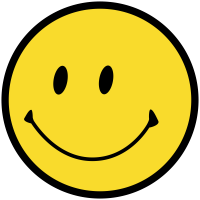
Custom Indicator call error
09 Aug 2021, 17:02
Hi,
I m trying to use 2 EMAs from a custom multi timeframe indicators. I get an error when I call the indicator in my cBot.
This is the indicator code:
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
namespace cAlgo.Indicators
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC)]
public class MyMTFEMA : Indicator
{
[Parameter(DefaultValue = 8)]
public int EMAPeriods1 { get; set; }
[Parameter(DefaultValue = 21)]
public int EMAPeriods2 { get; set; }
[Parameter("EMA Timeframe1", DefaultValue = "Hour")]
public TimeFrame EMATimeframe1 { get; set; }
[Parameter("EMA Timeframe2", DefaultValue = "Hour")]
public TimeFrame EMATimeframe2 { get; set; }
[Output("EMA1", Color = Colors.Blue)]
public IndicatorDataSeries EMA1 { get; set; }
[Output("EMA2", Color = Colors.Red)]
public IndicatorDataSeries EMA2 { get; set; }
private MarketSeries series1;
private MarketSeries series2;
private ExponentialMovingAverage Ema1;
private ExponentialMovingAverage Ema2;
protected override void Initialize()
{
series1 = MarketData.GetSeries(EMATimeframe1);
series2 = MarketData.GetSeries(EMATimeframe2);
Ema1 = Indicators.ExponentialMovingAverage(series1.Close, EMAPeriods1);
Ema2 = Indicators.ExponentialMovingAverage(series2.Close, EMAPeriods2);
}
public override void Calculate(int index)
{
var index1 = GetIndexByDate(series1, MarketSeries.OpenTime[index]);
if (index1 != -1)
{
EMA1[index] = Ema1.Result[index1];
}
var index2 = GetIndexByDate(series2, MarketSeries.OpenTime[index]);
if (index2 != -1)
{
EMA2[index] = Ema2.Result[index2];
}
}
private int GetIndexByDate(MarketSeries series, DateTime time)
{
for (int i = series.Close.Count - 1; i > 0; i--)
{
if (time == series.OpenTime[i])
return i;
}
return -1;
}
}
}
The I call it like this:
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class SIMPLEandPROFITABLEForexScalping : Robot
{
[Parameter("Risk %", DefaultValue = 0.02)]
public double RiskPct { get; set; }
[Parameter("EMA1 period", DefaultValue = 8)]
public int EMA1Period { get; set; }
[Parameter("EMA2 period", DefaultValue = 13)]
public int EMA2Period { get; set; }
[Parameter("EMA3 period", DefaultValue = 21)]
public int EMA3Period { get; set; }
private MovingAverage EMA1;
private MovingAverage EMA2;
private MovingAverage EMA3;
private MyMTFEMA MTFEMA;
protected override void OnStart()
{
// Put your initialization logic here
EMA1 = Indicators.MovingAverage(Bars.ClosePrices, EMA1Period, MovingAverageType.Exponential);
EMA2 = Indicators.MovingAverage(Bars.ClosePrices, EMA2Period, MovingAverageType.Exponential);
EMA3 = Indicators.MovingAverage(Bars.ClosePrices, EMA3Period, MovingAverageType.Exponential);
MTFEMA = Indicators.GetIndicator<MyMTFEMA>(8, 21, "Hour", "Hour");
}
However I get an error when trying to run the cBot with this message:
31/12/2019 02:00:00.000 | Backtesting started
31/12/2019 02:00:00.000 | Crashed in OnStart with AlgoActivationException: Can not set "Hour" as a value for "EMATimeframe1" parameter
31/12/2019 02:00:00.000 | Backtesting was stopped
I have tried various ways to change the way I call the parameters using the 8, 21 and two "Hour" options but still get an error.
Any assistance would be appreciated.
firemyst
29 Aug 2021, 12:25
RE:
Nickachino said:
Of course it's crashing there. You're passing in "Hour" as a string. The parameter is specifically for a TimeFrame object.
So you have to pass the timeframe similar to:
MTFEMA = Indicators.GetIndicator<MyMTFEMA>(8, 21, TimeFrame.Hour, TimeFrame.Hour);
@firemyst