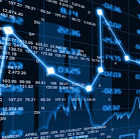
cBot based on the DSS Bressert indicator
09 Mar 2019, 14:28
Many thanks for your time looking at this.
Problem with error code received: Error CS1038: #endregion directive expected
This is the code for the cBot
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class Sampletemplate : Robot { #region User defined parameters // Parameters DSS Bressert [Parameter("Source DSS")] public DataSeries SourceDSS { get; set; } [Parameter("Stochastic Period")] public int PeriodsStochastic { get; set; } [Parameter("EMA Period")] public int PeriodsEMA { get; set; } [Parameter("WMA Period")] public int PeriodsWMA { get; set; } // Parameters for trading positions [Parameter(DefaultValue = 1000, MinValue = 0)] public int Volume { get; set; } #region Indicator declarations private DSSBressert _DSS { get; set; } private const string label = "cBot_A"; protected override void OnStart() { // construct the indicators _DSS = Indicators.GetIndicator<DSSBressert>(SourceDSS, PeriodsStochastic, PeriodsEMA, PeriodsWMA); } protected override void OnBar() { var longPosition = Positions.Find(label, Symbol, TradeType.Buy); var shortPosition = Positions.Find(label, Symbol, TradeType.Sell); var current_DSS = _DSS.Result.Last(0); var previous_DSS = _DSS.Result.Last(1); if (current_DSS > previous_DSS && longPosition == null) { if (shortPosition != null) ClosePosition(shortPosition); ExecuteMarketOrder(TradeType.Buy, Symbol, Volume, label); } else if (current_DSS < previous_DSS && shortPosition == null) { if (longPosition != null) ClosePosition(longPosition); ExecuteMarketOrder(TradeType.Sell, Symbol, Volume, label); } } } }
The logic of the cBot is to open and close trades based on the swing of the DSS Bressert indicator
This is the code for the indicator https://ctrader.com/algos/indicators/show/1224#comment-5195
using System; using cAlgo.API; using cAlgo.API.Internals; using cAlgo.API.Indicators; namespace cAlgo { [Levels(0, 20, 40, 60, 80, 100)] [Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None, ScalePrecision = 2)] public class DSSBressert : Indicator { [Parameter("Stochastic period", DefaultValue = 13)] public int Stochastic_Period { get; set; } [Parameter("EMA period", DefaultValue = 8)] public int EMA_Period { get; set; } [Parameter("WMA period", DefaultValue = 8)] public int WMA_Period { get; set; } [Output("DSS", Color = Colors.White, Thickness = 1)] public IndicatorDataSeries DSS { get; set; } [Output("DSS Up", Color = Colors.Green, PlotType = PlotType.Points, Thickness = 5)] public IndicatorDataSeries DSS_Up { get; set; } [Output("DSS Down", Color = Colors.Red, PlotType = PlotType.Points, Thickness = 5)] public IndicatorDataSeries DSS_Down { get; set; } [Output("WMA", Color = Colors.Gold, Thickness = 1)] public IndicatorDataSeries WmaResult { get; set; } [Output("L1", LineStyle = LineStyle.Solid, Color = Colors.LightGray)] public IndicatorDataSeries L1 { get; set; } [Output("L2", LineStyle = LineStyle.DotsRare, Color = Colors.LightGray)] public IndicatorDataSeries L2 { get; set; } [Output("L3", LineStyle = LineStyle.DotsRare, Color = Colors.LightGray)] public IndicatorDataSeries L3 { get; set; } [Output("L4", LineStyle = LineStyle.Solid, Color = Colors.LightGray)] public IndicatorDataSeries L4 { get; set; } private double Ln = 0; private double Hn = 0; private double LXn = 0; private double HXn = 0; private double alpha = 0; private IndicatorDataSeries mit; private WeightedMovingAverage WMA; protected override void Initialize() { mit = CreateDataSeries(); alpha = 2.0 / (1.0 + EMA_Period); WMA = Indicators.WeightedMovingAverage(DSS, WMA_Period); } public override void Calculate(int index) { L1[index] = 20; L2[index] = 40; L3[index] = 60; L4[index] = 80; if (double.IsNaN(mit[index - 1])) { mit[index - 1] = 0; } if (double.IsNaN(DSS[index - 1])) { DSS[index - 1] = 0; } Ln = MarketSeries.Low.Minimum(Stochastic_Period); Hn = MarketSeries.High.Maximum(Stochastic_Period); mit[index] = mit[index - 1] + alpha * ((((MarketSeries.Close[index] - Ln) / (Hn - Ln)) * 100) - mit[index - 1]); LXn = mit.Minimum(Stochastic_Period); HXn = mit.Maximum(Stochastic_Period); DSS[index] = DSS[index - 1] + alpha * ((((mit[index] - LXn) / (HXn - LXn)) * 100) - DSS[index - 1]); if (DSS[index] > DSS[index - 1]) { DSS_Up[index] = DSS[index]; DSS_Down[index] = double.NaN; } if (DSS[index] < DSS[index - 1]) { DSS_Down[index] = DSS[index]; DSS_Up[index] = double.NaN; } WmaResult[index] = WMA.Result[index]; } }
Replies
jamespeterwilkinson
12 Mar 2019, 13:54
RE: Thank you
Panagiotis Charalampous said:
Hi James,
As the message clearly states, you need to end your region somewhere. You start your region here
#region Indicator declarations private DSSBressert _DSS { get; set; }Best Regards,
Panagiotis
@jamespeterwilkinson
PanagiotisCharalampous
12 Mar 2019, 11:30
Hi James,
As the message clearly states, you need to end your region somewhere. You start your region here
Best Regards,
Panagiotis
@PanagiotisCharalampous