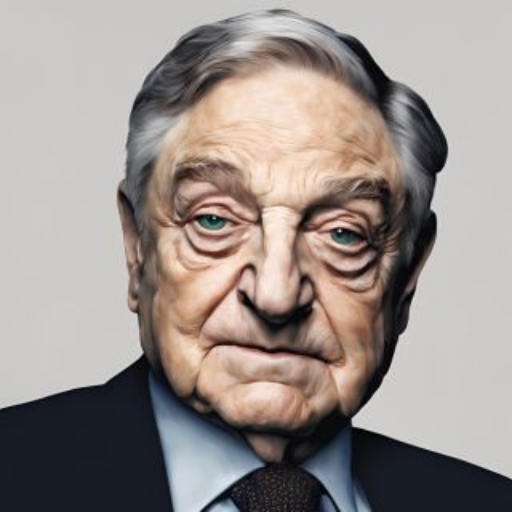
Arbitrage bot
09 Sep 2024, 18:44
GM
i build an indicator to show the Theoretical price of EURJPY on the chart to get a possible Arbitrage if there is an imbalance of the real price. it calclates with the EURUSD and USDJPY Price in realtime from cTrader broker data.
It behaves like a third price Line
____________
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using System;
namespace cAlgo.Indicators
{
[Indicator(AccessRights = AccessRights.None)]
public class Arbitrage_Theoretical_Price_EURJPY : Indicator
{
private Symbol eurusd;
private Symbol usdjpy;
private Symbol eurjpy;
[Output("Theoretical Price", LineColor = "Blue")]
public IndicatorDataSeries TheoreticalPrice { get; set; }
protected override void Initialize()
{
eurusd = Symbols.GetSymbol("EURUSD");
usdjpy = Symbols.GetSymbol("USDJPY");
eurjpy = Symbol; // Automatically fetch the EURJPY symbol for the current chart
}
public override void Calculate(int index)
{
double eurusdBid = eurusd.Bid;
double usdjpyBid = usdjpy.Bid;
double eurusdAsk = eurusd.Ask;
double usdjpyAsk = usdjpy.Ask;
double theoreticalBidPrice = eurusdBid * usdjpyBid;
double theoreticalAskPrice = eurusdAsk * usdjpyAsk;
double theoreticalPrice = (theoreticalBidPrice + theoreticalAskPrice) / 2;
for (int i = 0; i <= index; i++)
{
TheoreticalPrice[i] = theoreticalPrice;
}
}
}
}
___________
Now i want to automatically arbitrage it if the price is above or below from the ask or bid price by 0,025% to open a Long or short and setting a Target at the Theoretical price line. Now my problem is that the bot can't dfind it trough the name and i dont know how to code the bot for my plan. the following code is below:
______
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using System;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class ArbitrageBot : Robot
{
private Arbitrage_Theoretical_Price_EURJPY theoreticalPriceIndicator;
private Symbol eurjpy;
private const double ThresholdPercentage = 0.025; // 0.025%
protected override void OnStart()
{
// Initialize the symbol
eurjpy = Symbol; // Automatically fetch the EURJPY symbol for the current chart
// Initialize the theoretical price indicator
theoreticalPriceIndicator = Indicators.GetIndicator<Arbitrage_Theoretical_Price_EURJPY>();
}
protected override void OnTick()
{
// Get the current Bid and Ask prices of EURJPY
double currentBidPrice = eurjpy.Bid;
double currentAskPrice = eurjpy.Ask;
// Get the theoretical price from the indicator
double theoreticalPrice = theoreticalPriceIndicator.TheoreticalPrice.Last(1);
// Calculate the percentage difference between theoretical and actual prices
double bidDifference = (theoreticalPrice - currentBidPrice) / currentBidPrice * 100;
double askDifference = (currentAskPrice - theoreticalPrice) / theoreticalPrice * 100;
// Check if the differences exceed the threshold
if (bidDifference >= ThresholdPercentage)
{
// Open a long position (buy) since the theoretical price is lower than the current bid price
var positionSize = 1; // Define your position size here
var stopLoss = currentBidPrice - (currentBidPrice * 0.01); // Example stop loss (1% away)
var takeProfit = theoreticalPrice; // Set the theoretical price as the target
ExecuteMarketOrder(TradeType.Buy, eurjpy.Name, positionSize, "ArbitrageBot", stopLoss, takeProfit);
}
else if (askDifference >= ThresholdPercentage)
{
// Open a short position (sell) since the theoretical price is higher than the current ask price
var positionSize = 1; // Define your position size here
var stopLoss = currentAskPrice + (currentAskPrice * 0.01); // Example stop loss (1% away)
var takeProfit = theoreticalPrice; // Set the theoretical price as the target
ExecuteMarketOrder(TradeType.Sell, eurjpy.Name, positionSize, "ArbitrageBot", stopLoss, takeProfit);
}
}
}
}
_______
here is the error code that i get when i want to compile the bot

Kompilieren Fehlgeschlagen (2 Fehler)
Fehler CS0246: Der Typ oder der Namespace-Name 'Arbitrage_Theoretical_Price_EURJPY' konnte nicht gefunden werden (Fehlt eine using-Direktive oder eine Assembly-Referenz?)
Dateipfad: C:\Users\fufu\Documents\cAlgo\Sources\Robots\Arbitrage_EURJPYBot\ArbitrageTheoreticalPriceEURJPYBot\ArbitrageTheoreticalPriceEURJPYBot.cs
Zeile: 11, Spalte: 17
Fehler CS0246: Der Typ oder der Namespace-Name 'Arbitrage_Theoretical_Price_EURJPY' konnte nicht gefunden werden (Fehlt eine using-Direktive oder eine Assembly-Referenz?)
Dateipfad: C:\Users\fufu\Documents\cAlgo\Sources\Robots\ArbitrageTheoreticalPrice_EURJPYBot\ArbitrageTheoreticalPriceEURJPYBot\ArbitrageTheoreticalPriceEURJPYBot.cs
Zeile: 22, Spalte: 65
_______
I also want to set also setting a Stop loss at 0,03% from the entry point by this bot.
Can somebody help me to code this bot for my indicator or help me to get the bot run with the indicator, so i can backtest it?
Thanks in Advance!
King Regards
firemyst
09 Sep 2024, 23:47 ( Updated at: 10 Sep 2024, 05:12 )
Add the reference into your cbot project:
https://help.ctrader.com/ctrader-algo/articles/for-developers/how-to-use-custom-indicators-in-cbots/
@firemyst