How to send and recive message from TCP server ---- TCP Client
Created at 10 Apr 2014, 22:54
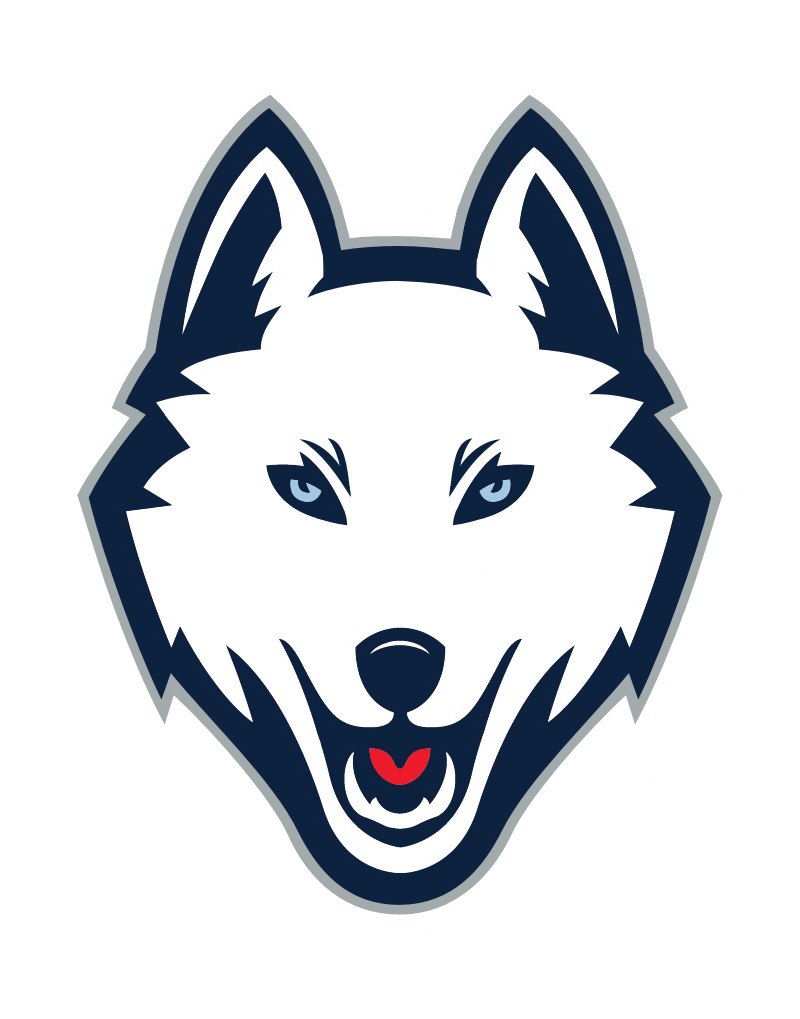
How to send and recive message from TCP server ---- TCP Client
10 Apr 2014, 22:54
Hi,
TCP Client:
using System; using System.Text; using System.Linq; using System.Net; using System.Net.Sockets; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)] public class SocketC : Robot { [Parameter(DefaultValue = 0.0)] public double Parameter { get; set; } public static string server = "192.168.0.102"; public static string responseData = ""; protected override void OnStart() { } protected override void OnBar() { Connect("Hello server"); } public void Connect(String mm) { try { // Create a TcpClient to send and recive message from server. Int32 port = 8888; TcpClient client = new TcpClient(server, port); // Translate the passed message into ASCII and store it as a Byte array. Byte[] data = System.Text.Encoding.ASCII.GetBytes(mm); // Get a client stream for reading and writing. NetworkStream stream = client.GetStream(); // Send the message to the connected TcpServer. stream.Write(data, 0, data.Length); Print("Sent: " + mm); // Buffer to store the response bytes. data = new Byte[256]; // Read the first batch of the TcpServer response bytes. Int32 bytes = stream.Read(data, 0, data.Length); responseData = System.Text.Encoding.ASCII.GetString(data, 0, bytes); Print("Received: {0}", responseData); // Close everything. stream.Close(); client.Close(); } catch (ArgumentNullException e) { Print("ArgumentNullException: {0}", e); } catch (SocketException e) { Print("SocketException: {0}", e); } Print("\n Press Enter to continue..."); //Console.Read(); } } }
Bye
Replies
mindbreaker
24 Jan 2015, 22:21
RE: Socket Connections
cAlgo server:
using System; using System.Text; using System.Linq; using System.Net; using System.Threading; using System.Net.Sockets; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)] public class Server : Robot { [Parameter(DefaultValue = 8888)] private int Port { get; set; } public static string ClientData = ""; public TcpListener Myserver = null; public TcpClient client = null; protected override void OnStart() { Thread t1 = new Thread(p => { ServerCopier(); }); t1.Start(); } protected override void OnTick() { Print("Tick Tak Tok"); Print("Data from client[OnBar]: " + ClientData); var name = "Up"; var text = "Received : " + ClientData; var staticPos = StaticPosition.TopRight; var color = Colors.Green; ChartObjects.DrawText(name, text, staticPos, color); } public void ServerCopier() { try { // Set the TcpListener on port Int32 port = Port; IPAddress localAddr = IPAddress.Parse("127.0.0.1"); // TcpListener server = new TcpListener(port); Myserver = new TcpListener(localAddr, port); // Start listening for client requests. Myserver.Start(); // Buffer for reading data Byte[] bytes = new Byte[1024]; String data = null; // Enter the listening loop. while (true) { Print("Waiting for a connection... "); // Perform a blocking call to accept requests. // You could also user server.AcceptSocket() here. client = Myserver.AcceptTcpClient(); Print("Connected!"); data = null; // Get a stream object for reading and writing NetworkStream stream = client.GetStream(); int i; // Loop to receive all the data sent by the client. while ((i = stream.Read(bytes, 0, bytes.Length)) != 0) { // Translate data bytes to a ASCII string. data = System.Text.Encoding.ASCII.GetString(bytes, 0, i); Print("Received: {0}", data); ClientData = "" + data; Print("Tick Tak Tok"); Print("Data from client[OnBar]: " + ClientData); var name = "Up"; var text = "Received : " + ClientData; var staticPos = StaticPosition.TopRight; var color = Colors.Green; ChartObjects.DrawText(name, text, staticPos, color); string pos = Symbol.Spread + " XX "; foreach (var pendingOrder in PendingOrders) { pos = pos + pendingOrder.TradeType + "XX" + pendingOrder.Volume + "XX" + pendingOrder.Id + "XXXX"; } data = pos.ToUpper(); byte[] msg = System.Text.Encoding.ASCII.GetBytes(data); // Send back a response. stream.Write(msg, 0, msg.Length); Print("Sent: {0}", data); } // Shutdown and end connection client.Close(); } } catch (SocketException e) { Print("SocketException: {0}", e); } finally { // Stop listening for new clients. client.Close(); Myserver.Stop(); } } protected override void OnStop() { client.Close(); Myserver.Stop(); Print("Server stoped."); } } }
@mindbreaker
breakermind
11 Apr 2014, 23:08 ( Updated at: 21 Dec 2023, 09:20 )
Socket Connections
TCP Multi-user Socket (Client/Server) scheme:
next will be: multi-client server example in java
Bye.
@breakermind