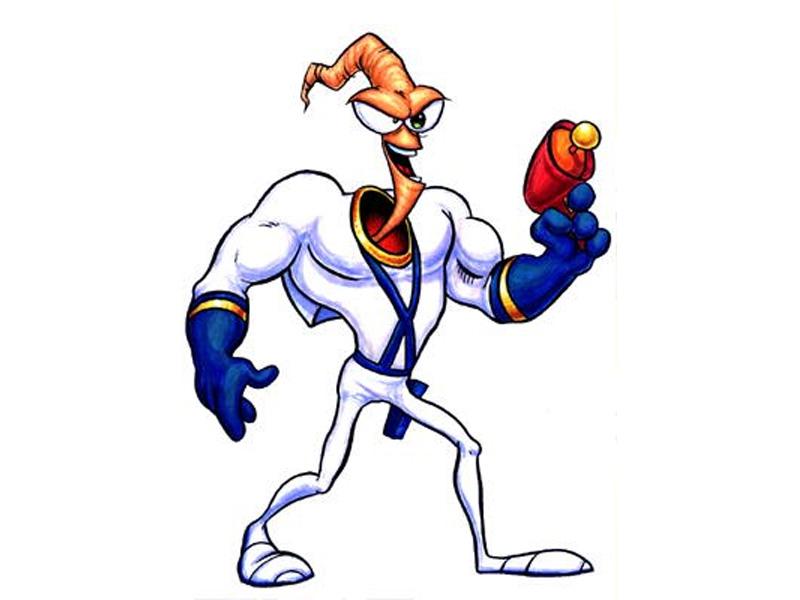
Heiken Ashi
26 Nov 2017, 19:27
Hey guys.
Could anyone help me converting an indicator into a cBot, please?
using System; using cAlgo.API; using cAlgo.API.Indicators; namespace cAlgo.Indicators { [Indicator(IsOverlay = true, AccessRights = AccessRights.None)] public class HeikenAshi : Indicator { private IndicatorDataSeries _haOpen; private IndicatorDataSeries _haClose; [Parameter("Candle width", DefaultValue = 5)] public int CandleWidth { get; set; } [Parameter("Up color", DefaultValue = "Blue")] public string UpColor { get; set; } [Parameter("Down color", DefaultValue = "Red")] public string DownColor { get; set; } private Colors _upColor; private Colors _downColor; private bool _incorrectColors; private Random _random = new Random(); protected override void Initialize() { _haOpen = CreateDataSeries(); _haClose = CreateDataSeries(); if (!Enum.TryParse<Colors>(UpColor, out _upColor) || !Enum.TryParse<Colors>(DownColor, out _downColor)) _incorrectColors = true; } public override void Calculate(int index) { if (_incorrectColors) { var errorColor = _random.Next(2) == 0 ? Colors.Red : Colors.White; ChartObjects.DrawText("Error", "Incorrect colors", StaticPosition.Center, errorColor); return; } var open = MarketSeries.Open[index]; var high = MarketSeries.High[index]; var low = MarketSeries.Low[index]; var close = MarketSeries.Close[index]; var haClose = (open + high + low + close) / 4; double haOpen; if (index > 0) haOpen = (_haOpen[index - 1] + _haClose[index - 1]) / 2; else haOpen = (open + close) / 2; var haHigh = Math.Max(Math.Max(high, haOpen), haClose); var haLow = Math.Min(Math.Min(low, haOpen), haClose); var color = haOpen > haClose ? _downColor : _upColor; ChartObjects.DrawLine("candle" + index, index, haOpen, index, haClose, color, CandleWidth, LineStyle.Solid); ChartObjects.DrawLine("line" + index, index, haHigh, index, haLow, color, 1, LineStyle.Solid); _haOpen[index] = haOpen; _haClose[index] = haClose; } } }
I'm creating a bot where I would like to use Heiken Ashi data, so I need the following variables to be calculated ina cbot.
haOpen
haClose
haHigh
haLow
I've tried to do this myself but it seems that I'm doing something wrong. I'm a bit confused with [index -1] too, it doesn;t work in the cbot format it seems.
So would be VERY MUCH appreciated if someone could help.
Thanks! :)
Replies
irmscher9
27 Nov 2017, 19:01
Ok, I maanged to put it together myself through "onbar":
protected override void OnBar() { var open2 = MarketSeries.Open.Last(2); var high2 = MarketSeries.High.Last(2); var low2 = MarketSeries.Low.Last(2); var close2 = MarketSeries.Close.Last(2); var open1 = MarketSeries.Open.Last(1); var high1 = MarketSeries.High.Last(1); var low1 = MarketSeries.Low.Last(1); var close1 = MarketSeries.Close.Last(1); haClose1 = (open2 + high2 + low2 + close2) / 4; haClose = (open1 + high1 + low1 + close1) / 4; if (haOpen > 0) { haOpen = (haOpen + haClose1) / 2; } if (haOpen == 0) { haOpen = (open1 + close1) / 2; } haHigh = Math.Max(Math.Max(high1, haOpen), haClose); haLow = Math.Min(Math.Min(low1, haOpen), haClose); }
@irmscher9
nguyendan81985
02 Dec 2018, 08:28
RE:
irmscher9 said:
Ok, I maanged to put it together myself through "onbar":
protected override void OnBar() { var open2 = MarketSeries.Open.Last(2); var high2 = MarketSeries.High.Last(2); var low2 = MarketSeries.Low.Last(2); var close2 = MarketSeries.Close.Last(2); var open1 = MarketSeries.Open.Last(1); var high1 = MarketSeries.High.Last(1); var low1 = MarketSeries.Low.Last(1); var close1 = MarketSeries.Close.Last(1); haClose1 = (open2 + high2 + low2 + close2) / 4; haClose = (open1 + high1 + low1 + close1) / 4; if (haOpen > 0) { haOpen = (haOpen + haClose1) / 2; } if (haOpen == 0) { haOpen = (open1 + close1) / 2; } haHigh = Math.Max(Math.Max(high1, haOpen), haClose); haLow = Math.Min(Math.Min(low1, haOpen), haClose); }hi,
i copied your code, but there is error: "Error CS0103: The name 'haOpen' does not exist in the current context"
did you fix it?
@nguyendan81985
irmscher9
27 Nov 2017, 14:11
I'm actually OK with everything except for [index - 1]
So I'm a bit confused on how to get values of haOpen and haClose of the previous period.
[index - 1] doesn;t work in cbot, only in indicators.
@irmscher9