Description
Robot use the indicators RSI and ATR, with dynamics Stop and Take Profit
Money management, supports and resistance.
Symbol = EURUSD
TimeFrame = H4
TP Factor = 2.43
Volatility Factor = 2.7
MM Factor = 5 // Money Management
RSI Source = Close
RSI Period = 14
RSI Ceil = 1
ATR Period = 20
ATR MAType = VIDYA
Results :
Results = entre le 01/01/2014 et 5/8/2014 a 13h00 gain de 44559 euros(+89%).
Net profit = 47599.19 euros
Ending Equity = 47599.19 euros
The PipsATR indicator is here
Author : Abdallah HACID
#region Licence
//The MIT License (MIT)
//Copyright (c) 2014 abdallah HACID, https://www.facebook.com/ab.hacid
//Permission is hereby granted, free of charge, to any person obtaining a copy of this software
//and associated documentation files (the "Software"), to deal in the Software without restriction,
//including without limitation the rights to use, copy, modify, merge, publish, distribute,
//sublicense, and/or sell copies of the Software, and to permit persons to whom the Software
//is furnished to do so, subject to the following conditions:
//The above copyright notice and this permission notice shall be included in all copies or
//substantial portions of the Software.
//THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING
//BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
//NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM,
//DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
//OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
// Project Hosting for Open Source Software on Codeplex : https://calgobots.codeplex.com/
#endregion
#region cBot Infos
// -------------------------------------------------------------------------------
//
// RsiAtrII (5 Aout 2014)
// version 2.2014.8.5.13h00
// Author : https://www.facebook.com/ab.hacid
//
// -------------------------------------------------------------------------------
#endregion
#region cBot Parameters Comments
// Robot using the indicators RSI and ATR
//
// Symbol = EURUSD
// TimeFrame = H4
//
// TP Factor = 2.43
// Volatility Factor = 2.7
// MM Factor = 5 // Money Management
// RSI Source = Close
// RSI Period = 14
// RSI Ceil = 1
//
// ATR Period = 20
// ATR MAType = VIDYA
//
// Results :
// Results = entre le 01/01/2014 et 5/8/2014 a 13h00 gain de 44559 euros(+89%).
// Net profit = 47599.19 euros
// Ending Equity = 47599.19 euros
//
// -------------------------------------------------------------------------------
// Trading using leverage carries a high degree of risk to your capital, and it is possible to lose more than
// your initial investment. Only speculate with money you can afford to lose.
// -------------------------------------------------------------------------------
#endregion
#region advertisement
// -------------------------------------------------------------------------------
// Trading using leverage carries a high degree of risk to your capital, and it is possible to lose more than
// your initial investment. Only speculate with money you can afford to lose.
// -------------------------------------------------------------------------------
#endregion
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Lib;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC)]
public class RsiAtrII : Robot
{
#region cBot parameters
[Parameter("TP Factor", DefaultValue = 2.43, MinValue = 0.1)]
public double TPFactor { get; set; }
[Parameter("Volatility Factor", DefaultValue = 2.7, MinValue = 0.1)]
public double VolFactor { get; set; }
[Parameter("MM Factor", DefaultValue = 5, MinValue = 0.1)]
public double MMFactor { get; set; }
[Parameter("RSI Source")]
public DataSeries RsiSource { get; set; }
// Close
[Parameter("RSI Period", DefaultValue = 14, MinValue = 1)]
public int RsiPeriod { get; set; }
[Parameter("RSI Ceil", DefaultValue = 1)]
public int RsiCeil { get; set; }
[Parameter("ATR Period", DefaultValue = 20, MinValue = 1)]
public int AtrPeriod { get; set; }
[Parameter("ATR MAType", DefaultValue = 4)]
public MovingAverageType AtrMaType { get; set; }
// VIDYA
#endregion
private RelativeStrengthIndex rsi;
private PipsATRIndicator pipsATR;
// Prefix commands the robot passes
private const string botPrefix = "RSI-ATR-II";
// Label orders the robot passes
private string botLabel;
double minPipsATR;
double maxPipsATR;
double ceilSignalPipsATR;
double minRSI;
double maxRSI;
protected override void OnStart()
{
botLabel = string.Format("{0}-{1} {2}", botPrefix, Symbol.Code, TimeFrame);
rsi = Indicators.RelativeStrengthIndex(RsiSource, RsiPeriod);
pipsATR = Indicators.GetIndicator<PipsATRIndicator>(TimeFrame, AtrPeriod, AtrMaType);
minPipsATR = pipsATR.Result.Minimum(pipsATR.Result.Count);
maxPipsATR = pipsATR.Result.Maximum(pipsATR.Result.Count);
}
protected override void OnTick()
{
double volatility = pipsATR.Result.lastRealValue(0);
int minimaxPeriod = (int)((4.0 / 3.0) * volatility);
minPipsATR = Math.Min(minPipsATR, pipsATR.Result.LastValue);
maxPipsATR = Math.Max(maxPipsATR, pipsATR.Result.LastValue);
minRSI = rsi.Result.Minimum(minimaxPeriod);
maxRSI = rsi.Result.Maximum(minimaxPeriod);
ceilSignalPipsATR = minPipsATR + ((maxPipsATR - minPipsATR) / 9) * VolFactor;
if (rsi.Result.LastValue < minRSI)
this.closeAllSellPositions();
else if (rsi.Result.LastValue > maxRSI)
this.closeAllBuyPositions();
// Do nothing if daily ATR > Max allowed
if (pipsATR.Result.LastValue <= ceilSignalPipsATR)
{
if ((!(this.existBuyPositions())) && rsi.Result.HasCrossedAbove(minRSI + 1, 0))
{
double stopLoss = VolFactor * volatility;
this.closeAllSellPositions();
ExecuteMarketOrder(TradeType.Buy, Symbol, Symbol.NormalizeVolume(this.moneyManagement(MMFactor / 100, stopLoss), RoundingMode.ToNearest), this.botName(), stopLoss, TPFactor * stopLoss);
}
else if (!(this.existSellPositions()) && rsi.Result.HasCrossedBelow(maxRSI - 1, 0))
{
double stopLoss = VolFactor * volatility;
this.closeAllBuyPositions();
ExecuteMarketOrder(TradeType.Sell, Symbol, Symbol.NormalizeVolume(this.moneyManagement(MMFactor / 100, stopLoss), RoundingMode.ToNearest), this.botName(), stopLoss, TPFactor * stopLoss);
}
}
}
protected override void OnStop()
{
base.OnStop();
this.closeAllPositions();
}
}
}
aysos75
Joined on 28.09.2013
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: RsiAtr II.algo
- Rating: 0
- Installs: 5505
- Modified: 13/10/2021 09:54
Comments
Thanks Spotware but that wasn't the question asked. I'm also trying to modify the bot slightly for my own use and getting the same error RV13 got. Anyone know how to fix the "duplicate references" problem?
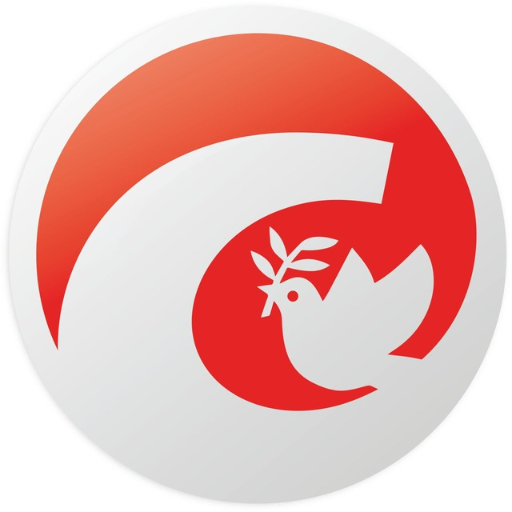
Dear Traders,
All algorithms that you download from cTDN are already compiled. Once you install cBot to the platform (by double clicking on the file) it is ready to be used.
You need to compile (build) cBot only if you made a change in the source code. If you do not change code of the algorithm you do not need to compile (build) it.
hello,
My English is not very good, I made a youtube video that explains how to compile all the robots and indicators, it is in French, but you can understand with the video without sound.
https://www.youtube.com/watch?v=BMgI88rZs7U
kind regards
But now I get
Error CS1703: An assembly with the same identity 'cAlgo.API, Version=1.0.0.0, Culture=neutral, PublicKeyToken=3499da3018340880' has already been imported. Try removing one of the duplicate references.
How to fix this?
Found a solution to calgo.lib.dll problem.
1. Go here: https://calgobots.codeplex.com/#
2. Download the zip and extract
3. Click "Manage references" next to build button in cAlgo
4. Click Libaries and then "browse"
5. Browse to extract zip\Build-.....\Solution-calgobots-.....\API where "....." is some build number
6. Untick missing "f:" dll and re-try build.
Gamezxz, I get what you mean now - but a little bit of extra help content would have been good :)
I too get
Error CS0006: Metadata file 'f:\dev\calgo\sources\library\calgolib\bin\debug\calgo.lib.dll' could not be found
And the download link at
http://sendbox.fr/pro/7oyl6oe909m8/MQ4.zip.html
Is broke...
Gamezxz, no idea what you are referring to
emeeder & Wilfried
Look at description please...
I can't find the cAlgo. Lib. What is that file and where can i get it so that i can make minor changes and save it.
Thanks
Hi Aysos,
I test this robot using a demo account and the result is good. I just have one concern, how to adjust the volume to 2000 or 5000? it seems that the volume is always opening at 1000 even if I change the MM factor. Or can you modify the code to show volume instead of MM? Thanks.