Description
Contact Us: t.me/irumbukai
Donate : $10
specialized for funded accounts.
Entry Master
Welcome to Entry_Master This manual provides detailed instructions on utilizing various functions and shortcuts to manage your trades effectively.
1. Placing Orders:
Ctrl + Left-click: Sell Limit Order
- Use this action to place a sell limit order at the clicked price.
Alt + Left-click: Buy Limit Order
- Use this action to place a buy limit order at the clicked price.
Shift + Ctrl + Left-click: Sell Market Order
- Use this action to place a sell market order at the clicked price.
Shift + Alt + Left-click: Buy Market Order
- Use this action to place a buy market order at the clicked price.
Shift + Alt + Ctrl + Left-click: Close Order
- Use this action to close the nearest position or cancel the nearest order.
Ctrl + Alt + Left-click: Set Break Even
- This action sets the stop-loss level of the nearest position to break even.
Ctrl + Alt + Space: Reverse Order
- This action reverse the current position.
Alt + Space: Quick Limit order
- This action Limit line color and execute the market order when any line reach the Price line
2. Drawing Levels:
1 or Numpad 1: Draw Price Line
- Use this shortcut to draw a price level line on the chart.
2 or Numpad 2: Draw Stop Loss Line
- Use this shortcut to draw the stop-loss level line on the chart.
3 or Numpad 3: Draw Target Line
- Use this shortcut to draw the target level line on the chart.
R or Numpad 7: Remove Line
- Use this shortcut to remove the last drawn line from the chart.
3. Placing Orders:
- Space or Numpad 0: Place Order
- Press this shortcut to execute an order based on the drawn levels.
- The cBot will determine the order type (limit or market) based on the drawn levels and market conditions.
4. Important Notes:
Proper risk management is crucial for successful trading. Ensure that you set appropriate stop-loss and target levels before executing orders.
Always double-check the placement of lines and orders before confirming to avoid errors.
Customize the hotkeys according to your preference using the cTrader Hotkey Settings. (Release Space bar from quick search)
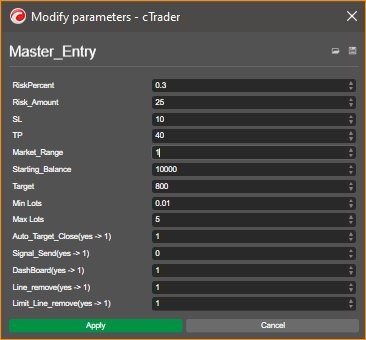
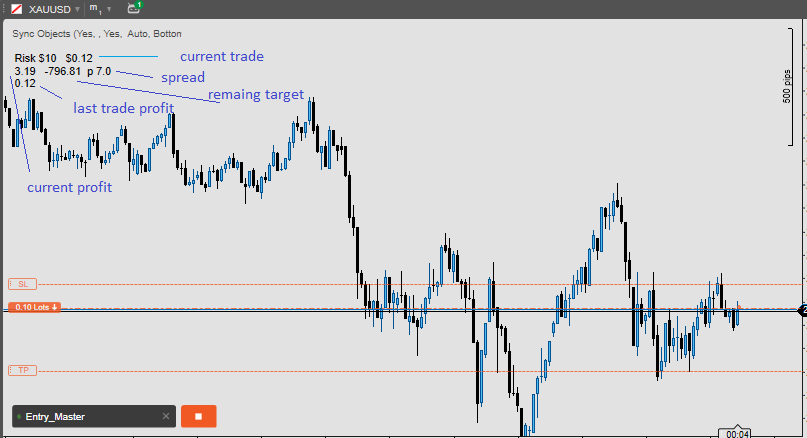
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
using System.Threading;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)]
public class MyRobot : Robot
{
[Parameter("RiskPercent", DefaultValue = 0.1)]
public double RiskPercent { get; set; }
[Parameter("Risk_Amount", DefaultValue = 10, MinValue = 1)]
public int RiskAmount { get; set; }
[Parameter("SL", DefaultValue = 100)]
public double Sl { get; set; }
[Parameter("TP", DefaultValue = 400)]
public double Tp { get; set; }
[Parameter("Market_Range", DefaultValue = 2)]
public double Range { get; set; }
[Parameter("Starting_Balance", DefaultValue = 10000)]
public double Start { get; set; }
[Parameter("Target", DefaultValue = 800)]
public double Targt { get; set; }
[Parameter("Min Lots", DefaultValue = 0.01, MinValue = 0.01, Step = 0.1)]
public double MinLots { get; set; }
[Parameter("Max Lots", DefaultValue = 5, MinValue = 0.01, Step = 0.1)]
public double MaxLots { get; set; }
[Parameter("Auto_Target_Close(yes -> 1)", DefaultValue = 1, MinValue = 0, MaxValue = 1)]
public int Auttg { get; set; }
[Parameter("Signal_Send(yes -> 1)", DefaultValue = 1, MinValue = 0, MaxValue = 1)]
public int Sig { get; set; }
[Parameter("DashBoard(yes -> 1)", DefaultValue = 1, MinValue = 0, MaxValue = 1)]
public int Dass { get; set; }
[Parameter("Line_remove(yes -> 1)", DefaultValue = 1, MinValue = 0, MaxValue = 1)]
public int LineR { get; set; }
[Parameter("Limit_Line_remove(yes -> 1)", DefaultValue = 1, MinValue = 0, MaxValue = 1)]
public int LLineR { get; set; }
[Parameter("Balance and target(yes -> 1)", DefaultValue = 1, MinValue = 0, MaxValue = 1)]
public int Target_bal { get; set; }
private ChartHorizontalLine Priceline;
private ChartHorizontalLine Stopline;
private ChartHorizontalLine Targetline;
private double price;
private double Entry = 0;
private double stop = 0;
private double target = 0;
protected override void OnStart()
{
Chart.MouseDown += OnChartMouseDown;
Chart.MouseMove += ChartOnMouseMove;
Positions.Opened += OnPositionOpened;
Chart.AddHotkey(ReverseLastPosition, "Ctrl+Alt+Space");
Chart.AddHotkey(Drawprice, Key.D1, ModifierKeys.None);
Chart.AddHotkey(Drawprice, Key.NumPad1, ModifierKeys.None);
Chart.AddHotkey(Drawstop, Key.D2, ModifierKeys.None);
Chart.AddHotkey(Drawstop, Key.NumPad2, ModifierKeys.None);
Chart.AddHotkey(Drawtarget, Key.D3, ModifierKeys.None);
Chart.AddHotkey(Drawtarget, Key.NumPad3, ModifierKeys.None);
Chart.AddHotkey(Order, Key.Space, ModifierKeys.None);
Chart.AddHotkey(Order, Key.NumPad0, ModifierKeys.None);
Chart.AddHotkey(Removeobject, Key.R, ModifierKeys.None);
Chart.AddHotkey(Removeobject, Key.NumPad7, ModifierKeys.None);
Chart.AddHotkey(Line_color_Change, Key.NumPad5, ModifierKeys.None);
Chart.AddHotkey(Line_color_Change, Key.Space, ModifierKeys.Alt);
}
private void Order()
{
if (stop != 0 && Entry != 0 && target != 0)
{
Limitorder(Entry, stop, target);
if(LLineR==1){Removeobject();}
}
else if (stop != 0 && Entry != 0 && target == 0)
{
Limitorder(Entry, stop, 0);
if(LLineR==1){Removeobject();}
}
else if (stop != 0 && Entry == 0 && target != 0)
{
Marketorder(stop, target);
if(LineR==1){Removeobject();}
}
else if (stop != 0 && Entry == 0 && target == 0)
{
Marketorder(stop, 0);
if(LineR==1){Removeobject();}
}
else { Removeobject(); }
}
private void Limitorder(double entry, double stop, double target)
{
var digit = (int)(Math.Log10(Symbol.PipSize) - Math.Log10(Symbol.TickSize));
var stopPips = Math.Round(Math.Abs(entry - stop) / Symbol.PipSize, digit);
double targetPips = 0;
if (target != 0)
{
targetPips = Math.Round(Math.Abs(entry - target) / Symbol.PipSize, digit);
}
var permitalbleLoss = Math.Min((Account.Balance) * RiskPercent / 100, RiskAmount);
var volume = permitalbleLoss / stopPips / Symbol.PipValue;
volume = Symbol.NormalizeVolumeInUnits(volume);
if (volume < Symbol.QuantityToVolumeInUnits(MinLots)) volume = Symbol.QuantityToVolumeInUnits(MinLots);
if (volume > Symbol.QuantityToVolumeInUnits(MaxLots)) volume = Symbol.QuantityToVolumeInUnits(MaxLots);
if (stop < entry)
{
if (Symbol.Ask > entry) { PlaceLimitOrder(TradeType.Buy, SymbolName, volume, entry, "", stopPips, targetPips); }
else { PlaceStopOrder(TradeType.Buy, SymbolName, volume, entry, "", stopPips, targetPips); }
}
else if (stop > entry)
{
if (Symbol.Bid < entry) { PlaceLimitOrder(TradeType.Sell, SymbolName, volume, entry, "", stopPips, targetPips); }
else { PlaceStopOrder(TradeType.Sell, SymbolName, volume, entry, "", stopPips, targetPips); }
}
else { }
}
private void Marketorder(double stop, double target)
{
var digit = (int)(Math.Log10(Symbol.PipSize) - Math.Log10(Symbol.TickSize));
double stopPips;
double targetPips;
double rr;
TradeType tt;
if (stop != 0 && Symbol.Ask < stop && Symbol.Bid < stop)
{ stopPips = Math.Round(Math.Abs(stop - Symbol.Bid) / Symbol.PipSize, digit); tt = TradeType.Sell; rr = Symbol.Bid; }
else { stopPips = Math.Round(Math.Abs(Symbol.Ask - stop) / Symbol.PipSize, digit); tt = TradeType.Buy; rr = Symbol.Ask; }
if (target != 0 && Symbol.Ask < target && Symbol.Bid < target)
{ targetPips = Math.Round(Math.Abs(target - Symbol.Bid) / Symbol.PipSize, digit); }
else { targetPips = Math.Round(Math.Abs(Symbol.Ask - target) / Symbol.PipSize, digit); }
var permitalbleLoss = Math.Min((Account.Balance) * RiskPercent / 100, RiskAmount);
var volume = permitalbleLoss / stopPips / Symbol.PipValue;
volume = Symbol.NormalizeVolumeInUnits(volume);
if (volume < Symbol.QuantityToVolumeInUnits(MinLots)) volume = Symbol.QuantityToVolumeInUnits(MinLots);
if (volume > Symbol.QuantityToVolumeInUnits(MaxLots)) volume = Symbol.QuantityToVolumeInUnits(MaxLots);
ExecuteMarketRangeOrder(tt, SymbolName, volume, Range, rr, "", stopPips, targetPips);
}
private void Removeobject()
{
if (Stopline.Y != 0)
{
Chart.RemoveObject(Stopline.Name);
stop = 0;
if (Priceline.Y != 0)
{
Chart.RemoveObject(Priceline.Name);
Entry = 0;
}
if (Targetline.Y != 0)
{
Chart.RemoveObject(Targetline.Name);
target = 0;
}
}
}
private double Volum()
{
int permitalbleLoss = Math.Min((int)Math.Floor((Account.Balance * RiskPercent) / 100), RiskAmount);
var volume = permitalbleLoss / Sl / Symbol.PipValue;
volume = Symbol.NormalizeVolumeInUnits(volume);
if (volume < Symbol.QuantityToVolumeInUnits(MinLots)) volume = Symbol.QuantityToVolumeInUnits(MinLots);
if (volume > Symbol.QuantityToVolumeInUnits(MaxLots)) volume = Symbol.QuantityToVolumeInUnits(MaxLots);
return volume;
}
private void ChartOnMouseMove(ChartMouseEventArgs obj)
{
price = TruncateDecimalDigits(obj.YValue);
}
double TruncateDecimalDigits(double value)
{
string valueStr = ((decimal)Symbol.TickSize).ToString();
int decimalIndex = valueStr.IndexOf('.');
int d = decimalIndex == -1 ? 0 : valueStr.Length - decimalIndex - 1;
double factor = Math.Pow(10, d);
double truncatedValue = Math.Truncate(value * factor) / factor;
return truncatedValue;
}
private void Drawprice(ChartKeyboardEventArgs obj)
{
if (price != 0)
{
if (Priceline != null)
{
Chart.RemoveObject(Priceline.Name);
}
Priceline = Chart.DrawHorizontalLine($"Price", price, Color.Blue);
Entry = price;
}
}
private void Drawstop(ChartKeyboardEventArgs obj)
{
if (price != 0)
{
if (Stopline != null)
{
Chart.RemoveObject(Stopline.Name);
}
Stopline = Chart.DrawHorizontalLine($"Stop", price, Color.Red);
stop = price;
}
}
private void Drawtarget(ChartKeyboardEventArgs obj)
{
if (price != 0)
{
if (Targetline != null)
{
Chart.RemoveObject(Targetline.Name);
}
Targetline = Chart.DrawHorizontalLine($"Target", price, Color.Green);
target = price;
}
}
private void ReverseLastPosition()
{
int l = Positions.Count - 1;
Position pos = Positions[l];
if (l != -1)
{
double mod_sl = Symbol.Ask - (Symbol.PipValue * Sl);
double mod_tp = Symbol.Ask + (Symbol.PipValue * Tp);
if (pos.TradeType == TradeType.Buy)
{
mod_sl = Symbol.Bid + (Symbol.PipValue * Sl);
mod_tp = Symbol.Bid - (Symbol.PipValue * Tp);
}
if (ReversePosition(pos).IsSuccessful)
{
int last = Positions.Count - 1;
Position repos = Positions[last];
ModifyPosition(repos, mod_sl, mod_tp);
}
}
}
void OnChartMouseDown(ChartMouseEventArgs obj)
{
double entb = Symbol.Ask;
double ents = Symbol.Bid;
double Volu = Volum();
if (obj.AltKey && !obj.CtrlKey && !obj.ShiftKey)
{
if (obj.YValue > Symbol.Bid)
PlaceStopOrder(TradeType.Buy, SymbolName, Volu, obj.YValue, "", Sl, Tp);
else
PlaceLimitOrder(TradeType.Buy, SymbolName, Volu, obj.YValue, "", Sl, Tp);
}
if (obj.CtrlKey && !obj.AltKey && !obj.ShiftKey)
{
if (obj.YValue > Symbol.Bid)
PlaceLimitOrder(TradeType.Sell, SymbolName, Volu, obj.YValue, "", Sl, Tp);
else
PlaceStopOrder(TradeType.Sell, SymbolName, Volu, obj.YValue, "", Sl, Tp);
}
if (obj.AltKey && obj.CtrlKey && !obj.ShiftKey)
BreakEvenPosition(obj.YValue);
if (obj.ShiftKey && !obj.CtrlKey && obj.AltKey)
ExecuteMarketRangeOrder(TradeType.Buy, SymbolName, Volu, Range, entb, "", Sl, Tp);
if (obj.ShiftKey && obj.CtrlKey && !obj.AltKey)
ExecuteMarketRangeOrder(TradeType.Sell, SymbolName, Volu, Range, ents, "", Sl, Tp);
if (!obj.AltKey && !obj.CtrlKey && obj.ShiftKey)
CancelClosestOrder(obj.YValue);
if (obj.AltKey && obj.CtrlKey && obj.ShiftKey)
CloseClosestPosition(obj.YValue);
}
private void CloseClosestPosition(double price)
{
if (Positions.Count == 0)
return;
Position pos = Positions[0];
foreach (var _pos in Positions)
{
if (Math.Abs(price - _pos.EntryPrice) < Math.Abs(price - pos.EntryPrice))
pos = _pos;
}
ClosePosition(pos);
}
private void BreakEvenPosition(double price)
{
if (Positions.Count == 0)
return;
Position pos = Positions[0];
foreach (var _pos in Positions)
{
if (Math.Abs(price - _pos.EntryPrice) < Math.Abs(price - pos.EntryPrice))
pos = _pos;
}
if (pos.EntryPrice == pos.StopLoss || (pos.TradeType == TradeType.Buy && Symbol.Bid <= pos.EntryPrice) || (pos.TradeType == TradeType.Sell && Symbol.Ask >= pos.EntryPrice))
return;
ModifyPosition(pos, pos.EntryPrice, pos.TakeProfit);
}
private void CancelClosestOrder(double price)
{
if (PendingOrders.Count == 0)
return;
PendingOrder ord = PendingOrders[0];
foreach (var _ord in PendingOrders)
{
if (Math.Abs(price - _ord.TargetPrice) < Math.Abs(price - ord.TargetPrice))
ord = _ord;
}
CancelPendingOrder(ord);
}
private void OnPositionOpened(PositionOpenedEventArgs args)
{
Position position = args.Position;
string tradeInfo = $"New Position Opened:\nSymbol: {position.SymbolName}\nType: {position.TradeType}\nVolume: {position.VolumeInUnits}\nEntry Price: {position.EntryPrice}\nStop Loss: {position.StopLoss}\nTake Profit: {position.TakeProfit}";
if (Sig == 1) { SaveTextToFile(tradeInfo); }
}
private void SaveTextToFile(string textContent)
{
try
{
string filePath = @"C:\Users\A\Pictures\Screenshots\TradeParameters.txt";
System.IO.File.AppendAllText(filePath, textContent + "\n\n");
}
catch (Exception ex)
{
Print("Error saving trade parameters: " + ex.Message);
}
}
private void Line_color_Change()
{
if(Entry != 0 && stop != 0)
{
if (Priceline.Color == "Blue" && Stopline.Color == "Red")
{ Priceline.Color = "SkyBlue";
Stopline.Color = "Orange";
}
else
{
Priceline.Color = "Blue";
Stopline.Color = "Red";
}
}
}
private void Optimie_Limit()
{
if(Entry != 0 && stop != 0)
{
if (Entry > stop)
{
if (!(Entry < Symbol.Bid) || !(Entry < Symbol.Ask))
{
Marketorder(stop,target);
Removeobject();
}
}
if (Entry < stop)
{
if (!(Entry > Symbol.Bid) || !(Entry > Symbol.Ask))
{
Marketorder(stop,target);
Removeobject();
}
}
}
}
protected override void OnTick()
{
if (Priceline != null && Stopline != null)
{
if (Priceline.Color == "SkyBlue" && Stopline.Color == "Orange"){ Optimie_Limit(); }
}
if (Dass == 1)
{
string blan_tar = "";
double stopPips = Sl;
double stopPip = 0;
double cumulativeProfit = 0;
var digit = (int)(Math.Log10(Symbol.PipSize) - Math.Log10(Symbol.TickSize));
if (Entry != 0 && stop != 0)
{
stopPip = Math.Round(Math.Abs(Entry - stop) / Symbol.PipSize, digit);
}
else if (Entry == 0 && stop != 0)
{
if (Symbol.Ask < stop && Symbol.Bid < stop)
{
stopPip = Math.Round(Math.Abs(Symbol.Bid - (stop + Symbol.TickSize)) / Symbol.PipSize, digit);
}
else { stopPip = Math.Round(Math.Abs(Symbol.Ask - (stop + Symbol.TickSize)) / Symbol.PipSize, digit); }
}
if (stopPip != 0) { stopPips = stopPip; } else { }
var permitalbleLoss = Math.Min((Account.Balance) * RiskPercent / 100, RiskAmount);
var volume = permitalbleLoss / stopPips / Symbol.PipValue;
volume = Symbol.NormalizeVolumeInUnits(volume);
if (volume < Symbol.QuantityToVolumeInUnits(MinLots)) volume = Symbol.QuantityToVolumeInUnits(MinLots);
if (volume > Symbol.QuantityToVolumeInUnits(MaxLots)) volume = Symbol.QuantityToVolumeInUnits(MaxLots);
var risk_price = volume * stopPips * Symbol.PipValue;
foreach (var position in Positions)
{
cumulativeProfit += position.NetProfit;
}
if (Auttg == 1)
{
if (((Account.Balance - Start) + cumulativeProfit) >= (Targt + (Account.Balance / 1000)))
{
foreach (var pos in Positions)
{
ClosePosition(pos);
}
}
if (Target_bal ==1)
{
blan_tar = (Account.Balance - Start).ToString("0.00") + "__" + ((Account.Balance - Start) - Targt).ToString("0.00") ;
}
Chart.DrawStaticText("Dashboard", "lot " + Symbol.VolumeInUnitsToQuantity(volume).ToString("0.00") + "__$" + risk_price.ToString("0.00") +"__"+stopPips.ToString() + "__$" + cumulativeProfit.ToString("0.00") + "\n" +blan_tar + " p" + (Symbol.Spread / Symbol.PipValue).ToString("0.0"), VerticalAlignment.Top, HorizontalAlignment.Left, Color.Gray );
if (Positions.Count == 0) { Chart.DrawStaticText("Profit", "\n" + "\n" + "" + LastResult.Position.NetProfit.ToString("0.00"), VerticalAlignment.Top, HorizontalAlignment.Left, Color.Gray); }
}
}
}
protected override void OnStop()
{
Chart.MouseMove -= ChartOnMouseMove;
}
}
}
Irumbukkai
Joined on 02.02.2024 Blocked
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Master_Entry.algo
- Rating: 5
- Installs: 207
- Modified: 06/06/2024 02:22
Comments
if you couldn't know that video because of that language we could conatact in telegram
send your id and i will contact you
wish best for you
telegram_id updated in description