Warning! This section will be deprecated on February 1st 2025. Please move all your Indicators to the cTrader Store catalogue.
Description
In addition to the regular three lines, you can add three lines each above and below the midline (six lines in total).
You can change the numbers to whatever you like, such as the Fibonacci sequence.
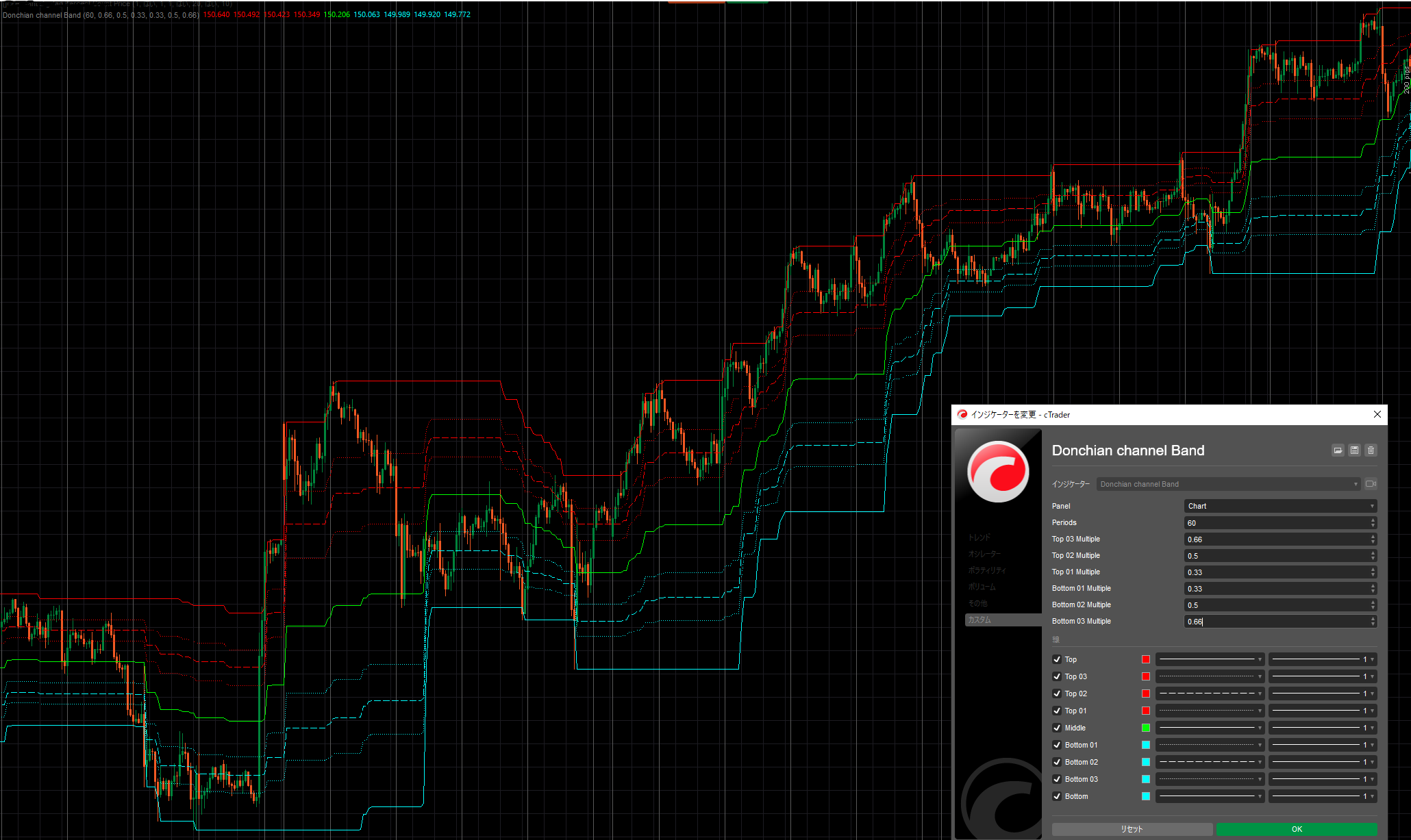
Support developers
https://cpheasant.gumroad.com/l/wzxuvf
Other indicators
・Donchian Channel Break update
・Donchian Channel Candle body Break update
・Donchian Channel Candle body Min width
・Zig Zag Count&Price&Percent&Fibo
YouTube / X(Twitter) / Telegram
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using cAlgo.API;
using cAlgo.API.Collections;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AutoRescale = false,AccessRights = AccessRights.None)]
public class cPeasantDonchianchannelBand : Indicator
{
[Parameter("Periods",DefaultValue = 20, MinValue = 1, Step = 1)]
public int Periods { get; set; }
[Parameter("Top 03 Multiple",DefaultValue = 0.66, MaxValue = 1)]
public double t3 { get; set; }
[Parameter("Top 02 Multiple",DefaultValue = 0.5, MaxValue = 1)]
public double t2 { get; set; }
[Parameter("Top 01 Multiple",DefaultValue = 0.33, MaxValue = 1)]
public double t1 { get; set; }
[Parameter("Bottom 01 Multiple",DefaultValue = 0.33, MaxValue = 1)]
public double b1 { get; set; }
[Parameter("Bottom 02 Multiple",DefaultValue = 0.5, MaxValue = 1)]
public double b2 { get; set; }
[Parameter("Bottom 03 Multiple",DefaultValue = 0.66, MaxValue = 1)]
public double b3 { get; set; }
[Output("Top", LineColor = "Red")]
public IndicatorDataSeries Top { get; set; }
[Output("Top 03", LineColor = "Red", LineStyle = LineStyle.Dots)]
public IndicatorDataSeries Top03 { get; set; }
[Output("Top 02", LineColor = "Red", LineStyle = LineStyle.Lines)]
public IndicatorDataSeries Top02 { get; set; }
[Output("Top 01", LineColor = "Red", LineStyle = LineStyle.Dots)]
public IndicatorDataSeries Top01 { get; set; }
[Output("Middle", LineColor = "Lime")]
public IndicatorDataSeries Middle { get; set; }
[Output("Bottom 01", LineColor = "Cyan", LineStyle = LineStyle.Dots)]
public IndicatorDataSeries Bottom01 { get; set; }
[Output("Bottom 02", LineColor = "Cyan", LineStyle = LineStyle.Lines)]
public IndicatorDataSeries Bottom02 { get; set; }
[Output("Bottom 03", LineColor = "Cyan", LineStyle = LineStyle.Dots)]
public IndicatorDataSeries Bottom03 { get; set; }
[Output("Bottom", LineColor = "Cyan")]
public IndicatorDataSeries Bottom { get; set; }
int N,nt,nb;
protected override void Initialize()
{
N = (Periods+1);
nt=1;
nb=1;
}
public override void Calculate(int index)
{
double HL;
nt++;
nb++;
if (Bars.ClosePrices.Count < N) //左からN個は計算しない
return;
else if(Bars.ClosePrices.Count == N)
{
Top[index] = Bars.HighPrices[index - Periods];
Bottom[index] = Bars.LowPrices[index - Periods];
for (var i = index - Periods + 1; i <= index-1; i++)
{
if(Top[index] < Bars.HighPrices[i])
{
Top[index] = Bars.HighPrices[i];
nt = index-i;
}
if(Bottom[index] > Bars.LowPrices[i])
{
Bottom[index] = Bars.LowPrices[i];
nb = index-i;
}
}
}
else
{
/////////////高値処理
if(nt <= Periods)
{
//高値更新
if(Top[index-1] < Bars.HighPrices[index-1])
{
Top[index] = Bars.HighPrices[index-1];
nt = 1;
}
//更新無し
else
{
Top[index] = Top[index-1];
}
}
else if(nt > Periods)
{
Top[index] = Bars.HighPrices[index - Periods];
for (var i = index - Periods + 1; i <= index-1; i++)
{
if(Top[index] < Bars.HighPrices[i])
{
Top[index] = Bars.HighPrices[i];
nt = index-i;
}
}
}
/////////////安値処理
if(nb <= Periods)
{
//安値更新
if(Bottom[index-1] > Bars.LowPrices[index-1])
{
Bottom[index] = Bars.LowPrices[index-1];
nb = 1;
}
//更新無し
else
{
Bottom[index] = Bottom[index-1];
}
}
else if(nb > Periods)
{
Bottom[index] = Bars.LowPrices[index - Periods];
for (var i = index - Periods + 1; i <= index-1; i++)
{
if(Bottom[index] > Bars.LowPrices[i])
{
Bottom[index] = Bars.LowPrices[i];
nb = index-i;
}
}
}
}
HL = (Top[index] - Bottom[index]) / 2;
Middle[index] = Bottom[index] + HL;
Top01[index] = Middle[index] + HL*t1;
Bottom01[index] = Middle[index] - HL*b1;
Top02[index] = Middle[index] + HL*t2;
Bottom02[index] = Middle[index] - HL*b2;
Top03[index] = Middle[index] + HL*t3;
Bottom03[index] = Middle[index] - HL*b3;
}
}
}
CP
cPheasant
Joined on 14.11.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: [cPeasant] Donchian channel Band.algo
- Rating: 0
- Installs: 466
- Modified: 22/02/2024 18:10
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
The program has been improved to reduce processing.
If you have any problems or requests, please feel free to message me.
処理を少なくするようにプログラムを改良しました。
何か問題や要望などあれば気軽にメッセージしてください。