cBot doesn't receive updated value from indicator - why?
Created at 17 Feb 2020, 16:03
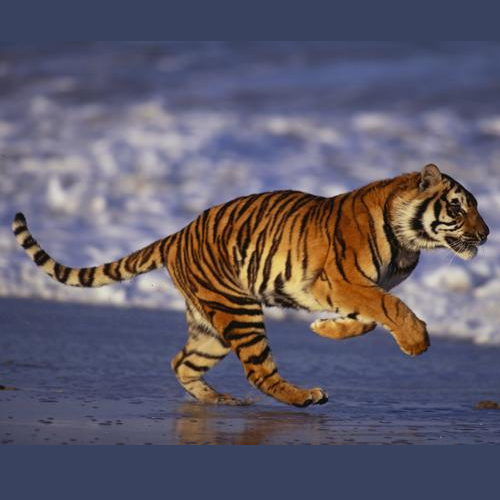
cBot doesn't receive updated value from indicator - why?
17 Feb 2020, 16:03
The BOT below only shows the value for "counter" at initialisation time, while the indicator clearly displays the increased counter in its window.
What do I need to change to be able to use indicator values that dynamically change?
[Obviosly the code below has no practical purpose, other than to demonstrate the problem at hand.]
The BOT
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class ChickenCounter : Robot
{
[Parameter("Source")]
public DataSeries Source { get; set; }
private Chickens chick;
protected override void OnStart()
{
chick = Indicators.GetIndicator<Chickens>(Source);
}
protected override void OnTick()
{
Print("OnTick | counter " + chick.counter);
}
protected override void OnStop()
{
// Put your deinitialization logic here
}
}
}
The INDICATOR:
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class Chickens : Indicator
{
[Parameter("Source")]
public DataSeries Source { get; set; }
public int counter = 1;
protected override void Initialize()
{
Print("INDICATOR PRINT | calculate | initial value for \"counter\" " + counter);
}
public override void Calculate(int index)
{
counter = counter > 100 ? 0 : ++counter;
ChartObjects.DrawText("max100", "counter " + counter, StaticPosition.BottomRight, Colors.Plum);
}
}
}
PanagiotisCharalampous
17 Feb 2020, 16:17
Hi there,
To fix this issue you need to add a dummy to the indicator and call it from the cBot. See below
Best Regards,
Panagiotis
Join us on Telegram
@PanagiotisCharalampous