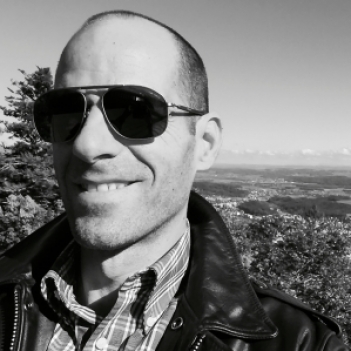
Deserialize JSON string
27 Jun 2021, 12:55
I want to deserialize this JSON response
{
success: true,
data: {
id: 14,
orderId: 21,
productId: 20,
userId: 1,
licenseKey: "RS-KH-0G-6B-FD-A7-CS-W7",
expiresAt: "2021-07-26 00:00:00",
validFor: null,
source: 1,
status: 3,
timesActivated: null,
timesActivatedMax: 1,
createdAt: "2021-06-26 11:36:46",
createdBy: 1,
updatedAt: "2021-06-26 15:25:54",
updatedBy: 1
}
}
into this object:
public class Licence
{
public class Root
{
public string success { get; set; }
public Data data { get; set; }
}
public class Data
{
public int id{ get; set; }
public int orderId { get; set; }
public int productId { get; set; }
public int userId { get; set; }
public string licenseKey { get; set; }
public string expiresAt { get; set; }
public int validFor { get; set; }
public int source { get; set; }
public int status { get; set; }
public int timesActivated { get; set; }
public int timesActivatedMax { get; set; }
public string createdAt { get; set; }
public int createdBy { get; set; }
public string updatedAt { get; set; }
public int updatedBy { get; set; }
public string name { get; set; }
public string address { get; set; }
public string city { get; set; }
public string state { get; set; }
public string zip { get; set; }
}
}
Replies
amusleh
28 Jun 2021, 11:46
Hi,
Your question is not related to cTrader Automate API, you have to Google these types of questions or post your question on Stackoverflow.
Your object and Json have invalid format, try this:
using cAlgo.API;
using Newtonsoft.Json;
using System;
namespace cAlgo
{
[Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)]
public class JsonDeserializeTest : Indicator
{
private string _json = @"{
success: true,
data:
{
id: 14,
orderId: 21,
productId: 20,
userId: 1,
licenseKey: 'RS-KH-0G-6B-FD-A7-CS-W7',
expiresAt: '2021-07-26 00:00:00'
}
}";
protected override void Initialize()
{
var licence = JsonConvert.DeserializeObject<Licence>(_json);
Print(licence.Data.ExpiresAt);
}
public override void Calculate(int index)
{
}
}
public class Licence
{
public string Success { get; set; }
public Data Data { get; set; }
}
public class Data
{
public int Id { get; set; }
public int OrderId { get; set; }
public int ProductId { get; set; }
public int UserId { get; set; }
public string LicenseKey { get; set; }
public DateTime ExpiresAt { get; set; }
}
}
It works fine on my system, I removed some of your data object properties for brevity.
@amusleh
sifneos4fx
30 Jun 2021, 10:43
RE:
Thank you for your Answer. Indeed, both the JSON and the Object were not in a valid format. This is how I implement the classes:
public partial class License
{
[JsonProperty("success")]
public bool Success { get; set; }
[JsonProperty("data")]
public Data Data { get; set; }
}
public partial class Data
{
[JsonProperty("id")]
public long Id { get; set; }
[JsonProperty("orderId")]
public long OrderId { get; set; }
[JsonProperty("productId")]
public long ProductId { get; set; }
[JsonProperty("userId")]
public long UserId { get; set; }
[JsonProperty("licenseKey")]
public string LicenseKey { get; set; }
[JsonProperty("expiresAt")]
public DateTimeOffset ExpiresAt { get; set; }
[JsonProperty("validFor")]
public object ValidFor { get; set; }
[JsonProperty("source")]
public long Source { get; set; }
[JsonProperty("status")]
public long Status { get; set; }
[JsonProperty("timesActivated")]
public object TimesActivated { get; set; }
[JsonProperty("timesActivatedMax")]
public long TimesActivatedMax { get; set; }
[JsonProperty("createdAt")]
public DateTimeOffset CreatedAt { get; set; }
[JsonProperty("createdBy")]
public long CreatedBy { get; set; }
[JsonProperty("updatedAt")]
public DateTimeOffset UpdatedAt { get; set; }
[JsonProperty("updatedBy")]
public long UpdatedBy { get; set; }
}
Thank you once again!
amusleh said:
Hi,
Your question is not related to cTrader Automate API, you have to Google these types of questions or post your question on Stackoverflow.
Your object and Json have invalid format, try this:
using cAlgo.API; using Newtonsoft.Json; using System; namespace cAlgo { [Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)] public class JsonDeserializeTest : Indicator { private string _json = @"{ success: true, data: { id: 14, orderId: 21, productId: 20, userId: 1, licenseKey: 'RS-KH-0G-6B-FD-A7-CS-W7', expiresAt: '2021-07-26 00:00:00' } }"; protected override void Initialize() { var licence = JsonConvert.DeserializeObject<Licence>(_json); Print(licence.Data.ExpiresAt); } public override void Calculate(int index) { } } public class Licence { public string Success { get; set; } public Data Data { get; set; } } public class Data { public int Id { get; set; } public int OrderId { get; set; } public int ProductId { get; set; } public int UserId { get; set; } public string LicenseKey { get; set; } public DateTime ExpiresAt { get; set; } } }
It works fine on my system, I removed some of your data object properties for brevity.
@sifneos4fx
sifneos4fx
28 Jun 2021, 09:59 ( Updated at: 28 Jun 2021, 10:14 )
This is what I want to use:
To do so, I'am referencing to the Newtonsoft.Json.dll -> https://www.nuget.org/packages/Newtonsoft.Json/
The namespace using Newtonsoft.Json; is now recognized, however, when building the project, i get the error "unable to load assembly", however, the bot works, but the "licence" object remains null.
@sifneos4fx