How to Make CBot Stop and get back on the next day
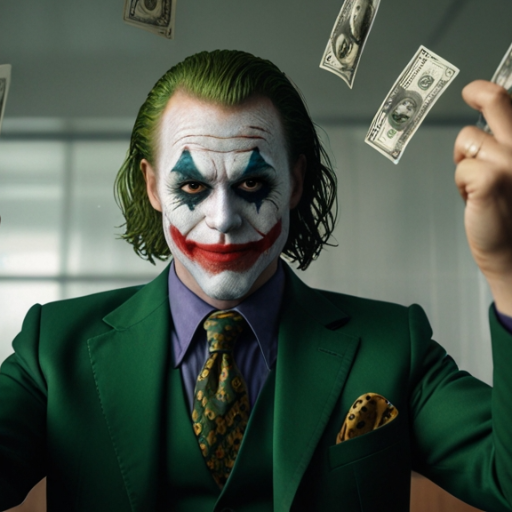
How to Make CBot Stop and get back on the next day
07 Aug 2023, 17:49
How to make my cbot stop and wait until next trading day to get back to trade after I lose 3 trades in a row?
I managed to count the last 3 trades and stop, but how do I make it back to trade on the following day?
// -------------------------------------------------------------------------------------------------
//
// This code is a cTrader Automate API example.
//
// This cBot is intended to be used as a sample and does not guarantee any particular outcome or
// profit of any kind. Use it at your own risk.
//
// All changes to this file might be lost on the next application update.
// If you are going to modify this file please make a copy using the "Duplicate" command.
//
// The "Sample RSI cBot" will create a buy order when the Relative Strength Index indicator crosses the level 30,
// and a Sell order when the RSI indicator crosses the level 70. The order is closed be either a Stop Loss, defined in
// the "Stop Loss" parameter, or by the opposite RSI crossing signal (buy orders close when RSI crosses the 70 level
// and sell orders are closed when RSI crosses the 30 level).
//
// The cBot can generate only one Buy or Sell order at any given time.
//
// -------------------------------------------------------------------------------------------------
using cAlgo.API;
using cAlgo.API.Indicators;
namespace cAlgo
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class NewRSI : Robot
{
[Parameter("Quantity (Lots)", Group = "Volume", DefaultValue = 1, MinValue = 0.01, Step = 0.01)]
public double Quantity { get; set; }
[Parameter("Source", Group = "RSI")]
public DataSeries Source { get; set; }
[Parameter("Periods", Group = "RSI", DefaultValue = 14)]
public int Periods { get; set; }
private RelativeStrengthIndex rsi;
protected override void OnStart()
{
rsi = Indicators.RelativeStrengthIndex(Source, Periods);
}
protected override void OnTick()
{
if (rsi.Result.LastValue < 30)
{
Close(TradeType.Sell);
Open(TradeType.Buy);
}
else if (rsi.Result.LastValue > 70)
{
Close(TradeType.Buy);
Open(TradeType.Sell);
}
foreach (var hist in History)
{
if (hist.Label == "NewRSI")
{
if (hist.NetProfit < 0)
{
losercount++;
if (losercount == 3)
Stop();
}
else
{
losercount = 0;
}
}
}
}
private void Close(TradeType tradeType)
{
foreach (var position in Positions.FindAll("NewRSI", SymbolName, tradeType))
ClosePosition(position);
}
private void Open(TradeType tradeType)
{
var position = Positions.Find("SampleRSI", SymbolName, tradeType);
var volumeInUnits = Symbol.QuantityToVolumeInUnits(Quantity);
if (position == null)
ExecuteMarketOrder(tradeType, SymbolName, volumeInUnits, "NewRSI");
}
}
}
Replies
PanagiotisChar
08 Aug 2023, 05:35
Hi there,
Instead of stopping it, you should use a flag and set it to false. When the flag is set to false, don't trade. On the change of each day, set the flag back to true.
Need help? Join us on Telegram
@PanagiotisChar
firemyst
07 Aug 2023, 23:58
If there's losing trades, you may want to consider leaving it as it is so you have to manually restart it. This does two things:
If you really want it to start again the next day, you can try this:
if (!allowTrades && Server.Time > 9am)
{
allowTrades = true;
}
In your OnTick method:
if (allowTrades)
{
////rest of method
}
else
return;
and you'd have to update your code:
if (losercount == 3)
{
allowTrades = false;
Stop();
}
@firemyst