Description
The "Sample RSI Range Robot" will create a buy order when the Relative Strength Index indicator crosses the level 30,
and a Sell order when the RSI indicator crosses the level 70. The order is closed be either a Stop Loss, defined in
the "Stop Loss" parameter, or by the opposite RSI crossing signal (buy orders close when RSI crosses the 70 level
and sell orders are closed when RSI crosses the 30 level).
The robot can generate only one Buy or Sell order at any given time.
/
akleanthous
Joined on 11.07.2012
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: RSI Range Robot.algo
- Rating: 0
- Installs: 6569
- Modified: 13/10/2021 09:54
Comments
Hi,
can anyone enter the trail stop in this bot?
Basic adjustment to code to make all parameters configurable, including upper and lower RSI range and stop-loss / take-profit.
// -------------------------------------------------------------------------------------------------
//
// This code is a cTrader Automate API example.
//
// This cBot is intended to be used as a sample and does not guarantee any particular outcome or
// profit of any kind. Use it at your own risk.
//
// All changes to this file might be lost on the next application update.
// If you are going to modify this file please make a copy using the "Duplicate" command.
//
// The "Sample RSI cBot" will create a buy order when the Relative Strength Index indicator crosses the level 30,
// and a Sell order when the RSI indicator crosses the level 70. The order is closed be either a Stop Loss, defined in
// the "Stop Loss" parameter, or by the opposite RSI crossing signal (buy orders close when RSI crosses the 70 level
// and sell orders are closed when RSI crosses the 30 level).
//
// The cBot can generate only one Buy or Sell order at any given time.
//
// -------------------------------------------------------------------------------------------------
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class SampleRSIcBot : Robot
{
[Parameter("Quantity (Lots)", Group = "Volume", DefaultValue = 1, MinValue = 0.01, Step = 0.01)]
public double Quantity { get; set; }
[Parameter("Source", Group = "RSI")]
public DataSeries Source { get; set; }
[Parameter("Periods", Group = "RSI", DefaultValue = 14)]
public int Periods { get; set; }
[Parameter("Lower RSI Level", Group = "RSI", DefaultValue = 30, MinValue = 0, MaxValue = 50)]
public int LRL { get; set; }
[Parameter("Upper RSI Level", Group = "RSI", DefaultValue = 70, MinValue = 50, MaxValue = 100)]
public int URL { get; set; }
[Parameter("Take Profit in pips", Group = "TP SL", DefaultValue = 100)]
public int TP { get; set; }
[Parameter("Stop Loss in pips", Group = "TP SL", DefaultValue = 100)]
public int SL { get; set; }
private RelativeStrengthIndex rsi;
protected override void OnStart()
{
rsi = Indicators.RelativeStrengthIndex(Source, Periods);
}
protected override void OnTick()
{
if (rsi.Result.LastValue < LRL)
{
Close(TradeType.Sell);
Open(TradeType.Buy);
}
else if (rsi.Result.LastValue > URL)
{
Close(TradeType.Buy);
Open(TradeType.Sell);
}
}
private void Close(TradeType tradeType)
{
foreach (var position in Positions.FindAll("SampleRSI", SymbolName, tradeType))
ClosePosition(position);
}
private void Open(TradeType tradeType)
{
var position = Positions.Find("SampleRSI", SymbolName, tradeType);
var volumeInUnits = Symbol.QuantityToVolumeInUnits(Quantity);
if (position == null)
ExecuteMarketOrder(tradeType, SymbolName, volumeInUnits, "SampleRSI", SL, TP);
}
}
}
STOPLOSS IS OK BUT HOW CAN I ADD TAKEPROFIT ALSO
The stoploss still does not work!
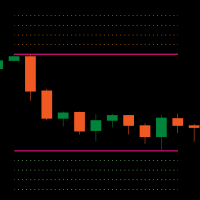
...Stop Loss not working !!!
Please anyone can fix it ???
Good robot, but SL is not included, has anyone solved this problem?
it works perfectly