Description
Is Analysis Paralysis giving you Nightmares ? Then put this on a chart and watch it and you should soon see what the market is doing.
This filters out the noise so you can see the movments
It has a High and Low setting to see the action. Bands are based on ATR
High filter out more noise and Low has less filtering.
Red and Green Cloud is 3 Wilder MA's 8, 32, 96.
Yellow and Purple Cloud is a MACD on chart
and the bars show low activity with Blue and Orange
with higher movments as Green and Red.
Also if you turn off the indicator the color bars still remain so you can use other indicators and not have this in the way.
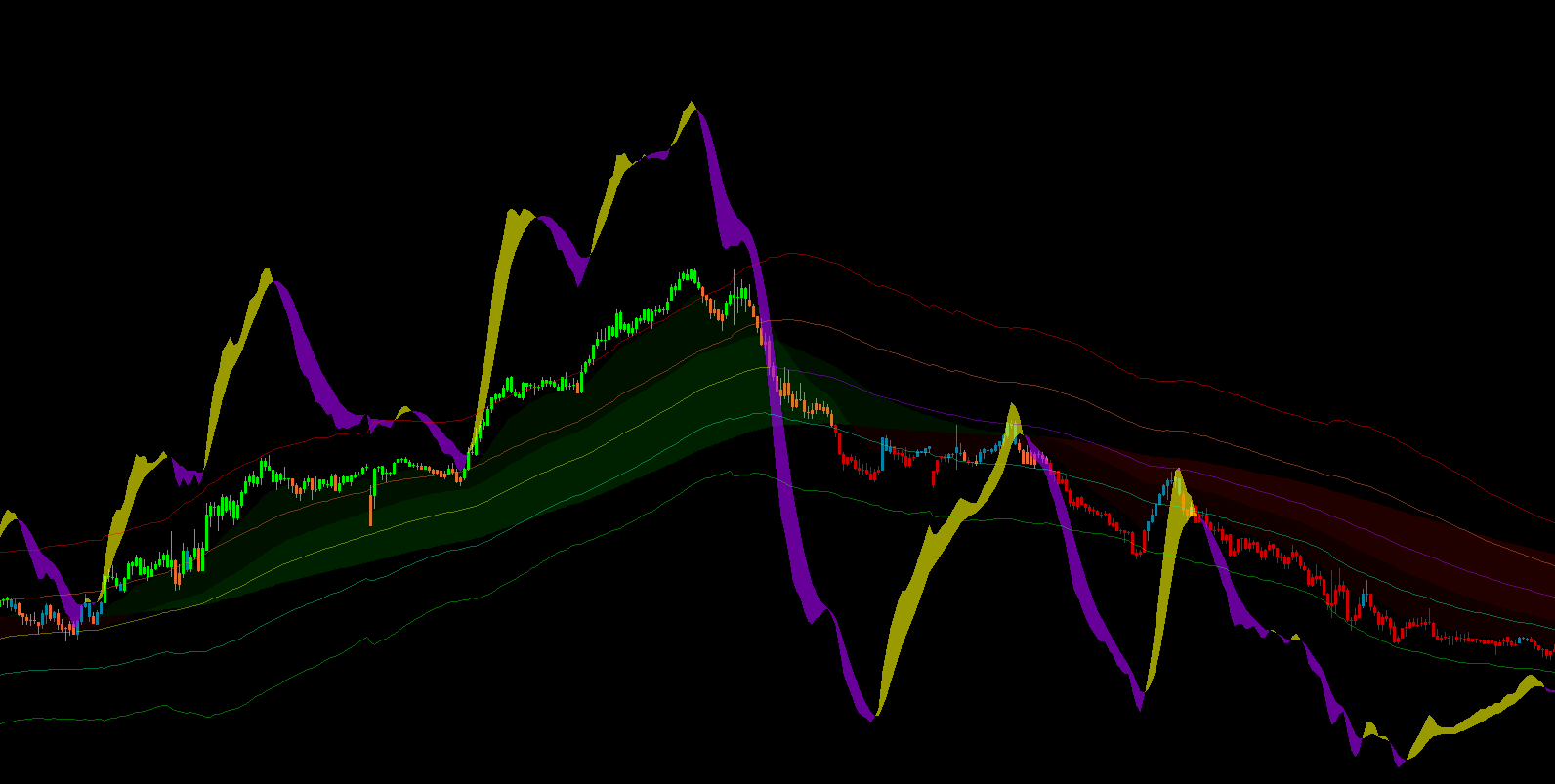
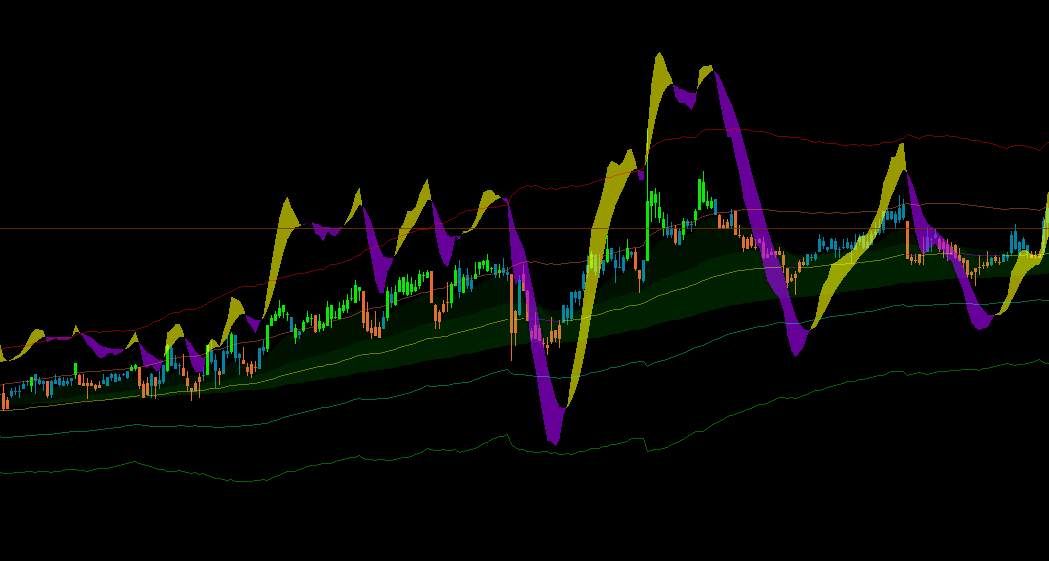
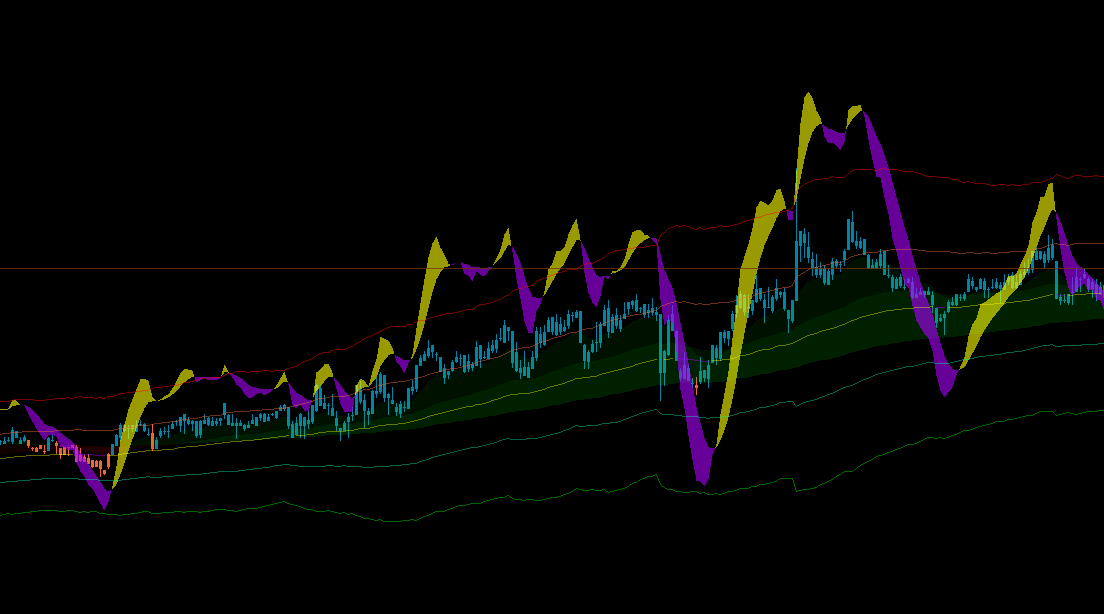
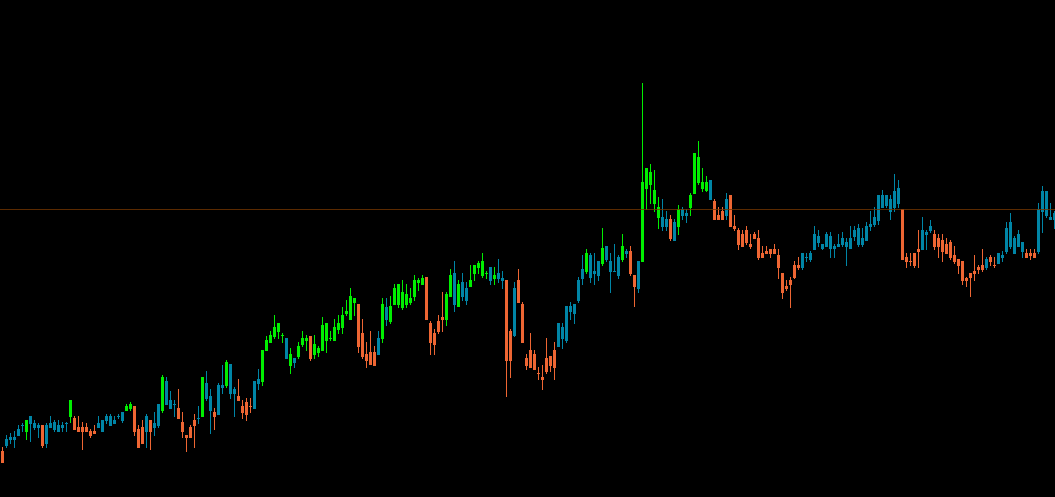
using cAlgo.API;
using cAlgo.API.Indicators;
namespace cAlgo
{
[Cloud("CU0", "CD2", Opacity = 1, FirstColor = "1100FF00", SecondColor = "11FF0000" )]
[Cloud("CU1", "CD2", Opacity = 1, FirstColor = "1100FF00", SecondColor = "11FF0000" )]
[Cloud ("Macd", "Signal", Opacity = 1, FirstColor = "99FFFF00", SecondColor = "99AA00FF")]
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class NIGHTMARESOC : Indicator
{
[Output("Macd" ,LineColor = "000000FF")] public IndicatorDataSeries Macd {get;set;}
[Output("Signal",LineColor = "00FF0000")] public IndicatorDataSeries Signal {get;set;}
[Parameter("MACD Cloud" , DefaultValue = MACDCloud.Yes, Group = "MACD Cloud" )] public MACDCloud MACC { get; set; }
private MacdCrossOver MACDC;
[Parameter ("MA Cloud" , DefaultValue = MACloud.Yes, Group = "MA Cloud" ) ]
public MACloud Cloud {get;set;}
[Output ("CU0" ,LineColor = "0000FF00")] public IndicatorDataSeries CU0 {get;set;}
[Output ("CU1" ,LineColor = "0000FF00")] public IndicatorDataSeries CU1 {get;set;}
[Output ("CD1" ,LineColor = "00FF0000")] public IndicatorDataSeries CD1 {get;set;}
[Output ("CD2" ,LineColor = "00FF0000")] public IndicatorDataSeries CD2 {get;set;}
[Parameter("Color Bars High or Low Volume ?" , DefaultValue = ColorBars.LowVolume , Group = "Color BARS" )]
public ColorBars CHB { get; set; }
[Output("Up ATR 1 cc" , LineColor = "6600FFBB" , PlotType = PlotType.DiscontinuousLine, Thickness = 1 )]
public IndicatorDataSeries UpATR1 { get; set; }
[Output("Dn ATR 1 ff" , LineColor = "77EE6633" , PlotType = PlotType.DiscontinuousLine, Thickness = 1 )]
public IndicatorDataSeries DnATR1 { get; set; }
[Output("Up ATR 2 ee" , LineColor = "6600FF00" , PlotType = PlotType.DiscontinuousLine, Thickness = 1 )]
public IndicatorDataSeries UpATR2 { get; set; }
[Output("Dn ATR 2 cc" , LineColor = "77FF0000" , PlotType = PlotType.DiscontinuousLine, Thickness = 1 )]
public IndicatorDataSeries DnATR2 { get; set; }
[Output("UpA1 66" , LineColor = "66FFFF00" , PlotType = PlotType.DiscontinuousLine, Thickness = 1 )]
public IndicatorDataSeries UpA1 { get; set; }
[Output("DnA1 66" , LineColor = "77AA00FF" , PlotType = PlotType.DiscontinuousLine, Thickness = 1 )]
public IndicatorDataSeries DnA1 { get; set; }
MovingAverage MA;
MovingAverage M1;
MovingAverage M2;
MovingAverage M3;
MovingAverage MATR;
AverageTrueRange ATR;
public enum ColorBars {LowVolume,HighVolume}
public enum MACloud {Yes,No}
public enum MACDCloud {Yes,No}
protected override void Initialize()
{
M1 = Indicators.WellesWilderSmoothing(Bars.ClosePrices,8 );
M2 = Indicators.WellesWilderSmoothing(Bars.ClosePrices,32 );
M3 = Indicators.WellesWilderSmoothing(Bars.ClosePrices,96 );
MACDC = Indicators.MacdCrossOver(26,12,9);
MA = Indicators.WellesWilderSmoothing(Bars.ClosePrices,14);
MATR = Indicators.WellesWilderSmoothing(Bars.ClosePrices,50 );
ATR = Indicators.AverageTrueRange (50,MovingAverageType.WilderSmoothing);
}
public override void Calculate(int index)
{
var X = MA.Result.Last(0);
if (MACC == MACDCloud.Yes)
{
Macd[index] = MACDC.MACD[index] * ((X*10)/X)+X;
Signal[index] = MACDC.Signal[index] * ((X*10)/X)+X;
}
var Aspace = ATR.Result.Last(0);
UpATR1[index] = MATR.Result[index] - (Aspace*3);
DnATR1[index] = MATR.Result[index] + (Aspace*3);
UpATR2[index] = MATR.Result[index] - (Aspace*7);
DnATR2[index] = MATR.Result[index] + (Aspace*7);
// Moving Average Cloud
if (Cloud == MACloud.Yes)
{
CU0[index] = M1.Result.Last(0);
CU1[index] = M2.Result.Last(0);
CD1[index] = M2.Result.Last(0);
CD2[index] = M3.Result.Last(0);
}
// ATR Bands
if (MATR.Result.Last(0) >= MATR.Result.Last(1))
{ UpA1[index] = MATR.Result[index]; }
if (MATR.Result.Last(0) <= MATR.Result.Last(1))
{ DnA1[index] = MATR.Result[index]; }
// Color Bars
if (CHB == ColorBars.LowVolume)
{
if ( Bars.ClosePrices.Last(0) > DnATR1.Last(0))
Chart.SetBarColor(index, "EE00FF00" );
if ( Bars.ClosePrices.Last(0) < UpATR1.Last(0))
Chart.SetBarColor(index, "CCFF0000" );
if ( Bars.ClosePrices.Last(0) > M1.Result.Last(0) && Bars.ClosePrices.Last(0) < DnATR1.Last(0))
Chart.SetBarColor(index, "A600CCFF" );
if ( Bars.ClosePrices.Last(0) < M1.Result.Last(0) && Bars.ClosePrices.Last(0) > UpATR1.Last(0))
Chart.SetBarColor(index, "FFEE6633" );
}
if (CHB == ColorBars.HighVolume)
{
if ( Bars.ClosePrices.Last(0) > DnATR2.Last(0))
Chart.SetBarColor(index, "EE00FF00" );
if ( Bars.ClosePrices.Last(0) < UpATR2.Last(0))
Chart.SetBarColor(index, "CCFF0000" );
if ( Bars.ClosePrices.Last(0) > M3.Result.Last(0) && Bars.ClosePrices.Last(0) < DnATR2.Last(0))
Chart.SetBarColor(index, "A600CCFF" );
if ( Bars.ClosePrices.Last(0) < M3.Result.Last(0) && Bars.ClosePrices.Last(0) > UpATR2.Last(0))
Chart.SetBarColor(index, "FFEE6633" );
}
}
}
}
VEI5S6C4OUNT0
Joined on 06.12.2022
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: NIGHTMARES ON CHART.algo
- Rating: 5
- Installs: 473
Comments
Up Date Change to line 7 , 11, 12 for MACD Cloud to make this more palatable and adjustable

top de mas parabens