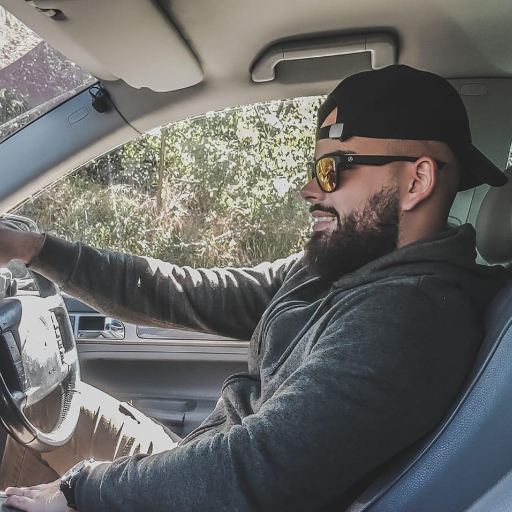
The bot does not set a tp as it should every time it opens a position
09 Oct 2023, 14:56
I have this code of a robot that works perfectly fine, but the only issue is that it doesn't set a tp on the position it opens:
using System;
using cAlgo.API;
namespace CandlestickBot
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)]
public class CandlestickBot : Robot
{
[Parameter("Lot Size", DefaultValue = 10000, MinValue = 1)]
public int LotSize { get; set; }
[Parameter("Take Profit (pips)", DefaultValue = 20, MinValue = 1)]
public int TakeProfitPips { get; set; } // Changed parameter name to avoid conflict
private int _bullishCount;
private int _bearishCount;
private PositionState _positionState;
private double _lastClose;
private double _lastOpen;
protected override void OnStart()
{
_bullishCount = 0;
_bearishCount = 0;
_positionState = PositionState.Flat;
}
protected override void OnTick()
{
if (_positionState == PositionState.Long &&
(_lastClose < _lastOpen || _lastClose < _lastOpen))
{
ClosePosition();
_positionState = PositionState.Flat;
}
else if (_positionState == PositionState.Short &&
(_lastClose > _lastOpen || _lastClose > _lastOpen))
{
ClosePosition();
_positionState = PositionState.Flat;
}
}
protected override void OnBar()
{
var currentBar = Bars.Last(1);
var previousBar = Bars.Last(2);
_lastClose = currentBar.Close;
_lastOpen = currentBar.Open;
double takeProfit = TakeProfitPips * Symbol.PipSize; // Calculate TP in price units
double lotSize = (double)LotSize;
if (_lastClose > _lastOpen && currentBar.Close > previousBar.Close)
{
_bullishCount++;
if (_bullishCount >= 2 && _positionState == PositionState.Flat)
{
var takeProfitPrice = Symbol.Bid + takeProfit;
ExecuteMarketOrder(TradeType.Buy, SymbolName, lotSize, "Buy Order", 0, takeProfitPrice, null);
_positionState = PositionState.Long;
}
}
else if (_lastClose < _lastOpen && currentBar.Close < previousBar.Close)
{
_bearishCount++;
if (_bearishCount >= 2 && _positionState == PositionState.Flat)
{
var takeProfitPrice = Symbol.Ask - takeProfit;
ExecuteMarketOrder(TradeType.Sell, SymbolName, lotSize, "Sell Order", 0, takeProfitPrice, null);
_positionState = PositionState.Short;
}
}
else
{
_bullishCount = 0;
_bearishCount = 0;
}
}
private void ClosePosition()
{
foreach (var position in Positions)
{
ClosePosition(position);
}
}
private enum PositionState
{
Flat,
Long,
Short
}
}
}
PanagiotisChar
11 Oct 2023, 06:39 ( Updated at: 11 Oct 2023, 07:00 )
Hi there,
The Take Profit parameter is set in pips but you are setting in in price units
@PanagiotisChar