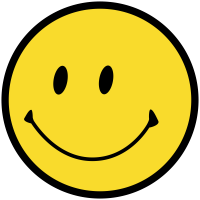
Swing high code
02 Sep 2021, 22:48
Hi,
Anyone that can help me code the following:
I want to store the last two swing highs and compare it to each other. A swing high is defined as a 3 bar high. For example if the candle high is higher than the previous three bar highs and higher than the next bar's high then it is regarded as a swing high. What I want is two have the last two swing high to compare them to each other to see if the last swing high is higher or lower than the previous one.
Below is a screen shot of the last two swing highs as defined above:
amusleh
03 Sep 2021, 10:31
Hi,
Try these extension methods (Copy this and paste it at the bottom of your indicator class):
Usage:
@amusleh