Time Series Moving Average
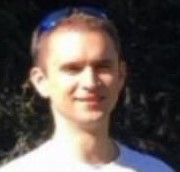
Time Series Moving Average
27 Feb 2017, 00:59
Can somebody point me to the algorithm (or better, C# code) used to calculate Time Series Moving Average? I have been searching but I'm not sure how cAlgo calculates this.
Thanks!
Replies
jaredthirsk
27 Feb 2017, 09:35
RE:
tmc. said:
Hi, Time Series Moving Average is equal to Linear Regression Forecast. The cAlgo platform calculates it by multiplying Linear Regression Slope with Period plus Linear Regression Intercept. Here's is better solution that gives you same result.
Awesome, thanks!
Vidya is the other moving average I was wondering about. Do you know the best way to calculate that?
@jaredthirsk
whis.gg
27 Feb 2017, 12:19
Not sure if it's the best way but it's the one cAlgo uses.
using System; using cAlgo.API; namespace cAlgo { [Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class Vidya : Indicator { [Parameter()] public DataSeries Source { get; set; } [Parameter("Period", DefaultValue = 14)] public int Period { get; set; } [Parameter("Sigma", DefaultValue = 0.65, MinValue = 0.1, MaxValue = 0.95)] public double Sigma { get; set; } [Output("Main")] public IndicatorDataSeries Result { get; set; } public override void Calculate(int index) { double num = this.Result[index - 1]; if (double.IsNaN(num)) { num = Source[index - 1]; } double num2 = Sigma * Cmo(index); Result[index] = (1.0 - num2) * num + num2 * Source[index]; } private double Cmo(int index) { double num = 0.0; double num2 = 0.0; for (int i = 0; i < Period; i++) { double num3 = Source[index - i] - Source[index - i - 1]; if (num3 > 0.0) { num += num3; } else { num2 += -num3; } } if (num + num2 == 0.0) { return 0.0; } return Math.Abs((num - num2) / (num + num2)); } } }
@whis.gg
whis.gg
27 Feb 2017, 02:34
Hi, Time Series Moving Average is equal to Linear Regression Forecast. The cAlgo platform calculates it by multiplying Linear Regression Slope with Period plus Linear Regression Intercept. Here's is better solution that gives you same result.
@whis.gg