Ichimoku cBot with Triling Stop
Created at 21 Jun 2018, 11:15
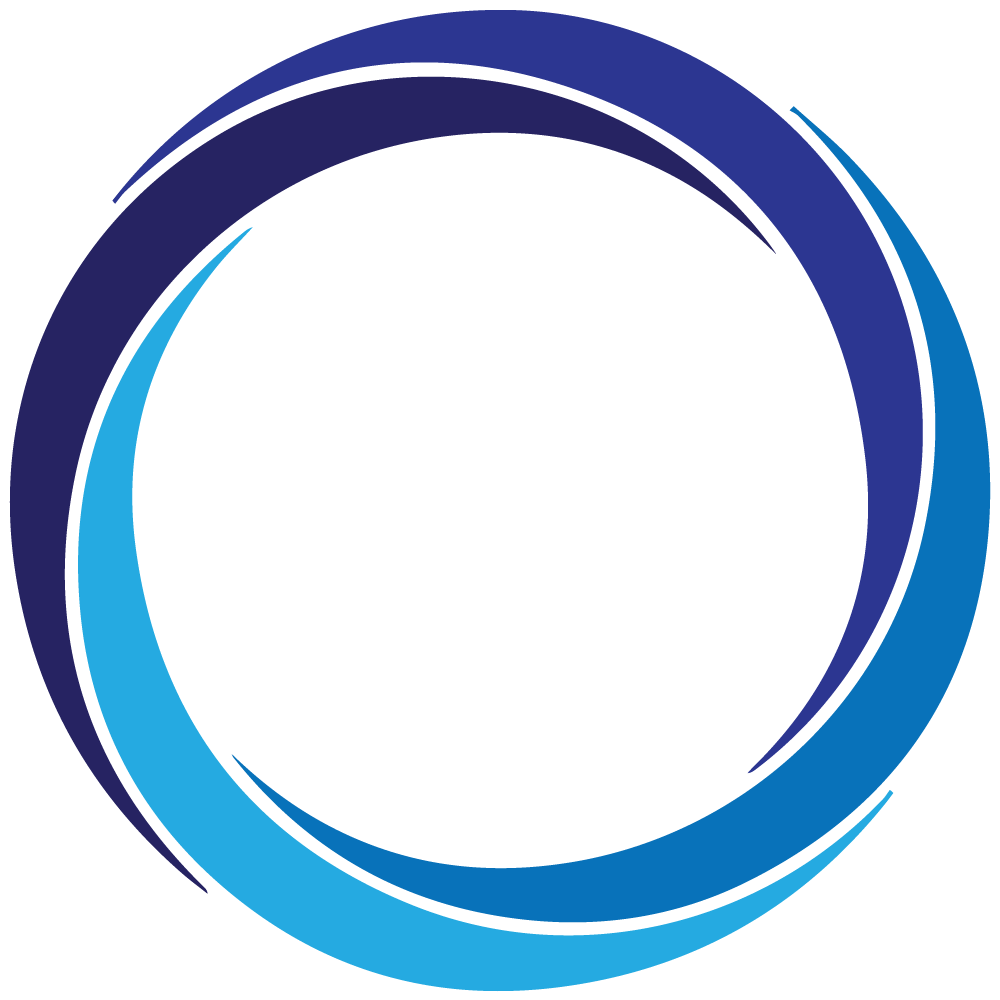
Ichimoku cBot with Triling Stop
21 Jun 2018, 11:15
Hi Guys,
Looking for som help. i am tryin gto add a trailing stop to my cBot. it was backtesting before, but now it is no longer placing any trades. Could someone please have a glance over and see what the problem is?
using System; using cAlgo.API; using cAlgo.API.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class IchimokucBotTrailingStopLoss : Robot { //Ichimoku Settings - NOT BE BE CHANGED UNLESS YOU KNOW WHAT YOU ARE DOING [Parameter(DefaultValue = 9)] private int periodFast { get; set; } [Parameter(DefaultValue = 26)] private int periodMedium { get; set; } [Parameter(DefaultValue = 52)] private int periodSlow { get; set; } // //Default Pip Value [Parameter(" Volume Size", DefaultValue = 1000)] public int Volume { get; set; } // //Stop Losses and Take Profit in Pip Value [Parameter("Stop Loss", DefaultValue = 20)] public int StopLossPips { get; set; } [Parameter("Take Profit", DefaultValue = 25)] public int TakeProfitPips { get; set; } // //Trailing Stop Loss Settings [Parameter("Trigger When Gaining", DefaultValue = 1)] public double TriggerWhenGaining { get; set; } [Parameter("Trailing Stop Loss Distance", DefaultValue = 1)] public double TrailingStopLossDistance { get; set; } private double _highestGain; private bool _isTrailing; // IchimokuKinkoHyo ichimoku; protected override void OnStart() { ichimoku = Indicators.IchimokuKinkoHyo(periodFast, periodMedium, periodSlow); } protected override void OnBar() { var positionsBuy = Positions.FindAll("Ichimoku cBot"); var positionsSell = Positions.FindAll("Ichimoku cBot"); var distanceFromUpKumo = (Symbol.Bid - ichimoku.SenkouSpanA.Last(26)) / Symbol.PipSize; var distanceFromDownKumo = (ichimoku.SenkouSpanA.Last(26) - Symbol.Ask) / Symbol.PipSize; if (positionsBuy.Length == 0 && positionsSell.Length == 0) { if (MarketSeries.Open.Last(1) <= ichimoku.SenkouSpanA.Last(27) && MarketSeries.Open.Last(1) > ichimoku.SenkouSpanB.Last(27)) { if (MarketSeries.Close.Last(1) > ichimoku.SenkouSpanA.Last(27)) { if (distanceFromUpKumo <= 30) { ExecuteMarketOrder(TradeType.Buy, Symbol, Volume, "Ichimoku cBot", StopLossPips, TakeProfitPips); _highestGain = Positions[0].Pips; } } } if (MarketSeries.Open.Last(1) >= ichimoku.SenkouSpanA.Last(27) && MarketSeries.Open.Last(1) < ichimoku.SenkouSpanB.Last(27)) { if (MarketSeries.Close.Last(1) < ichimoku.SenkouSpanA.Last(27)) { if (distanceFromDownKumo <= 30) { ExecuteMarketOrder(TradeType.Sell, Symbol, Volume, "Ichimoku cBot", StopLossPips, TakeProfitPips); _highestGain = Positions[0].Pips; } } } } } protected override void OnTick() { var position = Positions.Find("Ichimoku cBot"); if (position == null) { return; } //If the trigger is reached, the robot starts trailing if (!_isTrailing && position.Pips >= TriggerWhenGaining) { _isTrailing = true; } //If the cBot is trailing and the profit in pips is at the highest level, we need to readjust the stop loss if (_isTrailing && _highestGain < position.Pips) { //Based on the position's direction, we calculate the new stop loss price and we modify the position if (position.TradeType == TradeType.Buy) { var newSLprice = Symbol.Ask - (Symbol.PipSize * TrailingStopLossDistance); if (newSLprice > position.StopLoss) { ModifyPosition(position, newSLprice, null); } } else { var newSLprice = Symbol.Bid + (Symbol.PipSize * TrailingStopLossDistance); if (newSLprice < position.StopLoss) { ModifyPosition(position, newSLprice, null); } } if (position.TradeType == TradeType.Sell) { var newSLprice = Symbol.Ask + (Symbol.PipSize * TrailingStopLossDistance); if (newSLprice < position.StopLoss) { ModifyPosition(position, newSLprice, null); } } else { var newSLprice = Symbol.Bid - (Symbol.PipSize * TrailingStopLossDistance); if (newSLprice > position.StopLoss) { ModifyPosition(position, newSLprice, null); } } //We reset the highest gain _highestGain = position.Pips; } } } }
PanagiotisCharalampous
21 Jun 2018, 11:30
Hi sean.n.long,
Thanks for posting in our forum. Note that trailing stop loss is now offered by cAlgo.API, therefore you do not need to program it yourself anymore. Order functions now feature a trailing stop loss parameter. See below
https://ctdn.com/api/reference/robot/executemarketorder
https://ctdn.com/api/reference/robot/placelimitorder
https://ctdn.com/api/reference/robot/placestoporder
https://ctdn.com/api/reference/robot/placestoplimitorder
Let me know if this helps.
Best Regards,
Panagiotis
@PanagiotisCharalampous