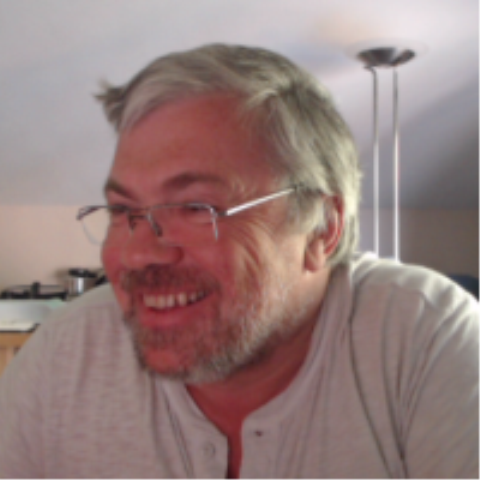
Optimization would not work , backtesting is working
26 Feb 2018, 11:01
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.RussianStandardTime, AccessRights = AccessRights.FullAccess)] public class Donci3Av7 : Robot { #region Parameters [Parameter("Instance Name", DefaultValue = "Blue")] public string InstanceName { get; set; } [Parameter()] public DataSeries Source { get; set; } [Parameter("Time Frame 2", DefaultValue = "Daily")] public TimeFrame TimeFrame2 { get; set; } [Parameter("Time Frame 3", DefaultValue = "Hour12")] public TimeFrame TimeFrame3 { get; set; } [Parameter("SL ATR multiple", DefaultValue = 2.2)] public double ATRfactor { get; set; } [Parameter("Hi Periods", DefaultValue = 20, MinValue =15, MaxValue =40, Step =1)] public int HiPer { get; set; } [Parameter("Low Periods", DefaultValue = 10, MinValue = 5, MaxValue =30, Step =1)] public int LowPer { get; set; } [Parameter("Quantity (Lots)", DefaultValue = 0.1, MinValue = 0.01, Step = 0.01)] public double Quantity { get; set; } [Parameter("Include Trading Hours", DefaultValue = false)] public bool IncludeTradinHours { get; set; } [Parameter("Start Hour", DefaultValue = 0.0, MinValue = 0.0, MaxValue = 24.0, Step = 0.5)] public double StartTime { get; set; } [Parameter("Stop Hour", DefaultValue = 24.0, MinValue = 0.0, MaxValue = 24.0, Step = 0.5)] public double StopTime { get; set; } [Parameter("Include Break-Even", DefaultValue = true)] public bool IncludeBreakEven { get; set; } [Parameter("Break-Even Trigger (SL)", DefaultValue = 1, MinValue = 0.4, MaxValue = 4.0)] public double Trigger { get; set; } [Parameter("Break-Even Extra (pips)", DefaultValue = 1.0, MinValue = 0.0)] public double ExtraPips { get; set; } [Parameter("Include Trailing Stop", DefaultValue = true)] public bool IncludeTrailingStop { get; set; } [Parameter("Trailing Stop Trigger (SL)", DefaultValue = 1.5, MinValue = 0.3, Step = 0.1)] public double TrailingStopTrigger { get; set; } [Parameter("Trailing Stop Step (SL)", DefaultValue = 1.2, MinValue = 0.1, Step = 0.1)] public double TrailingStopStep { get; set; } [Parameter("Max Spread (Pips)", DefaultValue = 2.0, MaxValue = 50.0, Step = 0.1)] public double MaxSpread { get; set; } private DateTime _startTime; private DateTime _stopTime; private double ma_Distance; private MarketSeries series2; private MarketSeries series3; private MovingAverage MA2; private MovingAverage MA3; private MovingAverage MA; private string _Blue; public double SL; private double _ATR; private DonchianChannel DCH; private DonchianChannel DCL; private AverageTrueRange ATR; private TradeType _tradetype; private bool BuyTradeOK; private bool SellTradeOK; #endregion #region OnStart protected override void OnStart() { // Start Time is the same day at 22:00:00 Server Time _startTime = Server.Time.Date.AddHours(StartTime); // Stop Time is the next day at 06:00:00 _stopTime = Server.Time.Date.AddHours(StopTime); Print("Start Time {0},", _startTime); Print("Stop Time {0},", _stopTime); ma_Distance = 0.2; ma_Distance = ma_Distance * Symbol.PipSize; series2 = MarketData.GetSeries(TimeFrame2); series3 = MarketData.GetSeries(TimeFrame3); MA = Indicators.MovingAverage(Source, HiPer, MovingAverageType.Exponential); MA2 = Indicators.MovingAverage(series2.Close, HiPer, MovingAverageType.Exponential); MA3 = Indicators.MovingAverage(series3.Close, HiPer, MovingAverageType.Exponential); _Blue = "Donci 3Av7 " + "Blue" + " " + Symbol.Code; InstanceName = _Blue; ATR = Indicators.AverageTrueRange(LowPer, MovingAverageType.Exponential); SL = ATR.Result.LastValue * ATRfactor / Symbol.PipSize; DCH = Indicators.DonchianChannel(HiPer); DCL = Indicators.DonchianChannel(LowPer); _ATR = ATR.Result.LastValue; } #endregion #region OnTick protected override void OnTick() { if (IncludeTradinHours) { var currentHours = Server.Time.TimeOfDay.TotalHours; bool tradeTime = StartTime < StopTime ? currentHours > StartTime && currentHours < StopTime : currentHours < StopTime || currentHours > StartTime; if (!tradeTime) return; } var position = Positions.Find(InstanceName, Symbol); if (position == null) { if (MA2.Result.LastValue < Symbol.Bid - ma_Distance && MA3.Result.LastValue < Symbol.Bid - ma_Distance && MA2.Result.IsRising() && MA3.Result.IsRising() && Symbol.Spread <= MaxSpread) { BuyTradeOK = true; SellTradeOK = false; } if (MA2.Result.LastValue > Symbol.Ask + ma_Distance && MA3.Result.LastValue > Symbol.Ask + ma_Distance && MA2.Result.IsFalling() && MA3.Result.IsFalling() && Symbol.Spread <= MaxSpread) { BuyTradeOK = false; SellTradeOK = true; } if ((DCH.Top.LastValue < Symbol.Bid && BuyTradeOK) || (DCH.Bottom.LastValue > Symbol.Bid && SellTradeOK)) { _tradetype = BuyTradeOK ? TradeType.Buy : TradeType.Sell; Open(_tradetype); } } else if (position != null) { if ((position.TradeType == TradeType.Buy && DCL.Bottom.LastValue > Symbol.Bid) || (position.TradeType == TradeType.Sell && DCL.Top.LastValue < Symbol.Bid)) { ClosePosition(position); } } if (IncludeBreakEven == true) GoToBreakEven(); if (IncludeTrailingStop == true) SetTrailingStop(); } #endregion #region Open trade void Open(TradeType tradeType) { var volumeInUnits = Symbol.QuantityToVolume(Quantity); SL = ATR.Result.LastValue * ATRfactor / Symbol.PipSize; ExecuteMarketOrder(tradeType, Symbol, volumeInUnits, InstanceName, SL, null); } #endregion #region Break Even void GoToBreakEven() { var position = Positions.Find(InstanceName, Symbol); if (position != null) { var entryPrice = position.EntryPrice; var distance = 0.0; var adjEntryPrice = 0.0; if (position.TradeType == TradeType.Buy) { adjEntryPrice = entryPrice + ExtraPips * Symbol.PipSize; distance = Symbol.Bid - entryPrice; } else { adjEntryPrice = entryPrice - ExtraPips * Symbol.PipSize; distance = entryPrice - Symbol.Ask; } if (distance >= Trigger * SL * Symbol.PipSize) { if (position.TradeType == TradeType.Sell && position.StopLoss > adjEntryPrice) ModifyPosition(position, adjEntryPrice, null); if (position.TradeType == TradeType.Buy && position.StopLoss < adjEntryPrice) ModifyPosition(position, adjEntryPrice, null); } } } #endregion #region Trailing Stop private void SetTrailingStop() { var sellPositions = Positions.FindAll(InstanceName, Symbol, TradeType.Sell); foreach (Position position in sellPositions) { double distance = position.EntryPrice - Symbol.Ask; if (distance < TrailingStopTrigger * SL * Symbol.PipSize) continue; double newStopLossPrice = Symbol.Ask + TrailingStopStep * SL * Symbol.PipSize; if (position.StopLoss == null || newStopLossPrice < position.StopLoss) { ModifyPosition(position, newStopLossPrice, position.TakeProfit); } } var buyPositions = Positions.FindAll(InstanceName, Symbol, TradeType.Buy); foreach (Position position in buyPositions) { double distance = Symbol.Bid - position.EntryPrice; if (distance < TrailingStopTrigger * SL * Symbol.PipSize) continue; double newStopLossPrice = Symbol.Bid - TrailingStopStep * SL * Symbol.PipSize; if (position.StopLoss == null || newStopLossPrice > position.StopLoss) { ModifyPosition(position, newStopLossPrice, position.TakeProfit); } } } #endregion } }
I will try to add the screens
Replies
alexander.n.fedorov
26 Feb 2018, 11:58
Backtesting
The funny thing is that I did not change anything on both computers, and all of a sudden optimization is woking OK now.
What could it possibly be?
@alexander.n.fedorov
PanagiotisCharalampous
26 Feb 2018, 12:21
Hi Alexander,
It is not clear what was the problem. What do you mean that optimization was not working?
Best Regards,
Panagiotis
@PanagiotisCharalampous
alexander.n.fedorov
26 Feb 2018, 12:50
Hi, Panagiotis,
it starts, and after few seconds it stops with remaining time 0 seconds
I tryied to drop the *.png screenshot files, or *.jpg, but also could not do much, as it was taking the whole screen. How do I upload a jpg file?
@alexander.n.fedorov
alexander.n.fedorov
26 Feb 2018, 12:52
Backtesting
If you could send me your skype or e-mail,
I could send you the screenshots
just in case my skype is alexander.n.fedorov
and mail is alexander.n.fedorov@gmail.com
@alexander.n.fedorov
PanagiotisCharalampous
26 Feb 2018, 12:53
( Updated at: 19 Mar 2025, 08:57 )
Hi Alexander,
There is an image button when you are creating the post, use that. Else send the screenshots to support@ctrader.com. Also sending the cBot would be helpful so that we can reproduce the problem.
Best Regards,
Panagiotis
@PanagiotisCharalampous
alexander.n.fedorov
26 Feb 2018, 12:55
Backtesting
the Bot code is up the screen
I will try the image button again
@alexander.n.fedorov
alexander.n.fedorov
26 Feb 2018, 13:16
Backtesting
Panagiotis,
I tryied to do it on two differen computers. On both it did not work
Now it is working on both.
Out of that I think it was something with a server connection.
Alexander
@alexander.n.fedorov
alexander.n.fedorov
26 Feb 2018, 11:11
How do you add the screenshots?
I did not figure out how to post the screens.
@alexander.n.fedorov