cBot based on the DSS Bressert indicator
Created at 20 Apr 2019, 09:23
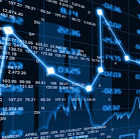
cBot based on the DSS Bressert indicator
20 Apr 2019, 09:23
Good day,
Would appreciate if you can have a look at this
This is the logic of the cBot:
Buy when DSS has crossed above WMA
Sell when DSS has crossed below WMA
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class ClickAlgoSchoolSMA : Robot { #region User defined parameters [Parameter("Instance Name", DefaultValue = "001")] public string InstanceName { get; set; } [Parameter("Lot Size", DefaultValue = 0.1)] public double LotSize { get; set; } [Parameter("Stochastic period", DefaultValue = 13)] public int Stochastic_Period { get; set; } [Parameter("EMA period", DefaultValue = 8)] public int EMA_Period { get; set; } [Parameter("WMA period", DefaultValue = 8)] public int WMA_Period { get; set; } [Parameter("Calculate OnBar", DefaultValue = false)] public bool CalculateOnBar { get; set; } [Parameter()] public DataSeries SourceSeries { get; set; } #endregion #region Indicator declarations private DSSBressert _DSSBressert { get; set; } private WeightedMovingAverage WMA { get; set; } #endregion #region cTrader events /// <summary> /// This is called when the robot first starts, it is only called once. /// </summary> protected override void OnStart() { // construct the indicators _DSSBressert = Indicators.GetIndicator<DSSBressert>(Stochastic_Period, EMA_Period); WMA = Indicators.WeightedMovingAverage(SourceSeries, WMA_Period); } /// <summary> /// This method is called every time the price changes for the symbol /// </summary> protected override void OnTick() { if (CalculateOnBar) { return; } ManagePositions(); } /// <summary> /// This method is called at every candle (bar) close, when it has formed /// </summary> protected override void OnBar() { if (!CalculateOnBar) { return; } ManagePositions(); } /// <summary> /// This method is called when your robot stops, can be used to clean-up memory resources. /// </summary> protected override void OnStop() { // unused } #endregion #region Position management private void ManagePositions() { if (_DSSBressert.HasCrossedAbove(WMA.Result)) { // if there is no buy position open, open one and close any sell position that is open if (!IsPositionOpenByType(TradeType.Buy)) { OpenPosition(TradeType.Buy); } ClosePosition(TradeType.Sell); } // if a sell position is already open and signal is buy do nothing if (_DSSBressert.HasCrossedAbove(WMA.Result)) { // if there is no sell position open, open one and close any buy position that is open if (!IsPositionOpenByType(TradeType.Sell)) { OpenPosition(TradeType.Sell); } ClosePosition(TradeType.Buy); } } /// <summary> /// Opens a new long position /// </summary> /// <param name="type"></param> private void OpenPosition(TradeType type) { // calculate volume from lot size. long volume = Symbol.QuantityToVolume(LotSize); // open a new position ExecuteMarketOrder(type, this.Symbol, volume, InstanceName, null, null); } /// <summary> /// /// </summary> /// <param name="type"></param> private void ClosePosition(TradeType type) { var p = Positions.Find(InstanceName, this.Symbol, type); if (p != null) { ClosePosition(p); } } #endregion #region Position Information /// <summary> /// /// </summary> /// <param name="type"></param> /// <returns></returns> private bool IsPositionOpenByType(TradeType type) { var p = Positions.FindAll(InstanceName, Symbol, type); if (p.Count() >= 1) { return true; } return false; } #endregion } }
indicator code
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class ClickAlgoSchoolSMA : Robot { #region User defined parameters [Parameter("Instance Name", DefaultValue = "001")] public string InstanceName { get; set; } [Parameter("Lot Size", DefaultValue = 0.1)] public double LotSize { get; set; } [Parameter("Stochastic period", DefaultValue = 13)] public int Stochastic_Period { get; set; } [Parameter("EMA period", DefaultValue = 8)] public int EMA_Period { get; set; } [Parameter("WMA period", DefaultValue = 8)] public int WMA_Period { get; set; } [Parameter("Calculate OnBar", DefaultValue = false)] public bool CalculateOnBar { get; set; } [Parameter()] public DataSeries SourceSeries { get; set; } #endregion #region Indicator declarations private DSSBressert _DSSBressert { get; set; } private WeightedMovingAverage WMA { get; set; } #endregion #region cTrader events /// <summary> /// This is called when the robot first starts, it is only called once. /// </summary> protected override void OnStart() { // construct the indicators _DSSBressert = Indicators.GetIndicator<DSSBressert>(Stochastic_Period, EMA_Period); WMA = Indicators.WeightedMovingAverage(SourceSeries, WMA_Period); } /// <summary> /// This method is called every time the price changes for the symbol /// </summary> protected override void OnTick() { if (CalculateOnBar) { return; } ManagePositions(); } /// <summary> /// This method is called at every candle (bar) close, when it has formed /// </summary> protected override void OnBar() { if (!CalculateOnBar) { return; } ManagePositions(); } /// <summary> /// This method is called when your robot stops, can be used to clean-up memory resources. /// </summary> protected override void OnStop() { // unused } #endregion #region Position management private void ManagePositions() { if (_DSSBressert.HasCrossedAbove(WMA.Result)) { // if there is no buy position open, open one and close any sell position that is open if (!IsPositionOpenByType(TradeType.Buy)) { OpenPosition(TradeType.Buy); } ClosePosition(TradeType.Sell); } // if a sell position is already open and signal is buy do nothing if (_DSSBressert.HasCrossedAbove(WMA.Result)) { // if there is no sell position open, open one and close any buy position that is open if (!IsPositionOpenByType(TradeType.Sell)) { OpenPosition(TradeType.Sell); } ClosePosition(TradeType.Buy); } } /// <summary> /// Opens a new long position /// </summary> /// <param name="type"></param> private void OpenPosition(TradeType type) { // calculate volume from lot size. long volume = Symbol.QuantityToVolume(LotSize); // open a new position ExecuteMarketOrder(type, this.Symbol, volume, InstanceName, null, null); } /// <summary> /// /// </summary> /// <param name="type"></param> private void ClosePosition(TradeType type) { var p = Positions.Find(InstanceName, this.Symbol, type); if (p != null) { ClosePosition(p); } } #endregion #region Position Information /// <summary> /// /// </summary> /// <param name="type"></param> /// <returns></returns> private bool IsPositionOpenByType(TradeType type) { var p = Positions.FindAll(InstanceName, Symbol, type); if (p.Count() >= 1) { return true; } return false; } #endregion } }
Replies
amusleh
09 Mar 2022, 08:42
Hi,
The indicator code is not posted by him, so it's not clear how it works or which output properties it has.
If the DSS indicator output property name is Result then try this:
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class ClickAlgoSchoolSMA : Robot
{
#region User defined parameters
[Parameter("Instance Name", DefaultValue = "001")]
public string InstanceName { get; set; }
[Parameter("Lot Size", DefaultValue = 0.1)]
public double LotSize { get; set; }
[Parameter("Stochastic period", DefaultValue = 13)]
public int Stochastic_Period { get; set; }
[Parameter("EMA period", DefaultValue = 8)]
public int EMA_Period { get; set; }
[Parameter("WMA period", DefaultValue = 8)]
public int WMA_Period { get; set; }
[Parameter("Calculate OnBar", DefaultValue = false)]
public bool CalculateOnBar { get; set; }
[Parameter()]
public DataSeries SourceSeries { get; set; }
#endregion
#region Indicator declarations
private DSSBressert _DSSBressert { get; set; }
private WeightedMovingAverage WMA { get; set; }
#endregion
#region cTrader events
/// <summary>
/// This is called when the robot first starts, it is only called once.
/// </summary>
protected override void OnStart()
{
// construct the indicators
_DSSBressert = Indicators.GetIndicator<DSSBressert>(Stochastic_Period, EMA_Period);
WMA = Indicators.WeightedMovingAverage(SourceSeries, WMA_Period);
}
/// <summary>
/// This method is called every time the price changes for the symbol
/// </summary>
protected override void OnTick()
{
if (CalculateOnBar)
{
return;
}
ManagePositions();
}
/// <summary>
/// This method is called at every candle (bar) close, when it has formed
/// </summary>
protected override void OnBar()
{
if (!CalculateOnBar)
{
return;
}
ManagePositions();
}
/// <summary>
/// This method is called when your robot stops, can be used to clean-up memory resources.
/// </summary>
protected override void OnStop()
{
// unused
}
#endregion
#region Position management
private void ManagePositions()
{
if (_DSSBressert.Result.HasCrossedAbove(WMA.Result, 1))
{
// if there is no buy position open, open one and close any sell position that is open
if (!IsPositionOpenByType(TradeType.Buy))
{
OpenPosition(TradeType.Buy);
}
ClosePosition(TradeType.Sell);
}
// if a sell position is already open and signal is buy do nothing
if (_DSSBressert.Result.HasCrossedAbove(WMA.Result, 1))
{
// if there is no sell position open, open one and close any buy position that is open
if (!IsPositionOpenByType(TradeType.Sell))
{
OpenPosition(TradeType.Sell);
}
ClosePosition(TradeType.Buy);
}
}
/// <summary>
/// Opens a new long position
/// </summary>
/// <param name="type"></param>
private void OpenPosition(TradeType type)
{
// calculate volume from lot size.
long volume = Symbol.QuantityToVolume(LotSize);
// open a new position
ExecuteMarketOrder(type, this.Symbol, volume, InstanceName, null, null);
}
/// <summary>
///
/// </summary>
/// <param name="type"></param>
private void ClosePosition(TradeType type)
{
var p = Positions.Find(InstanceName, this.Symbol, type);
if (p != null)
{
ClosePosition(p);
}
}
#endregion
#region Position Information
/// <summary>
///
/// </summary>
/// <param name="type"></param>
/// <returns></returns>
private bool IsPositionOpenByType(TradeType type)
{
var p = Positions.FindAll(InstanceName, Symbol, type);
if (p.Count() >= 1)
{
return true;
}
return false;
}
#endregion
}
}
@amusleh
philippus.badenhorst
08 Mar 2022, 19:32
Final DSS Bressert Cbot code
Can the final corrected code be posted please.
@philippus.badenhorst