Orders cancelled - not sure why
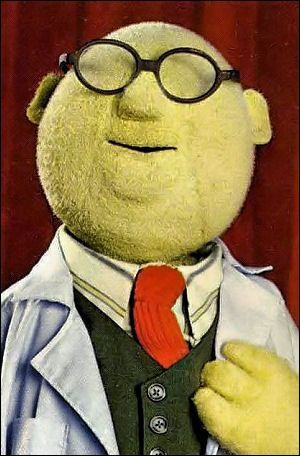
Orders cancelled - not sure why
15 May 2019, 23:37
I created a bot that enters stop limit orders with an expiry, but many of the orders are cancelled before their expiry time. I am not sure why this is happening.
I created some sample code that demonstrates the problem locally for me:
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class ProblemBot : Robot { private int _campaignId; private Random _random; [Parameter(DefaultValue = 1.0)] public double RiskPercent { get; set; } [Parameter(DefaultValue = 1000)] public int TradeSizeIncrement { get; set; } protected override void OnStart() { PendingOrders.Cancelled += PendingOrders_Cancelled; _random = new Random(); } private void PendingOrders_Cancelled(PendingOrderCancelledEventArgs args) { Print("Order {0} (expires {1}) cancelled: {2}", args.PendingOrder.Label, args.PendingOrder.ExpirationTime, args.Reason); } protected override void OnBar() { var index = CurrentBar - 1; if (IsSignal()) { _campaignId += 1; var high = MarketSeries.High[index]; var low = MarketSeries.Low[index]; var rangePips = (high - low) / Symbol.PipSize; var size = RoundDown(100 * RiskPercent * Account.Balance / rangePips); var interval = new TimeSpan(0, 12, 0, 0); var expiry = MarketSeries.OpenTime[CurrentBar].AddMilliseconds(-1) + interval; PlaceEntryOrder(TradeType.Buy, size, high, 1, _campaignId + "L0", rangePips, rangePips, expiry); PlaceEntryOrder(TradeType.Sell, size, low, 1, _campaignId + "S0", rangePips, rangePips, expiry); } } private TradeResult PlaceEntryOrder(TradeType side, double size, double trigger, double limitPips, string label, double islPips, double? takeProfitPips, DateTime expiry) { var res = PlaceStopLimitOrder(side, Symbol, size, trigger, limitPips, label, islPips, takeProfitPips, expiry); if (res.IsSuccessful) Print("Created {0} entry order {1}, size: {2}, trigger: {3}, expires: {4}", side, label, size, trigger, expiry); else { Print("Failed to create order {0}: {1}", label, res.Error); } return res; } private double RoundDown(double n, int mod = 2) { return mod * Math.Floor(n / (mod * TradeSizeIncrement)) * TradeSizeIncrement; } private bool IsSignal() { return _random.NextDouble() > 0.95; } private int CurrentBar { get { return MarketSeries.Close.Count - 1; } } } }
Here's the tail end of a sample log output running against GBPUSD 12h, showing several examples of orders that are getting cancelled before they expire:
Any clues?
Replies
PanagiotisCharalampous
17 May 2019, 14:37
Hi bishbashbosh,
In the absence of anyone from cTrader replying, I would be very interested if anyone else runs the above code and does/does not see the same behaviour.
We managed to reproduce and we are investigating :) we will reply!
Best Regards,
Panagiotis
@PanagiotisCharalampous
bishbashbosh
17 May 2019, 14:59
RE:
Panagiotis Charalampous said:
We managed to reproduce and we are investigating :) we will reply!
Hi Panagiotis,
Good to know it's not just me then!
Will await the outcome of your investigation with interest.
Best regards.
@bishbashbosh
PanagiotisCharalampous
20 May 2019, 09:19
Hi bishbashbosh,
We had a look at this behavior. It is caused because you are using Stop Limit orders. If the execution price of the order falls outside the range of the order, it will be cancelled.
Best Regards,
Panagiotis
@PanagiotisCharalampous
bishbashbosh
20 May 2019, 10:15
Hi Panagiotis,
Yes, when I increase the value of the parameter limitPips, the orders start filling.
Best regards.
@bishbashbosh
bishbashbosh
17 May 2019, 09:47
BTW, when I was running the above code using genuine signal code - i.e. so the buys/sells would trigger at the same bar each time, rather than randomly as above - the orders would cancel at exactly the same time, every time.
This leads me to suspect that perhaps the orders were about to fill, but got cancelled instead for some reason. Could be something to do with my demo account or the order settings or..?
In the absence of anyone from cTrader replying, I would be very interested if anyone else runs the above code and does/does not see the same behaviour.
@bishbashbosh