Description
The Rsi 1.0
The Rsi 1.0 is a new way to interpret RSI values.
RSI Trend:
- The values are calculated based on the Highs and Lows, not just on a single value (High, Low, Close, etc.). When the RSI close price is above 50, it displays the High value; conversely, when the RSI is below 50, it displays the Low value.
- A smoothing option is added to smooth out the values.
- A signal option is added, because having a signal on the RSI is always fun =)
RSI Accurate (Innovation):
The calculation of this RSI allows for changing the period parameters. Each time the RSI crosses a line from the "Start Accurate Level on RSI Trend" menu, it increments the number of bars and transforms it into a period, which enables it to have a different calculation parameter for each bar.
- The values are calculated based on the Highs and Lows, not just on a single value (High, Low, Close, etc.). When the RSI close price is above 50, it displays the High value; conversely, when the RSI is below 50, it displays the Low value.
- A smoothing option is added to smooth out the values.
- A signal option is added, because having a signal on the RSI is always fun =)
Signal Fun ? :
Buy = when the RSI is green and crosses above the green line.
Sell = when the RSI is red and crosses below the red line.
Please don't say Thx, that it's great etc.. What would really make me happy is if you could tell me what we need to do to make version 2.0 exceptional. <3
Enjoy for Free =)
Previous account here : https://ctrader.com/users/profile/70920
Contact telegram : https://t.me/nimi012
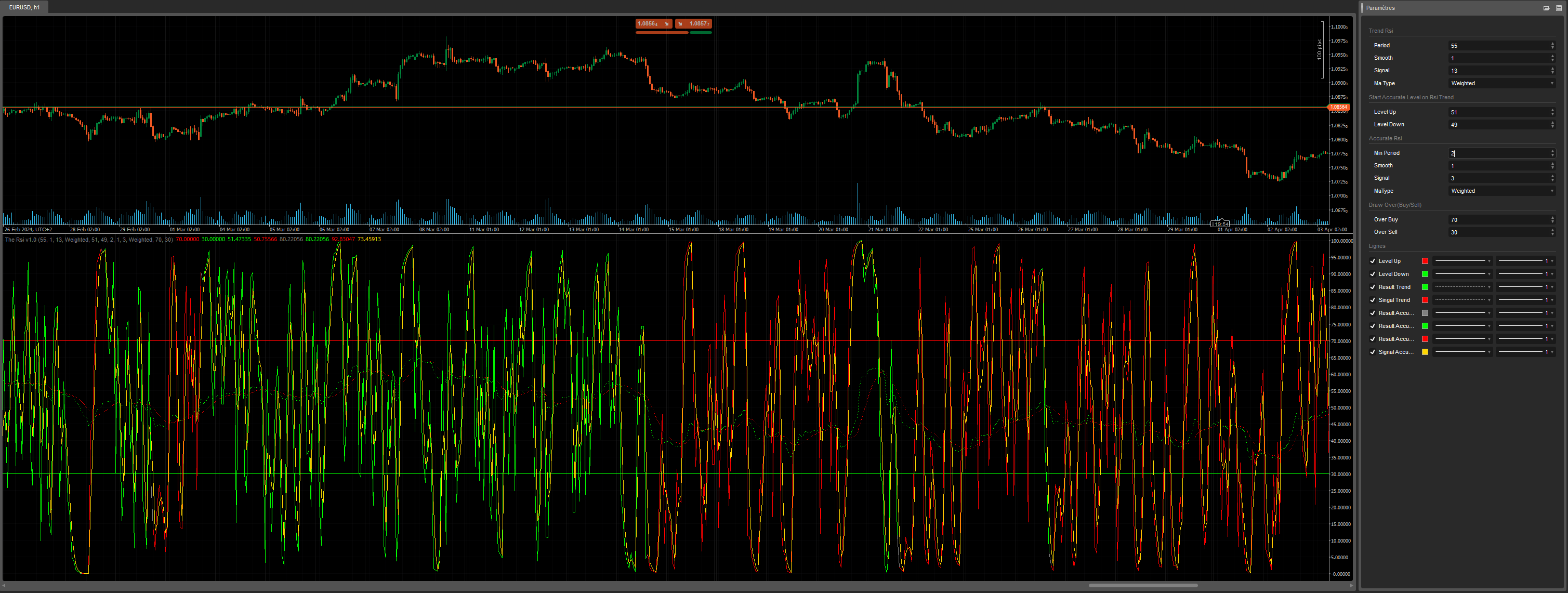
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using cAlgo.API;
using cAlgo.API.Collections;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Indicator(IsOverlay = false, AccessRights = AccessRights.None)]
public class TheRsiHilo : Indicator
{
[Parameter("Period ", DefaultValue = 55, Group = "Trend Rsi")]
public int RsiTrendPeriod { get; set; }
[Parameter("Smooth ", DefaultValue = 1, Group = "Trend Rsi")]
public int RsiTrendSmooth { get; set; }
[Parameter("Signal ", DefaultValue = 13, Group = "Trend Rsi")]
public int RsiTrendSignal { get; set; }
[Parameter("Ma Type ", DefaultValue = MovingAverageType.Weighted, Group = "Trend Rsi")]
public MovingAverageType RsiTrendMaType { get; set; }
[Parameter("Level Up", DefaultValue = 51, Group = "Start Accurate Level on Rsi Trend")]
public int TrendStartLevelUp { get; set; }
[Parameter("Level Down", DefaultValue = 49, Group = "Start Accurate Level on Rsi Trend")]
public int TrendStartLevelDown { get; set; }
[Parameter("Increase Period", DefaultValue = 2, Group = "Accurate Rsi")]
public bool RsiAccuIncreasePeriod { get; set; }
[Parameter("Min Period ", DefaultValue = 2, Group = "Accurate Rsi")]
public int RsiAccuMinPeriod { get; set; }
[Parameter("Max Period ", DefaultValue = 55, Group = "Accurate Rsi")]
public int RsiAccuMaxPeriod { get; set; }
[Parameter("Smooth ", DefaultValue = 1, Group = "Accurate Rsi")]
public int RsiAccuSmooth { get; set; }
[Parameter("Signal ", DefaultValue = 3, Group = "Accurate Rsi")]
public int RsiAccuSignal { get; set; }
[Parameter("MaType ", DefaultValue = MovingAverageType.Weighted, Group = "Accurate Rsi")]
public MovingAverageType RsiAccuMaType { get; set; }
[Parameter("Over Buy", DefaultValue = 70, Group = "Draw Over(Buy/Sell) ")]
public int OverBuy { get; set; }
[Parameter("Over Sell", DefaultValue = 30, Group = "Draw Over(Buy/Sell) ")]
public int OverSell { get; set; }
[Output("Level Up", LineColor = "Red", PlotType = PlotType.Line, LineStyle = LineStyle.Solid)]
public IndicatorDataSeries LevelUp { get; set; }
[Output("Level Down", LineColor = "Lime", PlotType = PlotType.Line, LineStyle = LineStyle.Solid)]
public IndicatorDataSeries LevelDown { get; set; }
[Output("Result Trend", LineColor = "Lime", PlotType = PlotType.Line, LineStyle = LineStyle.Dots)]
public IndicatorDataSeries ResultTrend { get; set; }
[Output("Singal Trend", LineColor = "Red", PlotType = PlotType.Line, LineStyle = LineStyle.Dots)]
public IndicatorDataSeries SingalTrend { get; set; }
[Output("Result Accurate", LineColor = "Gray", PlotType = PlotType.Line)]
public IndicatorDataSeries ResultAccu { get; set; }
[Output("Result Accurate Up", LineColor = "Lime", PlotType = PlotType.DiscontinuousLine)]
public IndicatorDataSeries ResultAccuUp { get; set; }
[Output("Result Accurate Down", LineColor = "Red", PlotType = PlotType.DiscontinuousLine)]
public IndicatorDataSeries ResultAccuDown { get; set; }
[Output("Signal Accurate", LineColor = "Gold", PlotType = PlotType.DiscontinuousLine)]
public IndicatorDataSeries SignalAccu { get; set; }
private IndicatorDataSeries highData, medData, lowData, res1, res2;
private IndicatorDataSeries highData2, lowData2;
private MovingAverage rsiTrendSmooth, rsiTrendSignal, rsiAccuSmooth, rsiAccuSignal;
private RelativeStrengthIndex rsiHighInit, rsiMedInit, rsiLowInit, rsiHighAccu, rsiLowAccu;
private int CountUp;
private int CountDown;
private int indexUp;
private int indexDown;
private int initialPeriod = 1;
private bool StartCountUp;
private bool StartCountDown;
protected override void Initialize()
{
highData = CreateDataSeries();
medData = CreateDataSeries();
lowData = CreateDataSeries();
res1 = CreateDataSeries();
res2 = CreateDataSeries();
highData2 = CreateDataSeries();
lowData2 = CreateDataSeries();
rsiHighInit = Indicators.RelativeStrengthIndex(Bars.HighPrices, RsiTrendPeriod);
rsiMedInit = Indicators.RelativeStrengthIndex(Bars.MedianPrices, RsiTrendPeriod);
rsiLowInit = Indicators.RelativeStrengthIndex(Bars.LowPrices, RsiTrendPeriod);
rsiHighAccu = Indicators.RelativeStrengthIndex(Bars.HighPrices, initialPeriod);
rsiLowAccu = Indicators.RelativeStrengthIndex(Bars.LowPrices, initialPeriod);
rsiTrendSmooth = Indicators.MovingAverage(res1, RsiTrendSmooth, RsiTrendMaType);
rsiTrendSignal = Indicators.MovingAverage(rsiTrendSmooth.Result, RsiTrendSignal, RsiTrendMaType);
rsiAccuSmooth = Indicators.MovingAverage(res2, RsiAccuSmooth, RsiAccuMaType);
rsiAccuSignal = Indicators.MovingAverage(rsiAccuSmooth.Result, RsiAccuSignal, RsiAccuMaType);
CountUp = 0;
CountDown = 0;
StartCountUp = false;
StartCountDown = false;
}
public override void Calculate(int index)
{
highData[index] = rsiHighInit.Result.Last(0);
medData[index] = rsiMedInit.Result.Last(0);
lowData[index] = rsiLowInit.Result.Last(0);
res1[index] = medData[index] < 50 ? highData[index] : medData[index] > 50 ? lowData[index] : medData[index];
ResultTrend[index] = rsiTrendSmooth.Result.Last(0);
SingalTrend[index] = rsiTrendSignal.Result.Last(0);
LevelUp[index] = OverBuy;
LevelDown[index] = OverSell;
if (medData[index] > TrendStartLevelUp)
{
StartCountDown = false;
StartCountUp = true;
CountDown = 0;
}
else if (medData[index] < TrendStartLevelDown)
{
StartCountUp = false;
StartCountDown = true;
CountUp = 0;
}
if (StartCountUp && indexUp < index)
{
CountUp++;
CountDown = 0;
initialPeriod = RsiAccuMinPeriod >= CountUp ? RsiAccuMinPeriod : RsiAccuMaxPeriod <= CountUp ? RsiAccuMaxPeriod : CountUp;
rsiLowAccu = Indicators.RelativeStrengthIndex(Bars.LowPrices, RsiAccuIncreasePeriod ? initialPeriod : RsiAccuMinPeriod);
rsiHighAccu = Indicators.RelativeStrengthIndex(Bars.HighPrices, RsiAccuIncreasePeriod ? initialPeriod : RsiAccuMinPeriod);
highData2[index] = rsiHighAccu.Result.Last(0);
lowData2[index] = rsiLowAccu.Result.Last(0);
res2[index] = lowData2[index];
ResultAccuUp[index] = rsiAccuSmooth.Result.Last(0);
indexUp = index;
}
else if (StartCountDown && indexDown < index)
{
CountDown++;
CountUp = 0;
initialPeriod = RsiAccuMinPeriod >= CountDown ? RsiAccuMinPeriod : RsiAccuMaxPeriod <= CountDown ? RsiAccuMaxPeriod : CountDown;
rsiHighAccu = Indicators.RelativeStrengthIndex(Bars.HighPrices, RsiAccuIncreasePeriod ? initialPeriod : RsiAccuMinPeriod);
rsiLowAccu = Indicators.RelativeStrengthIndex(Bars.LowPrices, RsiAccuIncreasePeriod ? initialPeriod : RsiAccuMinPeriod);
highData2[index] = rsiHighAccu.Result.Last(0);
lowData2[index] = rsiLowAccu.Result.Last(0);
res2[index] = highData2[index];
ResultAccuDown[index] = rsiAccuSmooth.Result.Last(0);
indexDown = index;
}
ResultAccu[index] = rsiAccuSmooth.Result.Last(0);
SignalAccu[index] = rsiAccuSignal.Result.Last(0);
}
}
}
YesOrNot2
Joined on 17.05.2024
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: The Rsi v1.0_withSourceCode.algo
- Rating: 5
- Installs: 605
- Modified: 21/05/2024 23:41
Thank you sir. And I'm glad you were released. ) If possible, please make this RSI the MTF version. And sorry for the broken English. )