Description
This custom oscillator is based on the 'Automated Support And Resistance' concept from an article by Mel Widner published in TASC.
The indicator consists of two components: the WSO (Widner Support Oscillator) and the WRO (Widner Resistance Oscillator).
The WSO compares the current Close with the six most recent support levels. Values range from 0 to 100. WSO = 0 indicates that the Close is below all six support levels, while WSO = 100 indicates that the current Close is above all six support levels. Changes in WSO indicate shifts in support, either breaking an old level or establishing a new one.
A similar concept applies to the WRO. The WRO compares the current Close with the six most recent resistance levels. Values also range from 0 to 100. WRO = 0 means that the Close is below all six resistance levels, and WRO = 100 means that the current Close is above all six resistance levels. Changes in WRO indicate changes in resistance, which can involve breaking an old level or establishing a new one.
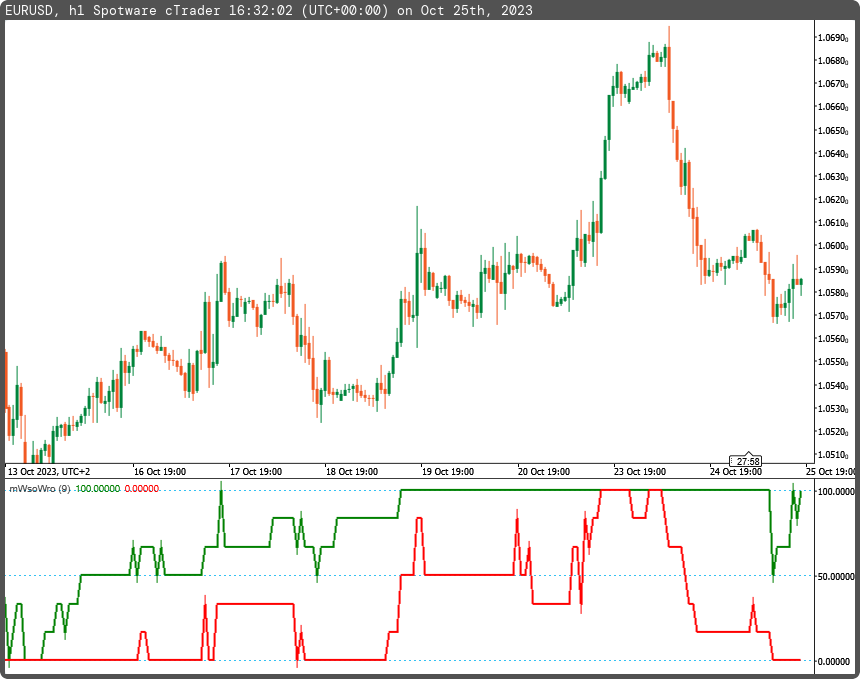
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Levels(0, 50, 100)]
[Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class mWsoWro : Indicator
{
[Parameter("Periods (9)", DefaultValue = 9)]
public int inpPeriod { get; set; }
[Output("WSO Support", LineColor = "Green", PlotType = PlotType.Line, Thickness = 2)]
public IndicatorDataSeries outWSO { get; set; }
[Output("WRO Resistance", LineColor = "Red", PlotType = PlotType.Line, Thickness = 2)]
public IndicatorDataSeries outWRO { get; set; }
private IndicatorDataSeries _wso, _wro;
private double[] _min, _max;
private int centerid;
protected override void Initialize()
{
_min = new double[6];
_max = new double[6];
_wso = CreateDataSeries();
_wro = CreateDataSeries();
centerid = Convert.ToInt16((inpPeriod - 1) / 2); //4
}
public override void Calculate(int i)
{
if(Bars.LowPrices.Minimum(inpPeriod) == Bars.LowPrices[i-centerid])
{
_min[5] = _min[4];
_min[4] = _min[3];
_min[3] = _min[2];
_min[2] = _min[1];
_min[1] = _min[0];
_min[0] = Bars.LowPrices[i-centerid];
}
if(Bars.HighPrices.Maximum(inpPeriod) == Bars.HighPrices[i-centerid])
{
_max[5] = _max[4];
_max[4] = _max[3];
_max[3] = _max[2];
_max[2] = _max[1];
_max[1] = _max[0];
_max[0] = Bars.HighPrices[i-centerid];
}
_wso[i] = 100 * (1 -
( Sigmo(_min[0] / Bars.ClosePrices[i])
+ Sigmo(_min[1] / Bars.ClosePrices[i])
+ Sigmo(_min[2] / Bars.ClosePrices[i])
+ Sigmo(_min[3] / Bars.ClosePrices[i])
+ Sigmo(_min[4] / Bars.ClosePrices[i])
+ Sigmo(_min[5] / Bars.ClosePrices[i])
) / 6.0);
_wro[i] = 100 * (1 -
( Sigmo(_max[0] / Bars.ClosePrices[i])
+ Sigmo(_max[1] / Bars.ClosePrices[i])
+ Sigmo(_max[2] / Bars.ClosePrices[i])
+ Sigmo(_max[3] / Bars.ClosePrices[i])
+ Sigmo(_max[4] / Bars.ClosePrices[i])
+ Sigmo(_max[5] / Bars.ClosePrices[i])
) / 6.0);
outWSO[i] = _wso[i];
outWRO[i] = _wro[i];
}
private double Sigmo(double inValue)
{
return(inValue >= 1.0 ? 1 : 0);
}
}
}
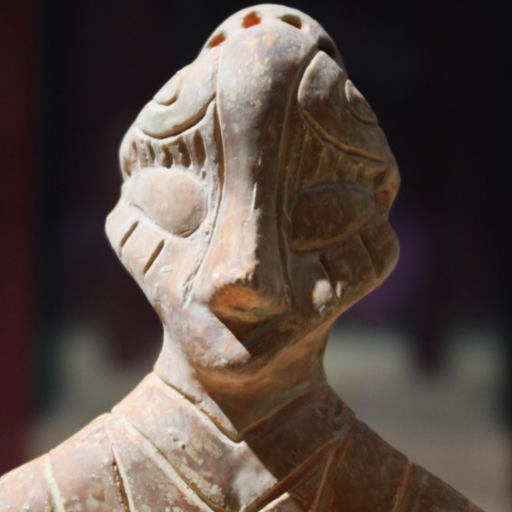
mfejza
Joined on 25.01.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: mWsoWro.algo
- Rating: 5
- Installs: 675
- Modified: 25/10/2023 16:36
http://traders.com/documentation/feedbk_docs/1998/05/Abstracts_new/Widner/WIDMER.html