How do I get / retrieve all open charts?
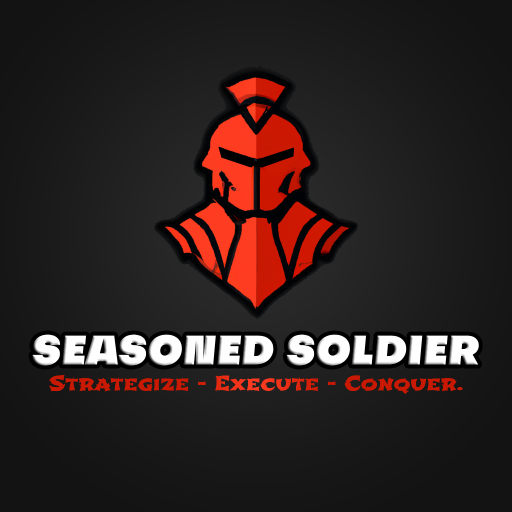
How do I get / retrieve all open charts?
19 Dec 2022, 15:40
I am trying to copy the lines I draw using the code below onto all other open charts. I am not exactly sure how to do it. Here is what I am thinking off:
I should iterate through the list of open charts and use the ChartObjects.DrawLine()
method to draw the lines onto each chart.
I am not exactly sure how to retrieve all charts. I looked through the reference section and under charts, there aren't any methods that can be used to retrieve all charts. Can anyone make a suggestion on how I could tackle this problem?
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class MyRobot : Robot
{
[Parameter("X", DefaultValue = 10)]
public int X { get; set; }
private string line1Name = "line1";
private string line2Name = "line2";
protected override void OnStart()
{
// Get the previous candle
var previousCandleClose = Bars.ClosePrices.Last(1);
var previousCandleOpen = Bars.OpenPrices.Last(1);
// If the previous candle close is higher than the previous candle open
if (previousCandleClose > previousCandleOpen)
{
// Draw a horizontal line at the previous candle close + X * 2
Chart.DrawHorizontalLine(line1Name, previousCandleClose + X, Color.Yellow, 2, LineStyle.Solid);
// Draw a horizontal line at the previous candle open - X * 2
Chart.DrawHorizontalLine(line2Name, previousCandleOpen - X, Color.Yellow, 2, LineStyle.Solid);
}
else
{
// Draw a horizontal line at the previous candle close - X * 2
Chart.DrawHorizontalLine(line1Name, previousCandleClose - X, Color.Yellow, 2, LineStyle.Solid);
// Draw a horizontal line at the previous candle open + X * 2
Chart.DrawHorizontalLine(line2Name, previousCandleOpen + X, Color.Yellow, 2, LineStyle.Solid);
}
}
protected override void OnBar()
{
// Get the previous candle
var previousCandleClose = Bars.ClosePrices.Last(1);
var previousCandleOpen = Bars.OpenPrices.Last(1);
// Remove the previous lines
Chart.RemoveObject(line1Name);
Chart.RemoveObject(line2Name);
// If the previous candle close is higher than the previous candle open
if (previousCandleClose > previousCandleOpen)
{
// Draw a horizontal line at the previous candle close + X * 2
Chart.DrawHorizontalLine(line1Name, previousCandleClose + X, Color.Yellow, 2, LineStyle.Solid);
// Draw a horizontal line at the previous candle open - X * 2
Chart.DrawHorizontalLine(line2Name, previousCandleOpen - X, Color.Yellow, 2, LineStyle.Solid);
}
else
{
// Draw a horizontal line at the previous candle close - X * 2
Chart.DrawHorizontalLine(line1Name, previousCandleClose - X, Color.Yellow, 2, LineStyle.Solid);
// Draw a horizontal line at the previous candle open + X * 2
Chart.DrawHorizontalLine(line2Name, previousCandleOpen + X, Color.Yellow, 2, LineStyle.Solid);
}
}
}
}
Replies
SeasonedSoldier
20 Dec 2022, 11:10
RE:
PanagiotisChar said:
Hi there,
You can't. You only have access to the chart on which the algo is attached too.
Thank you for your response. You have saved me countless hrs of research - at least I know that such method is not possible and can stop searching for it lol. Can you maybe recommend or suggest a method that would copy the lines drawn onto 1 chart be pasted onto all other open charts?
@SeasonedSoldier
SeasonedSoldier
20 Dec 2022, 11:10
( Updated at: 20 Dec 2022, 11:26 )
RE:
PanagiotisChar said:
Hi there,
You can't. You only have access to the chart on which the algo is attached too.
I was also thinking that create a "Price alert" (which get copied onto the rest of the same charts automatically). However, I run into the same problem again. I dont think there is a method in cAlgo that I could use to create an alert? I tried searching but could not find it. There is this "Notification" but it says it plays sound. It wouldn't create a Price alert line
@SeasonedSoldier
ctid9231468
02 Apr 2025, 13:23
( Updated at: 03 Apr 2025, 05:37 )
In a custom Plugin you can access all charts and retrieve a list of all open charts (frames) like this:
foreach (var chart in ChartManager)
{
Print("chart: " + chart.Id);
}
@ctid9231468
PanagiotisChar
20 Dec 2022, 09:27
Hi there,
You can't. You only have access to the chart on which the algo is attached too.
Aieden Technologies
Need help? Join us on Telegram
Need premium support? Trade with us
@PanagiotisChar