignment.Center, }; scrollViewer.Content = grid; grid.AddChild(new TextBlock { Text = "Name", Margin = 5, ForegroundColor = Color.Black, FontWeight = FontWeight.ExtraBold }, 0, 0); grid.AddChild(new TextBlock { Text = "Description", Margin = 5, ForegroundColor = Color.Black, FontWeight = FontWeight.ExtraBold }, 0, 1); for (int iSymbol = 1; iSymbol < Symbols.Count + 1; iSymbol++) { var symbolName = Symbols[iSymbol]; var symbol = Symbols.GetSymbol(symbolName); if (!symbol.MarketHours.IsOpened()) continue; grid.AddChild(new TextBlock { Text = symbolName, Margin = 5, ForegroundColor = Color.Black, FontWeight = FontWeight.ExtraBold }, iSymbol, 0); grid.AddChild(new Button { Text = symbol.Description, Margin = 5, ForegroundColor = Color.Black, FontWeight = FontWeight.ExtraBold }, iSymbol, 1); } Chart.AddControl(scrollViewer); } public override void Calculate(int index) { } } }
amusleh
19 Jul 2021, 09:22 ( Updated at: 19 Jul 2021, 09:31 )
Hi,
You can use Print method to check if the indicator thread execution flow reaches the Chart.AddControl(scrollViewer) line or not, if it desn't reach the line and stuck somewhere then the scroll viewer control will not be displayed on your chart.
There is no issue with indicator code, it just gets some time to iterate over all available symbols of your broker, if you limit the number of symbols to 50 by using a break it will come up faster:
@amusleh