SimpleWeekRobot - earn monday to friday but ...
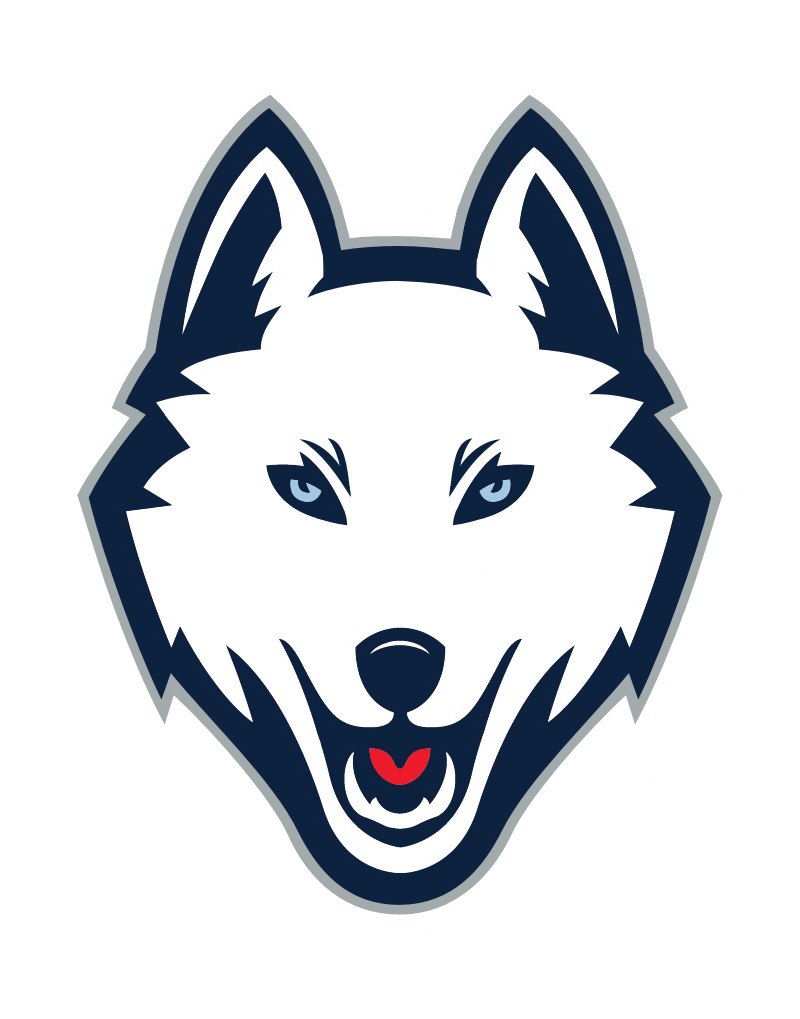
SimpleWeekRobot - earn monday to friday but ...
17 Aug 2013, 16:10
Hello,
This is my simple robot IT set pending positions on levels abowe and below week open price
but I need restart week price when
Day == Monday and Hour == 0 and minute == 0 and seconds < 10 only one time in week
how to do that ?
//================================================================================ // SimpleWeekRobo // Start at Monday 00:00 close on Friday 23:00 // Simple Monday-Friday script without any security with close when earn // If CloseWhenEarn == 0 then dont close positions //================================================================================ using System; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Requests; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC)] public class SimpleWeekRobo : Robot { //================================================================================ // Parametrs //================================================================================ private Position _position; private double WeekStart; [Parameter(DefaultValue = false)] public bool CloseOnlyWithProfit { get; set; } [Parameter(DefaultValue = 10000, MinValue = 1000)] public int Volume { get; set; } [Parameter(DefaultValue = 25, MinValue = 5)] public int Spacing { get; set; } [Parameter(DefaultValue = 10, MinValue = 2)] public int HowMuchPositions { get; set; } [Parameter(DefaultValue = 100, MinValue = 0)] public int CloseWhenEarn { get; set; } //================================================================================ // OnStart //================================================================================ protected override void OnStart() { // week start price or run script price if (CloseOnlyWithProfit == true) { foreach (var openedPosition in Account.Positions) { if (openedPosition.GrossProfit > 0) { Trade.Close(openedPosition); } } } WeekStart = Symbol.Ask; for (int i = 1; i < HowMuchPositions; i++) { Trade.CreateBuyStopOrder(Symbol, 10000, Symbol.Ask + Spacing * i * Symbol.PipSize); } for (int j = 1; j < HowMuchPositions; j++) { Trade.CreateSellStopOrder(Symbol, 10000, Symbol.Bid - Spacing * j * Symbol.PipSize); } } //================================================================================ // OnTick //================================================================================ protected override void OnTick() { var netProfit = 0.0; foreach (var openedPosition in Account.Positions) { netProfit += openedPosition.NetProfit; } if (netProfit >= CloseWhenEarn && CloseWhenEarn != 0) { foreach (var openedPosition in Account.Positions) { Trade.Close(openedPosition); } Stop(); } } //================================================================================ // OnPositionOpened //================================================================================ protected override void OnPositionOpened(Position openedPosition) { int BuyPos = 0; int SellPos = 0; foreach (var position in Account.Positions) { // Count opened positions if (position.TradeType == TradeType.Buy) { BuyPos = BuyPos + 1; } if (position.TradeType == TradeType.Sell) { SellPos = SellPos + 1; } } Print("All Buy positions: " + BuyPos); Print("All Sell positions: " + SellPos); } // end OnPositionOpened //================================================================================ // OnBar //================================================================================ protected override void OnBar() { } //================================================================================ // OnPositionClosed //================================================================================ protected override void OnPositionClosed(Position closedPosition) { } //================================================================================ // OnStop //================================================================================ protected override void OnStop() { } //================================================================================ // Function //================================================================================ private void Buy() { Trade.CreateBuyMarketOrder(Symbol, Volume); } private void Sell() { Trade.CreateSellMarketOrder(Symbol, Volume); } private void ClosePosition() { if (_position != null) { Trade.Close(_position); _position = null; } } //================================================================================ // Robot end //================================================================================ } }
Thanks
Replies
breakermind
21 Aug 2013, 16:26
RE: RE:
Hi
with TrailingStop
//================================================================================ // SimpleWeekRobo // Start at Monday 00:00 close on Friday 21:00 // Simple Monday-Friday script without any security with close when earn // If CloseWhenEarn == 0 then dont close positions // if BackStop > 0 then Trailink dont work and BackStop set minimal SL above entryprice // if TrailingStop == 0 then Trailing dont work // Trailing and BackStop (Pips value) //================================================================================ using System; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Requests; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC)] public class SimpleWeekRobo : Robot { //================================================================================ // Parametrs //================================================================================ private Position _position; private double WeekStart; [Parameter(DefaultValue = false)] public bool CloseOnlyWithProfit { get; set; } [Parameter(DefaultValue = 10000, MinValue = 1000)] public int Volume { get; set; } [Parameter(DefaultValue = 25, MinValue = 5)] public int Spacing { get; set; } [Parameter(DefaultValue = 10, MinValue = 2)] public int HowMuchPositions { get; set; } [Parameter(DefaultValue = 100, MinValue = 0)] public int CloseWhenEarn { get; set; } [Parameter(DefaultValue = 0, MinValue = 0)] public int BackStop { get; set; } [Parameter(DefaultValue = 5, MinValue = 0)] public int BackStopValue { get; set; } [Parameter(DefaultValue = 0, MinValue = 0)] public int TrailingStop { get; set; } private bool isFirstBarInitialized; //================================================================================ // OnStart //================================================================================ protected override void OnStart() { // week start price or run script price if (CloseOnlyWithProfit == true) { foreach (var openedPosition in Account.Positions) { if (openedPosition.GrossProfit > 0) { Trade.Close(openedPosition); } } } WeekStart = Symbol.Ask; for (int i = 1; i < HowMuchPositions; i++) { Trade.CreateBuyStopOrder(Symbol, Volume, Symbol.Ask + Spacing * i * Symbol.PipSize); } for (int j = 1; j < HowMuchPositions; j++) { Trade.CreateSellStopOrder(Symbol, Volume, Symbol.Bid - Spacing * j * Symbol.PipSize); } } //================================================================================ // OnTick //================================================================================ protected override void OnTick() { //===================================================== Back Trailing if (BackStop > 0) { foreach (var openedPosition in Account.Positions) { Position Position = openedPosition; if (Position.TradeType == TradeType.Buy) { double distance = Symbol.Bid - Position.EntryPrice; if (distance >= BackStop * Symbol.PipSize) { double newStopLossPrice = Math.Round(Symbol.Bid - BackStopValue * Symbol.PipSize, Symbol.Digits); if (Position.StopLoss == null) { Trade.ModifyPosition(Position, newStopLossPrice, Position.TakeProfit); } } } else { double distance = Position.EntryPrice - Symbol.Ask; if (distance >= BackStop * Symbol.PipSize) { double newStopLossPrice = Math.Round(Symbol.Ask + BackStopValue * Symbol.PipSize, Symbol.Digits); if (Position.StopLoss == null) { Trade.ModifyPosition(Position, newStopLossPrice, Position.TakeProfit); } } } } } //===================================================== Trailing if (TrailingStop > 0 && BackStop == 0) { foreach (var openedPosition in Account.Positions) { Position Position = openedPosition; if (Position.TradeType == TradeType.Buy) { double distance = Symbol.Bid - Position.EntryPrice; if (distance >= TrailingStop * Symbol.PipSize) { double newStopLossPrice = Math.Round(Symbol.Bid - TrailingStop * Symbol.PipSize, Symbol.Digits); if (Position.StopLoss == null || newStopLossPrice > Position.StopLoss) { Trade.ModifyPosition(Position, newStopLossPrice, Position.TakeProfit); } } } else { double distance = Position.EntryPrice - Symbol.Ask; if (distance >= TrailingStop * Symbol.PipSize) { double newStopLossPrice = Math.Round(Symbol.Ask + TrailingStop * Symbol.PipSize, Symbol.Digits); if (Position.StopLoss == null || newStopLossPrice < Position.StopLoss) { Trade.ModifyPosition(Position, newStopLossPrice, Position.TakeProfit); } } } } } //============================================================= Profit close var netProfit = 0.0; foreach (var openedPosition in Account.Positions) { netProfit += openedPosition.NetProfit; } if (netProfit >= CloseWhenEarn && CloseWhenEarn != 0) { foreach (var openedPosition in Account.Positions) { Trade.Close(openedPosition); } Stop(); } } //================================================================================ // OnPositionOpened //================================================================================ protected override void OnPositionOpened(Position openedPosition) { int BuyPos = 0; int SellPos = 0; foreach (var position in Account.Positions) { // Count opened positions if (position.TradeType == TradeType.Buy) { BuyPos = BuyPos + 1; } if (position.TradeType == TradeType.Sell) { SellPos = SellPos + 1; } } Print("All Buy positions: " + BuyPos); Print("All Sell positions: " + SellPos); } // end OnPositionOpened //================================================================================ // Robot end //================================================================================ } }
@breakermind
breakermind
12 Jun 2014, 15:13
WeekStart-WeekEnd
Update :D
// Start deposit: 1000$ Position volume: 500000 leverage 1:500 week: 14/04/2014 <-> 19/04/2014 Ending equity: 51055,30
// Start deposit: 1000$ Position volume: 300000 leverage 1:500 week: 14/04/2014 <-> 19/04/2014 Ending equity: 24,736.95
// Start deposit: 1000$ Position volume: 300000 leverage 1:500 week: 2/06/2014 <-> 7/06/2014 Ending equity: 16043.74
// Start deposit: 1000$ Position volume: 500000 leverage 1:500 week: 2/06/2014 <-> 7/06/2014 Ending equity: 26073.84
Have a nice day:
//================================================================================ // WeekStart-WeekEnd // Start at Monday 00:00 close on Friday 23:00 // Simple Monday-Friday script without any security with close when earn // If CloseWhenEarn == 0 then dont close positions //================================================================================ using System; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Requests; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)] public class SimpleWeekRobo : Robot { //================================================================================ // Parametrs //================================================================================ private Position _position; private double WeekStart; [Parameter(DefaultValue = false)] public bool CloseOnlyWithProfit { get; set; } [Parameter(DefaultValue = 300000, MinValue = 1000)] public int Volume { get; set; } [Parameter(DefaultValue = 25, MinValue = 5)] public int Spacing { get; set; } [Parameter(DefaultValue = 10, MinValue = 2)] public int HowMuchPositions { get; set; } [Parameter(DefaultValue = 0, MinValue = 0)] public int CloseWhenEarn { get; set; } //================================================================================ // OnStart //================================================================================ protected override void OnStart() { // week start price or run script price if (CloseOnlyWithProfit == true) { foreach (var openedPosition in Positions) { if (openedPosition.GrossProfit > 0) { ClosePosition(openedPosition); } } } WeekStart = Symbol.Ask; for (int i = 1; i < HowMuchPositions; i++) { PlaceStopOrder(TradeType.Buy, Symbol, Volume, Symbol.Ask + Spacing * i * Symbol.PipSize); } for (int j = 1; j < HowMuchPositions; j++) { PlaceStopOrder(TradeType.Sell, Symbol, Volume, Symbol.Bid - Spacing * j * Symbol.PipSize); } } //================================================================================ // OnTick //================================================================================ protected override void OnTick() { var netProfit = 0.0; foreach (var openedPosition in Positions) { netProfit += openedPosition.NetProfit; } if (netProfit >= CloseWhenEarn && CloseWhenEarn != 0) { foreach (var openedPosition in Positions) { ClosePosition(openedPosition); } Stop(); } } //================================================================================ // OnPositionOpened //================================================================================ protected override void OnPositionOpened(Position openedPosition) { int BuyPos = 0; int SellPos = 0; foreach (var position in Positions) { // Count opened positions if (position.TradeType == TradeType.Buy) { BuyPos = BuyPos + 1; } if (position.TradeType == TradeType.Sell) { SellPos = SellPos + 1; } } Print("All Buy positions: " + BuyPos); Print("All Sell positions: " + SellPos); } // end OnPositionOpened //================================================================================ // OnBar //================================================================================ protected override void OnBar() { } //================================================================================ // OnPositionClosed //================================================================================ protected override void OnPositionClosed(Position closedPosition) { } //================================================================================ // OnStop //================================================================================ protected override void OnStop() { } //================================================================================ // Function //================================================================================ private void Buy() { ExecuteMarketOrder(TradeType.Buy, Symbol, 10000, "", 1000, 1000); } private void Sell() { ExecuteMarketOrder(TradeType.Sell, Symbol, 10000, "", 1000, 1000); } private void ClosePosition() { if (_position != null) { ClosePosition(_position); _position = null; } } //================================================================================ // Robot end //================================================================================ } }
Bye
@breakermind
breakermind
12 Jun 2014, 16:10
( Updated at: 23 Jan 2024, 13:11 )
RE: WeekStart-WeekEnd
[/algos/cbots/show/503]
@breakermind
breakermind
12 Jun 2014, 17:29
( Updated at: 23 Jan 2024, 13:11 )
RE: RE: WeekStart-WeekEnd
breakermind said:
[/algos/cbots/show/503]
New with safe profits:
//================================================================================ // SimpleWeekRobo // Start at Monday 00:00 close on Friday 23:00 // Simple Monday-Friday script without any security with close when earn // If CloseWhenEarn == 0 then dont close positions //================================================================================ using System; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Requests; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)] public class SimpleWeekRobo : Robot { //================================================================================ // Parametrs //================================================================================ private double WeekStart; private double LastProfit = 0; private double StopMoneyLoss = 0; [Parameter(DefaultValue = 300000, MinValue = 1000)] public int Volume { get; set; } [Parameter(DefaultValue = 25, MinValue = 5)] public int Spacing { get; set; } [Parameter(DefaultValue = 10, MinValue = 2)] public int HowMuchPositions { get; set; } // close when earn [Parameter(DefaultValue = 0, MinValue = 0)] public int CloseWhenEarn { get; set; } // safe deposit works when earn 100% [Parameter(DefaultValue = false)] public bool MoneyStopLossOn { get; set; } [Parameter(DefaultValue = 1000, MinValue = 100)] public int StartDeposit { get; set; } [Parameter(DefaultValue = 50, MinValue = 10, MaxValue = 100)] public int StopLossPercent { get; set; } [Parameter(DefaultValue = 2, MinValue = 1, MaxValue = 100)] public int OnWhenDepositX { get; set; } //================================================================================ // OnStart //================================================================================ protected override void OnStart() { WeekStart = Symbol.Ask; for (int i = 1; i < HowMuchPositions; i++) { PlaceStopOrder(TradeType.Buy, Symbol, Volume, Symbol.Ask + Spacing * i * Symbol.PipSize); } for (int j = 1; j < HowMuchPositions; j++) { PlaceStopOrder(TradeType.Sell, Symbol, Volume, Symbol.Bid - Spacing * j * Symbol.PipSize); } } //================================================================================ // OnTick //================================================================================ protected override void OnTick() { var netProfit = 0.0; foreach (var openedPosition in Positions) { netProfit += openedPosition.NetProfit; } if (MoneyStopLossOn == true) { // safe deposit works when earn startdeposit x 1,2,3,4,5... if (LastProfit < Account.Equity && Account.Equity > (StartDeposit * OnWhenDepositX)) { LastProfit = Account.Equity; } if (Account.Equity > StartDeposit * 3) { //StopLossPercent = 90; } StopMoneyLoss = LastProfit * (StopLossPercent * 0.01); Print("LastProfit: ", LastProfit); Print("Equity: ", Account.Equity); Print("StopLossMoney: ", StopMoneyLoss); if (Account.Equity < StopMoneyLoss) { foreach (var openedPosition in Positions) { ClosePosition(openedPosition); } Stop(); } } if (netProfit >= CloseWhenEarn && CloseWhenEarn != 0) { foreach (var openedPosition in Positions) { ClosePosition(openedPosition); } Stop(); } } protected override void OnStop() { Print("cBot stop"); } //================================================================================ // Robot end //================================================================================ } }
Bye.
@breakermind
breakermind
03 Jul 2014, 20:21
RE:
fwends said:
When running your bot with the latest code
It fails to enter the take profit, it tries to modify the position, but the brokers says "invalid request"
Can you fix this please
Hi,
You have to change yourself.
cBots made me bored :)
Regards and Bye.
@breakermind
breakermind
05 Jul 2014, 15:27
RE: RE:
Update
//================================================================================ // SimpleWeekRobo // Start at Week start(Day start 00:00) // (M15-M30 regression(2000,2,3)(1.7,2,2000)) // 100Pips levels, suport or resistance // Simple Monday-Friday script without any security with close when earn // If CloseWhenEarn == 0 then dont close positions // If TP or SL == 0 dont modifing positions // If TrailingStop > 0 ==> BackStop == 0 //================================================================================ using System; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Requests; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)] public class SimpleWeekRobo : Robot { //================================================================================ // Parametrs //================================================================================ private Position _position; private double WeekStart; private double LastProfit = 0; private double StopMoneyLoss = 0; [Parameter(DefaultValue = true)] public bool SetBUY { get; set; } [Parameter(DefaultValue = true)] public bool SetSELL { get; set; } [Parameter(DefaultValue = 300000, MinValue = 1000)] public int Volume { get; set; } [Parameter(DefaultValue = 25, MinValue = 5)] public int Spacing { get; set; } [Parameter(DefaultValue = 10, MinValue = 2)] public int HowMuchPositions { get; set; } [Parameter("TakeProfitPips", DefaultValue = 0, MinValue = 0)] public int TP { get; set; } [Parameter("StopLossPips", DefaultValue = 0, MinValue = 0)] public int SL { get; set; } [Parameter(DefaultValue = 0, MinValue = 0)] public int BackStop { get; set; } [Parameter(DefaultValue = 5, MinValue = 0)] public int BackStopValue { get; set; } [Parameter(DefaultValue = 0, MinValue = 0)] public int TrailingStop { get; set; } // close when earn [Parameter(DefaultValue = 0, MinValue = 0)] public int CloseWhenEarn { get; set; } // safe deposit works when earn 100% [Parameter(DefaultValue = false)] public bool MoneyStopLossOn { get; set; } [Parameter(DefaultValue = 1000, MinValue = 100)] public int StartDeposit { get; set; } [Parameter(DefaultValue = 50, MinValue = 10, MaxValue = 100)] public int StopLossPercent { get; set; } [Parameter(DefaultValue = 2, MinValue = 1, MaxValue = 100)] public int OnWhenDepositX { get; set; } //================================================================================ // OnStart //================================================================================ protected override void OnStart() { WeekStart = Symbol.Ask; // set pending up(BUY) if (SetBUY) { for (int i = 1; i < HowMuchPositions; i++) { PlaceStopOrder(TradeType.Buy, Symbol, Volume, Symbol.Ask + Spacing * i * Symbol.PipSize); } } // set pending down(SELL) if (SetSELL) { for (int j = 1; j < HowMuchPositions; j++) { PlaceStopOrder(TradeType.Sell, Symbol, Volume, Symbol.Bid - Spacing * j * Symbol.PipSize); } } } //================================================================================ // OnTick //================================================================================ protected override void OnTick() { var netProfit = 0.0; // Take profit if (TP > 0) { foreach (var position in Positions) { // Modifing position tp if (position.TakeProfit == null) { Print("Modifying {0}", position.Id); ModifyPosition(position, position.StopLoss, GetAbsoluteTakeProfit(position, TP)); } } } // Stop loss if (SL > 0) { foreach (var position in Positions) { // Modifing position sl and tp if (position.StopLoss == null) { Print("Modifying {0}", position.Id); ModifyPosition(position, GetAbsoluteStopLoss(position, SL), position.TakeProfit); } } } foreach (var openedPosition in Positions) { netProfit += openedPosition.NetProfit; } if (MoneyStopLossOn == true) { // safe deposit works when earn 100% if (LastProfit < Account.Equity && Account.Equity > StartDeposit * OnWhenDepositX) { LastProfit = Account.Equity; } if (Account.Equity > StartDeposit * 3) { //StopLossPercent = 90; } StopMoneyLoss = LastProfit * (StopLossPercent * 0.01); Print("LastProfit: ", LastProfit); Print("Equity: ", Account.Equity); Print("StopLossMoney: ", StopMoneyLoss); if (Account.Equity < StopMoneyLoss) { //open orders foreach (var openedPosition in Positions) { ClosePosition(openedPosition); } // pending orders foreach (var order in PendingOrders) { CancelPendingOrder(order); } Stop(); } } if (netProfit >= CloseWhenEarn && CloseWhenEarn > 0) { // open orders foreach (var openedPosition in Positions) { ClosePosition(openedPosition); } // pending orders foreach (var order in PendingOrders) { CancelPendingOrder(order); } Stop(); } //===================================================== Back Trailing if (BackStop > 0) { foreach (var openedPosition in Positions) { Position Position = openedPosition; if (Position.TradeType == TradeType.Buy) { double distance = Symbol.Bid - Position.EntryPrice; if (distance >= BackStop * Symbol.PipSize) { double newStopLossPrice = Math.Round(Symbol.Bid - BackStopValue * Symbol.PipSize, Symbol.Digits); if (Position.StopLoss == null) { ModifyPosition(Position, newStopLossPrice, Position.TakeProfit); } } } else { double distance = Position.EntryPrice - Symbol.Ask; if (distance >= BackStop * Symbol.PipSize) { double newStopLossPrice = Math.Round(Symbol.Ask + BackStopValue * Symbol.PipSize, Symbol.Digits); if (Position.StopLoss == null) { ModifyPosition(Position, newStopLossPrice, Position.TakeProfit); } } } } } //===================================================== Trailing if (TrailingStop > 0 && BackStop == 0) { foreach (var openedPosition in Positions) { Position Position = openedPosition; if (Position.TradeType == TradeType.Buy) { double distance = Symbol.Bid - Position.EntryPrice; if (distance >= TrailingStop * Symbol.PipSize) { double newStopLossPrice = Math.Round(Symbol.Bid - TrailingStop * Symbol.PipSize, Symbol.Digits); if (Position.StopLoss == null || newStopLossPrice > Position.StopLoss) { ModifyPosition(Position, newStopLossPrice, Position.TakeProfit); } } } else { double distance = Position.EntryPrice - Symbol.Ask; if (distance >= TrailingStop * Symbol.PipSize) { double newStopLossPrice = Math.Round(Symbol.Ask + TrailingStop * Symbol.PipSize, Symbol.Digits); if (Position.StopLoss == null || newStopLossPrice < Position.StopLoss) { ModifyPosition(Position, newStopLossPrice, Position.TakeProfit); } } } } } } //================================================================================ // OnPositionOpened //================================================================================ protected override void OnPositionOpened(Position openedPosition) { int BuyPos = 0; int SellPos = 0; foreach (var position in Positions) { // Count opened positions if (position.TradeType == TradeType.Buy) { BuyPos = BuyPos + 1; } if (position.TradeType == TradeType.Sell) { SellPos = SellPos + 1; } } Print("All Buy positions: " + BuyPos); Print("All Sell positions: " + SellPos); } // end OnPositionOpened //================================================================================ // OnBar //================================================================================ protected override void OnBar() { } //================================================================================ // OnPositionClosed //================================================================================ protected override void OnPositionClosed(Position closedPosition) { } //================================================================================ // OnStop //================================================================================ protected override void OnStop() { } //================================================================================ // Function //================================================================================ private void Buy() { ExecuteMarketOrder(TradeType.Buy, Symbol, 10000, "", 1000, 1000); } private void Sell() { ExecuteMarketOrder(TradeType.Sell, Symbol, 10000, "", 1000, 1000); } private void ClosePosition() { if (_position != null) { ClosePosition(_position); _position = null; } } //================================================================================ // modify SL and TP //================================================================================ private double GetAbsoluteStopLoss(Position position, int stopLossInPips) { //Symbol Symbol = MarketData.GetSymbol(position.SymbolCode); return position.TradeType == TradeType.Buy ? position.EntryPrice - (Symbol.PipSize * stopLossInPips) : position.EntryPrice + (Symbol.PipSize * stopLossInPips); } private double GetAbsoluteTakeProfit(Position position, int takeProfitInPips) { //Symbol Symbol = MarketData.GetSymbol(position.SymbolCode); return position.TradeType == TradeType.Buy ? position.EntryPrice + (Symbol.PipSize * takeProfitInPips) : position.EntryPrice - (Symbol.PipSize * takeProfitInPips); } //================================================================================ // Robot end //================================================================================ } }
Bye.
@breakermind
breakermind
07 Jul 2014, 13:29
( Updated at: 21 Dec 2023, 09:20 )
The End
2 days 50 positions:]
@breakermind
breakermind
11 Jul 2014, 12:17
( Updated at: 21 Dec 2023, 09:20 )
:]
Week 30/06/2014 - 04/07/2014:
Week 07/07/2014 - 10/07/2014:
@breakermind
breakermind
11 Jul 2014, 13:15
( Updated at: 21 Dec 2023, 09:20 )
Cool indicator
Indicator (when start cBot):
M15(strdDev: 1.8, Period 2000, degree: 3) only PRC, SQH, SQL
Regards
@breakermind
breakermind
14 Jul 2014, 13:06
RE: RE: RE: Cool indicator
Hi,
is there a better way to make money with cBot?
because I do not know whether should I fight with the calgo?
Thanks and Regards
@breakermind
breakermind
14 Jul 2014, 22:14
( Updated at: 23 Jan 2024, 13:11 )
Fire cBot
Hi,
FIre cBot (300 Pips for luck)
[/algos/cbots/show/521]
//================================================================================ // SimpleWeekRobo // Start at Week start(Day start 00:00) // (M15-M30 regression(2000,2,3)(1.8,2,2000)) // 100Pips levels, suport or resistance // Simple Monday-Friday script without any security with close when earn // If CloseWhenEarn == 0 then dont close positions // If TP or SL == 0 dont modifing positions // If TrailingStop > 0 ==> BackStop == 0 // Multi positions yes or no //================================================================================ using System; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Requests; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)] public class Fire : Robot { //================================================================================ // Parametrs //================================================================================ private Position _position; private double WeekStart; private double LastProfit = 0; private double StopMoneyLoss = 0; [Parameter(DefaultValue = true)] public bool SetBUY { get; set; } [Parameter(DefaultValue = true)] public bool SetSELL { get; set; } [Parameter(DefaultValue = 300000, MinValue = 1000)] public int Volume { get; set; } [Parameter(DefaultValue = true)] public bool MultiVolume { get; set; } [Parameter(DefaultValue = 10, MinValue = 5)] public int Spacing { get; set; } [Parameter(DefaultValue = 10, MinValue = 2)] public int HowMuchPositions { get; set; } [Parameter("TakeProfitPips", DefaultValue = 0, MinValue = 0)] public int TP { get; set; } [Parameter("StopLossPips", DefaultValue = 0, MinValue = 0)] public int SL { get; set; } [Parameter(DefaultValue = 0, MinValue = 0)] public int BackStop { get; set; } [Parameter(DefaultValue = 5, MinValue = 0)] public int BackStopValue { get; set; } [Parameter(DefaultValue = 0, MinValue = 0)] public int TrailingStop { get; set; } // close when earn [Parameter(DefaultValue = 0, MinValue = 0)] public int CloseWhenEarn { get; set; } // safe deposit works when earn 100% [Parameter(DefaultValue = false)] public bool MoneyStopLossOn { get; set; } [Parameter(DefaultValue = 1000, MinValue = 100)] public int StartDeposit { get; set; } [Parameter(DefaultValue = 50, MinValue = 10, MaxValue = 100)] public int StopLossPercent { get; set; } [Parameter(DefaultValue = 2, MinValue = 1, MaxValue = 100)] public int OnWhenDepositX { get; set; } private int Multi = 0; private int VolumeBuy = 0; private int VolumeSell = 0; //================================================================================ // OnStart //================================================================================ protected override void OnStart() { VolumeSell = Volume; VolumeBuy = Volume; WeekStart = Symbol.Ask; // set pending up(BUY) if (SetBUY) { for (int i = 1; i < HowMuchPositions; i++) { Multi = Multi + Spacing; if (MultiVolume == true) { if (Multi > 100) { VolumeBuy = VolumeBuy + Volume; } if (Multi > 200) { VolumeBuy = VolumeBuy + Volume; } if (Multi > 300) { VolumeBuy = VolumeBuy + Volume; } } PlaceStopOrder(TradeType.Buy, Symbol, VolumeBuy, Symbol.Ask + Spacing * i * Symbol.PipSize); } } // set pending down(SELL) if (SetSELL) { Multi = 0; for (int j = 1; j < HowMuchPositions; j++) { Multi = Multi + Spacing; if (MultiVolume == true) { if (Multi > 100) { VolumeBuy = VolumeSell + Volume; } if (Multi > 200) { VolumeBuy = VolumeSell + Volume; } if (Multi > 300) { VolumeBuy = VolumeSell + Volume; } } PlaceStopOrder(TradeType.Sell, Symbol, VolumeSell, Symbol.Bid - Spacing * j * Symbol.PipSize); } } } //================================================================================ // OnTick //================================================================================ protected override void OnTick() { var netProfit = 0.0; // Take profit if (TP > 0) { foreach (var position in Positions) { // Modifing position tp if (position.TakeProfit == null) { Print("Modifying {0}", position.Id); ModifyPosition(position, position.StopLoss, GetAbsoluteTakeProfit(position, TP)); } } } // Stop loss if (SL > 0) { foreach (var position in Positions) { // Modifing position sl and tp if (position.StopLoss == null) { Print("Modifying {0}", position.Id); ModifyPosition(position, GetAbsoluteStopLoss(position, SL), position.TakeProfit); } } } foreach (var openedPosition in Positions) { netProfit += openedPosition.NetProfit; } if (MoneyStopLossOn == true) { // safe deposit works when earn 100% if (LastProfit < Account.Equity && Account.Equity > StartDeposit * OnWhenDepositX) { LastProfit = Account.Equity; } if (Account.Equity > StartDeposit * 3) { //StopLossPercent = 90; } StopMoneyLoss = LastProfit * (StopLossPercent * 0.01); Print("LastProfit: ", LastProfit); Print("Equity: ", Account.Equity); Print("StopLossMoney: ", StopMoneyLoss); if (Account.Equity < StopMoneyLoss) { //open orders foreach (var openedPosition in Positions) { ClosePosition(openedPosition); } // pending orders foreach (var order in PendingOrders) { CancelPendingOrder(order); } Stop(); } } if (netProfit >= CloseWhenEarn && CloseWhenEarn > 0) { // open orders foreach (var openedPosition in Positions) { ClosePosition(openedPosition); } // pending orders foreach (var order in PendingOrders) { CancelPendingOrder(order); } Stop(); } //===================================================== Back Trailing if (BackStop > 0) { foreach (var openedPosition in Positions) { Position Position = openedPosition; if (Position.TradeType == TradeType.Buy) { double distance = Symbol.Bid - Position.EntryPrice; if (distance >= BackStop * Symbol.PipSize) { double newStopLossPrice = Math.Round(Symbol.Bid - BackStopValue * Symbol.PipSize, Symbol.Digits); if (Position.StopLoss == null) { ModifyPosition(Position, newStopLossPrice, Position.TakeProfit); } } } else { double distance = Position.EntryPrice - Symbol.Ask; if (distance >= BackStop * Symbol.PipSize) { double newStopLossPrice = Math.Round(Symbol.Ask + BackStopValue * Symbol.PipSize, Symbol.Digits); if (Position.StopLoss == null) { ModifyPosition(Position, newStopLossPrice, Position.TakeProfit); } } } } } //===================================================== Trailing if (TrailingStop > 0 && BackStop == 0) { foreach (var openedPosition in Positions) { Position Position = openedPosition; if (Position.TradeType == TradeType.Buy) { double distance = Symbol.Bid - Position.EntryPrice; if (distance >= TrailingStop * Symbol.PipSize) { double newStopLossPrice = Math.Round(Symbol.Bid - TrailingStop * Symbol.PipSize, Symbol.Digits); if (Position.StopLoss == null || newStopLossPrice > Position.StopLoss) { ModifyPosition(Position, newStopLossPrice, Position.TakeProfit); } } } else { double distance = Position.EntryPrice - Symbol.Ask; if (distance >= TrailingStop * Symbol.PipSize) { double newStopLossPrice = Math.Round(Symbol.Ask + TrailingStop * Symbol.PipSize, Symbol.Digits); if (Position.StopLoss == null || newStopLossPrice < Position.StopLoss) { ModifyPosition(Position, newStopLossPrice, Position.TakeProfit); } } } } } } //================================================================================ // OnPositionOpened //================================================================================ protected override void OnPositionOpened(Position openedPosition) { int BuyPos = 0; int SellPos = 0; foreach (var position in Positions) { // Count opened positions if (position.TradeType == TradeType.Buy) { BuyPos = BuyPos + 1; } if (position.TradeType == TradeType.Sell) { SellPos = SellPos + 1; } } Print("All Buy positions: " + BuyPos); Print("All Sell positions: " + SellPos); } // end OnPositionOpened //================================================================================ // OnBar //================================================================================ protected override void OnBar() { } //================================================================================ // OnPositionClosed //================================================================================ protected override void OnPositionClosed(Position closedPosition) { } //================================================================================ // OnStop //================================================================================ protected override void OnStop() { } //================================================================================ // Function //================================================================================ private void Buy() { ExecuteMarketOrder(TradeType.Buy, Symbol, 10000, "", 1000, 1000); } private void Sell() { ExecuteMarketOrder(TradeType.Sell, Symbol, 10000, "", 1000, 1000); } private void ClosePosition() { if (_position != null) { ClosePosition(_position); _position = null; } } //================================================================================ // modify SL and TP //================================================================================ private double GetAbsoluteStopLoss(Position position, int stopLossInPips) { //Symbol Symbol = MarketData.GetSymbol(position.SymbolCode); return position.TradeType == TradeType.Buy ? position.EntryPrice - (Symbol.PipSize * stopLossInPips) : position.EntryPrice + (Symbol.PipSize * stopLossInPips); } private double GetAbsoluteTakeProfit(Position position, int takeProfitInPips) { //Symbol Symbol = MarketData.GetSymbol(position.SymbolCode); return position.TradeType == TradeType.Buy ? position.EntryPrice + (Symbol.PipSize * takeProfitInPips) : position.EntryPrice - (Symbol.PipSize * takeProfitInPips); } //================================================================================ // Robot end //================================================================================ } }
Regards
@breakermind
tynamite
21 Jan 2015, 10:14
Cbot FireBot
Hi Breakermind,
I see an alternative idea for this cbot, not considering the weekly open and end price.
For example, your presumption would be that the price of a currency-pair goes down or up to a specific price-level,
it would be cool to set this end-price as data for this cbot and it opens several trades for xy pips and starts
again if the price comes back in between. The cbots stops when your presumption is fulfilled and the price set
in the cbot is reached.
Hope you understand what I want to say- it is a kind of grid-system for a predefined price-area.
Greetings !
@tynamite
mindbreaker
21 Jan 2015, 13:57
RE: Cbot FireBot
Hi,
Deposit 100$ one position in week 0.01 on week open price => 25% per week (on GBPJPY, GBPNZD ...)
it is good profit for me if You need more (its a lot of money in the year):
Learn and write something more meaningful and not coming up with.
Such wises there is a lot but nobody has written a reasonable solution.
Nice day.
@mindbreaker
cjdduarte
22 Jan 2015, 12:48
Could detail the strategy of this robot (FIREBOT) and parameters?
I have had consistent results on backtest at various periods, but did not understand what it does right, which I'm afraid to use it.
@cjdduarte
cjdduarte
22 Jan 2015, 15:49
RE:
Answer in FIREBOT forum.
Thank's
cjdduarte said:
Could detail the strategy of this robot (FIREBOT) and parameters?
I have had consistent results on backtest at various periods, but did not understand what it does right, which I'm afraid to use it.
@cjdduarte
mindbreaker
04 Feb 2015, 11:53
cBots - Indicators
Hi,
cBots and Indicators(100PipsEnvelope, Regression2000, FireBot ... ):
https://github.com/breakermind/cAlgoRobotsIndicators
Bye
@mindbreaker
breakermind
17 Aug 2013, 16:52 ( Updated at: 21 Dec 2023, 09:20 )
RE:
breakermind said:
Earn in one week
but is only test version.
Bye
@breakermind