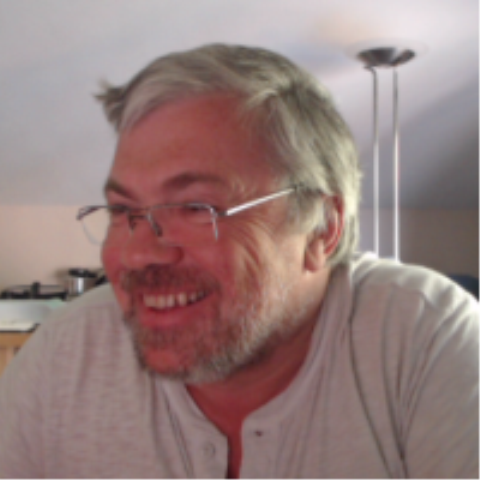
Finding lowest high in dictionary
02 Nov 2018, 13:05
Dear Panagiotis, hi!
This much I was able to achieve:
But I still cannot find programmaticaly the closest upper price (or lover) even if print them on a screen
please, find the code
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
using System.Collections.Generic;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.RussianStandardTime, AccessRights = AccessRights.FullAccess)]
public class SandDTrader3 : Robot
{
[Parameter("Source")]
public DataSeries Source { get; set; }
[Parameter("LookBackDays", DefaultValue = 800)]
public int LookBackDays { get; set; }
private Dictionary<int, double> highsDict = new Dictionary<int, double>();
private int index, _index;
private double high, low, value, _high, _low;
private Colors color;
protected override void OnStart()
{
_index = MarketSeries.Close.Count;
for (int k = 1; k < 100; k++)
{
high = MarketSeries.High.Last(0);
low = MarketSeries.Low.Last(0);
for (int i = 0; i < LookBackDays + 1 - k; i++)
{
if (MarketSeries.High.Last(i) > high)
{
high = value = MarketSeries.High.Last(i);
index = i;
}
if (MarketSeries.Low.Last(i) < low)
{
low = value = MarketSeries.Low.Last(i);
index = i;
}
else
{
continue;
}
}
LookBackDays = index - 1;
try
{
highsDict.Add(index, value);
} catch (ArgumentException)
{
Print("An element with Key = {0} already exists.", index);
}
var _high = highsDict.OrderByDescending(v => v.Value).First();
foreach (var v in highsDict.OrderByDescending(x => x.Value).Take(100))
{
_high = v.Value < _high && v.Value>MarketSeries.Close.Last(0) ? _high = v.Value: _high;
color = (v.Value < MarketSeries.Close.Last(0) ? color = Colors.Red : Colors.Aqua);
Print("Index: " + v.Key + " Value: " + v.Value + " lookBackDays: " + LookBackDays);
ChartObjects.DrawLine("High" + v.Key, MarketSeries.OpenTime.Last(v.Key), v.Value, MarketSeries.OpenTime.Last(0), v.Value, color, 1, LineStyle.Solid);
ChartObjects.DrawText(v.Key.ToString(), "Price = " + v.Value, _index , v.Value, VerticalAlignment.Top, HorizontalAlignment.Right, Colors.DarkGray);
}
}
}
}
}
Replies
... Deleted by UFO ...
PanagiotisCharalampous
02 Nov 2018, 14:43
Hi Alexander,
The code you posted does not build. What help do you need? To fix the error?
Best Regards,
Panagiotis
@PanagiotisCharalampous
alexander.n.fedorov
02 Nov 2018, 14:47
Thank you for reply, Panagiotis!
The code will bild if you take away the underscored row
That will give me the picture I posted
but I was trying to find it programmatically out of dictionary, and so far I could not
If you can, please help find programmatically the first price above (on a blue line) and below (on a red line)
Regards,
Alexander
@alexander.n.fedorov
alexander.n.fedorov
02 Nov 2018, 15:13
highsDict.Where(x => x.Value > MarketSeries.Close.Last(0)).OrderByDescending(x => x.Value).Last();
This one builds, but I do not know what to do with it. I need to find value (1.155)
I am not even concerned about the key
@alexander.n.fedorov
PanagiotisCharalampous
02 Nov 2018, 15:19
Hi Alexander,
Can you please confirm that what you are looking for is the highest and lowest values for a number of bars? It is hard to understand from the code what are you trying to do.
Best Regards,
Panagiotis
@PanagiotisCharalampous
alexander.n.fedorov
02 Nov 2018, 15:25
Current price is (bid or ask - does not matter)
The blue line gives you the price (which is written on a chart) above the current price
The red line gives you the price below.
I am tryiing to find them programmatically, as well as there keys, as the are a part of dictionary
@alexander.n.fedorov
alexander.n.fedorov
02 Nov 2018, 15:28
When I look at the chart, I know the prices. The the cBot does not
I have to tell him some how
@alexander.n.fedorov
alexander.n.fedorov
02 Nov 2018, 15:29
RE:
alexander.n.fedorov said:
When I look at the chart, I know the prices. The the cBot does not
I have to tell him some how
The first blue and the first red
@alexander.n.fedorov
PanagiotisCharalampous
02 Nov 2018, 15:39
Is it this what you need?
using cAlgo.API; using cAlgo.API.Internals; using System; using System.Collections.Generic; using System.Linq; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.RussianStandardTime, AccessRights = AccessRights.FullAccess)] public class SandDTrader3 : Robot { [Parameter("Source")] public DataSeries Source { get; set; } [Parameter("LookBackDays", DefaultValue = 800)] public int Bars { get; set; } private Dictionary<int, double> highsDict = new Dictionary<int, double>(); private Dictionary<int, double> lowsDict = new Dictionary<int, double>(); private int index, _index; private double high, low, value, _high, _low; private Colors color; protected override void OnStart() { for (int k = 1; k < Bars; k++) { highsDict.Add(k, MarketSeries.High.Last(k)); lowsDict.Add(k, MarketSeries.Low.Last(k)); } var high = highsDict.OrderByDescending(x => x.Value).First(); ChartObjects.DrawLine("High" + high.Key, MarketSeries.OpenTime.Last(high.Key), high.Value, MarketSeries.OpenTime.Last(0), high.Value, Colors.Aqua, 1, LineStyle.Solid); ChartObjects.DrawText(high.Key.ToString(), "Price = " + high.Value, _index, high.Value, VerticalAlignment.Top, HorizontalAlignment.Right, Colors.DarkGray); var low = lowsDict.OrderBy(x => x.Value).First(); ChartObjects.DrawLine("Low" + low.Key, MarketSeries.OpenTime.Last(low.Key), low.Value, MarketSeries.OpenTime.Last(0), low.Value, Colors.Red, 1, LineStyle.Solid); ChartObjects.DrawText(low.Key.ToString(), "Price = " + low.Value, _index, low.Value, VerticalAlignment.Top, HorizontalAlignment.Right, Colors.DarkGray); } } }
Best Regards,
Panagiotis
@PanagiotisCharalampous
alexander.n.fedorov
02 Nov 2018, 15:48
RE:
Panagiotis Charalampous said:
Is it this what you need?
using cAlgo.API; using cAlgo.API.Internals; using System; using System.Collections.Generic; using System.Linq; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.RussianStandardTime, AccessRights = AccessRights.FullAccess)] public class SandDTrader3 : Robot { [Parameter("Source")] public DataSeries Source { get; set; } [Parameter("LookBackDays", DefaultValue = 800)] public int Bars { get; set; } private Dictionary<int, double> highsDict = new Dictionary<int, double>(); private Dictionary<int, double> lowsDict = new Dictionary<int, double>(); private int index, _index; private double high, low, value, _high, _low; private Colors color; protected override void OnStart() { for (int k = 1; k < Bars; k++) { highsDict.Add(k, MarketSeries.High.Last(k)); lowsDict.Add(k, MarketSeries.Low.Last(k)); } var high = highsDict.OrderByDescending(x => x.Value).First(); ChartObjects.DrawLine("High" + high.Key, MarketSeries.OpenTime.Last(high.Key), high.Value, MarketSeries.OpenTime.Last(0), high.Value, Colors.Aqua, 1, LineStyle.Solid); ChartObjects.DrawText(high.Key.ToString(), "Price = " + high.Value, _index, high.Value, VerticalAlignment.Top, HorizontalAlignment.Right, Colors.DarkGray); var low = lowsDict.OrderBy(x => x.Value).First(); ChartObjects.DrawLine("Low" + low.Key, MarketSeries.OpenTime.Last(low.Key), low.Value, MarketSeries.OpenTime.Last(0), low.Value, Colors.Red, 1, LineStyle.Solid); ChartObjects.DrawText(low.Key.ToString(), "Price = " + low.Value, _index, low.Value, VerticalAlignment.Top, HorizontalAlignment.Right, Colors.DarkGray); } } }Best Regards,
Panagiotis
I tried. It is not what I need
I will have to do mo homework, myabe then I write again
Regards
Alexander
@alexander.n.fedorov
alexander.n.fedorov
04 Nov 2018, 11:25
( Updated at: 21 Dec 2023, 09:20 )
I solved the issue
The screen looks like that :
@alexander.n.fedorov
alexander.n.fedorov
04 Nov 2018, 11:28
A little bit too congested, still helpfull,
And it works only on 3.3
The next quesion of mine is in my next thread.
As far as this on is concerned it is that: will it be difficult to convert to indicator?
And how can I achieve transparancy on the yellow cubes?
Regards,
Alexander
@alexander.n.fedorov
PanagiotisCharalampous
05 Nov 2018, 10:43
Hi Alexander,
It should be easy to convert it to an Indicator. Regarding the trasparency, you can use FromARGB and adjust the alpha of your color.
Best Regards,
Panagiotis
@PanagiotisCharalampous
alexander.n.fedorov
05 Nov 2018, 10:52
Well, thank you again. There is so much to learn! Will never have enough time.
I made a cBot from this
What it does, it tries to locate Demand & Suplly zones, the ones where the institutions are trading.
Then I start the bot and with hands place pending order, stoploss & takeprofit.
The bot substitutes hard stops with bot line (OnTimer())
And if I have to stop the bot it updates the order (or the position by then) with an SL and TP
Could be interesting
If it will be, I'll let you know
Regards,
Sasha
@alexander.n.fedorov
alexander.n.fedorov
02 Nov 2018, 13:06 ( Updated at: 21 Dec 2023, 09:20 )
RE:
alexander.n.fedorov said:
Please , help
Regards,
Alexander
@alexander.n.fedorov