Save to file and read from file and copy trades.
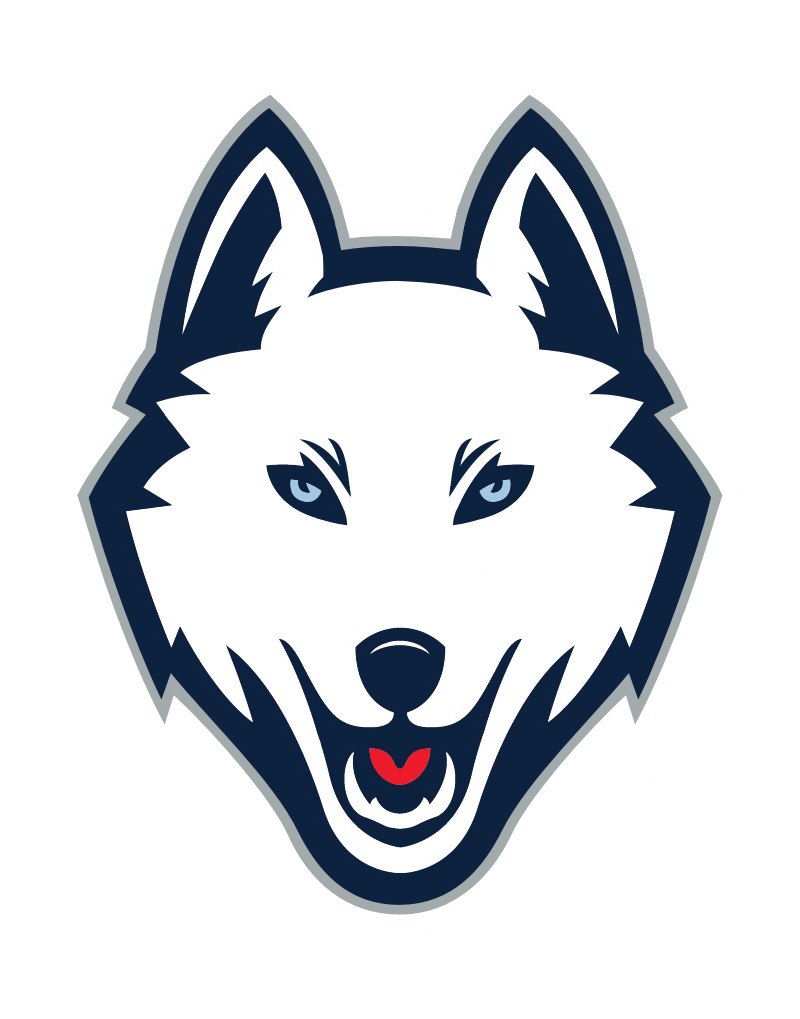
Save to file and read from file and copy trades.
07 Apr 2014, 12:53
Hi write and read from file:
using System; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.Indicators; using System.IO; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)] public class WriteToFileExample : Robot { //================================================================================ // Vars //================================================================================ // stream for file StreamWriter _fileWriter; // desktop folder static string desktopFolder = Environment.GetFolderPath(Environment.SpecialFolder.DesktopDirectory); // path to file string filePath = Path.Combine(desktopFolder, "z.txt"); //================================================================================ // OnStart //================================================================================ protected override void OnStart() { Print("Hello Master ... "); } //================================================================================ // OnTick //================================================================================ protected override void OnTick() { //=============================================================================== save to file _fileWriter = File.AppendText(filePath); //creating file _fileWriter.AutoFlush = true; //file will be saved on each change _fileWriter.WriteLine("Server Time: " + Server.Time); // close file and next read from file _fileWriter.Close(); //=========================================================================== read from file var dFolder = Environment.GetFolderPath(Environment.SpecialFolder.DesktopDirectory); var fPath = Path.Combine(dFolder, "z.txt"); string[] lines = System.IO.File.ReadAllLines(fPath); foreach (string line in lines) { // Use a tab to indent each line of the file. Print("Text from file: \t" + line); } } //================================================================================ // OnStop //================================================================================ protected override void OnStop() { Print("Bye Master ..."); } } }
Bye ...
Replies
breakermind
07 Apr 2014, 14:29
RE: Write and read opened positions from one robot to another
and why it does not work?
string[] stringArray = { "one", "two", "three", "four" }; var last=stringArray.Last(); var first=stringArray.First();
thx
@breakermind
deansi
06 Mar 2017, 05:24
Caution this code may open trades every bar..!
I wouldnt run this live, it looks like the lines in the second bot:
//================================================================================ protected override void OnBar() { if (Positions.Count < MaxPositions) { if (Volume > VolumeMax) { Volume = VolumeMax; } ExecuteMarketOrder(TradeType.Buy, Symbol, Volume, MyLabel, StopLoss, TakeProfit); ExecuteMarketOrder(TradeType.Sell, Symbol, Volume, MyLabel, StopLoss, TakeProfit); } } //================================================================================
would open a trade in both directions every single bar regardless of any input file or copy trades. In other words it wont do what it says and will empty your account.!
@deansi
mindbreaker
06 Mar 2017, 12:33
RE: Caution this code may open trades every bar..!
It was only example (for tests).
Working example with mysql databse you can see here:
https://github.com/fxstar/Copex-FxStar
Client: https://github.com/fxstar/Copex-FxStar/blob/master/_Copex-FxStar-Client-SL-TP-EquityStopLoss-SSL-Secure-Last.cs
Provider: https://github.com/fxstar/Copex-FxStar/blob/master/_Copex-FxStar-Trader.cs
@mindbreaker
breakermind
07 Apr 2014, 14:26
Write and read opened positions from one robot to another
Write robot:
second robot read from file opened positions:
@breakermind