Description
This cBots dumps trendbars data to CSV file. Instructions:
- create an instance
- open backtesting tab
- choose max available date range
- backtest cBot
CSV format: time, open, high, low, close, volume
In OnStop method cBot will flush all market series data to the CSV file on your Desktop.
using System;
using System.Globalization;
using System.IO;
using System.Linq;
using System.Threading;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)]
public class DumpToCSV : Robot
{
protected override void OnStop()
{
Thread.CurrentThread.CurrentCulture = CultureInfo.InvariantCulture;
var desktopFolder = Environment.GetFolderPath(Environment.SpecialFolder.Desktop);
var folderPath = Path.Combine(desktopFolder, "trendbars");
Directory.CreateDirectory(folderPath);
var filePath = Path.Combine(folderPath, Symbol.Code + " " + TimeFrame + ".csv");
using (var writer = File.CreateText(filePath))
{
for (var i = 0; i < MarketSeries.Close.Count; i++)
{
writer.WriteLine(ConcatWithComma(MarketSeries.OpenTime[i], MarketSeries.Open[i], MarketSeries.High[i], MarketSeries.Low[i], MarketSeries.Close[i], MarketSeries.TickVolume[i]));
}
}
}
private string ConcatWithComma(params object[] parameters)
{
return string.Join(",", parameters.Select(p => p.ToString()));
}
}
}
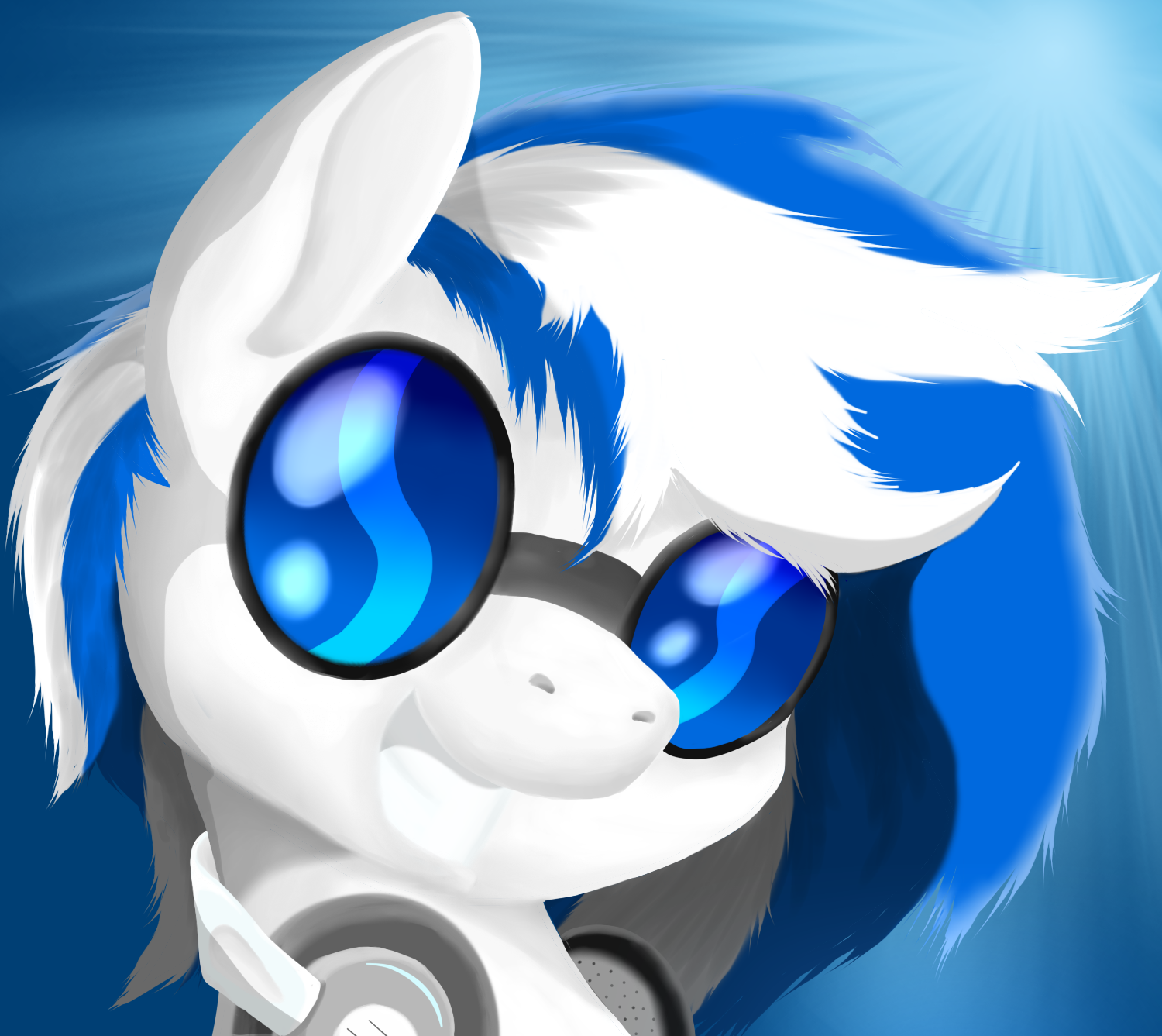
modarkat
Joined on 25.12.2013
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: DumpToCSV.algo
- Rating: 5
- Installs: 3713
- Modified: 13/10/2021 09:54
Warning! Running cBots downloaded from this section may lead to financial losses. Use them at your own risk.
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
JO
See: /algos/cbots/show/588 and /algos/cbots/show/591
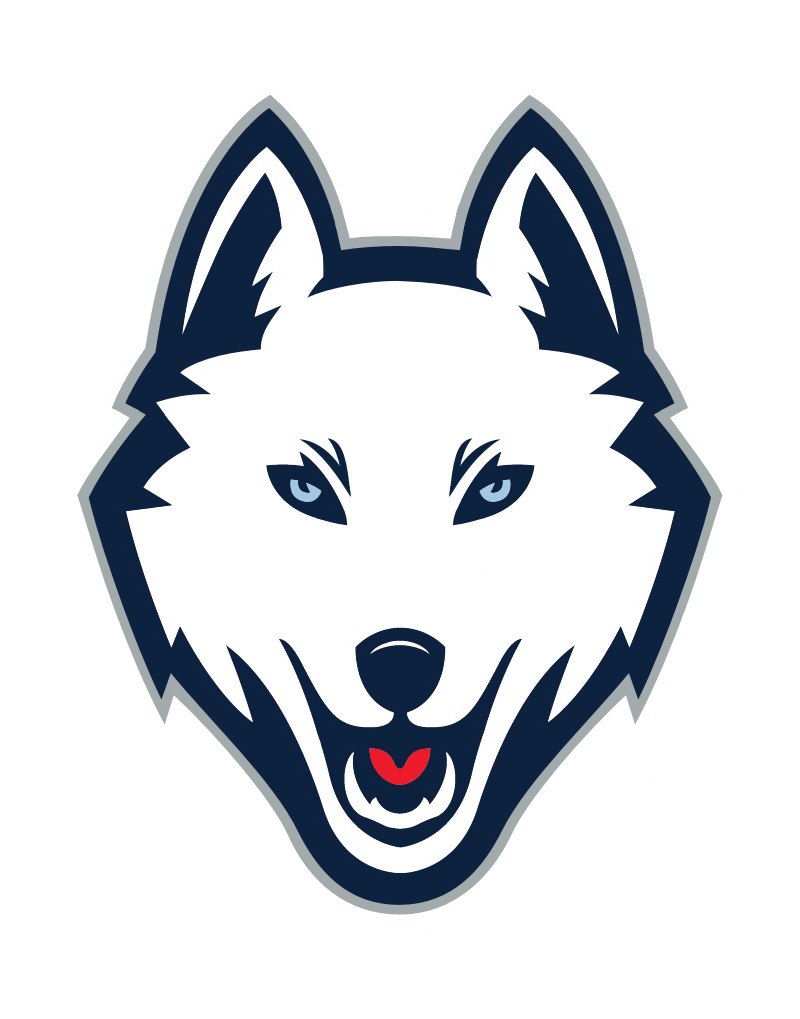
Hi @modarkat
may y have a version of saving a few currencys and a few intervals in one cbot ?
like
each min
m1(gbpjpy,gbpaud...) file gbpjpy_m1.csv ...
each 5min
m5(gbpjpy,gbpaud...) file gbpjpy_m5.csv ...
each 15min
...
it would be nice :]
Regards
Hi,
I need a simple cBOT. Can you please check the link with the description Payment will be made through SKRILL.
http://ctrader.com/jobs/252
thank you
Saleem Khan