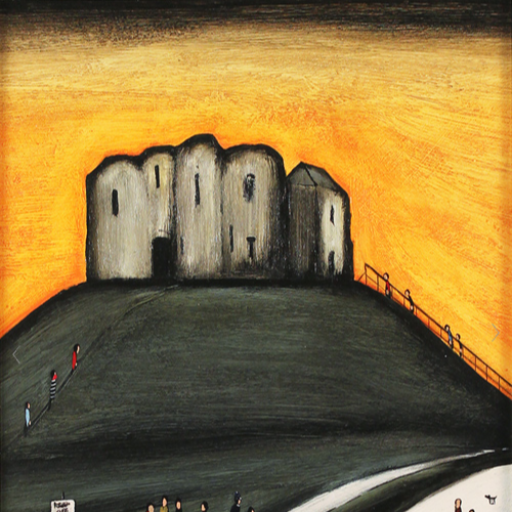
One operation but two modification on the HorizentalLine
09 Feb 2022, 16:30
Hi Support Team,
I have the below code, which draws a horizontal line at the last tick bid price. And if I drag the line to a new position, the code will update the horizontal line's comment with the new price of it.
The issue I have is that the 'private void SLModified' would run twice, once when I DragEnd of it. And the 2nd run was comments updated. Though it doesn't affect my programme running, I still want to know is there any way to avoid the 2nd run when I drag the line?
Thanks,
L
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Internals;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class HorizentalLineTest : Robot
{
private ChartHorizontalLine hlStopLoss;
protected override void OnStart()
{
hlStopLoss = Chart.DrawHorizontalLine("StopLoss", Symbol.Bid, Color.FromHex("#BBFF7777"), 1, LineStyle.LinesDots);
hlStopLoss.IsInteractive = true; hlStopLoss.IsLocked = false; hlStopLoss.Comment = Symbol.Bid.ToString();
Chart.ObjectsUpdated += SLModified;
}
protected override void OnTick() { }
protected override void OnStop() { Chart.RemoveObject(hlStopLoss.Name); }
private void SLModified(ChartObjectsUpdatedEventArgs obj) //Modify HorizentialLine, to update Price in Comments
{
var horizentalLine = obj.ChartObjects.FirstOrDefault(iObject => iObject.ObjectType == ChartObjectType.HorizontalLine && iObject.Name.Equals(hlStopLoss.Name, StringComparison.OrdinalIgnoreCase));
if (horizentalLine != null)
{
hlStopLoss.Comment = hlStopLoss.Y.ToString("0.00000");
Print("HorizentalLine's Y: " + hlStopLoss.Y.ToString("0.00000"));
}
}
}
}
Replies
Capt.Z-Fort.Builder
10 Feb 2022, 15:59
RE:
Thank you Musleh as always, it works pretty well. :)
amusleh said:
Hi,
You can use a bool flag to avoid the second call:
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Internals; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class HorizentalLineTest : Robot { private ChartHorizontalLine hlStopLoss; private bool _updatingComment; protected override void OnStart() { hlStopLoss = Chart.DrawHorizontalLine("StopLoss", Symbol.Bid, Color.FromHex("#BBFF7777"), 1, LineStyle.LinesDots); hlStopLoss.IsInteractive = true; hlStopLoss.IsLocked = false; hlStopLoss.Comment = Symbol.Bid.ToString(); Chart.ObjectsUpdated += SLModified; } protected override void OnTick() { } protected override void OnStop() { Chart.RemoveObject(hlStopLoss.Name); } private void SLModified(ChartObjectsUpdatedEventArgs obj) //Modify HorizentialLine, to update Price in Comments { if (_updatingComment) { _updatingComment = false; return; } var horizentalLine = obj.ChartObjects.FirstOrDefault(iObject => iObject.ObjectType == ChartObjectType.HorizontalLine && iObject.Name.Equals(hlStopLoss.Name, StringComparison.OrdinalIgnoreCase)); if (horizentalLine != null) { _updatingComment = true; hlStopLoss.Comment = hlStopLoss.Y.ToString("0.00000"); Print("HorizentalLine's Y: " + hlStopLoss.Y.ToString("0.00000")); } } } }
@Capt.Z-Fort.Builder
amusleh
10 Feb 2022, 08:32
Hi,
You can use a bool flag to avoid the second call:
@amusleh