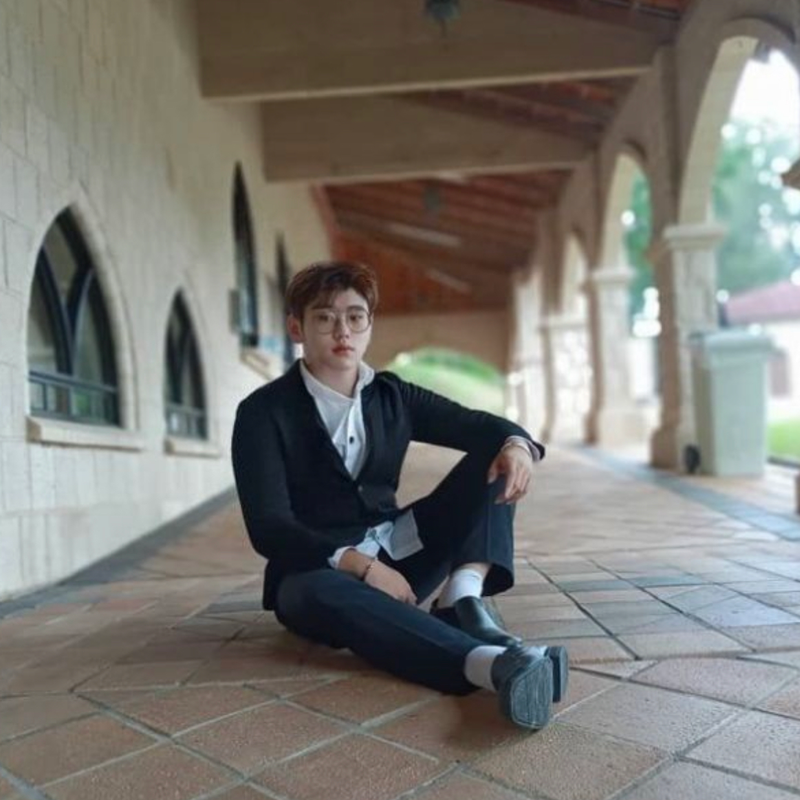
Replies
bryantan1012
23 Oct 2024, 12:16
( Updated at: 24 Oct 2024, 05:11 )
RE: my cbot create but cant run,is there any problem
PanagiotisCharalampous said:
Hi there,
Please provide the code in text format so that we can copy and paste it, and let us know what the actual problem is.
Best regards,
Panagiotis
using cAlgo.API;
using cAlgo.API.Indicators;
using System;
using System.Linq;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class GeekTraderV2 : Robot
{
[Parameter("风险百分比 (%)", DefaultValue = 1, MinValue = 0.1, MaxValue = 10, Step = 0.1)]
public double RiskPercentage { get; set; }
[Parameter("ADX 趋势阈值", DefaultValue = 25, MinValue = 1, MaxValue = 100)]
public int AdxTrendThreshold { get; set; }
[Parameter("止损 (点)", DefaultValue = 10, MinValue = 1, Step = 1)]
public int StopLoss { get; set; }
[Parameter("止盈 (点)", DefaultValue = 20, MinValue = 1, Step = 1)]
public int TakeProfit { get; set; }
private ExponentialMovingAverage ema200;
private AverageDirectionalMovementIndex adx;
protected override void OnStart()
{
ema200 = Indicators.ExponentialMovingAverage(Bars.ClosePrices, 200);
adx = Indicators.AverageDirectionalMovementIndex(14);
// GeekTrader V2 已启动,开始自动交易!
Print("GeekTrader V2 已启动,开始自动交易!");
}
protected override void OnBar()
{
// 1. 确定趋势方向
var trend = GetTrend();
// 2. 找到支撑位和阻力位
var (support, resistance) = GetSupportResistance();
// 3. 在支撑位或阻力位附近进行交易
if (trend == TradeType.Buy && Symbol.Bid <= support && adx.ADX.LastValue > AdxTrendThreshold)
{
var volume = CalculateVolume(Symbol.Bid, support);
ExecuteMarketOrder(TradeType.Buy, SymbolName, volume, "Long", StopLoss, TakeProfit);
}
else if (trend == TradeType.Sell && Symbol.Ask >= resistance && adx.ADX.LastValue > AdxTrendThreshold)
{
var volume = CalculateVolume(Symbol.Ask, resistance);
ExecuteMarketOrder(TradeType.Sell, SymbolName, volume, "Short", StopLoss, TakeProfit);
}
}
private TradeType GetTrend()
{
// 使用 EMA200 判断趋势方向
if (Bars.ClosePrices.Last(1) > ema200.Result.Last(1))
return TradeType.Buy;
else if (Bars.ClosePrices.Last(1) < ema200.Result.Last(1))
return TradeType.Sell;
else
return TradeType.None; // 注意这里,使用 TradeType.None 表示无趋势
}
private (double, double) GetSupportResistance()
{
// 使用最近的 swing high 和 swing low 作为阻力位和支撑位
var swingHigh = Bars.HighPrices.Last(100).Max();
var swingLow = Bars.LowPrices.Last(100).Min();
return (swingLow, swingHigh);
}
private double CalculateVolume(double currentPrice, double stopLossPrice)
{
// 计算止损点数
var stopLossPips = Math.Abs(currentPrice - stopLossPrice) / Symbol.PipSize;
// 计算最大允许风险金额
var riskAmount = Account.Balance * RiskPercentage / 100;
// 计算交易量 (手)
var volume = riskAmount / (stopLossPips * Symbol.PipValue);
// 限制最大交易量为 0.01 手
volume = Math.Min(volume, 0.01);
return volume;
}
}
}
@bryantan1012
firemyst
24 Oct 2024, 01:22
Just glancing at your code, I noticed one way to improve it.
The “GetTrend()” method is a waste of CPU cycles. If the trend doesn't match a “sell”, it's always going to return a “buy”. If this is actually what you want, then redo the code like follows to remove a conditional check:
private TradeType GetTrend()
{
//Remove the first conditional check to save on CPU cycles and make your bot code
//that few nano-seconds faster
if (Bars.ClosePrices.Last(1) < ema200.Result.Last(1))
return TradeType.Sell;
else
return TradeType.Buy;
}
@firemyst
PanagiotisCharalampous
24 Oct 2024, 05:28
RE: RE: my cbot create but cant run,is there any problem
bryantan1012 said:
PanagiotisCharalampous said:
Hi there,
Please provide the code in text format so that we can copy and paste it, and let us know what the actual problem is.
Best regards,
Panagiotis
using cAlgo.API;
using cAlgo.API.Indicators;
using System;
using System.Linq;namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class GeekTraderV2 : Robot
{
[Parameter("风险百分比 (%)", DefaultValue = 1, MinValue = 0.1, MaxValue = 10, Step = 0.1)]
public double RiskPercentage { get; set; }[Parameter("ADX 趋势阈值", DefaultValue = 25, MinValue = 1, MaxValue = 100)]
public int AdxTrendThreshold { get; set; }[Parameter("止损 (点)", DefaultValue = 10, MinValue = 1, Step = 1)]
public int StopLoss { get; set; }[Parameter("止盈 (点)", DefaultValue = 20, MinValue = 1, Step = 1)]
public int TakeProfit { get; set; }private ExponentialMovingAverage ema200;
private AverageDirectionalMovementIndex adx;protected override void OnStart()
{
ema200 = Indicators.ExponentialMovingAverage(Bars.ClosePrices, 200);
adx = Indicators.AverageDirectionalMovementIndex(14);// GeekTrader V2 已启动,开始自动交易!
Print("GeekTrader V2 已启动,开始自动交易!");
}protected override void OnBar()
{
// 1. 确定趋势方向
var trend = GetTrend();// 2. 找到支撑位和阻力位
var (support, resistance) = GetSupportResistance();// 3. 在支撑位或阻力位附近进行交易
if (trend == TradeType.Buy && Symbol.Bid <= support && adx.ADX.LastValue > AdxTrendThreshold)
{
var volume = CalculateVolume(Symbol.Bid, support);
ExecuteMarketOrder(TradeType.Buy, SymbolName, volume, "Long", StopLoss, TakeProfit);
}
else if (trend == TradeType.Sell && Symbol.Ask >= resistance && adx.ADX.LastValue > AdxTrendThreshold)
{
var volume = CalculateVolume(Symbol.Ask, resistance);
ExecuteMarketOrder(TradeType.Sell, SymbolName, volume, "Short", StopLoss, TakeProfit);
}
}private TradeType GetTrend()
{
// 使用 EMA200 判断趋势方向
if (Bars.ClosePrices.Last(1) > ema200.Result.Last(1))
return TradeType.Buy;
else if (Bars.ClosePrices.Last(1) < ema200.Result.Last(1))
return TradeType.Sell;
else
return TradeType.None; // 注意这里,使用 TradeType.None 表示无趋势
}private (double, double) GetSupportResistance()
{
// 使用最近的 swing high 和 swing low 作为阻力位和支撑位
var swingHigh = Bars.HighPrices.Last(100).Max();
var swingLow = Bars.LowPrices.Last(100).Min();return (swingLow, swingHigh);
}private double CalculateVolume(double currentPrice, double stopLossPrice)
{
// 计算止损点数
var stopLossPips = Math.Abs(currentPrice - stopLossPrice) / Symbol.PipSize;// 计算最大允许风险金额
var riskAmount = Account.Balance * RiskPercentage / 100;// 计算交易量 (手)
var volume = riskAmount / (stopLossPips * Symbol.PipValue);// 限制最大交易量为 0.01 手
volume = Math.Min(volume, 0.01);return volume;
}
}
}
There is a lot on nonsense in this code. Was it generated by ChatGPT?
@PanagiotisCharalampous
PanagiotisCharalampous
23 Oct 2024, 09:11
Hi there,
Please provide the code in text format so that we can copy and paste it, and let us know what the actual problem is.
Best regards,
Panagiotis
@PanagiotisCharalampous